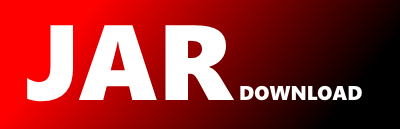
com.pulumi.azure.lb.kotlin.NatRule.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.lb.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
/**
* Builder for [NatRule].
*/
@PulumiTagMarker
public class NatRuleResourceBuilder internal constructor() {
public var name: String? = null
public var args: NatRuleArgs = NatRuleArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend NatRuleArgsBuilder.() -> Unit) {
val builder = NatRuleArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): NatRule {
val builtJavaResource = com.pulumi.azure.lb.NatRule(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return NatRule(builtJavaResource)
}
}
/**
* Manages a Load Balancer NAT Rule.
* > **NOTE:** This resource cannot be used with with virtual machine scale sets, instead use the `azure.lb.NatPool` resource.
* > **NOTE** When using this resource, the Load Balancer needs to have a FrontEnd IP Configuration Attached
* ## Example Usage
*
* ```yaml
* resources:
* example:
* type: azure:core:ResourceGroup
* properties:
* name: LoadBalancerRG
* location: West Europe
* examplePublicIp:
* type: azure:network:PublicIp
* name: example
* properties:
* name: PublicIPForLB
* location: West US
* resourceGroupName: ${example.name}
* allocationMethod: Static
* exampleLoadBalancer:
* type: azure:lb:LoadBalancer
* name: example
* properties:
* name: TestLoadBalancer
* location: West US
* resourceGroupName: ${example.name}
* frontendIpConfigurations:
* - name: PublicIPAddress
* publicIpAddressId: ${examplePublicIp.id}
* exampleBackendAddressPool:
* type: azure:lb:BackendAddressPool
* name: example
* properties:
* resourceGroupName: ${example.name}
* loadbalancerId: ${exampleLoadBalancer.id}
* name: be
* exampleNatRule:
* type: azure:lb:NatRule
* name: example
* properties:
* resourceGroupName: ${example.name}
* loadbalancerId: ${exampleLoadBalancer.id}
* name: RDPAccess
* protocol: Tcp
* frontendPort: 3389
* backendPort: 3389
* frontendIpConfigurationName: PublicIPAddress
* example1:
* type: azure:lb:NatRule
* properties:
* resourceGroupName: ${example.name}
* loadbalancerId: ${exampleLoadBalancer.id}
* name: RDPAccess
* protocol: Tcp
* frontendPortStart: 3000
* frontendPortEnd: 3389
* backendPort: 3389
* backendAddressPoolId: ${exampleBackendAddressPool.id}
* frontendIpConfigurationName: PublicIPAddress
* ```
*
* ## Import
* Load Balancer NAT Rules can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:lb/natRule:NatRule example /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/group1/providers/Microsoft.Network/loadBalancers/lb1/inboundNatRules/rule1
* ```
*/
public class NatRule internal constructor(
override val javaResource: com.pulumi.azure.lb.NatRule,
) : KotlinCustomResource(javaResource, NatRuleMapper) {
/**
* Specifies a reference to backendAddressPool resource.
*/
public val backendAddressPoolId: Output?
get() = javaResource.backendAddressPoolId().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
public val backendIpConfigurationId: Output
get() = javaResource.backendIpConfigurationId().applyValue({ args0 -> args0 })
/**
* The port used for internal connections on the endpoint. Possible values range between 1 and 65535, inclusive.
*/
public val backendPort: Output
get() = javaResource.backendPort().applyValue({ args0 -> args0 })
/**
* Are the Floating IPs enabled for this Load Balancer Rule? A "floating” IP is reassigned to a secondary server in case the primary server fails. Required to configure a SQL AlwaysOn Availability Group. Defaults to `false`.
*/
public val enableFloatingIp: Output
get() = javaResource.enableFloatingIp().applyValue({ args0 -> args0 })
/**
* Is TCP Reset enabled for this Load Balancer Rule?
*/
public val enableTcpReset: Output?
get() = javaResource.enableTcpReset().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
public val frontendIpConfigurationId: Output
get() = javaResource.frontendIpConfigurationId().applyValue({ args0 -> args0 })
/**
* The name of the frontend IP configuration exposing this rule.
*/
public val frontendIpConfigurationName: Output
get() = javaResource.frontendIpConfigurationName().applyValue({ args0 -> args0 })
/**
* The port for the external endpoint. Port numbers for each Rule must be unique within the Load Balancer. Possible values range between 1 and 65534, inclusive.
*/
public val frontendPort: Output?
get() = javaResource.frontendPort().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The port range end for the external endpoint. This property is used together with BackendAddressPool and FrontendPortRangeStart. Individual inbound NAT rule port mappings will be created for each backend address from BackendAddressPool. Acceptable values range from 1 to 65534, inclusive.
*/
public val frontendPortEnd: Output?
get() = javaResource.frontendPortEnd().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The port range start for the external endpoint. This property is used together with BackendAddressPool and FrontendPortRangeEnd. Individual inbound NAT rule port mappings will be created for each backend address from BackendAddressPool. Acceptable values range from 1 to 65534, inclusive.
*/
public val frontendPortStart: Output?
get() = javaResource.frontendPortStart().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Specifies the idle timeout in minutes for TCP connections. Valid values are between `4` and `30` minutes. Defaults to `4` minutes.
*/
public val idleTimeoutInMinutes: Output
get() = javaResource.idleTimeoutInMinutes().applyValue({ args0 -> args0 })
/**
* The ID of the Load Balancer in which to create the NAT Rule. Changing this forces a new resource to be created.
*/
public val loadbalancerId: Output
get() = javaResource.loadbalancerId().applyValue({ args0 -> args0 })
/**
* Specifies the name of the NAT Rule. Changing this forces a new resource to be created.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* The transport protocol for the external endpoint. Possible values are `Udp`, `Tcp` or `All`.
*/
public val protocol: Output
get() = javaResource.protocol().applyValue({ args0 -> args0 })
/**
* The name of the resource group in which to create the resource. Changing this forces a new resource to be created.
*/
public val resourceGroupName: Output
get() = javaResource.resourceGroupName().applyValue({ args0 -> args0 })
}
public object NatRuleMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.azure.lb.NatRule::class == javaResource::class
override fun map(javaResource: Resource): NatRule = NatRule(
javaResource as
com.pulumi.azure.lb.NatRule,
)
}
/**
* @see [NatRule].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [NatRule].
*/
public suspend fun natRule(name: String, block: suspend NatRuleResourceBuilder.() -> Unit): NatRule {
val builder = NatRuleResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [NatRule].
* @param name The _unique_ name of the resulting resource.
*/
public fun natRule(name: String): NatRule {
val builder = NatRuleResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy