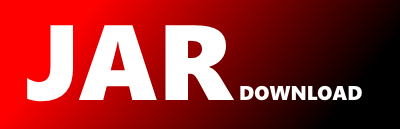
com.pulumi.azure.lb.kotlin.inputs.LoadBalancerFrontendIpConfigurationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.lb.kotlin.inputs
import com.pulumi.azure.lb.inputs.LoadBalancerFrontendIpConfigurationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property gatewayLoadBalancerFrontendIpConfigurationId The Frontend IP Configuration ID of a Gateway SKU Load Balancer.
* @property id The id of the Frontend IP Configuration.
* @property inboundNatRules The list of IDs of inbound rules that use this frontend IP.
* @property loadBalancerRules The list of IDs of load balancing rules that use this frontend IP.
* @property name Specifies the name of the frontend IP configuration.
* @property outboundRules The list of IDs outbound rules that use this frontend IP.
* @property privateIpAddress Private IP Address to assign to the Load Balancer. The last one and first four IPs in any range are reserved and cannot be manually assigned.
* @property privateIpAddressAllocation The allocation method for the Private IP Address used by this Load Balancer. Possible values as `Dynamic` and `Static`.
* @property privateIpAddressVersion The version of IP that the Private IP Address is. Possible values are `IPv4` or `IPv6`.
* @property publicIpAddressId The ID of a Public IP Address which should be associated with the Load Balancer.
* @property publicIpPrefixId The ID of a Public IP Prefix which should be associated with the Load Balancer. Public IP Prefix can only be used with outbound rules.
* @property subnetId The ID of the Subnet which should be associated with the IP Configuration.
* @property zones Specifies a list of Availability Zones in which the IP Address for this Load Balancer should be located.
* > **NOTE:** Availability Zones are only supported with a [Standard SKU](https://docs.microsoft.com/azure/load-balancer/load-balancer-standard-availability-zones) and [in select regions](https://docs.microsoft.com/azure/availability-zones/az-overview) at this time.
*/
public data class LoadBalancerFrontendIpConfigurationArgs(
public val gatewayLoadBalancerFrontendIpConfigurationId: Output? = null,
public val id: Output? = null,
public val inboundNatRules: Output>? = null,
public val loadBalancerRules: Output>? = null,
public val name: Output,
public val outboundRules: Output>? = null,
public val privateIpAddress: Output? = null,
public val privateIpAddressAllocation: Output? = null,
public val privateIpAddressVersion: Output? = null,
public val publicIpAddressId: Output? = null,
public val publicIpPrefixId: Output? = null,
public val subnetId: Output? = null,
public val zones: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.lb.inputs.LoadBalancerFrontendIpConfigurationArgs =
com.pulumi.azure.lb.inputs.LoadBalancerFrontendIpConfigurationArgs.builder()
.gatewayLoadBalancerFrontendIpConfigurationId(
gatewayLoadBalancerFrontendIpConfigurationId?.applyValue({ args0 ->
args0
}),
)
.id(id?.applyValue({ args0 -> args0 }))
.inboundNatRules(inboundNatRules?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.loadBalancerRules(loadBalancerRules?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.name(name.applyValue({ args0 -> args0 }))
.outboundRules(outboundRules?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.privateIpAddress(privateIpAddress?.applyValue({ args0 -> args0 }))
.privateIpAddressAllocation(privateIpAddressAllocation?.applyValue({ args0 -> args0 }))
.privateIpAddressVersion(privateIpAddressVersion?.applyValue({ args0 -> args0 }))
.publicIpAddressId(publicIpAddressId?.applyValue({ args0 -> args0 }))
.publicIpPrefixId(publicIpPrefixId?.applyValue({ args0 -> args0 }))
.subnetId(subnetId?.applyValue({ args0 -> args0 }))
.zones(zones?.applyValue({ args0 -> args0.map({ args0 -> args0 }) })).build()
}
/**
* Builder for [LoadBalancerFrontendIpConfigurationArgs].
*/
@PulumiTagMarker
public class LoadBalancerFrontendIpConfigurationArgsBuilder internal constructor() {
private var gatewayLoadBalancerFrontendIpConfigurationId: Output? = null
private var id: Output? = null
private var inboundNatRules: Output>? = null
private var loadBalancerRules: Output>? = null
private var name: Output? = null
private var outboundRules: Output>? = null
private var privateIpAddress: Output? = null
private var privateIpAddressAllocation: Output? = null
private var privateIpAddressVersion: Output? = null
private var publicIpAddressId: Output? = null
private var publicIpPrefixId: Output? = null
private var subnetId: Output? = null
private var zones: Output>? = null
/**
* @param value The Frontend IP Configuration ID of a Gateway SKU Load Balancer.
*/
@JvmName("yoffmnorunbiadtg")
public suspend fun gatewayLoadBalancerFrontendIpConfigurationId(`value`: Output) {
this.gatewayLoadBalancerFrontendIpConfigurationId = value
}
/**
* @param value The id of the Frontend IP Configuration.
*/
@JvmName("dbqedvacejeiuflu")
public suspend fun id(`value`: Output) {
this.id = value
}
/**
* @param value The list of IDs of inbound rules that use this frontend IP.
*/
@JvmName("johglqhhxratfjio")
public suspend fun inboundNatRules(`value`: Output>) {
this.inboundNatRules = value
}
@JvmName("srtbdfhmrdhngfhr")
public suspend fun inboundNatRules(vararg values: Output) {
this.inboundNatRules = Output.all(values.asList())
}
/**
* @param values The list of IDs of inbound rules that use this frontend IP.
*/
@JvmName("suonhqdtjuhwnghw")
public suspend fun inboundNatRules(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy