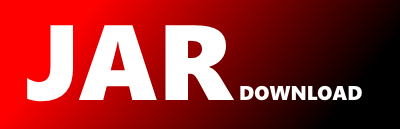
com.pulumi.azure.machinelearning.kotlin.inputs.InferenceClusterSslArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.machinelearning.kotlin.inputs
import com.pulumi.azure.machinelearning.inputs.InferenceClusterSslArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property cert The certificate for the SSL configuration.Conflicts with `ssl[0].leaf_domain_label`,`ssl[0].overwrite_existing_domain`. Changing this forces a new Machine Learning Inference Cluster to be created. Defaults to `""`.
* @property cname The cname of the SSL configuration.Conflicts with `ssl[0].leaf_domain_label`,`ssl[0].overwrite_existing_domain`. Changing this forces a new Machine Learning Inference Cluster to be created. Defaults to `""`.
* @property key The key content for the SSL configuration.Conflicts with `ssl[0].leaf_domain_label`,`ssl[0].overwrite_existing_domain`. Changing this forces a new Machine Learning Inference Cluster to be created. Defaults to `""`.
* @property leafDomainLabel The leaf domain label for the SSL configuration. Conflicts with `ssl[0].cert`,`ssl[0].key`,`ssl[0].cname`. Changing this forces a new Machine Learning Inference Cluster to be created. Defaults to `""`.
* @property overwriteExistingDomain Whether or not to overwrite existing leaf domain. Conflicts with `ssl[0].cert`,`ssl[0].key`,`ssl[0].cname` Changing this forces a new Machine Learning Inference Cluster to be created. Defaults to `""`.
*/
public data class InferenceClusterSslArgs(
public val cert: Output? = null,
public val cname: Output? = null,
public val key: Output? = null,
public val leafDomainLabel: Output? = null,
public val overwriteExistingDomain: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.machinelearning.inputs.InferenceClusterSslArgs =
com.pulumi.azure.machinelearning.inputs.InferenceClusterSslArgs.builder()
.cert(cert?.applyValue({ args0 -> args0 }))
.cname(cname?.applyValue({ args0 -> args0 }))
.key(key?.applyValue({ args0 -> args0 }))
.leafDomainLabel(leafDomainLabel?.applyValue({ args0 -> args0 }))
.overwriteExistingDomain(overwriteExistingDomain?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [InferenceClusterSslArgs].
*/
@PulumiTagMarker
public class InferenceClusterSslArgsBuilder internal constructor() {
private var cert: Output? = null
private var cname: Output? = null
private var key: Output? = null
private var leafDomainLabel: Output? = null
private var overwriteExistingDomain: Output? = null
/**
* @param value The certificate for the SSL configuration.Conflicts with `ssl[0].leaf_domain_label`,`ssl[0].overwrite_existing_domain`. Changing this forces a new Machine Learning Inference Cluster to be created. Defaults to `""`.
*/
@JvmName("towaedajxymmorda")
public suspend fun cert(`value`: Output) {
this.cert = value
}
/**
* @param value The cname of the SSL configuration.Conflicts with `ssl[0].leaf_domain_label`,`ssl[0].overwrite_existing_domain`. Changing this forces a new Machine Learning Inference Cluster to be created. Defaults to `""`.
*/
@JvmName("xgkibpwqaunllkmh")
public suspend fun cname(`value`: Output) {
this.cname = value
}
/**
* @param value The key content for the SSL configuration.Conflicts with `ssl[0].leaf_domain_label`,`ssl[0].overwrite_existing_domain`. Changing this forces a new Machine Learning Inference Cluster to be created. Defaults to `""`.
*/
@JvmName("xqsyyftkhuittplg")
public suspend fun key(`value`: Output) {
this.key = value
}
/**
* @param value The leaf domain label for the SSL configuration. Conflicts with `ssl[0].cert`,`ssl[0].key`,`ssl[0].cname`. Changing this forces a new Machine Learning Inference Cluster to be created. Defaults to `""`.
*/
@JvmName("yemmqohbqcdnlpnt")
public suspend fun leafDomainLabel(`value`: Output) {
this.leafDomainLabel = value
}
/**
* @param value Whether or not to overwrite existing leaf domain. Conflicts with `ssl[0].cert`,`ssl[0].key`,`ssl[0].cname` Changing this forces a new Machine Learning Inference Cluster to be created. Defaults to `""`.
*/
@JvmName("ofdtyjjksorpwhme")
public suspend fun overwriteExistingDomain(`value`: Output) {
this.overwriteExistingDomain = value
}
/**
* @param value The certificate for the SSL configuration.Conflicts with `ssl[0].leaf_domain_label`,`ssl[0].overwrite_existing_domain`. Changing this forces a new Machine Learning Inference Cluster to be created. Defaults to `""`.
*/
@JvmName("ydkvbryykshkxlyu")
public suspend fun cert(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cert = mapped
}
/**
* @param value The cname of the SSL configuration.Conflicts with `ssl[0].leaf_domain_label`,`ssl[0].overwrite_existing_domain`. Changing this forces a new Machine Learning Inference Cluster to be created. Defaults to `""`.
*/
@JvmName("brmdkeewduyjfsfm")
public suspend fun cname(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cname = mapped
}
/**
* @param value The key content for the SSL configuration.Conflicts with `ssl[0].leaf_domain_label`,`ssl[0].overwrite_existing_domain`. Changing this forces a new Machine Learning Inference Cluster to be created. Defaults to `""`.
*/
@JvmName("wlhxtfgbcaawddqx")
public suspend fun key(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.key = mapped
}
/**
* @param value The leaf domain label for the SSL configuration. Conflicts with `ssl[0].cert`,`ssl[0].key`,`ssl[0].cname`. Changing this forces a new Machine Learning Inference Cluster to be created. Defaults to `""`.
*/
@JvmName("nwqdophxhxejkyxv")
public suspend fun leafDomainLabel(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.leafDomainLabel = mapped
}
/**
* @param value Whether or not to overwrite existing leaf domain. Conflicts with `ssl[0].cert`,`ssl[0].key`,`ssl[0].cname` Changing this forces a new Machine Learning Inference Cluster to be created. Defaults to `""`.
*/
@JvmName("wmrsgmwqgntcymhk")
public suspend fun overwriteExistingDomain(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.overwriteExistingDomain = mapped
}
internal fun build(): InferenceClusterSslArgs = InferenceClusterSslArgs(
cert = cert,
cname = cname,
key = key,
leafDomainLabel = leafDomainLabel,
overwriteExistingDomain = overwriteExistingDomain,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy