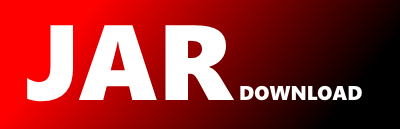
com.pulumi.azure.maintenance.kotlin.inputs.ConfigurationWindowArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.maintenance.kotlin.inputs
import com.pulumi.azure.maintenance.inputs.ConfigurationWindowArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property duration The duration of the maintenance window in HH:mm format.
* @property expirationDateTime Effective expiration date of the maintenance window in YYYY-MM-DD hh:mm format.
* @property recurEvery The rate at which a maintenance window is expected to recur. The rate can be expressed as daily, weekly, or monthly schedules.
* @property startDateTime Effective start date of the maintenance window in YYYY-MM-DD hh:mm format.
* @property timeZone The time zone for the maintenance window. A list of timezones can be obtained by executing [System.TimeZoneInfo]::GetSystemTimeZones() in PowerShell.
*/
public data class ConfigurationWindowArgs(
public val duration: Output? = null,
public val expirationDateTime: Output? = null,
public val recurEvery: Output? = null,
public val startDateTime: Output,
public val timeZone: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.maintenance.inputs.ConfigurationWindowArgs =
com.pulumi.azure.maintenance.inputs.ConfigurationWindowArgs.builder()
.duration(duration?.applyValue({ args0 -> args0 }))
.expirationDateTime(expirationDateTime?.applyValue({ args0 -> args0 }))
.recurEvery(recurEvery?.applyValue({ args0 -> args0 }))
.startDateTime(startDateTime.applyValue({ args0 -> args0 }))
.timeZone(timeZone.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ConfigurationWindowArgs].
*/
@PulumiTagMarker
public class ConfigurationWindowArgsBuilder internal constructor() {
private var duration: Output? = null
private var expirationDateTime: Output? = null
private var recurEvery: Output? = null
private var startDateTime: Output? = null
private var timeZone: Output? = null
/**
* @param value The duration of the maintenance window in HH:mm format.
*/
@JvmName("gaxctqlxlkelfmuk")
public suspend fun duration(`value`: Output) {
this.duration = value
}
/**
* @param value Effective expiration date of the maintenance window in YYYY-MM-DD hh:mm format.
*/
@JvmName("nswxgwkjedvdhidd")
public suspend fun expirationDateTime(`value`: Output) {
this.expirationDateTime = value
}
/**
* @param value The rate at which a maintenance window is expected to recur. The rate can be expressed as daily, weekly, or monthly schedules.
*/
@JvmName("ktwojdhkouvmhroy")
public suspend fun recurEvery(`value`: Output) {
this.recurEvery = value
}
/**
* @param value Effective start date of the maintenance window in YYYY-MM-DD hh:mm format.
*/
@JvmName("vuvgoivyhapgxqcp")
public suspend fun startDateTime(`value`: Output) {
this.startDateTime = value
}
/**
* @param value The time zone for the maintenance window. A list of timezones can be obtained by executing [System.TimeZoneInfo]::GetSystemTimeZones() in PowerShell.
*/
@JvmName("msyxdogluhmtmsst")
public suspend fun timeZone(`value`: Output) {
this.timeZone = value
}
/**
* @param value The duration of the maintenance window in HH:mm format.
*/
@JvmName("ctnxblxofnewneby")
public suspend fun duration(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.duration = mapped
}
/**
* @param value Effective expiration date of the maintenance window in YYYY-MM-DD hh:mm format.
*/
@JvmName("tvcoeiaabxfmxciv")
public suspend fun expirationDateTime(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.expirationDateTime = mapped
}
/**
* @param value The rate at which a maintenance window is expected to recur. The rate can be expressed as daily, weekly, or monthly schedules.
*/
@JvmName("gxjqmyfkqcnkkexx")
public suspend fun recurEvery(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.recurEvery = mapped
}
/**
* @param value Effective start date of the maintenance window in YYYY-MM-DD hh:mm format.
*/
@JvmName("uutdwlkhodyioomn")
public suspend fun startDateTime(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.startDateTime = mapped
}
/**
* @param value The time zone for the maintenance window. A list of timezones can be obtained by executing [System.TimeZoneInfo]::GetSystemTimeZones() in PowerShell.
*/
@JvmName("jrrxpbtldjyghesl")
public suspend fun timeZone(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.timeZone = mapped
}
internal fun build(): ConfigurationWindowArgs = ConfigurationWindowArgs(
duration = duration,
expirationDateTime = expirationDateTime,
recurEvery = recurEvery,
startDateTime = startDateTime ?: throw PulumiNullFieldException("startDateTime"),
timeZone = timeZone ?: throw PulumiNullFieldException("timeZone"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy