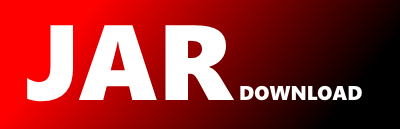
com.pulumi.azure.managedapplication.kotlin.ApplicationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.managedapplication.kotlin
import com.pulumi.azure.managedapplication.ApplicationArgs.builder
import com.pulumi.azure.managedapplication.kotlin.inputs.ApplicationPlanArgs
import com.pulumi.azure.managedapplication.kotlin.inputs.ApplicationPlanArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Deprecated
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Manages a Managed Application.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* import * as std from "@pulumi/std";
* const current = azure.core.getClientConfig({});
* const builtin = azure.authorization.getRoleDefinition({
* name: "Contributor",
* });
* const example = new azure.core.ResourceGroup("example", {
* name: "example-resources",
* location: "West Europe",
* });
* const exampleDefinition = new azure.managedapplication.Definition("example", {
* name: "examplemanagedapplicationdefinition",
* location: example.location,
* resourceGroupName: example.name,
* lockLevel: "ReadOnly",
* packageFileUri: "https://github.com/Azure/azure-managedapp-samples/raw/master/Managed Application Sample Packages/201-managed-storage-account/managedstorage.zip",
* displayName: "TestManagedAppDefinition",
* description: "Test Managed App Definition",
* authorizations: [{
* servicePrincipalId: current.then(current => current.objectId),
* roleDefinitionId: Promise.all([builtin.then(builtin => std.split({
* separator: "/",
* text: builtin.id,
* })), builtin.then(builtin => std.split({
* separator: "/",
* text: builtin.id,
* })).then(invoke => invoke.result).length]).then(([invoke, length]) => invoke.result[length - 1]),
* }],
* });
* const exampleApplication = new azure.managedapplication.Application("example", {
* name: "example-managedapplication",
* location: example.location,
* resourceGroupName: example.name,
* kind: "ServiceCatalog",
* managedResourceGroupName: "infrastructureGroup",
* applicationDefinitionId: exampleDefinition.id,
* parameterValues: pulumi.jsonStringify({
* location: {
* value: example.location,
* },
* storageAccountNamePrefix: {
* value: "storeNamePrefix",
* },
* storageAccountType: {
* value: "Standard_LRS",
* },
* }),
* });
* ```
* ```python
* import pulumi
* import json
* import pulumi_azure as azure
* import pulumi_std as std
* current = azure.core.get_client_config()
* builtin = azure.authorization.get_role_definition(name="Contributor")
* example = azure.core.ResourceGroup("example",
* name="example-resources",
* location="West Europe")
* example_definition = azure.managedapplication.Definition("example",
* name="examplemanagedapplicationdefinition",
* location=example.location,
* resource_group_name=example.name,
* lock_level="ReadOnly",
* package_file_uri="https://github.com/Azure/azure-managedapp-samples/raw/master/Managed Application Sample Packages/201-managed-storage-account/managedstorage.zip",
* display_name="TestManagedAppDefinition",
* description="Test Managed App Definition",
* authorizations=[{
* "service_principal_id": current.object_id,
* "role_definition_id": std.split(separator="/",
* text=builtin.id).result[len(std.split(separator="/",
* text=builtin.id).result) - 1],
* }])
* example_application = azure.managedapplication.Application("example",
* name="example-managedapplication",
* location=example.location,
* resource_group_name=example.name,
* kind="ServiceCatalog",
* managed_resource_group_name="infrastructureGroup",
* application_definition_id=example_definition.id,
* parameter_values=pulumi.Output.json_dumps({
* "location": {
* "value": example.location,
* },
* "storageAccountNamePrefix": {
* "value": "storeNamePrefix",
* },
* "storageAccountType": {
* "value": "Standard_LRS",
* },
* }))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using System.Text.Json;
* using Pulumi;
* using Azure = Pulumi.Azure;
* using Std = Pulumi.Std;
* return await Deployment.RunAsync(() =>
* {
* var current = Azure.Core.GetClientConfig.Invoke();
* var builtin = Azure.Authorization.GetRoleDefinition.Invoke(new()
* {
* Name = "Contributor",
* });
* var example = new Azure.Core.ResourceGroup("example", new()
* {
* Name = "example-resources",
* Location = "West Europe",
* });
* var exampleDefinition = new Azure.ManagedApplication.Definition("example", new()
* {
* Name = "examplemanagedapplicationdefinition",
* Location = example.Location,
* ResourceGroupName = example.Name,
* LockLevel = "ReadOnly",
* PackageFileUri = "https://github.com/Azure/azure-managedapp-samples/raw/master/Managed Application Sample Packages/201-managed-storage-account/managedstorage.zip",
* DisplayName = "TestManagedAppDefinition",
* Description = "Test Managed App Definition",
* Authorizations = new[]
* {
* new Azure.ManagedApplication.Inputs.DefinitionAuthorizationArgs
* {
* ServicePrincipalId = current.Apply(getClientConfigResult => getClientConfigResult.ObjectId),
* RoleDefinitionId = Output.Tuple(Std.Split.Invoke(new()
* {
* Separator = "/",
* Text = builtin.Apply(getRoleDefinitionResult => getRoleDefinitionResult.Id),
* }), Std.Split.Invoke(new()
* {
* Separator = "/",
* Text = builtin.Apply(getRoleDefinitionResult => getRoleDefinitionResult.Id),
* }).Apply(invoke => invoke.Result).Length).Apply(values =>
* {
* var invoke = values.Item1;
* var length = values.Item2;
* return invoke.Result[length - 1];
* }),
* },
* },
* });
* var exampleApplication = new Azure.ManagedApplication.Application("example", new()
* {
* Name = "example-managedapplication",
* Location = example.Location,
* ResourceGroupName = example.Name,
* Kind = "ServiceCatalog",
* ManagedResourceGroupName = "infrastructureGroup",
* ApplicationDefinitionId = exampleDefinition.Id,
* ParameterValues = Output.JsonSerialize(Output.Create(new Dictionary
* {
* ["location"] = new Dictionary
* {
* ["value"] = example.Location,
* },
* ["storageAccountNamePrefix"] = new Dictionary
* {
* ["value"] = "storeNamePrefix",
* },
* ["storageAccountType"] = new Dictionary
* {
* ["value"] = "Standard_LRS",
* },
* })),
* });
* });
* ```
* ```go
* package main
* import (
* "encoding/json"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/authorization"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/core"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/managedapplication"
* "github.com/pulumi/pulumi-std/sdk/go/std"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* current, err := core.GetClientConfig(ctx, nil, nil)
* if err != nil {
* return err
* }
* builtin, err := authorization.LookupRoleDefinition(ctx, &authorization.LookupRoleDefinitionArgs{
* Name: pulumi.StringRef("Contributor"),
* }, nil)
* if err != nil {
* return err
* }
* example, err := core.NewResourceGroup(ctx, "example", &core.ResourceGroupArgs{
* Name: pulumi.String("example-resources"),
* Location: pulumi.String("West Europe"),
* })
* if err != nil {
* return err
* }
* invokeSplit, err := std.Split(ctx, &std.SplitArgs{
* Separator: "/",
* Text: builtin.Id,
* }, nil)
* if err != nil {
* return err
* }
* invokeSplit1, err := std.Split(ctx, &std.SplitArgs{
* Separator: "/",
* Text: builtin.Id,
* }, nil)
* if err != nil {
* return err
* }
* exampleDefinition, err := managedapplication.NewDefinition(ctx, "example", &managedapplication.DefinitionArgs{
* Name: pulumi.String("examplemanagedapplicationdefinition"),
* Location: example.Location,
* ResourceGroupName: example.Name,
* LockLevel: pulumi.String("ReadOnly"),
* PackageFileUri: pulumi.String("https://github.com/Azure/azure-managedapp-samples/raw/master/Managed Application Sample Packages/201-managed-storage-account/managedstorage.zip"),
* DisplayName: pulumi.String("TestManagedAppDefinition"),
* Description: pulumi.String("Test Managed App Definition"),
* Authorizations: managedapplication.DefinitionAuthorizationArray{
* &managedapplication.DefinitionAuthorizationArgs{
* ServicePrincipalId: pulumi.String(current.ObjectId),
* RoleDefinitionId: pulumi.String(invokeSplit.Result[float64(pulumi.Float64(len(invokeSplit1.Result))-1)]),
* },
* },
* })
* if err != nil {
* return err
* }
* _, err = managedapplication.NewApplication(ctx, "example", &managedapplication.ApplicationArgs{
* Name: pulumi.String("example-managedapplication"),
* Location: example.Location,
* ResourceGroupName: example.Name,
* Kind: pulumi.String("ServiceCatalog"),
* ManagedResourceGroupName: pulumi.String("infrastructureGroup"),
* ApplicationDefinitionId: exampleDefinition.ID(),
* ParameterValues: example.Location.ApplyT(func(location string) (pulumi.String, error) {
* var _zero pulumi.String
* tmpJSON0, err := json.Marshal(map[string]interface{}{
* "location": map[string]interface{}{
* "value": location,
* },
* "storageAccountNamePrefix": map[string]interface{}{
* "value": "storeNamePrefix",
* },
* "storageAccountType": map[string]interface{}{
* "value": "Standard_LRS",
* },
* })
* if err != nil {
* return _zero, err
* }
* json0 := string(tmpJSON0)
* return pulumi.String(json0), nil
* }).(pulumi.StringOutput),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.CoreFunctions;
* import com.pulumi.azure.authorization.AuthorizationFunctions;
* import com.pulumi.azure.authorization.inputs.GetRoleDefinitionArgs;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.managedapplication.Definition;
* import com.pulumi.azure.managedapplication.DefinitionArgs;
* import com.pulumi.azure.managedapplication.inputs.DefinitionAuthorizationArgs;
* import com.pulumi.azure.managedapplication.Application;
* import com.pulumi.azure.managedapplication.ApplicationArgs;
* import static com.pulumi.codegen.internal.Serialization.*;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var current = CoreFunctions.getClientConfig();
* final var builtin = AuthorizationFunctions.getRoleDefinition(GetRoleDefinitionArgs.builder()
* .name("Contributor")
* .build());
* var example = new ResourceGroup("example", ResourceGroupArgs.builder()
* .name("example-resources")
* .location("West Europe")
* .build());
* var exampleDefinition = new Definition("exampleDefinition", DefinitionArgs.builder()
* .name("examplemanagedapplicationdefinition")
* .location(example.location())
* .resourceGroupName(example.name())
* .lockLevel("ReadOnly")
* .packageFileUri("https://github.com/Azure/azure-managedapp-samples/raw/master/Managed Application Sample Packages/201-managed-storage-account/managedstorage.zip")
* .displayName("TestManagedAppDefinition")
* .description("Test Managed App Definition")
* .authorizations(DefinitionAuthorizationArgs.builder()
* .servicePrincipalId(current.applyValue(getClientConfigResult -> getClientConfigResult.objectId()))
* .roleDefinitionId(StdFunctions.split(SplitArgs.builder()
* .separator("/")
* .text(builtin.applyValue(getRoleDefinitionResult -> getRoleDefinitionResult.id()))
* .build()).result()[StdFunctions.split(SplitArgs.builder()
* .separator("/")
* .text(builtin.applyValue(getRoleDefinitionResult -> getRoleDefinitionResult.id()))
* .build()).result().length() - 1])
* .build())
* .build());
* var exampleApplication = new Application("exampleApplication", ApplicationArgs.builder()
* .name("example-managedapplication")
* .location(example.location())
* .resourceGroupName(example.name())
* .kind("ServiceCatalog")
* .managedResourceGroupName("infrastructureGroup")
* .applicationDefinitionId(exampleDefinition.id())
* .parameterValues(example.location().applyValue(location -> serializeJson(
* jsonObject(
* jsonProperty("location", jsonObject(
* jsonProperty("value", location)
* )),
* jsonProperty("storageAccountNamePrefix", jsonObject(
* jsonProperty("value", "storeNamePrefix")
* )),
* jsonProperty("storageAccountType", jsonObject(
* jsonProperty("value", "Standard_LRS")
* ))
* ))))
* .build());
* }
* }
* ```
*
* ## Import
* Managed Application can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:managedapplication/application:Application example /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/group1/providers/Microsoft.Solutions/applications/app1
* ```
* @property applicationDefinitionId The application definition ID to deploy.
* @property kind The kind of the managed application to deploy. Possible values are `MarketPlace` and `ServiceCatalog`. Changing this forces a new resource to be created.
* @property location Specifies the supported Azure location where the resource exists. Changing this forces a new resource to be created.
* @property managedResourceGroupName The name of the target resource group where all the resources deployed by the managed application will reside. Changing this forces a new resource to be created.
* @property name Specifies the name of the Managed Application. Changing this forces a new resource to be created.
* @property parameterValues The parameter values to pass to the Managed Application. This field is a JSON object that allows you to assign parameters to this Managed Application.
* @property parameters A mapping of name and value pairs to pass to the managed application as parameters.
* > **NOTE:** `parameters` only supports values with `string` or `secureString` type and will be deprecated in version 4.0 of the provider - please use `parameter_values` instead which supports more parameter types.
* @property plan One `plan` block as defined below. Changing this forces a new resource to be created.
* @property resourceGroupName The name of the Resource Group where the Managed Application should exist. Changing this forces a new resource to be created.
* @property tags A mapping of tags to assign to the resource.
*/
public data class ApplicationArgs(
public val applicationDefinitionId: Output? = null,
public val kind: Output? = null,
public val location: Output? = null,
public val managedResourceGroupName: Output? = null,
public val name: Output? = null,
public val parameterValues: Output? = null,
@Deprecated(
message = """
This property has been deprecated in favour of `parameter_values`
""",
)
public val parameters: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy