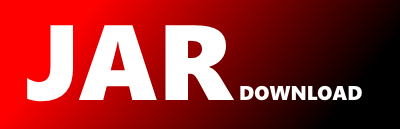
com.pulumi.azure.management.kotlin.GroupPolicyAssignmentArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.management.kotlin
import com.pulumi.azure.management.GroupPolicyAssignmentArgs.builder
import com.pulumi.azure.management.kotlin.inputs.GroupPolicyAssignmentIdentityArgs
import com.pulumi.azure.management.kotlin.inputs.GroupPolicyAssignmentIdentityArgsBuilder
import com.pulumi.azure.management.kotlin.inputs.GroupPolicyAssignmentNonComplianceMessageArgs
import com.pulumi.azure.management.kotlin.inputs.GroupPolicyAssignmentNonComplianceMessageArgsBuilder
import com.pulumi.azure.management.kotlin.inputs.GroupPolicyAssignmentOverrideArgs
import com.pulumi.azure.management.kotlin.inputs.GroupPolicyAssignmentOverrideArgsBuilder
import com.pulumi.azure.management.kotlin.inputs.GroupPolicyAssignmentResourceSelectorArgs
import com.pulumi.azure.management.kotlin.inputs.GroupPolicyAssignmentResourceSelectorArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Manages a Policy Assignment to a Management Group.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = new azure.management.Group("example", {displayName: "Some Management Group"});
* const exampleDefinition = new azure.policy.Definition("example", {
* name: "only-deploy-in-westeurope",
* policyType: "Custom",
* mode: "All",
* displayName: "my-policy-definition",
* managementGroupId: example.id,
* policyRule: ` {
* "if": {
* "not": {
* "field": "location",
* "equals": "westeurope"
* }
* },
* "then": {
* "effect": "Deny"
* }
* }
* `,
* });
* const exampleGroupPolicyAssignment = new azure.management.GroupPolicyAssignment("example", {
* name: "example-policy",
* policyDefinitionId: exampleDefinition.id,
* managementGroupId: example.id,
* });
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.management.Group("example", display_name="Some Management Group")
* example_definition = azure.policy.Definition("example",
* name="only-deploy-in-westeurope",
* policy_type="Custom",
* mode="All",
* display_name="my-policy-definition",
* management_group_id=example.id,
* policy_rule=""" {
* "if": {
* "not": {
* "field": "location",
* "equals": "westeurope"
* }
* },
* "then": {
* "effect": "Deny"
* }
* }
* """)
* example_group_policy_assignment = azure.management.GroupPolicyAssignment("example",
* name="example-policy",
* policy_definition_id=example_definition.id,
* management_group_id=example.id)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = new Azure.Management.Group("example", new()
* {
* DisplayName = "Some Management Group",
* });
* var exampleDefinition = new Azure.Policy.Definition("example", new()
* {
* Name = "only-deploy-in-westeurope",
* PolicyType = "Custom",
* Mode = "All",
* DisplayName = "my-policy-definition",
* ManagementGroupId = example.Id,
* PolicyRule = @" {
* ""if"": {
* ""not"": {
* ""field"": ""location"",
* ""equals"": ""westeurope""
* }
* },
* ""then"": {
* ""effect"": ""Deny""
* }
* }
* ",
* });
* var exampleGroupPolicyAssignment = new Azure.Management.GroupPolicyAssignment("example", new()
* {
* Name = "example-policy",
* PolicyDefinitionId = exampleDefinition.Id,
* ManagementGroupId = example.Id,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/management"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/policy"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := management.NewGroup(ctx, "example", &management.GroupArgs{
* DisplayName: pulumi.String("Some Management Group"),
* })
* if err != nil {
* return err
* }
* exampleDefinition, err := policy.NewDefinition(ctx, "example", &policy.DefinitionArgs{
* Name: pulumi.String("only-deploy-in-westeurope"),
* PolicyType: pulumi.String("Custom"),
* Mode: pulumi.String("All"),
* DisplayName: pulumi.String("my-policy-definition"),
* ManagementGroupId: example.ID(),
* PolicyRule: pulumi.String(` {
* "if": {
* "not": {
* "field": "location",
* "equals": "westeurope"
* }
* },
* "then": {
* "effect": "Deny"
* }
* }
* `),
* })
* if err != nil {
* return err
* }
* _, err = management.NewGroupPolicyAssignment(ctx, "example", &management.GroupPolicyAssignmentArgs{
* Name: pulumi.String("example-policy"),
* PolicyDefinitionId: exampleDefinition.ID(),
* ManagementGroupId: example.ID(),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.management.Group;
* import com.pulumi.azure.management.GroupArgs;
* import com.pulumi.azure.policy.Definition;
* import com.pulumi.azure.policy.DefinitionArgs;
* import com.pulumi.azure.management.GroupPolicyAssignment;
* import com.pulumi.azure.management.GroupPolicyAssignmentArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Group("example", GroupArgs.builder()
* .displayName("Some Management Group")
* .build());
* var exampleDefinition = new Definition("exampleDefinition", DefinitionArgs.builder()
* .name("only-deploy-in-westeurope")
* .policyType("Custom")
* .mode("All")
* .displayName("my-policy-definition")
* .managementGroupId(example.id())
* .policyRule("""
* {
* "if": {
* "not": {
* "field": "location",
* "equals": "westeurope"
* }
* },
* "then": {
* "effect": "Deny"
* }
* }
* """)
* .build());
* var exampleGroupPolicyAssignment = new GroupPolicyAssignment("exampleGroupPolicyAssignment", GroupPolicyAssignmentArgs.builder()
* .name("example-policy")
* .policyDefinitionId(exampleDefinition.id())
* .managementGroupId(example.id())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: azure:management:Group
* properties:
* displayName: Some Management Group
* exampleDefinition:
* type: azure:policy:Definition
* name: example
* properties:
* name: only-deploy-in-westeurope
* policyType: Custom
* mode: All
* displayName: my-policy-definition
* managementGroupId: ${example.id}
* policyRule: |2
* {
* "if": {
* "not": {
* "field": "location",
* "equals": "westeurope"
* }
* },
* "then": {
* "effect": "Deny"
* }
* }
* exampleGroupPolicyAssignment:
* type: azure:management:GroupPolicyAssignment
* name: example
* properties:
* name: example-policy
* policyDefinitionId: ${exampleDefinition.id}
* managementGroupId: ${example.id}
* ```
*
* ## Import
* Management Group Policy Assignments can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:management/groupPolicyAssignment:GroupPolicyAssignment example /providers/Microsoft.Management/managementGroups/group1/providers/Microsoft.Authorization/policyAssignments/assignment1
* ```
* @property description A description which should be used for this Policy Assignment.
* @property displayName The Display Name for this Policy Assignment.
* @property enforce Specifies if this Policy should be enforced or not? Defaults to `true`.
* @property identity An `identity` block as defined below.
* > **Note:** The `location` field must also be specified when `identity` is specified.
* @property location The Azure Region where the Policy Assignment should exist. Changing this forces a new Policy Assignment to be created.
* @property managementGroupId The ID of the Management Group. Changing this forces a new Policy Assignment to be created.
* @property metadata A JSON mapping of any Metadata for this Policy.
* @property name The name which should be used for this Policy Assignment. Possible values must be between 3 and 24 characters in length. Changing this forces a new Policy Assignment to be created.
* @property nonComplianceMessages One or more `non_compliance_message` blocks as defined below.
* @property notScopes Specifies a list of Resource Scopes (for example a Subscription, or a Resource Group) within this Management Group which are excluded from this Policy.
* @property overrides One or more `overrides` blocks as defined below. More detail about `overrides` and `resource_selectors` see [policy assignment structure](https://learn.microsoft.com/en-us/azure/governance/policy/concepts/assignment-structure#resource-selectors-preview)
* @property parameters A JSON mapping of any Parameters for this Policy.
* @property policyDefinitionId The ID of the Policy Definition or Policy Definition Set. Changing this forces a new Policy Assignment to be created.
* @property resourceSelectors One or more `resource_selectors` blocks as defined below to filter polices by resource properties.
*/
public data class GroupPolicyAssignmentArgs(
public val description: Output? = null,
public val displayName: Output? = null,
public val enforce: Output? = null,
public val identity: Output? = null,
public val location: Output? = null,
public val managementGroupId: Output? = null,
public val metadata: Output? = null,
public val name: Output? = null,
public val nonComplianceMessages: Output>? =
null,
public val notScopes: Output>? = null,
public val overrides: Output>? = null,
public val parameters: Output? = null,
public val policyDefinitionId: Output? = null,
public val resourceSelectors: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.management.GroupPolicyAssignmentArgs =
com.pulumi.azure.management.GroupPolicyAssignmentArgs.builder()
.description(description?.applyValue({ args0 -> args0 }))
.displayName(displayName?.applyValue({ args0 -> args0 }))
.enforce(enforce?.applyValue({ args0 -> args0 }))
.identity(identity?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.location(location?.applyValue({ args0 -> args0 }))
.managementGroupId(managementGroupId?.applyValue({ args0 -> args0 }))
.metadata(metadata?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.nonComplianceMessages(
nonComplianceMessages?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.notScopes(notScopes?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.overrides(
overrides?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.parameters(parameters?.applyValue({ args0 -> args0 }))
.policyDefinitionId(policyDefinitionId?.applyValue({ args0 -> args0 }))
.resourceSelectors(
resourceSelectors?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
).build()
}
/**
* Builder for [GroupPolicyAssignmentArgs].
*/
@PulumiTagMarker
public class GroupPolicyAssignmentArgsBuilder internal constructor() {
private var description: Output? = null
private var displayName: Output? = null
private var enforce: Output? = null
private var identity: Output? = null
private var location: Output? = null
private var managementGroupId: Output? = null
private var metadata: Output? = null
private var name: Output? = null
private var nonComplianceMessages: Output>? =
null
private var notScopes: Output>? = null
private var overrides: Output>? = null
private var parameters: Output? = null
private var policyDefinitionId: Output? = null
private var resourceSelectors: Output>? = null
/**
* @param value A description which should be used for this Policy Assignment.
*/
@JvmName("telsnschxibfmjjv")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value The Display Name for this Policy Assignment.
*/
@JvmName("jkjvxcjbgnefgbei")
public suspend fun displayName(`value`: Output) {
this.displayName = value
}
/**
* @param value Specifies if this Policy should be enforced or not? Defaults to `true`.
*/
@JvmName("evccdyivjvqylaeg")
public suspend fun enforce(`value`: Output) {
this.enforce = value
}
/**
* @param value An `identity` block as defined below.
* > **Note:** The `location` field must also be specified when `identity` is specified.
*/
@JvmName("vhqgsfjprhdktojv")
public suspend fun identity(`value`: Output) {
this.identity = value
}
/**
* @param value The Azure Region where the Policy Assignment should exist. Changing this forces a new Policy Assignment to be created.
*/
@JvmName("dfvxrywthnxniyln")
public suspend fun location(`value`: Output) {
this.location = value
}
/**
* @param value The ID of the Management Group. Changing this forces a new Policy Assignment to be created.
*/
@JvmName("gryfkpdhylqjjucp")
public suspend fun managementGroupId(`value`: Output) {
this.managementGroupId = value
}
/**
* @param value A JSON mapping of any Metadata for this Policy.
*/
@JvmName("xfxcfjtumblsrhfe")
public suspend fun metadata(`value`: Output) {
this.metadata = value
}
/**
* @param value The name which should be used for this Policy Assignment. Possible values must be between 3 and 24 characters in length. Changing this forces a new Policy Assignment to be created.
*/
@JvmName("vlpqwksmfqxohgeg")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value One or more `non_compliance_message` blocks as defined below.
*/
@JvmName("hwhjggulfjetjyrq")
public suspend fun nonComplianceMessages(`value`: Output>) {
this.nonComplianceMessages = value
}
@JvmName("bqpidafvxsrccrtq")
public suspend fun nonComplianceMessages(vararg values: Output) {
this.nonComplianceMessages = Output.all(values.asList())
}
/**
* @param values One or more `non_compliance_message` blocks as defined below.
*/
@JvmName("ybbiwjmlebgcxpoa")
public suspend fun nonComplianceMessages(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy