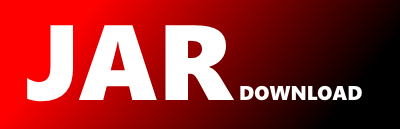
com.pulumi.azure.media.kotlin.inputs.ContentKeyPolicyPolicyOptionPlayreadyConfigurationLicenseArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.media.kotlin.inputs
import com.pulumi.azure.media.inputs.ContentKeyPolicyPolicyOptionPlayreadyConfigurationLicenseArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property allowTestDevices A flag indicating whether test devices can use the license.
* @property beginDate The begin date of license.
* @property contentKeyLocationFromHeaderEnabled Specifies that the content key ID is in the PlayReady header.
* @property contentKeyLocationFromKeyId The content key ID. Specifies that the content key ID is specified in the PlayReady configuration.
* > **NOTE:** You can only specify one content key location. For example if you specify `content_key_location_from_header_enabled` in true, you shouldn't specify `content_key_location_from_key_id` and vice versa.
* @property contentType The PlayReady content type. Supported values are `UltraVioletDownload`, `UltraVioletStreaming` or `Unspecified`.
* @property expirationDate The expiration date of license.
* @property gracePeriod The grace period of license.
* @property licenseType The license type. Supported values are `NonPersistent` or `Persistent`.
* @property playRight A `play_right` block as defined above.
* @property relativeBeginDate The relative begin date of license.
* @property relativeExpirationDate The relative expiration date of license.
* @property securityLevel The security level of the PlayReady license. Possible values are `SL150`, `SL2000` and `SL3000`. Please see [this document](https://learn.microsoft.com/en-us/rest/api/media/content-key-policies/create-or-update?tabs=HTTP#securitylevel) for more information about security level. See [this document](https://learn.microsoft.com/en-us/azure/media-services/latest/drm-playready-license-template-concept#playready-sl3000-support) for more information about `SL3000` support.
*/
public data class ContentKeyPolicyPolicyOptionPlayreadyConfigurationLicenseArgs(
public val allowTestDevices: Output? = null,
public val beginDate: Output? = null,
public val contentKeyLocationFromHeaderEnabled: Output? = null,
public val contentKeyLocationFromKeyId: Output? = null,
public val contentType: Output? = null,
public val expirationDate: Output? = null,
public val gracePeriod: Output? = null,
public val licenseType: Output? = null,
public val playRight: Output? = null,
public val relativeBeginDate: Output? = null,
public val relativeExpirationDate: Output? = null,
public val securityLevel: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.azure.media.inputs.ContentKeyPolicyPolicyOptionPlayreadyConfigurationLicenseArgs =
com.pulumi.azure.media.inputs.ContentKeyPolicyPolicyOptionPlayreadyConfigurationLicenseArgs.builder()
.allowTestDevices(allowTestDevices?.applyValue({ args0 -> args0 }))
.beginDate(beginDate?.applyValue({ args0 -> args0 }))
.contentKeyLocationFromHeaderEnabled(
contentKeyLocationFromHeaderEnabled?.applyValue({ args0 ->
args0
}),
)
.contentKeyLocationFromKeyId(contentKeyLocationFromKeyId?.applyValue({ args0 -> args0 }))
.contentType(contentType?.applyValue({ args0 -> args0 }))
.expirationDate(expirationDate?.applyValue({ args0 -> args0 }))
.gracePeriod(gracePeriod?.applyValue({ args0 -> args0 }))
.licenseType(licenseType?.applyValue({ args0 -> args0 }))
.playRight(playRight?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.relativeBeginDate(relativeBeginDate?.applyValue({ args0 -> args0 }))
.relativeExpirationDate(relativeExpirationDate?.applyValue({ args0 -> args0 }))
.securityLevel(securityLevel?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ContentKeyPolicyPolicyOptionPlayreadyConfigurationLicenseArgs].
*/
@PulumiTagMarker
public class ContentKeyPolicyPolicyOptionPlayreadyConfigurationLicenseArgsBuilder internal constructor() {
private var allowTestDevices: Output? = null
private var beginDate: Output? = null
private var contentKeyLocationFromHeaderEnabled: Output? = null
private var contentKeyLocationFromKeyId: Output? = null
private var contentType: Output? = null
private var expirationDate: Output? = null
private var gracePeriod: Output? = null
private var licenseType: Output? = null
private var playRight:
Output? = null
private var relativeBeginDate: Output? = null
private var relativeExpirationDate: Output? = null
private var securityLevel: Output? = null
/**
* @param value A flag indicating whether test devices can use the license.
*/
@JvmName("ouhuftkrrtonyhns")
public suspend fun allowTestDevices(`value`: Output) {
this.allowTestDevices = value
}
/**
* @param value The begin date of license.
*/
@JvmName("nqhjximrgscwqdtx")
public suspend fun beginDate(`value`: Output) {
this.beginDate = value
}
/**
* @param value Specifies that the content key ID is in the PlayReady header.
*/
@JvmName("gisksbqdrxrtxllj")
public suspend fun contentKeyLocationFromHeaderEnabled(`value`: Output) {
this.contentKeyLocationFromHeaderEnabled = value
}
/**
* @param value The content key ID. Specifies that the content key ID is specified in the PlayReady configuration.
* > **NOTE:** You can only specify one content key location. For example if you specify `content_key_location_from_header_enabled` in true, you shouldn't specify `content_key_location_from_key_id` and vice versa.
*/
@JvmName("bucfbbwtlbiorana")
public suspend fun contentKeyLocationFromKeyId(`value`: Output) {
this.contentKeyLocationFromKeyId = value
}
/**
* @param value The PlayReady content type. Supported values are `UltraVioletDownload`, `UltraVioletStreaming` or `Unspecified`.
*/
@JvmName("jnshknsyvsyisfau")
public suspend fun contentType(`value`: Output) {
this.contentType = value
}
/**
* @param value The expiration date of license.
*/
@JvmName("atcsvtsrotwgbivl")
public suspend fun expirationDate(`value`: Output) {
this.expirationDate = value
}
/**
* @param value The grace period of license.
*/
@JvmName("lpnnhkbwvontidtk")
public suspend fun gracePeriod(`value`: Output) {
this.gracePeriod = value
}
/**
* @param value The license type. Supported values are `NonPersistent` or `Persistent`.
*/
@JvmName("gnwhxetodveeemep")
public suspend fun licenseType(`value`: Output) {
this.licenseType = value
}
/**
* @param value A `play_right` block as defined above.
*/
@JvmName("wkkbdwnjwnuysshv")
public suspend fun playRight(`value`: Output) {
this.playRight = value
}
/**
* @param value The relative begin date of license.
*/
@JvmName("aqwqwluodtiluaji")
public suspend fun relativeBeginDate(`value`: Output) {
this.relativeBeginDate = value
}
/**
* @param value The relative expiration date of license.
*/
@JvmName("rcgpvmpdebvduvok")
public suspend fun relativeExpirationDate(`value`: Output) {
this.relativeExpirationDate = value
}
/**
* @param value The security level of the PlayReady license. Possible values are `SL150`, `SL2000` and `SL3000`. Please see [this document](https://learn.microsoft.com/en-us/rest/api/media/content-key-policies/create-or-update?tabs=HTTP#securitylevel) for more information about security level. See [this document](https://learn.microsoft.com/en-us/azure/media-services/latest/drm-playready-license-template-concept#playready-sl3000-support) for more information about `SL3000` support.
*/
@JvmName("rcebytavuasgjjyu")
public suspend fun securityLevel(`value`: Output) {
this.securityLevel = value
}
/**
* @param value A flag indicating whether test devices can use the license.
*/
@JvmName("uyhauqdpgoeemvby")
public suspend fun allowTestDevices(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.allowTestDevices = mapped
}
/**
* @param value The begin date of license.
*/
@JvmName("ihfjghndhrwomahg")
public suspend fun beginDate(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.beginDate = mapped
}
/**
* @param value Specifies that the content key ID is in the PlayReady header.
*/
@JvmName("kooalwdluqmtgsdm")
public suspend fun contentKeyLocationFromHeaderEnabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.contentKeyLocationFromHeaderEnabled = mapped
}
/**
* @param value The content key ID. Specifies that the content key ID is specified in the PlayReady configuration.
* > **NOTE:** You can only specify one content key location. For example if you specify `content_key_location_from_header_enabled` in true, you shouldn't specify `content_key_location_from_key_id` and vice versa.
*/
@JvmName("vscskpriaidgtthp")
public suspend fun contentKeyLocationFromKeyId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.contentKeyLocationFromKeyId = mapped
}
/**
* @param value The PlayReady content type. Supported values are `UltraVioletDownload`, `UltraVioletStreaming` or `Unspecified`.
*/
@JvmName("xwpbuqhmskqjyqtl")
public suspend fun contentType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.contentType = mapped
}
/**
* @param value The expiration date of license.
*/
@JvmName("xvkwesudejmulngv")
public suspend fun expirationDate(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.expirationDate = mapped
}
/**
* @param value The grace period of license.
*/
@JvmName("bndqxdwmdpkidhaw")
public suspend fun gracePeriod(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.gracePeriod = mapped
}
/**
* @param value The license type. Supported values are `NonPersistent` or `Persistent`.
*/
@JvmName("jroewnrhueiueogt")
public suspend fun licenseType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.licenseType = mapped
}
/**
* @param value A `play_right` block as defined above.
*/
@JvmName("epwqvfgyvifwxwcv")
public suspend fun playRight(`value`: ContentKeyPolicyPolicyOptionPlayreadyConfigurationLicensePlayRightArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.playRight = mapped
}
/**
* @param argument A `play_right` block as defined above.
*/
@JvmName("qxrajrsqnekxskdg")
public suspend fun playRight(argument: suspend ContentKeyPolicyPolicyOptionPlayreadyConfigurationLicensePlayRightArgsBuilder.() -> Unit) {
val toBeMapped =
ContentKeyPolicyPolicyOptionPlayreadyConfigurationLicensePlayRightArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.playRight = mapped
}
/**
* @param value The relative begin date of license.
*/
@JvmName("wgwkuuvqjxmdrddq")
public suspend fun relativeBeginDate(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.relativeBeginDate = mapped
}
/**
* @param value The relative expiration date of license.
*/
@JvmName("uudjopwkigshmgtd")
public suspend fun relativeExpirationDate(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.relativeExpirationDate = mapped
}
/**
* @param value The security level of the PlayReady license. Possible values are `SL150`, `SL2000` and `SL3000`. Please see [this document](https://learn.microsoft.com/en-us/rest/api/media/content-key-policies/create-or-update?tabs=HTTP#securitylevel) for more information about security level. See [this document](https://learn.microsoft.com/en-us/azure/media-services/latest/drm-playready-license-template-concept#playready-sl3000-support) for more information about `SL3000` support.
*/
@JvmName("lrywklxegvakukwk")
public suspend fun securityLevel(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.securityLevel = mapped
}
internal fun build(): ContentKeyPolicyPolicyOptionPlayreadyConfigurationLicenseArgs =
ContentKeyPolicyPolicyOptionPlayreadyConfigurationLicenseArgs(
allowTestDevices = allowTestDevices,
beginDate = beginDate,
contentKeyLocationFromHeaderEnabled = contentKeyLocationFromHeaderEnabled,
contentKeyLocationFromKeyId = contentKeyLocationFromKeyId,
contentType = contentType,
expirationDate = expirationDate,
gracePeriod = gracePeriod,
licenseType = licenseType,
playRight = playRight,
relativeBeginDate = relativeBeginDate,
relativeExpirationDate = relativeExpirationDate,
securityLevel = securityLevel,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy