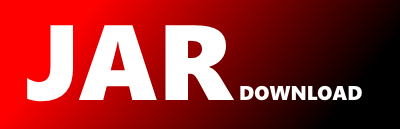
com.pulumi.azure.media.kotlin.inputs.ContentKeyPolicyPolicyOptionPlayreadyConfigurationLicensePlayRightArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.media.kotlin.inputs
import com.pulumi.azure.media.inputs.ContentKeyPolicyPolicyOptionPlayreadyConfigurationLicensePlayRightArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property agcAndColorStripeRestriction Configures Automatic Gain Control (AGC) and Color Stripe in the license. Must be between `0` and `3` inclusive.
* @property allowPassingVideoContentToUnknownOutput Configures Unknown output handling settings of the license. Supported values are `Allowed`, `AllowedWithVideoConstriction` or `NotAllowed`.
* @property analogVideoOpl Specifies the output protection level for compressed digital audio. Supported values are `100`, `150` or `200`.
* @property compressedDigitalAudioOpl Specifies the output protection level for compressed digital audio.Supported values are `100`, `150`, `200`, `250` or `300`.
* @property compressedDigitalVideoOpl Specifies the output protection level for compressed digital video. Supported values are `400` or `500`.
* @property digitalVideoOnlyContentRestriction Enables the Image Constraint For Analog Component Video Restriction in the license.
* @property explicitAnalogTelevisionOutputRestriction An `explicit_analog_television_output_restriction` block as defined above.
* @property firstPlayExpiration The amount of time that the license is valid after the license is first used to play content.
* @property imageConstraintForAnalogComponentVideoRestriction Enables the Image Constraint For Analog Component Video Restriction in the license.
* @property imageConstraintForAnalogComputerMonitorRestriction Enables the Image Constraint For Analog Component Video Restriction in the license.
* @property scmsRestriction Configures the Serial Copy Management System (SCMS) in the license. Must be between `0` and `3` inclusive.
* @property uncompressedDigitalAudioOpl Specifies the output protection level for uncompressed digital audio. Supported values are `100`, `150`, `200`, `250` or `300`.
* @property uncompressedDigitalVideoOpl Specifies the output protection level for uncompressed digital video. Supported values are `100`, `250`, `270` or `300`.
*/
public data class ContentKeyPolicyPolicyOptionPlayreadyConfigurationLicensePlayRightArgs(
public val agcAndColorStripeRestriction: Output? = null,
public val allowPassingVideoContentToUnknownOutput: Output? = null,
public val analogVideoOpl: Output? = null,
public val compressedDigitalAudioOpl: Output? = null,
public val compressedDigitalVideoOpl: Output? = null,
public val digitalVideoOnlyContentRestriction: Output? = null,
public val explicitAnalogTelevisionOutputRestriction: Output? =
null,
public val firstPlayExpiration: Output? = null,
public val imageConstraintForAnalogComponentVideoRestriction: Output? = null,
public val imageConstraintForAnalogComputerMonitorRestriction: Output? = null,
public val scmsRestriction: Output? = null,
public val uncompressedDigitalAudioOpl: Output? = null,
public val uncompressedDigitalVideoOpl: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.azure.media.inputs.ContentKeyPolicyPolicyOptionPlayreadyConfigurationLicensePlayRightArgs =
com.pulumi.azure.media.inputs.ContentKeyPolicyPolicyOptionPlayreadyConfigurationLicensePlayRightArgs.builder()
.agcAndColorStripeRestriction(agcAndColorStripeRestriction?.applyValue({ args0 -> args0 }))
.allowPassingVideoContentToUnknownOutput(
allowPassingVideoContentToUnknownOutput?.applyValue({ args0 ->
args0
}),
)
.analogVideoOpl(analogVideoOpl?.applyValue({ args0 -> args0 }))
.compressedDigitalAudioOpl(compressedDigitalAudioOpl?.applyValue({ args0 -> args0 }))
.compressedDigitalVideoOpl(compressedDigitalVideoOpl?.applyValue({ args0 -> args0 }))
.digitalVideoOnlyContentRestriction(
digitalVideoOnlyContentRestriction?.applyValue({ args0 ->
args0
}),
)
.explicitAnalogTelevisionOutputRestriction(
explicitAnalogTelevisionOutputRestriction?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.firstPlayExpiration(firstPlayExpiration?.applyValue({ args0 -> args0 }))
.imageConstraintForAnalogComponentVideoRestriction(
imageConstraintForAnalogComponentVideoRestriction?.applyValue({ args0 ->
args0
}),
)
.imageConstraintForAnalogComputerMonitorRestriction(
imageConstraintForAnalogComputerMonitorRestriction?.applyValue({ args0 ->
args0
}),
)
.scmsRestriction(scmsRestriction?.applyValue({ args0 -> args0 }))
.uncompressedDigitalAudioOpl(uncompressedDigitalAudioOpl?.applyValue({ args0 -> args0 }))
.uncompressedDigitalVideoOpl(uncompressedDigitalVideoOpl?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ContentKeyPolicyPolicyOptionPlayreadyConfigurationLicensePlayRightArgs].
*/
@PulumiTagMarker
public class ContentKeyPolicyPolicyOptionPlayreadyConfigurationLicensePlayRightArgsBuilder internal constructor() {
private var agcAndColorStripeRestriction: Output? = null
private var allowPassingVideoContentToUnknownOutput: Output? = null
private var analogVideoOpl: Output? = null
private var compressedDigitalAudioOpl: Output? = null
private var compressedDigitalVideoOpl: Output? = null
private var digitalVideoOnlyContentRestriction: Output? = null
private var explicitAnalogTelevisionOutputRestriction:
Output? =
null
private var firstPlayExpiration: Output? = null
private var imageConstraintForAnalogComponentVideoRestriction: Output? = null
private var imageConstraintForAnalogComputerMonitorRestriction: Output? = null
private var scmsRestriction: Output? = null
private var uncompressedDigitalAudioOpl: Output? = null
private var uncompressedDigitalVideoOpl: Output? = null
/**
* @param value Configures Automatic Gain Control (AGC) and Color Stripe in the license. Must be between `0` and `3` inclusive.
*/
@JvmName("ysctjugyrpnkpeql")
public suspend fun agcAndColorStripeRestriction(`value`: Output) {
this.agcAndColorStripeRestriction = value
}
/**
* @param value Configures Unknown output handling settings of the license. Supported values are `Allowed`, `AllowedWithVideoConstriction` or `NotAllowed`.
*/
@JvmName("qntdqqksdkvhlnhm")
public suspend fun allowPassingVideoContentToUnknownOutput(`value`: Output) {
this.allowPassingVideoContentToUnknownOutput = value
}
/**
* @param value Specifies the output protection level for compressed digital audio. Supported values are `100`, `150` or `200`.
*/
@JvmName("fvheunrixervbyoa")
public suspend fun analogVideoOpl(`value`: Output) {
this.analogVideoOpl = value
}
/**
* @param value Specifies the output protection level for compressed digital audio.Supported values are `100`, `150`, `200`, `250` or `300`.
*/
@JvmName("uxlxblscjjfdaogm")
public suspend fun compressedDigitalAudioOpl(`value`: Output) {
this.compressedDigitalAudioOpl = value
}
/**
* @param value Specifies the output protection level for compressed digital video. Supported values are `400` or `500`.
*/
@JvmName("qvnajhquelkyywag")
public suspend fun compressedDigitalVideoOpl(`value`: Output) {
this.compressedDigitalVideoOpl = value
}
/**
* @param value Enables the Image Constraint For Analog Component Video Restriction in the license.
*/
@JvmName("vuvtqjincjgllkok")
public suspend fun digitalVideoOnlyContentRestriction(`value`: Output) {
this.digitalVideoOnlyContentRestriction = value
}
/**
* @param value An `explicit_analog_television_output_restriction` block as defined above.
*/
@JvmName("xpyduxnpuwxhfnfk")
public suspend fun explicitAnalogTelevisionOutputRestriction(`value`: Output) {
this.explicitAnalogTelevisionOutputRestriction = value
}
/**
* @param value The amount of time that the license is valid after the license is first used to play content.
*/
@JvmName("fqaentokkhfkastw")
public suspend fun firstPlayExpiration(`value`: Output) {
this.firstPlayExpiration = value
}
/**
* @param value Enables the Image Constraint For Analog Component Video Restriction in the license.
*/
@JvmName("vqkuqohjrebwidit")
public suspend fun imageConstraintForAnalogComponentVideoRestriction(`value`: Output) {
this.imageConstraintForAnalogComponentVideoRestriction = value
}
/**
* @param value Enables the Image Constraint For Analog Component Video Restriction in the license.
*/
@JvmName("thvpfehqhustnhmi")
public suspend fun imageConstraintForAnalogComputerMonitorRestriction(`value`: Output) {
this.imageConstraintForAnalogComputerMonitorRestriction = value
}
/**
* @param value Configures the Serial Copy Management System (SCMS) in the license. Must be between `0` and `3` inclusive.
*/
@JvmName("bhmxjevrfsknxvai")
public suspend fun scmsRestriction(`value`: Output) {
this.scmsRestriction = value
}
/**
* @param value Specifies the output protection level for uncompressed digital audio. Supported values are `100`, `150`, `200`, `250` or `300`.
*/
@JvmName("exlnweprkfenafwl")
public suspend fun uncompressedDigitalAudioOpl(`value`: Output) {
this.uncompressedDigitalAudioOpl = value
}
/**
* @param value Specifies the output protection level for uncompressed digital video. Supported values are `100`, `250`, `270` or `300`.
*/
@JvmName("ebmpbevukqvsfslb")
public suspend fun uncompressedDigitalVideoOpl(`value`: Output) {
this.uncompressedDigitalVideoOpl = value
}
/**
* @param value Configures Automatic Gain Control (AGC) and Color Stripe in the license. Must be between `0` and `3` inclusive.
*/
@JvmName("svqwbskopbpquusq")
public suspend fun agcAndColorStripeRestriction(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.agcAndColorStripeRestriction = mapped
}
/**
* @param value Configures Unknown output handling settings of the license. Supported values are `Allowed`, `AllowedWithVideoConstriction` or `NotAllowed`.
*/
@JvmName("nucyxtypldhofhbe")
public suspend fun allowPassingVideoContentToUnknownOutput(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.allowPassingVideoContentToUnknownOutput = mapped
}
/**
* @param value Specifies the output protection level for compressed digital audio. Supported values are `100`, `150` or `200`.
*/
@JvmName("focakobtsclmvdsp")
public suspend fun analogVideoOpl(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.analogVideoOpl = mapped
}
/**
* @param value Specifies the output protection level for compressed digital audio.Supported values are `100`, `150`, `200`, `250` or `300`.
*/
@JvmName("vkshpxrssodqcwhp")
public suspend fun compressedDigitalAudioOpl(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.compressedDigitalAudioOpl = mapped
}
/**
* @param value Specifies the output protection level for compressed digital video. Supported values are `400` or `500`.
*/
@JvmName("owasqhkmvjkwlsvv")
public suspend fun compressedDigitalVideoOpl(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.compressedDigitalVideoOpl = mapped
}
/**
* @param value Enables the Image Constraint For Analog Component Video Restriction in the license.
*/
@JvmName("kavdridyvfpygqpy")
public suspend fun digitalVideoOnlyContentRestriction(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.digitalVideoOnlyContentRestriction = mapped
}
/**
* @param value An `explicit_analog_television_output_restriction` block as defined above.
*/
@JvmName("vbnyibipqxaqdkcb")
public suspend fun explicitAnalogTelevisionOutputRestriction(`value`: ContentKeyPolicyPolicyOptionPlayreadyConfigurationLicensePlayRightExplicitAnalogTelevisionOutputRestrictionArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.explicitAnalogTelevisionOutputRestriction = mapped
}
/**
* @param argument An `explicit_analog_television_output_restriction` block as defined above.
*/
@JvmName("jynolyuxxdcdahav")
public suspend fun explicitAnalogTelevisionOutputRestriction(argument: suspend ContentKeyPolicyPolicyOptionPlayreadyConfigurationLicensePlayRightExplicitAnalogTelevisionOutputRestrictionArgsBuilder.() -> Unit) {
val toBeMapped =
ContentKeyPolicyPolicyOptionPlayreadyConfigurationLicensePlayRightExplicitAnalogTelevisionOutputRestrictionArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.explicitAnalogTelevisionOutputRestriction = mapped
}
/**
* @param value The amount of time that the license is valid after the license is first used to play content.
*/
@JvmName("gnibccmbcbbjlkor")
public suspend fun firstPlayExpiration(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.firstPlayExpiration = mapped
}
/**
* @param value Enables the Image Constraint For Analog Component Video Restriction in the license.
*/
@JvmName("pxyrwhhhtbrlddbk")
public suspend fun imageConstraintForAnalogComponentVideoRestriction(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.imageConstraintForAnalogComponentVideoRestriction = mapped
}
/**
* @param value Enables the Image Constraint For Analog Component Video Restriction in the license.
*/
@JvmName("dfbnajnpambkpkxf")
public suspend fun imageConstraintForAnalogComputerMonitorRestriction(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.imageConstraintForAnalogComputerMonitorRestriction = mapped
}
/**
* @param value Configures the Serial Copy Management System (SCMS) in the license. Must be between `0` and `3` inclusive.
*/
@JvmName("mmrpuatfvqlfwcsr")
public suspend fun scmsRestriction(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.scmsRestriction = mapped
}
/**
* @param value Specifies the output protection level for uncompressed digital audio. Supported values are `100`, `150`, `200`, `250` or `300`.
*/
@JvmName("pqfkqgmuyflywnlf")
public suspend fun uncompressedDigitalAudioOpl(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.uncompressedDigitalAudioOpl = mapped
}
/**
* @param value Specifies the output protection level for uncompressed digital video. Supported values are `100`, `250`, `270` or `300`.
*/
@JvmName("hopprbxvrktwjvml")
public suspend fun uncompressedDigitalVideoOpl(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.uncompressedDigitalVideoOpl = mapped
}
internal fun build(): ContentKeyPolicyPolicyOptionPlayreadyConfigurationLicensePlayRightArgs =
ContentKeyPolicyPolicyOptionPlayreadyConfigurationLicensePlayRightArgs(
agcAndColorStripeRestriction = agcAndColorStripeRestriction,
allowPassingVideoContentToUnknownOutput = allowPassingVideoContentToUnknownOutput,
analogVideoOpl = analogVideoOpl,
compressedDigitalAudioOpl = compressedDigitalAudioOpl,
compressedDigitalVideoOpl = compressedDigitalVideoOpl,
digitalVideoOnlyContentRestriction = digitalVideoOnlyContentRestriction,
explicitAnalogTelevisionOutputRestriction = explicitAnalogTelevisionOutputRestriction,
firstPlayExpiration = firstPlayExpiration,
imageConstraintForAnalogComponentVideoRestriction = imageConstraintForAnalogComponentVideoRestriction,
imageConstraintForAnalogComputerMonitorRestriction = imageConstraintForAnalogComputerMonitorRestriction,
scmsRestriction = scmsRestriction,
uncompressedDigitalAudioOpl = uncompressedDigitalAudioOpl,
uncompressedDigitalVideoOpl = uncompressedDigitalVideoOpl,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy