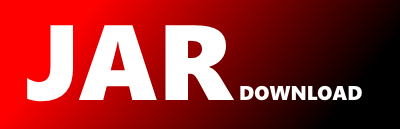
com.pulumi.azure.media.kotlin.inputs.LiveEventEncodingArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.media.kotlin.inputs
import com.pulumi.azure.media.inputs.LiveEventEncodingArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property keyFrameInterval Use an `ISO 8601` time value between 0.5 to 20 seconds to specify the output fragment length for the video and audio tracks of an encoding live event. For example, use `PT2S` to indicate 2 seconds. For the video track it also defines the key frame interval, or the length of a GoP (group of pictures). The value cannot be set for pass-through live events. Defaults to `PT2S`.
* @property presetName The optional encoding preset name, used when `type` is not `None`. If the `type` is set to `Standard`, then the default preset name is `Default720p`. Else if the `type` is set to `Premium1080p`, Changing this forces a new resource to be created.
* @property stretchMode Specifies how the input video will be resized to fit the desired output resolution(s). Allowed values are `None`, `AutoFit` or `AutoSize`. Default is `None`.
* @property type Live event type. Possible values are `None`, `Premium1080p`, `PassthroughBasic`, `PassthroughStandard` and `Standard`. When set to `None`, the service simply passes through the incoming video and audio layer(s) to the output. When `type` is set to `Standard` or `Premium1080p`, a live encoder transcodes the incoming stream into multiple bitrates or layers. Defaults to `None`. Changing this forces a new resource to be created.
* > [More information can be found in the Microsoft Documentation](https://go.microsoft.com/fwlink/?linkid=2095101).
*/
public data class LiveEventEncodingArgs(
public val keyFrameInterval: Output? = null,
public val presetName: Output? = null,
public val stretchMode: Output? = null,
public val type: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.media.inputs.LiveEventEncodingArgs =
com.pulumi.azure.media.inputs.LiveEventEncodingArgs.builder()
.keyFrameInterval(keyFrameInterval?.applyValue({ args0 -> args0 }))
.presetName(presetName?.applyValue({ args0 -> args0 }))
.stretchMode(stretchMode?.applyValue({ args0 -> args0 }))
.type(type?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [LiveEventEncodingArgs].
*/
@PulumiTagMarker
public class LiveEventEncodingArgsBuilder internal constructor() {
private var keyFrameInterval: Output? = null
private var presetName: Output? = null
private var stretchMode: Output? = null
private var type: Output? = null
/**
* @param value Use an `ISO 8601` time value between 0.5 to 20 seconds to specify the output fragment length for the video and audio tracks of an encoding live event. For example, use `PT2S` to indicate 2 seconds. For the video track it also defines the key frame interval, or the length of a GoP (group of pictures). The value cannot be set for pass-through live events. Defaults to `PT2S`.
*/
@JvmName("dkgpxcdkiuqqcswf")
public suspend fun keyFrameInterval(`value`: Output) {
this.keyFrameInterval = value
}
/**
* @param value The optional encoding preset name, used when `type` is not `None`. If the `type` is set to `Standard`, then the default preset name is `Default720p`. Else if the `type` is set to `Premium1080p`, Changing this forces a new resource to be created.
*/
@JvmName("xhfiuehyripirapk")
public suspend fun presetName(`value`: Output) {
this.presetName = value
}
/**
* @param value Specifies how the input video will be resized to fit the desired output resolution(s). Allowed values are `None`, `AutoFit` or `AutoSize`. Default is `None`.
*/
@JvmName("akecuboduqxgqupj")
public suspend fun stretchMode(`value`: Output) {
this.stretchMode = value
}
/**
* @param value Live event type. Possible values are `None`, `Premium1080p`, `PassthroughBasic`, `PassthroughStandard` and `Standard`. When set to `None`, the service simply passes through the incoming video and audio layer(s) to the output. When `type` is set to `Standard` or `Premium1080p`, a live encoder transcodes the incoming stream into multiple bitrates or layers. Defaults to `None`. Changing this forces a new resource to be created.
* > [More information can be found in the Microsoft Documentation](https://go.microsoft.com/fwlink/?linkid=2095101).
*/
@JvmName("ecvgnvhjyvqtjqpc")
public suspend fun type(`value`: Output) {
this.type = value
}
/**
* @param value Use an `ISO 8601` time value between 0.5 to 20 seconds to specify the output fragment length for the video and audio tracks of an encoding live event. For example, use `PT2S` to indicate 2 seconds. For the video track it also defines the key frame interval, or the length of a GoP (group of pictures). The value cannot be set for pass-through live events. Defaults to `PT2S`.
*/
@JvmName("yrhijujopivtaawo")
public suspend fun keyFrameInterval(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.keyFrameInterval = mapped
}
/**
* @param value The optional encoding preset name, used when `type` is not `None`. If the `type` is set to `Standard`, then the default preset name is `Default720p`. Else if the `type` is set to `Premium1080p`, Changing this forces a new resource to be created.
*/
@JvmName("bofaurliyyakytcn")
public suspend fun presetName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.presetName = mapped
}
/**
* @param value Specifies how the input video will be resized to fit the desired output resolution(s). Allowed values are `None`, `AutoFit` or `AutoSize`. Default is `None`.
*/
@JvmName("kmmviwppsfnkejes")
public suspend fun stretchMode(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.stretchMode = mapped
}
/**
* @param value Live event type. Possible values are `None`, `Premium1080p`, `PassthroughBasic`, `PassthroughStandard` and `Standard`. When set to `None`, the service simply passes through the incoming video and audio layer(s) to the output. When `type` is set to `Standard` or `Premium1080p`, a live encoder transcodes the incoming stream into multiple bitrates or layers. Defaults to `None`. Changing this forces a new resource to be created.
* > [More information can be found in the Microsoft Documentation](https://go.microsoft.com/fwlink/?linkid=2095101).
*/
@JvmName("stiiecdtcbkpxnft")
public suspend fun type(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.type = mapped
}
internal fun build(): LiveEventEncodingArgs = LiveEventEncodingArgs(
keyFrameInterval = keyFrameInterval,
presetName = presetName,
stretchMode = stretchMode,
type = type,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy