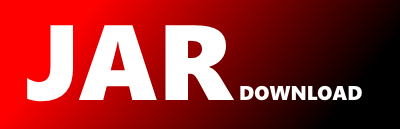
com.pulumi.azure.mobile.kotlin.NetworkAttachedDataNetwork.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.mobile.kotlin
import com.pulumi.azure.mobile.kotlin.outputs.NetworkAttachedDataNetworkNetworkAddressPortTranslation
import com.pulumi.azure.mobile.kotlin.outputs.NetworkAttachedDataNetworkNetworkAddressPortTranslation.Companion.toKotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
/**
* Builder for [NetworkAttachedDataNetwork].
*/
@PulumiTagMarker
public class NetworkAttachedDataNetworkResourceBuilder internal constructor() {
public var name: String? = null
public var args: NetworkAttachedDataNetworkArgs = NetworkAttachedDataNetworkArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend NetworkAttachedDataNetworkArgsBuilder.() -> Unit) {
val builder = NetworkAttachedDataNetworkArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): NetworkAttachedDataNetwork {
val builtJavaResource =
com.pulumi.azure.mobile.NetworkAttachedDataNetwork(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return NetworkAttachedDataNetwork(builtJavaResource)
}
}
/**
* Manages a Mobile Network Attached Data Network.
* ## Example Usage
*
* ```yaml
* resources:
* example:
* type: azure:core:ResourceGroup
* properties:
* name: example-resources
* location: West Europe
* exampleDevice:
* type: azure:databoxedge:Device
* name: example
* properties:
* name: example-device
* resourceGroupName: ${example.name}
* location: ${example.location}
* skuName: EdgeP_Base-Standard
* exampleNetwork:
* type: azure:mobile:Network
* name: example
* properties:
* name: example-mn
* resourceGroupName: ${example.name}
* location: ${example.location}
* mobileCountryCode: '001'
* mobileNetworkCode: '01'
* exampleNetworkPacketCoreControlPlane:
* type: azure:mobile:NetworkPacketCoreControlPlane
* name: example
* properties:
* name: example-mnpccp
* resourceGroupName: ${example.name}
* location: West Europe
* sku: G0
* mobileNetworkId: ${exampleNetwork.id}
* controlPlaneAccessName: default-interface
* controlPlaneAccessIpv4Address: 192.168.1.199
* controlPlaneAccessIpv4Gateway: 192.168.1.1
* controlPlaneAccessIpv4Subnet: 192.168.1.0/25
* platform:
* type: AKS-HCI
* edgeDeviceId: ${exampleDevice.id}
* exampleNetworkPacketCoreDataPlane:
* type: azure:mobile:NetworkPacketCoreDataPlane
* name: example
* properties:
* name: example-mnpcdp
* mobileNetworkPacketCoreControlPlaneId: ${exampleNetworkPacketCoreControlPlane.id}
* location: ${example.location}
* userPlaneAccessName: default-interface
* userPlaneAccessIpv4Address: 192.168.1.199
* userPlaneAccessIpv4Gateway: 192.168.1.1
* userPlaneAccessIpv4Subnet: 192.168.1.0/25
* exampleNetworkDataNetwork:
* type: azure:mobile:NetworkDataNetwork
* name: example
* properties:
* name: example-data-network
* mobileNetworkId: ${exampleNetwork.id}
* location: ${example.location}
* exampleNetworkAttachedDataNetwork:
* type: azure:mobile:NetworkAttachedDataNetwork
* name: example
* properties:
* mobileNetworkDataNetworkName: ${exampleNetworkDataNetwork.name}
* mobileNetworkPacketCoreDataPlaneId: ${exampleNetworkPacketCoreDataPlane.id}
* location: ${example.location}
* dnsAddresses:
* - 1.1.1.1
* userEquipmentAddressPoolPrefixes:
* - 2.4.1.0/24
* userEquipmentStaticAddressPoolPrefixes:
* - 2.4.2.0/24
* userPlaneAccessName: test
* userPlaneAccessIpv4Address: 10.204.141.4
* userPlaneAccessIpv4Gateway: 10.204.141.1
* userPlaneAccessIpv4Subnet: 10.204.141.0/24
* networkAddressPortTranslation:
* pinholeMaximumNumber: 65536
* icmpPinholeTimeoutInSeconds: 30
* tcpPinholeTimeoutInSeconds: 100
* udpPinholeTimeoutInSeconds: 39
* portRange:
* maximum: 49999
* minimum: 1024
* tcpPortReuseMinimumHoldTimeInSeconds: 120
* udpTcpPortReuseMinimumHoldTimeInSeconds: 60
* tags:
* key: value
* ```
*
* ## Import
* Mobile Network Attached Data Network can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:mobile/networkAttachedDataNetwork:NetworkAttachedDataNetwork example /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/resourceGroup1/providers/Microsoft.MobileNetwork/packetCoreControlPlanes/packetCoreControlPlane1/packetCoreDataPlanes/packetCoreDataPlane1/attachedDataNetworks/attachedDataNetwork1
* ```
*/
public class NetworkAttachedDataNetwork internal constructor(
override val javaResource: com.pulumi.azure.mobile.NetworkAttachedDataNetwork,
) : KotlinCustomResource(javaResource, NetworkAttachedDataNetworkMapper) {
/**
* Specifies the DNS servers to signal to UEs to use for this attached data network.
*/
public val dnsAddresses: Output>
get() = javaResource.dnsAddresses().applyValue({ args0 -> args0.map({ args0 -> args0 }) })
/**
* Specifies the Azure Region where the Mobile Network Attached Data Network should exist. Changing this forces a new Mobile Network Attached Data Network to be created.
*/
public val location: Output
get() = javaResource.location().applyValue({ args0 -> args0 })
/**
* Specifies the name of the `azure.mobile.NetworkDataNetwork` which the Attached Data Network belongs to, Changing this forces a new Mobile Network Attached Data Network to be created.
*/
public val mobileNetworkDataNetworkName: Output
get() = javaResource.mobileNetworkDataNetworkName().applyValue({ args0 -> args0 })
/**
* Specifies the ID of the `azure.mobile.NetworkPacketCoreDataPlane` which the Mobile Network Attached Data Network belongs to. Changing this forces a new Mobile Network Attached Data Network to be created.
*/
public val mobileNetworkPacketCoreDataPlaneId: Output
get() = javaResource.mobileNetworkPacketCoreDataPlaneId().applyValue({ args0 -> args0 })
/**
* A `network_address_port_translation` block as defined below.
*/
public val networkAddressPortTranslation:
Output?
get() = javaResource.networkAddressPortTranslation().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> toKotlin(args0) })
}).orElse(null)
})
/**
* A mapping of tags which should be assigned to the Mobile Network Attached Data Network.
*/
public val tags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy