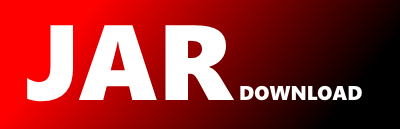
com.pulumi.azure.mobile.kotlin.inputs.NetworkServicePccRuleQosPolicyArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.mobile.kotlin.inputs
import com.pulumi.azure.mobile.inputs.NetworkServicePccRuleQosPolicyArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property allocationAndRetentionPriorityLevel QoS Flow allocation and retention priority (ARP) level. Flows with higher priority preempt flows with lower priority, if the settings of `preemption_capability` and `preemption_vulnerability` allow it. 1 is the highest level of priority. If this field is not specified then `qos_indicator` is used to derive the ARP value. See 3GPP TS23.501 section 5.7.2.2 for a full description of the ARP parameters.
* @property guaranteedBitRate A `guaranteed_bit_rate` block as defined below. The Guaranteed Bit Rate (GBR) for all service data flows that use this PCC Rule. If it's not specified, there will be no GBR set for the PCC Rule that uses this QoS definition.
* @property maximumBitRate A `maximum_bit_rate` block as defined below. The Maximum Bit Rate (MBR) for all service data flows that use this PCC Rule or Service.
* @property preemptionCapability The Preemption Capability of a QoS Flow controls whether it can preempt another QoS Flow with a lower priority level. See 3GPP TS23.501 section 5.7.2.2 for a full description of the ARP parameters. Possible values are `NotPreempt` and `MayPreempt`, Defaults to `NotPreempt`.
* @property preemptionVulnerability The Preemption Vulnerability of a QoS Flow controls whether it can be preempted by QoS Flow with a higher priority level. See 3GPP TS23.501 section 5.7.2.2 for a full description of the ARP parameters. Possible values are `NotPreemptable` and `Preemptable`. Defaults to `Preemptable`.
* @property qosIndicator The QoS Indicator (5QI for 5G network /QCI for 4G net work) value identifies a set of QoS characteristics that control QoS forwarding treatment for QoS flows or EPS bearers. Recommended values: 5-9; 69-70; 79-80. Must be between `1` and `127`.
*/
public data class NetworkServicePccRuleQosPolicyArgs(
public val allocationAndRetentionPriorityLevel: Output? = null,
public val guaranteedBitRate: Output? = null,
public val maximumBitRate: Output,
public val preemptionCapability: Output? = null,
public val preemptionVulnerability: Output? = null,
public val qosIndicator: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.mobile.inputs.NetworkServicePccRuleQosPolicyArgs =
com.pulumi.azure.mobile.inputs.NetworkServicePccRuleQosPolicyArgs.builder()
.allocationAndRetentionPriorityLevel(
allocationAndRetentionPriorityLevel?.applyValue({ args0 ->
args0
}),
)
.guaranteedBitRate(guaranteedBitRate?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.maximumBitRate(maximumBitRate.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.preemptionCapability(preemptionCapability?.applyValue({ args0 -> args0 }))
.preemptionVulnerability(preemptionVulnerability?.applyValue({ args0 -> args0 }))
.qosIndicator(qosIndicator.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [NetworkServicePccRuleQosPolicyArgs].
*/
@PulumiTagMarker
public class NetworkServicePccRuleQosPolicyArgsBuilder internal constructor() {
private var allocationAndRetentionPriorityLevel: Output? = null
private var guaranteedBitRate: Output? = null
private var maximumBitRate: Output? = null
private var preemptionCapability: Output? = null
private var preemptionVulnerability: Output? = null
private var qosIndicator: Output? = null
/**
* @param value QoS Flow allocation and retention priority (ARP) level. Flows with higher priority preempt flows with lower priority, if the settings of `preemption_capability` and `preemption_vulnerability` allow it. 1 is the highest level of priority. If this field is not specified then `qos_indicator` is used to derive the ARP value. See 3GPP TS23.501 section 5.7.2.2 for a full description of the ARP parameters.
*/
@JvmName("ropciucrmohxwotx")
public suspend fun allocationAndRetentionPriorityLevel(`value`: Output) {
this.allocationAndRetentionPriorityLevel = value
}
/**
* @param value A `guaranteed_bit_rate` block as defined below. The Guaranteed Bit Rate (GBR) for all service data flows that use this PCC Rule. If it's not specified, there will be no GBR set for the PCC Rule that uses this QoS definition.
*/
@JvmName("boixvbshhlnbjoiv")
public suspend fun guaranteedBitRate(`value`: Output) {
this.guaranteedBitRate = value
}
/**
* @param value A `maximum_bit_rate` block as defined below. The Maximum Bit Rate (MBR) for all service data flows that use this PCC Rule or Service.
*/
@JvmName("inamcdibnrjkjnpr")
public suspend fun maximumBitRate(`value`: Output) {
this.maximumBitRate = value
}
/**
* @param value The Preemption Capability of a QoS Flow controls whether it can preempt another QoS Flow with a lower priority level. See 3GPP TS23.501 section 5.7.2.2 for a full description of the ARP parameters. Possible values are `NotPreempt` and `MayPreempt`, Defaults to `NotPreempt`.
*/
@JvmName("mndhurxobiioflat")
public suspend fun preemptionCapability(`value`: Output) {
this.preemptionCapability = value
}
/**
* @param value The Preemption Vulnerability of a QoS Flow controls whether it can be preempted by QoS Flow with a higher priority level. See 3GPP TS23.501 section 5.7.2.2 for a full description of the ARP parameters. Possible values are `NotPreemptable` and `Preemptable`. Defaults to `Preemptable`.
*/
@JvmName("vxinlvgvucoifwdv")
public suspend fun preemptionVulnerability(`value`: Output) {
this.preemptionVulnerability = value
}
/**
* @param value The QoS Indicator (5QI for 5G network /QCI for 4G net work) value identifies a set of QoS characteristics that control QoS forwarding treatment for QoS flows or EPS bearers. Recommended values: 5-9; 69-70; 79-80. Must be between `1` and `127`.
*/
@JvmName("qsukxpekdkeyxfvm")
public suspend fun qosIndicator(`value`: Output) {
this.qosIndicator = value
}
/**
* @param value QoS Flow allocation and retention priority (ARP) level. Flows with higher priority preempt flows with lower priority, if the settings of `preemption_capability` and `preemption_vulnerability` allow it. 1 is the highest level of priority. If this field is not specified then `qos_indicator` is used to derive the ARP value. See 3GPP TS23.501 section 5.7.2.2 for a full description of the ARP parameters.
*/
@JvmName("tfljdfrpjwodiiyr")
public suspend fun allocationAndRetentionPriorityLevel(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.allocationAndRetentionPriorityLevel = mapped
}
/**
* @param value A `guaranteed_bit_rate` block as defined below. The Guaranteed Bit Rate (GBR) for all service data flows that use this PCC Rule. If it's not specified, there will be no GBR set for the PCC Rule that uses this QoS definition.
*/
@JvmName("doybxfskfimfgfrb")
public suspend fun guaranteedBitRate(`value`: NetworkServicePccRuleQosPolicyGuaranteedBitRateArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.guaranteedBitRate = mapped
}
/**
* @param argument A `guaranteed_bit_rate` block as defined below. The Guaranteed Bit Rate (GBR) for all service data flows that use this PCC Rule. If it's not specified, there will be no GBR set for the PCC Rule that uses this QoS definition.
*/
@JvmName("ylwjxfprspwndsjm")
public suspend fun guaranteedBitRate(argument: suspend NetworkServicePccRuleQosPolicyGuaranteedBitRateArgsBuilder.() -> Unit) {
val toBeMapped = NetworkServicePccRuleQosPolicyGuaranteedBitRateArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.guaranteedBitRate = mapped
}
/**
* @param value A `maximum_bit_rate` block as defined below. The Maximum Bit Rate (MBR) for all service data flows that use this PCC Rule or Service.
*/
@JvmName("gvuxgrmgmykbvier")
public suspend fun maximumBitRate(`value`: NetworkServicePccRuleQosPolicyMaximumBitRateArgs) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.maximumBitRate = mapped
}
/**
* @param argument A `maximum_bit_rate` block as defined below. The Maximum Bit Rate (MBR) for all service data flows that use this PCC Rule or Service.
*/
@JvmName("vxgyjsgkjhlqqqgj")
public suspend fun maximumBitRate(argument: suspend NetworkServicePccRuleQosPolicyMaximumBitRateArgsBuilder.() -> Unit) {
val toBeMapped = NetworkServicePccRuleQosPolicyMaximumBitRateArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.maximumBitRate = mapped
}
/**
* @param value The Preemption Capability of a QoS Flow controls whether it can preempt another QoS Flow with a lower priority level. See 3GPP TS23.501 section 5.7.2.2 for a full description of the ARP parameters. Possible values are `NotPreempt` and `MayPreempt`, Defaults to `NotPreempt`.
*/
@JvmName("fjwfgqywcriceqot")
public suspend fun preemptionCapability(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.preemptionCapability = mapped
}
/**
* @param value The Preemption Vulnerability of a QoS Flow controls whether it can be preempted by QoS Flow with a higher priority level. See 3GPP TS23.501 section 5.7.2.2 for a full description of the ARP parameters. Possible values are `NotPreemptable` and `Preemptable`. Defaults to `Preemptable`.
*/
@JvmName("kcywwqselysetshu")
public suspend fun preemptionVulnerability(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.preemptionVulnerability = mapped
}
/**
* @param value The QoS Indicator (5QI for 5G network /QCI for 4G net work) value identifies a set of QoS characteristics that control QoS forwarding treatment for QoS flows or EPS bearers. Recommended values: 5-9; 69-70; 79-80. Must be between `1` and `127`.
*/
@JvmName("ffeeypsgsplnhhju")
public suspend fun qosIndicator(`value`: Int) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.qosIndicator = mapped
}
internal fun build(): NetworkServicePccRuleQosPolicyArgs = NetworkServicePccRuleQosPolicyArgs(
allocationAndRetentionPriorityLevel = allocationAndRetentionPriorityLevel,
guaranteedBitRate = guaranteedBitRate,
maximumBitRate = maximumBitRate ?: throw PulumiNullFieldException("maximumBitRate"),
preemptionCapability = preemptionCapability,
preemptionVulnerability = preemptionVulnerability,
qosIndicator = qosIndicator ?: throw PulumiNullFieldException("qosIndicator"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy