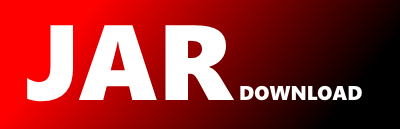
com.pulumi.azure.monitoring.kotlin.ScheduledQueryRulesAlert.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.monitoring.kotlin
import com.pulumi.azure.monitoring.kotlin.outputs.ScheduledQueryRulesAlertAction
import com.pulumi.azure.monitoring.kotlin.outputs.ScheduledQueryRulesAlertTrigger
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import com.pulumi.azure.monitoring.kotlin.outputs.ScheduledQueryRulesAlertAction.Companion.toKotlin as scheduledQueryRulesAlertActionToKotlin
import com.pulumi.azure.monitoring.kotlin.outputs.ScheduledQueryRulesAlertTrigger.Companion.toKotlin as scheduledQueryRulesAlertTriggerToKotlin
/**
* Builder for [ScheduledQueryRulesAlert].
*/
@PulumiTagMarker
public class ScheduledQueryRulesAlertResourceBuilder internal constructor() {
public var name: String? = null
public var args: ScheduledQueryRulesAlertArgs = ScheduledQueryRulesAlertArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend ScheduledQueryRulesAlertArgsBuilder.() -> Unit) {
val builder = ScheduledQueryRulesAlertArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): ScheduledQueryRulesAlert {
val builtJavaResource =
com.pulumi.azure.monitoring.ScheduledQueryRulesAlert(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return ScheduledQueryRulesAlert(builtJavaResource)
}
}
/**
* Manages an AlertingAction Scheduled Query Rules resource within Azure Monitor.
* > **Warning** This resource is using an older AzureRM API version which is known to cause problems e.g. with custom webhook properties not included in triggered alerts. This resource is superseded by the azure.monitoring.ScheduledQueryRulesAlertV2 resource using newer API versions.
* ## Import
* Scheduled Query Rule Alerts can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:monitoring/scheduledQueryRulesAlert:ScheduledQueryRulesAlert example /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/group1/providers/Microsoft.Insights/scheduledQueryRules/myrulename
* ```
*/
public class ScheduledQueryRulesAlert internal constructor(
override val javaResource: com.pulumi.azure.monitoring.ScheduledQueryRulesAlert,
) : KotlinCustomResource(javaResource, ScheduledQueryRulesAlertMapper) {
/**
* An `action` block as defined below.
*/
public val action: Output
get() = javaResource.action().applyValue({ args0 ->
args0.let({ args0 ->
scheduledQueryRulesAlertActionToKotlin(args0)
})
})
/**
* List of Resource IDs referred into query.
*/
public val authorizedResourceIds: Output>?
get() = javaResource.authorizedResourceIds().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
/**
* Should the alerts in this Metric Alert be auto resolved? Defaults to `false`.
* > **NOTE** `auto_mitigation_enabled` and `throttling` are mutually exclusive and cannot both be set.
*/
public val autoMitigationEnabled: Output?
get() = javaResource.autoMitigationEnabled().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The resource URI over which log search query is to be run. Changing this forces a new resource to be created.
*/
public val dataSourceId: Output
get() = javaResource.dataSourceId().applyValue({ args0 -> args0 })
/**
* The description of the scheduled query rule.
*/
public val description: Output?
get() = javaResource.description().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Whether this scheduled query rule is enabled. Default is `true`.
*/
public val enabled: Output?
get() = javaResource.enabled().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Frequency (in minutes) at which rule condition should be evaluated. Values must be between 5 and 1440 (inclusive).
*/
public val frequency: Output
get() = javaResource.frequency().applyValue({ args0 -> args0 })
/**
* Specifies the Azure Region where the resource should exist. Changing this forces a new resource to be created.
*/
public val location: Output
get() = javaResource.location().applyValue({ args0 -> args0 })
/**
* The name of the scheduled query rule. Changing this forces a new resource to be created.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* Log search query.
*/
public val query: Output
get() = javaResource.query().applyValue({ args0 -> args0 })
/**
* The type of query results. Possible values are `ResultCount` and `Number`. Default is `ResultCount`. If set to `ResultCount`, `query` must include an `AggregatedValue` column of a numeric type, for example, `Heartbeat | summarize AggregatedValue = count() by bin(TimeGenerated, 5m)`.
*/
public val queryType: Output?
get() = javaResource.queryType().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The name of the resource group in which to create the scheduled query rule instance. Changing this forces a new resource to be created.
*/
public val resourceGroupName: Output
get() = javaResource.resourceGroupName().applyValue({ args0 -> args0 })
/**
* Severity of the alert. Possible values include: 0, 1, 2, 3, or 4.
*/
public val severity: Output?
get() = javaResource.severity().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* A mapping of tags to assign to the resource.
*/
public val tags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy