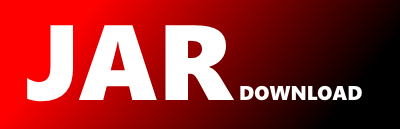
com.pulumi.azure.monitoring.kotlin.inputs.ActionGroupWebhookReceiverArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.monitoring.kotlin.inputs
import com.pulumi.azure.monitoring.inputs.ActionGroupWebhookReceiverArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property aadAuth The `aad_auth` block as defined below.
* > **NOTE:** Before adding a secure webhook receiver by setting `aad_auth`, please read [the configuration instruction of the AAD application](https://docs.microsoft.com/azure/azure-monitor/platform/action-groups#secure-webhook).
* @property name The name of the webhook receiver. Names must be unique (case-insensitive) across all receivers within an action group.
* @property serviceUri The URI where webhooks should be sent.
* @property useCommonAlertSchema Enables or disables the common alert schema.
*/
public data class ActionGroupWebhookReceiverArgs(
public val aadAuth: Output? = null,
public val name: Output,
public val serviceUri: Output,
public val useCommonAlertSchema: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.monitoring.inputs.ActionGroupWebhookReceiverArgs =
com.pulumi.azure.monitoring.inputs.ActionGroupWebhookReceiverArgs.builder()
.aadAuth(aadAuth?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.name(name.applyValue({ args0 -> args0 }))
.serviceUri(serviceUri.applyValue({ args0 -> args0 }))
.useCommonAlertSchema(useCommonAlertSchema?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ActionGroupWebhookReceiverArgs].
*/
@PulumiTagMarker
public class ActionGroupWebhookReceiverArgsBuilder internal constructor() {
private var aadAuth: Output? = null
private var name: Output? = null
private var serviceUri: Output? = null
private var useCommonAlertSchema: Output? = null
/**
* @param value The `aad_auth` block as defined below.
* > **NOTE:** Before adding a secure webhook receiver by setting `aad_auth`, please read [the configuration instruction of the AAD application](https://docs.microsoft.com/azure/azure-monitor/platform/action-groups#secure-webhook).
*/
@JvmName("ryqwhboayowjfyqn")
public suspend fun aadAuth(`value`: Output) {
this.aadAuth = value
}
/**
* @param value The name of the webhook receiver. Names must be unique (case-insensitive) across all receivers within an action group.
*/
@JvmName("mutbtijecspnqybs")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The URI where webhooks should be sent.
*/
@JvmName("adbpiulavckblhpd")
public suspend fun serviceUri(`value`: Output) {
this.serviceUri = value
}
/**
* @param value Enables or disables the common alert schema.
*/
@JvmName("qboprbagqgayraoi")
public suspend fun useCommonAlertSchema(`value`: Output) {
this.useCommonAlertSchema = value
}
/**
* @param value The `aad_auth` block as defined below.
* > **NOTE:** Before adding a secure webhook receiver by setting `aad_auth`, please read [the configuration instruction of the AAD application](https://docs.microsoft.com/azure/azure-monitor/platform/action-groups#secure-webhook).
*/
@JvmName("vrspnjwvahrgmwqa")
public suspend fun aadAuth(`value`: ActionGroupWebhookReceiverAadAuthArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.aadAuth = mapped
}
/**
* @param argument The `aad_auth` block as defined below.
* > **NOTE:** Before adding a secure webhook receiver by setting `aad_auth`, please read [the configuration instruction of the AAD application](https://docs.microsoft.com/azure/azure-monitor/platform/action-groups#secure-webhook).
*/
@JvmName("dltcuifiuuuffpfm")
public suspend fun aadAuth(argument: suspend ActionGroupWebhookReceiverAadAuthArgsBuilder.() -> Unit) {
val toBeMapped = ActionGroupWebhookReceiverAadAuthArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.aadAuth = mapped
}
/**
* @param value The name of the webhook receiver. Names must be unique (case-insensitive) across all receivers within an action group.
*/
@JvmName("prpmlkrxguteggsq")
public suspend fun name(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value The URI where webhooks should be sent.
*/
@JvmName("rtksjbdagihcaylt")
public suspend fun serviceUri(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.serviceUri = mapped
}
/**
* @param value Enables or disables the common alert schema.
*/
@JvmName("iwcxvjcyybeeeggx")
public suspend fun useCommonAlertSchema(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.useCommonAlertSchema = mapped
}
internal fun build(): ActionGroupWebhookReceiverArgs = ActionGroupWebhookReceiverArgs(
aadAuth = aadAuth,
name = name ?: throw PulumiNullFieldException("name"),
serviceUri = serviceUri ?: throw PulumiNullFieldException("serviceUri"),
useCommonAlertSchema = useCommonAlertSchema,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy