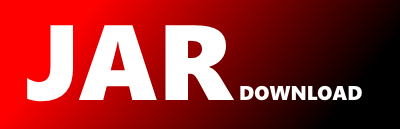
com.pulumi.azure.monitoring.kotlin.inputs.ScheduledQueryRulesAlertTriggerArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.monitoring.kotlin.inputs
import com.pulumi.azure.monitoring.inputs.ScheduledQueryRulesAlertTriggerArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Double
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property metricTrigger A `metric_trigger` block as defined above. Trigger condition for metric query rule.
* @property operator Evaluation operation for rule - 'GreaterThan', GreaterThanOrEqual', 'LessThan', or 'LessThanOrEqual'.
* @property threshold Result or count threshold based on which rule should be triggered. Values must be between 0 and 10000 inclusive.
*/
public data class ScheduledQueryRulesAlertTriggerArgs(
public val metricTrigger: Output? = null,
public val `operator`: Output,
public val threshold: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.monitoring.inputs.ScheduledQueryRulesAlertTriggerArgs =
com.pulumi.azure.monitoring.inputs.ScheduledQueryRulesAlertTriggerArgs.builder()
.metricTrigger(metricTrigger?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.`operator`(`operator`.applyValue({ args0 -> args0 }))
.threshold(threshold.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ScheduledQueryRulesAlertTriggerArgs].
*/
@PulumiTagMarker
public class ScheduledQueryRulesAlertTriggerArgsBuilder internal constructor() {
private var metricTrigger: Output? = null
private var `operator`: Output? = null
private var threshold: Output? = null
/**
* @param value A `metric_trigger` block as defined above. Trigger condition for metric query rule.
*/
@JvmName("tbpceiinmehohnrb")
public suspend fun metricTrigger(`value`: Output) {
this.metricTrigger = value
}
/**
* @param value Evaluation operation for rule - 'GreaterThan', GreaterThanOrEqual', 'LessThan', or 'LessThanOrEqual'.
*/
@JvmName("lnfmcpxxqswkoltm")
public suspend fun `operator`(`value`: Output) {
this.`operator` = value
}
/**
* @param value Result or count threshold based on which rule should be triggered. Values must be between 0 and 10000 inclusive.
*/
@JvmName("tusmvjfptuegnnex")
public suspend fun threshold(`value`: Output) {
this.threshold = value
}
/**
* @param value A `metric_trigger` block as defined above. Trigger condition for metric query rule.
*/
@JvmName("icakelhmmtgjymkt")
public suspend fun metricTrigger(`value`: ScheduledQueryRulesAlertTriggerMetricTriggerArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.metricTrigger = mapped
}
/**
* @param argument A `metric_trigger` block as defined above. Trigger condition for metric query rule.
*/
@JvmName("ojkenmhwmsqmntxf")
public suspend fun metricTrigger(argument: suspend ScheduledQueryRulesAlertTriggerMetricTriggerArgsBuilder.() -> Unit) {
val toBeMapped = ScheduledQueryRulesAlertTriggerMetricTriggerArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.metricTrigger = mapped
}
/**
* @param value Evaluation operation for rule - 'GreaterThan', GreaterThanOrEqual', 'LessThan', or 'LessThanOrEqual'.
*/
@JvmName("vvtiislcxconkrcd")
public suspend fun `operator`(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.`operator` = mapped
}
/**
* @param value Result or count threshold based on which rule should be triggered. Values must be between 0 and 10000 inclusive.
*/
@JvmName("wohkatktxgldfydn")
public suspend fun threshold(`value`: Double) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.threshold = mapped
}
internal fun build(): ScheduledQueryRulesAlertTriggerArgs = ScheduledQueryRulesAlertTriggerArgs(
metricTrigger = metricTrigger,
`operator` = `operator` ?: throw PulumiNullFieldException("operator"),
threshold = threshold ?: throw PulumiNullFieldException("threshold"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy