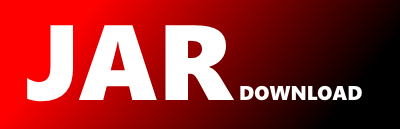
com.pulumi.azure.mssql.kotlin.inputs.DatabaseImportArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.mssql.kotlin.inputs
import com.pulumi.azure.mssql.inputs.DatabaseImportArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property administratorLogin Specifies the name of the SQL administrator.
* @property administratorLoginPassword Specifies the password of the SQL administrator.
* @property authenticationType Specifies the type of authentication used to access the server. Valid values are `SQL` or `ADPassword`.
* @property storageAccountId The resource id for the storage account used to store BACPAC file. If set, private endpoint connection will be created for the storage account. Must match storage account used for storage_uri parameter.
* @property storageKey Specifies the access key for the storage account.
* @property storageKeyType Specifies the type of access key for the storage account. Valid values are `StorageAccessKey` or `SharedAccessKey`.
* @property storageUri Specifies the blob URI of the .bacpac file.
*/
public data class DatabaseImportArgs(
public val administratorLogin: Output,
public val administratorLoginPassword: Output,
public val authenticationType: Output,
public val storageAccountId: Output? = null,
public val storageKey: Output,
public val storageKeyType: Output,
public val storageUri: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.mssql.inputs.DatabaseImportArgs =
com.pulumi.azure.mssql.inputs.DatabaseImportArgs.builder()
.administratorLogin(administratorLogin.applyValue({ args0 -> args0 }))
.administratorLoginPassword(administratorLoginPassword.applyValue({ args0 -> args0 }))
.authenticationType(authenticationType.applyValue({ args0 -> args0 }))
.storageAccountId(storageAccountId?.applyValue({ args0 -> args0 }))
.storageKey(storageKey.applyValue({ args0 -> args0 }))
.storageKeyType(storageKeyType.applyValue({ args0 -> args0 }))
.storageUri(storageUri.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [DatabaseImportArgs].
*/
@PulumiTagMarker
public class DatabaseImportArgsBuilder internal constructor() {
private var administratorLogin: Output? = null
private var administratorLoginPassword: Output? = null
private var authenticationType: Output? = null
private var storageAccountId: Output? = null
private var storageKey: Output? = null
private var storageKeyType: Output? = null
private var storageUri: Output? = null
/**
* @param value Specifies the name of the SQL administrator.
*/
@JvmName("kqtenadoldurnxtt")
public suspend fun administratorLogin(`value`: Output) {
this.administratorLogin = value
}
/**
* @param value Specifies the password of the SQL administrator.
*/
@JvmName("ohxqjubrdnpnuill")
public suspend fun administratorLoginPassword(`value`: Output) {
this.administratorLoginPassword = value
}
/**
* @param value Specifies the type of authentication used to access the server. Valid values are `SQL` or `ADPassword`.
*/
@JvmName("kkryssgfghxirjtc")
public suspend fun authenticationType(`value`: Output) {
this.authenticationType = value
}
/**
* @param value The resource id for the storage account used to store BACPAC file. If set, private endpoint connection will be created for the storage account. Must match storage account used for storage_uri parameter.
*/
@JvmName("jwykblkqeelcdmnp")
public suspend fun storageAccountId(`value`: Output) {
this.storageAccountId = value
}
/**
* @param value Specifies the access key for the storage account.
*/
@JvmName("fylyolekiopuplty")
public suspend fun storageKey(`value`: Output) {
this.storageKey = value
}
/**
* @param value Specifies the type of access key for the storage account. Valid values are `StorageAccessKey` or `SharedAccessKey`.
*/
@JvmName("fjosnejtvlwocdcd")
public suspend fun storageKeyType(`value`: Output) {
this.storageKeyType = value
}
/**
* @param value Specifies the blob URI of the .bacpac file.
*/
@JvmName("putmmpaytwubmdpr")
public suspend fun storageUri(`value`: Output) {
this.storageUri = value
}
/**
* @param value Specifies the name of the SQL administrator.
*/
@JvmName("xsluchtjcgfvqbdv")
public suspend fun administratorLogin(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.administratorLogin = mapped
}
/**
* @param value Specifies the password of the SQL administrator.
*/
@JvmName("slvntpokmmqdcjnw")
public suspend fun administratorLoginPassword(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.administratorLoginPassword = mapped
}
/**
* @param value Specifies the type of authentication used to access the server. Valid values are `SQL` or `ADPassword`.
*/
@JvmName("iornefhfewhkisrn")
public suspend fun authenticationType(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.authenticationType = mapped
}
/**
* @param value The resource id for the storage account used to store BACPAC file. If set, private endpoint connection will be created for the storage account. Must match storage account used for storage_uri parameter.
*/
@JvmName("plcfmcflvveipivv")
public suspend fun storageAccountId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.storageAccountId = mapped
}
/**
* @param value Specifies the access key for the storage account.
*/
@JvmName("nuadfxjabucjvghr")
public suspend fun storageKey(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.storageKey = mapped
}
/**
* @param value Specifies the type of access key for the storage account. Valid values are `StorageAccessKey` or `SharedAccessKey`.
*/
@JvmName("isrnkushreroxbdu")
public suspend fun storageKeyType(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.storageKeyType = mapped
}
/**
* @param value Specifies the blob URI of the .bacpac file.
*/
@JvmName("wbtjatqhdcadqmrn")
public suspend fun storageUri(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.storageUri = mapped
}
internal fun build(): DatabaseImportArgs = DatabaseImportArgs(
administratorLogin = administratorLogin ?: throw PulumiNullFieldException("administratorLogin"),
administratorLoginPassword = administratorLoginPassword ?: throw
PulumiNullFieldException("administratorLoginPassword"),
authenticationType = authenticationType ?: throw PulumiNullFieldException("authenticationType"),
storageAccountId = storageAccountId,
storageKey = storageKey ?: throw PulumiNullFieldException("storageKey"),
storageKeyType = storageKeyType ?: throw PulumiNullFieldException("storageKeyType"),
storageUri = storageUri ?: throw PulumiNullFieldException("storageUri"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy