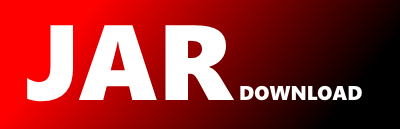
com.pulumi.azure.mssql.kotlin.inputs.VirtualMachineSqlInstanceArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.mssql.kotlin.inputs
import com.pulumi.azure.mssql.inputs.VirtualMachineSqlInstanceArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property adhocWorkloadsOptimizationEnabled Specifies if the SQL Server is optimized for adhoc workloads. Possible values are `true` and `false`. Defaults to `false`.
* @property collation Collation of the SQL Server. Defaults to `SQL_Latin1_General_CP1_CI_AS`. Changing this forces a new resource to be created.
* @property instantFileInitializationEnabled Specifies if Instant File Initialization is enabled for the SQL Server. Possible values are `true` and `false`. Defaults to `false`. Changing this forces a new resource to be created.
* @property lockPagesInMemoryEnabled Specifies if Lock Pages in Memory is enabled for the SQL Server. Possible values are `true` and `false`. Defaults to `false`. Changing this forces a new resource to be created.
* @property maxDop Maximum Degree of Parallelism of the SQL Server. Possible values are between `0` and `32767`. Defaults to `0`.
* @property maxServerMemoryMb Maximum amount memory that SQL Server Memory Manager can allocate to the SQL Server process. Possible values are between `128` and `2147483647` Defaults to `2147483647`.
* @property minServerMemoryMb Minimum amount memory that SQL Server Memory Manager can allocate to the SQL Server process. Possible values are between `0` and `2147483647` Defaults to `0`.
* > **NOTE:** `max_server_memory_mb` must be greater than or equal to `min_server_memory_mb`
*/
public data class VirtualMachineSqlInstanceArgs(
public val adhocWorkloadsOptimizationEnabled: Output? = null,
public val collation: Output? = null,
public val instantFileInitializationEnabled: Output? = null,
public val lockPagesInMemoryEnabled: Output? = null,
public val maxDop: Output? = null,
public val maxServerMemoryMb: Output? = null,
public val minServerMemoryMb: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.mssql.inputs.VirtualMachineSqlInstanceArgs =
com.pulumi.azure.mssql.inputs.VirtualMachineSqlInstanceArgs.builder()
.adhocWorkloadsOptimizationEnabled(
adhocWorkloadsOptimizationEnabled?.applyValue({ args0 ->
args0
}),
)
.collation(collation?.applyValue({ args0 -> args0 }))
.instantFileInitializationEnabled(instantFileInitializationEnabled?.applyValue({ args0 -> args0 }))
.lockPagesInMemoryEnabled(lockPagesInMemoryEnabled?.applyValue({ args0 -> args0 }))
.maxDop(maxDop?.applyValue({ args0 -> args0 }))
.maxServerMemoryMb(maxServerMemoryMb?.applyValue({ args0 -> args0 }))
.minServerMemoryMb(minServerMemoryMb?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [VirtualMachineSqlInstanceArgs].
*/
@PulumiTagMarker
public class VirtualMachineSqlInstanceArgsBuilder internal constructor() {
private var adhocWorkloadsOptimizationEnabled: Output? = null
private var collation: Output? = null
private var instantFileInitializationEnabled: Output? = null
private var lockPagesInMemoryEnabled: Output? = null
private var maxDop: Output? = null
private var maxServerMemoryMb: Output? = null
private var minServerMemoryMb: Output? = null
/**
* @param value Specifies if the SQL Server is optimized for adhoc workloads. Possible values are `true` and `false`. Defaults to `false`.
*/
@JvmName("oqjmciudafjlrofr")
public suspend fun adhocWorkloadsOptimizationEnabled(`value`: Output) {
this.adhocWorkloadsOptimizationEnabled = value
}
/**
* @param value Collation of the SQL Server. Defaults to `SQL_Latin1_General_CP1_CI_AS`. Changing this forces a new resource to be created.
*/
@JvmName("abvthbmsvinmetin")
public suspend fun collation(`value`: Output) {
this.collation = value
}
/**
* @param value Specifies if Instant File Initialization is enabled for the SQL Server. Possible values are `true` and `false`. Defaults to `false`. Changing this forces a new resource to be created.
*/
@JvmName("dwnvrnnfklhxtmkk")
public suspend fun instantFileInitializationEnabled(`value`: Output) {
this.instantFileInitializationEnabled = value
}
/**
* @param value Specifies if Lock Pages in Memory is enabled for the SQL Server. Possible values are `true` and `false`. Defaults to `false`. Changing this forces a new resource to be created.
*/
@JvmName("fvwyxxfhirfegira")
public suspend fun lockPagesInMemoryEnabled(`value`: Output) {
this.lockPagesInMemoryEnabled = value
}
/**
* @param value Maximum Degree of Parallelism of the SQL Server. Possible values are between `0` and `32767`. Defaults to `0`.
*/
@JvmName("fdnlffkaenuxkjas")
public suspend fun maxDop(`value`: Output) {
this.maxDop = value
}
/**
* @param value Maximum amount memory that SQL Server Memory Manager can allocate to the SQL Server process. Possible values are between `128` and `2147483647` Defaults to `2147483647`.
*/
@JvmName("bkdkbnnkbeghuwyf")
public suspend fun maxServerMemoryMb(`value`: Output) {
this.maxServerMemoryMb = value
}
/**
* @param value Minimum amount memory that SQL Server Memory Manager can allocate to the SQL Server process. Possible values are between `0` and `2147483647` Defaults to `0`.
* > **NOTE:** `max_server_memory_mb` must be greater than or equal to `min_server_memory_mb`
*/
@JvmName("dewhcnawalsfxtic")
public suspend fun minServerMemoryMb(`value`: Output) {
this.minServerMemoryMb = value
}
/**
* @param value Specifies if the SQL Server is optimized for adhoc workloads. Possible values are `true` and `false`. Defaults to `false`.
*/
@JvmName("uilkgpgpqqgvvolp")
public suspend fun adhocWorkloadsOptimizationEnabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.adhocWorkloadsOptimizationEnabled = mapped
}
/**
* @param value Collation of the SQL Server. Defaults to `SQL_Latin1_General_CP1_CI_AS`. Changing this forces a new resource to be created.
*/
@JvmName("wcuwdohouodkkedk")
public suspend fun collation(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.collation = mapped
}
/**
* @param value Specifies if Instant File Initialization is enabled for the SQL Server. Possible values are `true` and `false`. Defaults to `false`. Changing this forces a new resource to be created.
*/
@JvmName("tviegctdpubhyaqe")
public suspend fun instantFileInitializationEnabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.instantFileInitializationEnabled = mapped
}
/**
* @param value Specifies if Lock Pages in Memory is enabled for the SQL Server. Possible values are `true` and `false`. Defaults to `false`. Changing this forces a new resource to be created.
*/
@JvmName("kkvwujaoerwpxgxn")
public suspend fun lockPagesInMemoryEnabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.lockPagesInMemoryEnabled = mapped
}
/**
* @param value Maximum Degree of Parallelism of the SQL Server. Possible values are between `0` and `32767`. Defaults to `0`.
*/
@JvmName("xswklbxfuwwjwhhl")
public suspend fun maxDop(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxDop = mapped
}
/**
* @param value Maximum amount memory that SQL Server Memory Manager can allocate to the SQL Server process. Possible values are between `128` and `2147483647` Defaults to `2147483647`.
*/
@JvmName("ypwgxftqwcubsaqc")
public suspend fun maxServerMemoryMb(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxServerMemoryMb = mapped
}
/**
* @param value Minimum amount memory that SQL Server Memory Manager can allocate to the SQL Server process. Possible values are between `0` and `2147483647` Defaults to `0`.
* > **NOTE:** `max_server_memory_mb` must be greater than or equal to `min_server_memory_mb`
*/
@JvmName("fpekgiexrjqnfoma")
public suspend fun minServerMemoryMb(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.minServerMemoryMb = mapped
}
internal fun build(): VirtualMachineSqlInstanceArgs = VirtualMachineSqlInstanceArgs(
adhocWorkloadsOptimizationEnabled = adhocWorkloadsOptimizationEnabled,
collation = collation,
instantFileInitializationEnabled = instantFileInitializationEnabled,
lockPagesInMemoryEnabled = lockPagesInMemoryEnabled,
maxDop = maxDop,
maxServerMemoryMb = maxServerMemoryMb,
minServerMemoryMb = minServerMemoryMb,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy