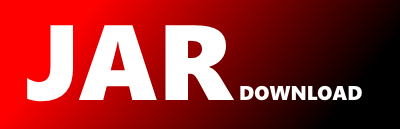
com.pulumi.azure.netapp.kotlin.inputs.VolumeDataProtectionReplicationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.netapp.kotlin.inputs
import com.pulumi.azure.netapp.inputs.VolumeDataProtectionReplicationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property endpointType The endpoint type, default value is `dst` for destination.
* @property remoteVolumeLocation Location of the primary volume. Changing this forces a new resource to be created.
* @property remoteVolumeResourceId Resource ID of the primary volume.
* @property replicationFrequency Replication frequency, supported values are '10minutes', 'hourly', 'daily', values are case sensitive.
* A full example of the `data_protection_replication` attribute can be found in the `./examples/netapp/volume_crr` directory within the GitHub Repository
* > **NOTE:** `data_protection_replication` can be defined only once per secondary volume, adding a second instance of it is not supported.
*/
public data class VolumeDataProtectionReplicationArgs(
public val endpointType: Output? = null,
public val remoteVolumeLocation: Output,
public val remoteVolumeResourceId: Output,
public val replicationFrequency: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.netapp.inputs.VolumeDataProtectionReplicationArgs =
com.pulumi.azure.netapp.inputs.VolumeDataProtectionReplicationArgs.builder()
.endpointType(endpointType?.applyValue({ args0 -> args0 }))
.remoteVolumeLocation(remoteVolumeLocation.applyValue({ args0 -> args0 }))
.remoteVolumeResourceId(remoteVolumeResourceId.applyValue({ args0 -> args0 }))
.replicationFrequency(replicationFrequency.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [VolumeDataProtectionReplicationArgs].
*/
@PulumiTagMarker
public class VolumeDataProtectionReplicationArgsBuilder internal constructor() {
private var endpointType: Output? = null
private var remoteVolumeLocation: Output? = null
private var remoteVolumeResourceId: Output? = null
private var replicationFrequency: Output? = null
/**
* @param value The endpoint type, default value is `dst` for destination.
*/
@JvmName("dbpnryupwxsdoegy")
public suspend fun endpointType(`value`: Output) {
this.endpointType = value
}
/**
* @param value Location of the primary volume. Changing this forces a new resource to be created.
*/
@JvmName("prekyhgusjkvvcvi")
public suspend fun remoteVolumeLocation(`value`: Output) {
this.remoteVolumeLocation = value
}
/**
* @param value Resource ID of the primary volume.
*/
@JvmName("tnxwlvipxlnffmix")
public suspend fun remoteVolumeResourceId(`value`: Output) {
this.remoteVolumeResourceId = value
}
/**
* @param value Replication frequency, supported values are '10minutes', 'hourly', 'daily', values are case sensitive.
* A full example of the `data_protection_replication` attribute can be found in the `./examples/netapp/volume_crr` directory within the GitHub Repository
* > **NOTE:** `data_protection_replication` can be defined only once per secondary volume, adding a second instance of it is not supported.
*/
@JvmName("pmfwycfgukptvoun")
public suspend fun replicationFrequency(`value`: Output) {
this.replicationFrequency = value
}
/**
* @param value The endpoint type, default value is `dst` for destination.
*/
@JvmName("soydppjjsxhpsmcc")
public suspend fun endpointType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.endpointType = mapped
}
/**
* @param value Location of the primary volume. Changing this forces a new resource to be created.
*/
@JvmName("rmryxacftghyqinb")
public suspend fun remoteVolumeLocation(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.remoteVolumeLocation = mapped
}
/**
* @param value Resource ID of the primary volume.
*/
@JvmName("qwvgjsrimgmxkxhd")
public suspend fun remoteVolumeResourceId(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.remoteVolumeResourceId = mapped
}
/**
* @param value Replication frequency, supported values are '10minutes', 'hourly', 'daily', values are case sensitive.
* A full example of the `data_protection_replication` attribute can be found in the `./examples/netapp/volume_crr` directory within the GitHub Repository
* > **NOTE:** `data_protection_replication` can be defined only once per secondary volume, adding a second instance of it is not supported.
*/
@JvmName("givndsscakmuonho")
public suspend fun replicationFrequency(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.replicationFrequency = mapped
}
internal fun build(): VolumeDataProtectionReplicationArgs = VolumeDataProtectionReplicationArgs(
endpointType = endpointType,
remoteVolumeLocation = remoteVolumeLocation ?: throw
PulumiNullFieldException("remoteVolumeLocation"),
remoteVolumeResourceId = remoteVolumeResourceId ?: throw
PulumiNullFieldException("remoteVolumeResourceId"),
replicationFrequency = replicationFrequency ?: throw
PulumiNullFieldException("replicationFrequency"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy