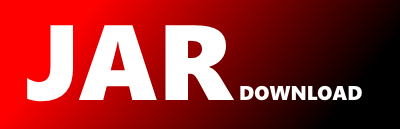
com.pulumi.azure.network.kotlin.inputs.ApplicationGatewaySslPolicyArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.network.kotlin.inputs
import com.pulumi.azure.network.inputs.ApplicationGatewaySslPolicyArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property cipherSuites
* @property disabledProtocols A list of SSL Protocols which should be disabled on this Application Gateway. Possible values are `TLSv1_0`, `TLSv1_1`, `TLSv1_2` and `TLSv1_3`.
* > **NOTE:** `disabled_protocols` cannot be set when `policy_name` or `policy_type` are set.
* @property minProtocolVersion
* @property policyName
* @property policyType The Type of the Policy. Possible values are `Predefined`, `Custom` and `CustomV2`.
* > **NOTE:** `policy_type` is Required when `policy_name` is set - cannot be set if `disabled_protocols` is set.
*/
public data class ApplicationGatewaySslPolicyArgs(
public val cipherSuites: Output>? = null,
public val disabledProtocols: Output>? = null,
public val minProtocolVersion: Output? = null,
public val policyName: Output? = null,
public val policyType: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.network.inputs.ApplicationGatewaySslPolicyArgs =
com.pulumi.azure.network.inputs.ApplicationGatewaySslPolicyArgs.builder()
.cipherSuites(cipherSuites?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.disabledProtocols(disabledProtocols?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.minProtocolVersion(minProtocolVersion?.applyValue({ args0 -> args0 }))
.policyName(policyName?.applyValue({ args0 -> args0 }))
.policyType(policyType?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ApplicationGatewaySslPolicyArgs].
*/
@PulumiTagMarker
public class ApplicationGatewaySslPolicyArgsBuilder internal constructor() {
private var cipherSuites: Output>? = null
private var disabledProtocols: Output>? = null
private var minProtocolVersion: Output? = null
private var policyName: Output? = null
private var policyType: Output? = null
/**
* @param value
*/
@JvmName("slmbtumiaxetedau")
public suspend fun cipherSuites(`value`: Output>) {
this.cipherSuites = value
}
@JvmName("baeawwwtbiuhvkql")
public suspend fun cipherSuites(vararg values: Output) {
this.cipherSuites = Output.all(values.asList())
}
/**
* @param values
*/
@JvmName("udbfclrkjnboemye")
public suspend fun cipherSuites(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy