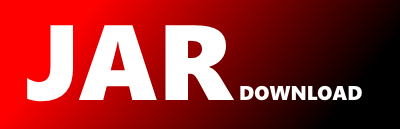
com.pulumi.azure.network.kotlin.outputs.VpnGatewayConnectionVpnLink.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.network.kotlin.outputs
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
*
* @property bandwidthMbps The expected connection bandwidth in MBPS. Defaults to `10`.
* @property bgpEnabled Should the BGP be enabled? Defaults to `false`. Changing this forces a new VPN Gateway Connection to be created.
* @property connectionMode The connection mode of this VPN Link. Possible values are `Default`, `InitiatorOnly` and `ResponderOnly`. Defaults to `Default`.
* @property customBgpAddresses One or more `custom_bgp_address` blocks as defined below.
* @property egressNatRuleIds A list of the egress NAT Rule Ids.
* @property ingressNatRuleIds A list of the ingress NAT Rule Ids.
* @property ipsecPolicies One or more `ipsec_policy` blocks as defined above.
* @property localAzureIpAddressEnabled Whether to use local Azure IP to initiate connection? Defaults to `false`.
* @property name The name which should be used for this VPN Link Connection.
* @property policyBasedTrafficSelectorEnabled Whether to enable policy-based traffic selectors? Defaults to `false`.
* @property protocol The protocol used for this VPN Link Connection. Possible values are `IKEv1` and `IKEv2`. Defaults to `IKEv2`.
* @property ratelimitEnabled Should the rate limit be enabled? Defaults to `false`.
* @property routeWeight Routing weight for this VPN Link Connection. Defaults to `0`.
* @property sharedKey SharedKey for this VPN Link Connection.
* @property vpnSiteLinkId The ID of the connected VPN Site Link. Changing this forces a new VPN Gateway Connection to be created.
*/
public data class VpnGatewayConnectionVpnLink(
public val bandwidthMbps: Int? = null,
public val bgpEnabled: Boolean? = null,
public val connectionMode: String? = null,
public val customBgpAddresses: List? = null,
public val egressNatRuleIds: List? = null,
public val ingressNatRuleIds: List? = null,
public val ipsecPolicies: List? = null,
public val localAzureIpAddressEnabled: Boolean? = null,
public val name: String,
public val policyBasedTrafficSelectorEnabled: Boolean? = null,
public val protocol: String? = null,
public val ratelimitEnabled: Boolean? = null,
public val routeWeight: Int? = null,
public val sharedKey: String? = null,
public val vpnSiteLinkId: String,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.azure.network.outputs.VpnGatewayConnectionVpnLink): VpnGatewayConnectionVpnLink = VpnGatewayConnectionVpnLink(
bandwidthMbps = javaType.bandwidthMbps().map({ args0 -> args0 }).orElse(null),
bgpEnabled = javaType.bgpEnabled().map({ args0 -> args0 }).orElse(null),
connectionMode = javaType.connectionMode().map({ args0 -> args0 }).orElse(null),
customBgpAddresses = javaType.customBgpAddresses().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.network.kotlin.outputs.VpnGatewayConnectionVpnLinkCustomBgpAddress.Companion.toKotlin(args0)
})
}),
egressNatRuleIds = javaType.egressNatRuleIds().map({ args0 -> args0 }),
ingressNatRuleIds = javaType.ingressNatRuleIds().map({ args0 -> args0 }),
ipsecPolicies = javaType.ipsecPolicies().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.network.kotlin.outputs.VpnGatewayConnectionVpnLinkIpsecPolicy.Companion.toKotlin(args0)
})
}),
localAzureIpAddressEnabled = javaType.localAzureIpAddressEnabled().map({ args0 ->
args0
}).orElse(null),
name = javaType.name(),
policyBasedTrafficSelectorEnabled = javaType.policyBasedTrafficSelectorEnabled().map({ args0 ->
args0
}).orElse(null),
protocol = javaType.protocol().map({ args0 -> args0 }).orElse(null),
ratelimitEnabled = javaType.ratelimitEnabled().map({ args0 -> args0 }).orElse(null),
routeWeight = javaType.routeWeight().map({ args0 -> args0 }).orElse(null),
sharedKey = javaType.sharedKey().map({ args0 -> args0 }).orElse(null),
vpnSiteLinkId = javaType.vpnSiteLinkId(),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy