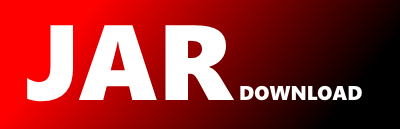
com.pulumi.azure.notificationhub.kotlin.inputs.HubApnsCredentialArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.notificationhub.kotlin.inputs
import com.pulumi.azure.notificationhub.inputs.HubApnsCredentialArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property applicationMode The Application Mode which defines which server the APNS Messages should be sent to. Possible values are `Production` and `Sandbox`.
* @property bundleId The Bundle ID of the iOS/macOS application to send push notifications for, such as `com.org.example`.
* @property keyId The Apple Push Notifications Service (APNS) Key.
* @property teamId The ID of the team the Token.
* @property token The Push Token associated with the Apple Developer Account. This is the contents of the `key` downloaded from [the Apple Developer Portal](https://developer.apple.com/account/ios/authkey/) between the `-----BEGIN PRIVATE KEY-----` and `-----END PRIVATE KEY-----` blocks.
*/
public data class HubApnsCredentialArgs(
public val applicationMode: Output,
public val bundleId: Output,
public val keyId: Output,
public val teamId: Output,
public val token: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.notificationhub.inputs.HubApnsCredentialArgs =
com.pulumi.azure.notificationhub.inputs.HubApnsCredentialArgs.builder()
.applicationMode(applicationMode.applyValue({ args0 -> args0 }))
.bundleId(bundleId.applyValue({ args0 -> args0 }))
.keyId(keyId.applyValue({ args0 -> args0 }))
.teamId(teamId.applyValue({ args0 -> args0 }))
.token(token.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [HubApnsCredentialArgs].
*/
@PulumiTagMarker
public class HubApnsCredentialArgsBuilder internal constructor() {
private var applicationMode: Output? = null
private var bundleId: Output? = null
private var keyId: Output? = null
private var teamId: Output? = null
private var token: Output? = null
/**
* @param value The Application Mode which defines which server the APNS Messages should be sent to. Possible values are `Production` and `Sandbox`.
*/
@JvmName("nkgyvvdtamjuuybv")
public suspend fun applicationMode(`value`: Output) {
this.applicationMode = value
}
/**
* @param value The Bundle ID of the iOS/macOS application to send push notifications for, such as `com.org.example`.
*/
@JvmName("uodsokmbsqybegar")
public suspend fun bundleId(`value`: Output) {
this.bundleId = value
}
/**
* @param value The Apple Push Notifications Service (APNS) Key.
*/
@JvmName("sfjyglmavqoxfppp")
public suspend fun keyId(`value`: Output) {
this.keyId = value
}
/**
* @param value The ID of the team the Token.
*/
@JvmName("cnauycjbfeyhxgrh")
public suspend fun teamId(`value`: Output) {
this.teamId = value
}
/**
* @param value The Push Token associated with the Apple Developer Account. This is the contents of the `key` downloaded from [the Apple Developer Portal](https://developer.apple.com/account/ios/authkey/) between the `-----BEGIN PRIVATE KEY-----` and `-----END PRIVATE KEY-----` blocks.
*/
@JvmName("vkowxgkaijhakjfd")
public suspend fun token(`value`: Output) {
this.token = value
}
/**
* @param value The Application Mode which defines which server the APNS Messages should be sent to. Possible values are `Production` and `Sandbox`.
*/
@JvmName("ahwtxqgdsgemiecd")
public suspend fun applicationMode(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.applicationMode = mapped
}
/**
* @param value The Bundle ID of the iOS/macOS application to send push notifications for, such as `com.org.example`.
*/
@JvmName("vabyadncwavcgpjg")
public suspend fun bundleId(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.bundleId = mapped
}
/**
* @param value The Apple Push Notifications Service (APNS) Key.
*/
@JvmName("imyrpqfygjfjxmyf")
public suspend fun keyId(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.keyId = mapped
}
/**
* @param value The ID of the team the Token.
*/
@JvmName("nbdapmrhnxmmstbc")
public suspend fun teamId(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.teamId = mapped
}
/**
* @param value The Push Token associated with the Apple Developer Account. This is the contents of the `key` downloaded from [the Apple Developer Portal](https://developer.apple.com/account/ios/authkey/) between the `-----BEGIN PRIVATE KEY-----` and `-----END PRIVATE KEY-----` blocks.
*/
@JvmName("owvewobsnnsxwaxn")
public suspend fun token(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.token = mapped
}
internal fun build(): HubApnsCredentialArgs = HubApnsCredentialArgs(
applicationMode = applicationMode ?: throw PulumiNullFieldException("applicationMode"),
bundleId = bundleId ?: throw PulumiNullFieldException("bundleId"),
keyId = keyId ?: throw PulumiNullFieldException("keyId"),
teamId = teamId ?: throw PulumiNullFieldException("teamId"),
token = token ?: throw PulumiNullFieldException("token"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy