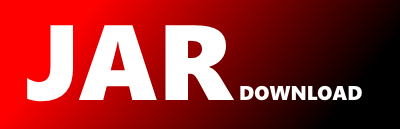
com.pulumi.azure.pim.kotlin.inputs.RoleManagementPolicyActivationRulesArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.pim.kotlin.inputs
import com.pulumi.azure.pim.inputs.RoleManagementPolicyActivationRulesArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property approvalStage An `approval_stage` block as defined below.
* @property maximumDuration The maximum length of time an activated role can be valid, in an ISO8601 Duration format (e.g. `PT8H`). Valid range is `PT30M` to `PT23H30M`, in 30 minute increments, or `PT1D`.
* @property requireApproval Is approval required for activation. If `true` an `approval_stage` block must be provided.
* @property requireJustification Is a justification required during activation of the role.
* @property requireMultifactorAuthentication Is multi-factor authentication required to activate the role. Conflicts with `required_conditional_access_authentication_context`.
* @property requireTicketInfo Is ticket information requrired during activation of the role.
* @property requiredConditionalAccessAuthenticationContext The Entra ID Conditional Access context that must be present for activation. Conflicts with `require_multifactor_authentication`.
*/
public data class RoleManagementPolicyActivationRulesArgs(
public val approvalStage: Output? = null,
public val maximumDuration: Output? = null,
public val requireApproval: Output? = null,
public val requireJustification: Output? = null,
public val requireMultifactorAuthentication: Output? = null,
public val requireTicketInfo: Output? = null,
public val requiredConditionalAccessAuthenticationContext: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.pim.inputs.RoleManagementPolicyActivationRulesArgs =
com.pulumi.azure.pim.inputs.RoleManagementPolicyActivationRulesArgs.builder()
.approvalStage(approvalStage?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.maximumDuration(maximumDuration?.applyValue({ args0 -> args0 }))
.requireApproval(requireApproval?.applyValue({ args0 -> args0 }))
.requireJustification(requireJustification?.applyValue({ args0 -> args0 }))
.requireMultifactorAuthentication(requireMultifactorAuthentication?.applyValue({ args0 -> args0 }))
.requireTicketInfo(requireTicketInfo?.applyValue({ args0 -> args0 }))
.requiredConditionalAccessAuthenticationContext(
requiredConditionalAccessAuthenticationContext?.applyValue({ args0 ->
args0
}),
).build()
}
/**
* Builder for [RoleManagementPolicyActivationRulesArgs].
*/
@PulumiTagMarker
public class RoleManagementPolicyActivationRulesArgsBuilder internal constructor() {
private var approvalStage: Output? = null
private var maximumDuration: Output? = null
private var requireApproval: Output? = null
private var requireJustification: Output? = null
private var requireMultifactorAuthentication: Output? = null
private var requireTicketInfo: Output? = null
private var requiredConditionalAccessAuthenticationContext: Output? = null
/**
* @param value An `approval_stage` block as defined below.
*/
@JvmName("ysykufojcpktenso")
public suspend fun approvalStage(`value`: Output) {
this.approvalStage = value
}
/**
* @param value The maximum length of time an activated role can be valid, in an ISO8601 Duration format (e.g. `PT8H`). Valid range is `PT30M` to `PT23H30M`, in 30 minute increments, or `PT1D`.
*/
@JvmName("fqiavlgabrhqeplu")
public suspend fun maximumDuration(`value`: Output) {
this.maximumDuration = value
}
/**
* @param value Is approval required for activation. If `true` an `approval_stage` block must be provided.
*/
@JvmName("defdycnpncebuugw")
public suspend fun requireApproval(`value`: Output) {
this.requireApproval = value
}
/**
* @param value Is a justification required during activation of the role.
*/
@JvmName("vnwssaopdyttuobk")
public suspend fun requireJustification(`value`: Output) {
this.requireJustification = value
}
/**
* @param value Is multi-factor authentication required to activate the role. Conflicts with `required_conditional_access_authentication_context`.
*/
@JvmName("awaacnkmmccetnqt")
public suspend fun requireMultifactorAuthentication(`value`: Output) {
this.requireMultifactorAuthentication = value
}
/**
* @param value Is ticket information requrired during activation of the role.
*/
@JvmName("rgtqhixhougymvhr")
public suspend fun requireTicketInfo(`value`: Output) {
this.requireTicketInfo = value
}
/**
* @param value The Entra ID Conditional Access context that must be present for activation. Conflicts with `require_multifactor_authentication`.
*/
@JvmName("liuarpxnjiswqmlk")
public suspend fun requiredConditionalAccessAuthenticationContext(`value`: Output) {
this.requiredConditionalAccessAuthenticationContext = value
}
/**
* @param value An `approval_stage` block as defined below.
*/
@JvmName("sugvfilqerprdvxk")
public suspend fun approvalStage(`value`: RoleManagementPolicyActivationRulesApprovalStageArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.approvalStage = mapped
}
/**
* @param argument An `approval_stage` block as defined below.
*/
@JvmName("krmkfuyffqsnbfix")
public suspend fun approvalStage(argument: suspend RoleManagementPolicyActivationRulesApprovalStageArgsBuilder.() -> Unit) {
val toBeMapped = RoleManagementPolicyActivationRulesApprovalStageArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.approvalStage = mapped
}
/**
* @param value The maximum length of time an activated role can be valid, in an ISO8601 Duration format (e.g. `PT8H`). Valid range is `PT30M` to `PT23H30M`, in 30 minute increments, or `PT1D`.
*/
@JvmName("rajmkywpisrldmjv")
public suspend fun maximumDuration(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maximumDuration = mapped
}
/**
* @param value Is approval required for activation. If `true` an `approval_stage` block must be provided.
*/
@JvmName("dntdyvmjrlxgbann")
public suspend fun requireApproval(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.requireApproval = mapped
}
/**
* @param value Is a justification required during activation of the role.
*/
@JvmName("cfruwvycqwdryrpn")
public suspend fun requireJustification(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.requireJustification = mapped
}
/**
* @param value Is multi-factor authentication required to activate the role. Conflicts with `required_conditional_access_authentication_context`.
*/
@JvmName("cgplvhcjddnypgrv")
public suspend fun requireMultifactorAuthentication(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.requireMultifactorAuthentication = mapped
}
/**
* @param value Is ticket information requrired during activation of the role.
*/
@JvmName("gggjhvyapeyanvtq")
public suspend fun requireTicketInfo(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.requireTicketInfo = mapped
}
/**
* @param value The Entra ID Conditional Access context that must be present for activation. Conflicts with `require_multifactor_authentication`.
*/
@JvmName("ucgwujxinagkjels")
public suspend fun requiredConditionalAccessAuthenticationContext(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.requiredConditionalAccessAuthenticationContext = mapped
}
internal fun build(): RoleManagementPolicyActivationRulesArgs =
RoleManagementPolicyActivationRulesArgs(
approvalStage = approvalStage,
maximumDuration = maximumDuration,
requireApproval = requireApproval,
requireJustification = requireJustification,
requireMultifactorAuthentication = requireMultifactorAuthentication,
requireTicketInfo = requireTicketInfo,
requiredConditionalAccessAuthenticationContext = requiredConditionalAccessAuthenticationContext,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy