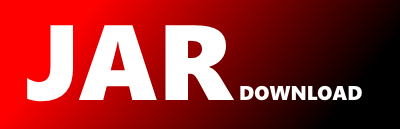
com.pulumi.azure.postgresql.kotlin.FlexibleServerActiveDirectoryAdministratorArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.postgresql.kotlin
import com.pulumi.azure.postgresql.FlexibleServerActiveDirectoryAdministratorArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Allows you to set a user or group as the AD administrator for a PostgreSQL Flexible Server.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* import * as azuread from "@pulumi/azuread";
* const current = azure.core.getClientConfig({});
* const example = current.then(current => azuread.getServicePrincipal({
* objectId: current.objectId,
* }));
* const exampleResourceGroup = new azure.core.ResourceGroup("example", {
* name: "example-resources",
* location: "West Europe",
* });
* const exampleFlexibleServer = new azure.postgresql.FlexibleServer("example", {
* name: "example-fs",
* resourceGroupName: exampleResourceGroup.name,
* location: exampleResourceGroup.location,
* administratorLogin: "adminTerraform",
* administratorPassword: "QAZwsx123",
* storageMb: 32768,
* version: "12",
* skuName: "GP_Standard_D2s_v3",
* zone: "2",
* authentication: {
* activeDirectoryAuthEnabled: true,
* tenantId: current.then(current => current.tenantId),
* },
* });
* const exampleFlexibleServerActiveDirectoryAdministrator = new azure.postgresql.FlexibleServerActiveDirectoryAdministrator("example", {
* serverName: exampleFlexibleServer.name,
* resourceGroupName: exampleResourceGroup.name,
* tenantId: current.then(current => current.tenantId),
* objectId: example.then(example => example.objectId),
* principalName: example.then(example => example.displayName),
* principalType: "ServicePrincipal",
* });
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* import pulumi_azuread as azuread
* current = azure.core.get_client_config()
* example = azuread.get_service_principal(object_id=current.object_id)
* example_resource_group = azure.core.ResourceGroup("example",
* name="example-resources",
* location="West Europe")
* example_flexible_server = azure.postgresql.FlexibleServer("example",
* name="example-fs",
* resource_group_name=example_resource_group.name,
* location=example_resource_group.location,
* administrator_login="adminTerraform",
* administrator_password="QAZwsx123",
* storage_mb=32768,
* version="12",
* sku_name="GP_Standard_D2s_v3",
* zone="2",
* authentication={
* "active_directory_auth_enabled": True,
* "tenant_id": current.tenant_id,
* })
* example_flexible_server_active_directory_administrator = azure.postgresql.FlexibleServerActiveDirectoryAdministrator("example",
* server_name=example_flexible_server.name,
* resource_group_name=example_resource_group.name,
* tenant_id=current.tenant_id,
* object_id=example.object_id,
* principal_name=example.display_name,
* principal_type="ServicePrincipal")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* using AzureAD = Pulumi.AzureAD;
* return await Deployment.RunAsync(() =>
* {
* var current = Azure.Core.GetClientConfig.Invoke();
* var example = AzureAD.GetServicePrincipal.Invoke(new()
* {
* ObjectId = current.Apply(getClientConfigResult => getClientConfigResult.ObjectId),
* });
* var exampleResourceGroup = new Azure.Core.ResourceGroup("example", new()
* {
* Name = "example-resources",
* Location = "West Europe",
* });
* var exampleFlexibleServer = new Azure.PostgreSql.FlexibleServer("example", new()
* {
* Name = "example-fs",
* ResourceGroupName = exampleResourceGroup.Name,
* Location = exampleResourceGroup.Location,
* AdministratorLogin = "adminTerraform",
* AdministratorPassword = "QAZwsx123",
* StorageMb = 32768,
* Version = "12",
* SkuName = "GP_Standard_D2s_v3",
* Zone = "2",
* Authentication = new Azure.PostgreSql.Inputs.FlexibleServerAuthenticationArgs
* {
* ActiveDirectoryAuthEnabled = true,
* TenantId = current.Apply(getClientConfigResult => getClientConfigResult.TenantId),
* },
* });
* var exampleFlexibleServerActiveDirectoryAdministrator = new Azure.PostgreSql.FlexibleServerActiveDirectoryAdministrator("example", new()
* {
* ServerName = exampleFlexibleServer.Name,
* ResourceGroupName = exampleResourceGroup.Name,
* TenantId = current.Apply(getClientConfigResult => getClientConfigResult.TenantId),
* ObjectId = example.Apply(getServicePrincipalResult => getServicePrincipalResult.ObjectId),
* PrincipalName = example.Apply(getServicePrincipalResult => getServicePrincipalResult.DisplayName),
* PrincipalType = "ServicePrincipal",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/core"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/postgresql"
* "github.com/pulumi/pulumi-azuread/sdk/v5/go/azuread"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* current, err := core.GetClientConfig(ctx, nil, nil)
* if err != nil {
* return err
* }
* example, err := azuread.LookupServicePrincipal(ctx, &azuread.LookupServicePrincipalArgs{
* ObjectId: pulumi.StringRef(current.ObjectId),
* }, nil)
* if err != nil {
* return err
* }
* exampleResourceGroup, err := core.NewResourceGroup(ctx, "example", &core.ResourceGroupArgs{
* Name: pulumi.String("example-resources"),
* Location: pulumi.String("West Europe"),
* })
* if err != nil {
* return err
* }
* exampleFlexibleServer, err := postgresql.NewFlexibleServer(ctx, "example", &postgresql.FlexibleServerArgs{
* Name: pulumi.String("example-fs"),
* ResourceGroupName: exampleResourceGroup.Name,
* Location: exampleResourceGroup.Location,
* AdministratorLogin: pulumi.String("adminTerraform"),
* AdministratorPassword: pulumi.String("QAZwsx123"),
* StorageMb: pulumi.Int(32768),
* Version: pulumi.String("12"),
* SkuName: pulumi.String("GP_Standard_D2s_v3"),
* Zone: pulumi.String("2"),
* Authentication: &postgresql.FlexibleServerAuthenticationArgs{
* ActiveDirectoryAuthEnabled: pulumi.Bool(true),
* TenantId: pulumi.String(current.TenantId),
* },
* })
* if err != nil {
* return err
* }
* _, err = postgresql.NewFlexibleServerActiveDirectoryAdministrator(ctx, "example", &postgresql.FlexibleServerActiveDirectoryAdministratorArgs{
* ServerName: exampleFlexibleServer.Name,
* ResourceGroupName: exampleResourceGroup.Name,
* TenantId: pulumi.String(current.TenantId),
* ObjectId: pulumi.String(example.ObjectId),
* PrincipalName: pulumi.String(example.DisplayName),
* PrincipalType: pulumi.String("ServicePrincipal"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.CoreFunctions;
* import com.pulumi.azuread.AzureadFunctions;
* import com.pulumi.azuread.inputs.GetServicePrincipalArgs;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.postgresql.FlexibleServer;
* import com.pulumi.azure.postgresql.FlexibleServerArgs;
* import com.pulumi.azure.postgresql.inputs.FlexibleServerAuthenticationArgs;
* import com.pulumi.azure.postgresql.FlexibleServerActiveDirectoryAdministrator;
* import com.pulumi.azure.postgresql.FlexibleServerActiveDirectoryAdministratorArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var current = CoreFunctions.getClientConfig();
* final var example = AzureadFunctions.getServicePrincipal(GetServicePrincipalArgs.builder()
* .objectId(current.applyValue(getClientConfigResult -> getClientConfigResult.objectId()))
* .build());
* var exampleResourceGroup = new ResourceGroup("exampleResourceGroup", ResourceGroupArgs.builder()
* .name("example-resources")
* .location("West Europe")
* .build());
* var exampleFlexibleServer = new FlexibleServer("exampleFlexibleServer", FlexibleServerArgs.builder()
* .name("example-fs")
* .resourceGroupName(exampleResourceGroup.name())
* .location(exampleResourceGroup.location())
* .administratorLogin("adminTerraform")
* .administratorPassword("QAZwsx123")
* .storageMb(32768)
* .version("12")
* .skuName("GP_Standard_D2s_v3")
* .zone("2")
* .authentication(FlexibleServerAuthenticationArgs.builder()
* .activeDirectoryAuthEnabled(true)
* .tenantId(current.applyValue(getClientConfigResult -> getClientConfigResult.tenantId()))
* .build())
* .build());
* var exampleFlexibleServerActiveDirectoryAdministrator = new FlexibleServerActiveDirectoryAdministrator("exampleFlexibleServerActiveDirectoryAdministrator", FlexibleServerActiveDirectoryAdministratorArgs.builder()
* .serverName(exampleFlexibleServer.name())
* .resourceGroupName(exampleResourceGroup.name())
* .tenantId(current.applyValue(getClientConfigResult -> getClientConfigResult.tenantId()))
* .objectId(example.applyValue(getServicePrincipalResult -> getServicePrincipalResult.objectId()))
* .principalName(example.applyValue(getServicePrincipalResult -> getServicePrincipalResult.displayName()))
* .principalType("ServicePrincipal")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* exampleResourceGroup:
* type: azure:core:ResourceGroup
* name: example
* properties:
* name: example-resources
* location: West Europe
* exampleFlexibleServer:
* type: azure:postgresql:FlexibleServer
* name: example
* properties:
* name: example-fs
* resourceGroupName: ${exampleResourceGroup.name}
* location: ${exampleResourceGroup.location}
* administratorLogin: adminTerraform
* administratorPassword: QAZwsx123
* storageMb: 32768
* version: '12'
* skuName: GP_Standard_D2s_v3
* zone: '2'
* authentication:
* activeDirectoryAuthEnabled: true
* tenantId: ${current.tenantId}
* exampleFlexibleServerActiveDirectoryAdministrator:
* type: azure:postgresql:FlexibleServerActiveDirectoryAdministrator
* name: example
* properties:
* serverName: ${exampleFlexibleServer.name}
* resourceGroupName: ${exampleResourceGroup.name}
* tenantId: ${current.tenantId}
* objectId: ${example.objectId}
* principalName: ${example.displayName}
* principalType: ServicePrincipal
* variables:
* current:
* fn::invoke:
* Function: azure:core:getClientConfig
* Arguments: {}
* example:
* fn::invoke:
* Function: azuread:getServicePrincipal
* Arguments:
* objectId: ${current.objectId}
* ```
*
* ## Import
* A PostgreSQL Flexible Server Active Directory Administrator can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:postgresql/flexibleServerActiveDirectoryAdministrator:FlexibleServerActiveDirectoryAdministrator example /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/myresourcegroup/providers/Microsoft.DBforPostgreSQL/flexibleServers/myserver/administrators/objectId
* ```
* @property objectId The object ID of a user, service principal or security group in the Azure Active Directory tenant set as the Flexible Server Admin. Changing this forces a new resource to be created.
* @property principalName The name of Azure Active Directory principal. Changing this forces a new resource to be created.
* @property principalType The type of Azure Active Directory principal. Possible values are `Group`, `ServicePrincipal` and `User`. Changing this forces a new resource to be created.
* @property resourceGroupName The name of the resource group for the PostgreSQL Server. Changing this forces a new resource to be created.
* @property serverName The name of the PostgreSQL Flexible Server on which to set the administrator. Changing this forces a new resource to be created.
* @property tenantId The Azure Tenant ID. Changing this forces a new resource to be created.
*/
public data class FlexibleServerActiveDirectoryAdministratorArgs(
public val objectId: Output? = null,
public val principalName: Output? = null,
public val principalType: Output? = null,
public val resourceGroupName: Output? = null,
public val serverName: Output? = null,
public val tenantId: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.postgresql.FlexibleServerActiveDirectoryAdministratorArgs = com.pulumi.azure.postgresql.FlexibleServerActiveDirectoryAdministratorArgs.builder()
.objectId(objectId?.applyValue({ args0 -> args0 }))
.principalName(principalName?.applyValue({ args0 -> args0 }))
.principalType(principalType?.applyValue({ args0 -> args0 }))
.resourceGroupName(resourceGroupName?.applyValue({ args0 -> args0 }))
.serverName(serverName?.applyValue({ args0 -> args0 }))
.tenantId(tenantId?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [FlexibleServerActiveDirectoryAdministratorArgs].
*/
@PulumiTagMarker
public class FlexibleServerActiveDirectoryAdministratorArgsBuilder internal constructor() {
private var objectId: Output? = null
private var principalName: Output? = null
private var principalType: Output? = null
private var resourceGroupName: Output? = null
private var serverName: Output? = null
private var tenantId: Output? = null
/**
* @param value The object ID of a user, service principal or security group in the Azure Active Directory tenant set as the Flexible Server Admin. Changing this forces a new resource to be created.
*/
@JvmName("dnbcjsrvhdxvndng")
public suspend fun objectId(`value`: Output) {
this.objectId = value
}
/**
* @param value The name of Azure Active Directory principal. Changing this forces a new resource to be created.
*/
@JvmName("vrhvbfviddorkvxg")
public suspend fun principalName(`value`: Output) {
this.principalName = value
}
/**
* @param value The type of Azure Active Directory principal. Possible values are `Group`, `ServicePrincipal` and `User`. Changing this forces a new resource to be created.
*/
@JvmName("sxkuqwlhgjurogkh")
public suspend fun principalType(`value`: Output) {
this.principalType = value
}
/**
* @param value The name of the resource group for the PostgreSQL Server. Changing this forces a new resource to be created.
*/
@JvmName("cssqornunocdcpwf")
public suspend fun resourceGroupName(`value`: Output) {
this.resourceGroupName = value
}
/**
* @param value The name of the PostgreSQL Flexible Server on which to set the administrator. Changing this forces a new resource to be created.
*/
@JvmName("xnacmwhhbnvfkuvm")
public suspend fun serverName(`value`: Output) {
this.serverName = value
}
/**
* @param value The Azure Tenant ID. Changing this forces a new resource to be created.
*/
@JvmName("ymmceajoqntgkxbc")
public suspend fun tenantId(`value`: Output) {
this.tenantId = value
}
/**
* @param value The object ID of a user, service principal or security group in the Azure Active Directory tenant set as the Flexible Server Admin. Changing this forces a new resource to be created.
*/
@JvmName("qriqoffgjbhfnvfl")
public suspend fun objectId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.objectId = mapped
}
/**
* @param value The name of Azure Active Directory principal. Changing this forces a new resource to be created.
*/
@JvmName("trgvlsovvhggqyfv")
public suspend fun principalName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.principalName = mapped
}
/**
* @param value The type of Azure Active Directory principal. Possible values are `Group`, `ServicePrincipal` and `User`. Changing this forces a new resource to be created.
*/
@JvmName("hroimmeftawpikan")
public suspend fun principalType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.principalType = mapped
}
/**
* @param value The name of the resource group for the PostgreSQL Server. Changing this forces a new resource to be created.
*/
@JvmName("hrtkfjqxhsiylnqs")
public suspend fun resourceGroupName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resourceGroupName = mapped
}
/**
* @param value The name of the PostgreSQL Flexible Server on which to set the administrator. Changing this forces a new resource to be created.
*/
@JvmName("blcvosuhxpohuyio")
public suspend fun serverName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.serverName = mapped
}
/**
* @param value The Azure Tenant ID. Changing this forces a new resource to be created.
*/
@JvmName("lnafdytwpoqsfuuk")
public suspend fun tenantId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tenantId = mapped
}
internal fun build(): FlexibleServerActiveDirectoryAdministratorArgs =
FlexibleServerActiveDirectoryAdministratorArgs(
objectId = objectId,
principalName = principalName,
principalType = principalType,
resourceGroupName = resourceGroupName,
serverName = serverName,
tenantId = tenantId,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy