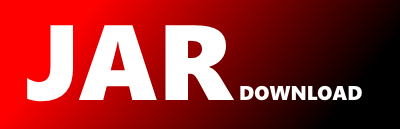
com.pulumi.azure.redhatopenshift.kotlin.inputs.ClusterClusterProfileArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.redhatopenshift.kotlin.inputs
import com.pulumi.azure.redhatopenshift.inputs.ClusterClusterProfileArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property domain The custom domain for the cluster. For more info, see [Prepare a custom domain for your cluster](https://docs.microsoft.com/azure/openshift/tutorial-create-cluster#prepare-a-custom-domain-for-your-cluster-optional). Changing this forces a new resource to be created.
* @property fipsEnabled Whether Federal Information Processing Standard (FIPS) validated cryptographic modules are used. Defaults to `false`. Changing this forces a new resource to be created.
* @property managedResourceGroupName The name of a Resource Group which will be created to host VMs of Azure Red Hat OpenShift Cluster. The value cannot contain uppercase characters. Defaults to `aro-{domain}`. Changing this forces a new resource to be created.
* @property pullSecret The Red Hat pull secret for the cluster. For more info, see [Get a Red Hat pull secret](https://learn.microsoft.com/azure/openshift/tutorial-create-cluster#get-a-red-hat-pull-secret-optional). Changing this forces a new resource to be created.
* @property resourceGroupId The resource group that the cluster profile is attached to.
* @property version The version of the OpenShift cluster. Available versions can be found with the Azure CLI command `az aro get-versions --location `. Changing this forces a new resource to be created.
*/
public data class ClusterClusterProfileArgs(
public val domain: Output,
public val fipsEnabled: Output? = null,
public val managedResourceGroupName: Output? = null,
public val pullSecret: Output? = null,
public val resourceGroupId: Output? = null,
public val version: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.redhatopenshift.inputs.ClusterClusterProfileArgs =
com.pulumi.azure.redhatopenshift.inputs.ClusterClusterProfileArgs.builder()
.domain(domain.applyValue({ args0 -> args0 }))
.fipsEnabled(fipsEnabled?.applyValue({ args0 -> args0 }))
.managedResourceGroupName(managedResourceGroupName?.applyValue({ args0 -> args0 }))
.pullSecret(pullSecret?.applyValue({ args0 -> args0 }))
.resourceGroupId(resourceGroupId?.applyValue({ args0 -> args0 }))
.version(version.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ClusterClusterProfileArgs].
*/
@PulumiTagMarker
public class ClusterClusterProfileArgsBuilder internal constructor() {
private var domain: Output? = null
private var fipsEnabled: Output? = null
private var managedResourceGroupName: Output? = null
private var pullSecret: Output? = null
private var resourceGroupId: Output? = null
private var version: Output? = null
/**
* @param value The custom domain for the cluster. For more info, see [Prepare a custom domain for your cluster](https://docs.microsoft.com/azure/openshift/tutorial-create-cluster#prepare-a-custom-domain-for-your-cluster-optional). Changing this forces a new resource to be created.
*/
@JvmName("uowfcjvafnbkaaws")
public suspend fun domain(`value`: Output) {
this.domain = value
}
/**
* @param value Whether Federal Information Processing Standard (FIPS) validated cryptographic modules are used. Defaults to `false`. Changing this forces a new resource to be created.
*/
@JvmName("vvdyedpcwkfkcxnm")
public suspend fun fipsEnabled(`value`: Output) {
this.fipsEnabled = value
}
/**
* @param value The name of a Resource Group which will be created to host VMs of Azure Red Hat OpenShift Cluster. The value cannot contain uppercase characters. Defaults to `aro-{domain}`. Changing this forces a new resource to be created.
*/
@JvmName("twyoxrkdairnktmj")
public suspend fun managedResourceGroupName(`value`: Output) {
this.managedResourceGroupName = value
}
/**
* @param value The Red Hat pull secret for the cluster. For more info, see [Get a Red Hat pull secret](https://learn.microsoft.com/azure/openshift/tutorial-create-cluster#get-a-red-hat-pull-secret-optional). Changing this forces a new resource to be created.
*/
@JvmName("vaovisdlnsnaqqrj")
public suspend fun pullSecret(`value`: Output) {
this.pullSecret = value
}
/**
* @param value The resource group that the cluster profile is attached to.
*/
@JvmName("jgladlgcbxoibwvx")
public suspend fun resourceGroupId(`value`: Output) {
this.resourceGroupId = value
}
/**
* @param value The version of the OpenShift cluster. Available versions can be found with the Azure CLI command `az aro get-versions --location `. Changing this forces a new resource to be created.
*/
@JvmName("trjewmonkogqsxsh")
public suspend fun version(`value`: Output) {
this.version = value
}
/**
* @param value The custom domain for the cluster. For more info, see [Prepare a custom domain for your cluster](https://docs.microsoft.com/azure/openshift/tutorial-create-cluster#prepare-a-custom-domain-for-your-cluster-optional). Changing this forces a new resource to be created.
*/
@JvmName("uhoptsssrvqrafyx")
public suspend fun domain(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.domain = mapped
}
/**
* @param value Whether Federal Information Processing Standard (FIPS) validated cryptographic modules are used. Defaults to `false`. Changing this forces a new resource to be created.
*/
@JvmName("glmnofycueptohiw")
public suspend fun fipsEnabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.fipsEnabled = mapped
}
/**
* @param value The name of a Resource Group which will be created to host VMs of Azure Red Hat OpenShift Cluster. The value cannot contain uppercase characters. Defaults to `aro-{domain}`. Changing this forces a new resource to be created.
*/
@JvmName("uiqwknaahjonlqqn")
public suspend fun managedResourceGroupName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.managedResourceGroupName = mapped
}
/**
* @param value The Red Hat pull secret for the cluster. For more info, see [Get a Red Hat pull secret](https://learn.microsoft.com/azure/openshift/tutorial-create-cluster#get-a-red-hat-pull-secret-optional). Changing this forces a new resource to be created.
*/
@JvmName("dwcbwevovadyarng")
public suspend fun pullSecret(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.pullSecret = mapped
}
/**
* @param value The resource group that the cluster profile is attached to.
*/
@JvmName("ybraptybxfqwaany")
public suspend fun resourceGroupId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resourceGroupId = mapped
}
/**
* @param value The version of the OpenShift cluster. Available versions can be found with the Azure CLI command `az aro get-versions --location `. Changing this forces a new resource to be created.
*/
@JvmName("npkphmdjpvqquixh")
public suspend fun version(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.version = mapped
}
internal fun build(): ClusterClusterProfileArgs = ClusterClusterProfileArgs(
domain = domain ?: throw PulumiNullFieldException("domain"),
fipsEnabled = fipsEnabled,
managedResourceGroupName = managedResourceGroupName,
pullSecret = pullSecret,
resourceGroupId = resourceGroupId,
version = version ?: throw PulumiNullFieldException("version"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy