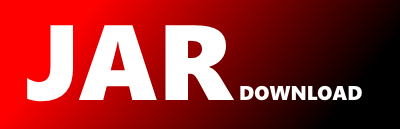
com.pulumi.azure.redhatopenshift.kotlin.inputs.ClusterNetworkProfileArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.redhatopenshift.kotlin.inputs
import com.pulumi.azure.redhatopenshift.inputs.ClusterNetworkProfileArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property outboundType The outbound (egress) routing method. Possible values are `Loadbalancer` and `UserDefinedRouting`. Defaults to `Loadbalancer`. Changing this forces a new resource to be created.
* @property podCidr The CIDR to use for pod IP addresses. Changing this forces a new resource to be created.
* @property preconfiguredNetworkSecurityGroupEnabled Whether a preconfigured network security group is being used on the subnets. Defaults to `false`. Changing this forces a new resource to be created.
* @property serviceCidr The network range used by the OpenShift service. Changing this forces a new resource to be created.
*/
public data class ClusterNetworkProfileArgs(
public val outboundType: Output? = null,
public val podCidr: Output,
public val preconfiguredNetworkSecurityGroupEnabled: Output? = null,
public val serviceCidr: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.redhatopenshift.inputs.ClusterNetworkProfileArgs =
com.pulumi.azure.redhatopenshift.inputs.ClusterNetworkProfileArgs.builder()
.outboundType(outboundType?.applyValue({ args0 -> args0 }))
.podCidr(podCidr.applyValue({ args0 -> args0 }))
.preconfiguredNetworkSecurityGroupEnabled(
preconfiguredNetworkSecurityGroupEnabled?.applyValue({ args0 ->
args0
}),
)
.serviceCidr(serviceCidr.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ClusterNetworkProfileArgs].
*/
@PulumiTagMarker
public class ClusterNetworkProfileArgsBuilder internal constructor() {
private var outboundType: Output? = null
private var podCidr: Output? = null
private var preconfiguredNetworkSecurityGroupEnabled: Output? = null
private var serviceCidr: Output? = null
/**
* @param value The outbound (egress) routing method. Possible values are `Loadbalancer` and `UserDefinedRouting`. Defaults to `Loadbalancer`. Changing this forces a new resource to be created.
*/
@JvmName("olkxdhahikrranht")
public suspend fun outboundType(`value`: Output) {
this.outboundType = value
}
/**
* @param value The CIDR to use for pod IP addresses. Changing this forces a new resource to be created.
*/
@JvmName("avmuxsyqdiglumkb")
public suspend fun podCidr(`value`: Output) {
this.podCidr = value
}
/**
* @param value Whether a preconfigured network security group is being used on the subnets. Defaults to `false`. Changing this forces a new resource to be created.
*/
@JvmName("jexfvwhigafkicpb")
public suspend fun preconfiguredNetworkSecurityGroupEnabled(`value`: Output) {
this.preconfiguredNetworkSecurityGroupEnabled = value
}
/**
* @param value The network range used by the OpenShift service. Changing this forces a new resource to be created.
*/
@JvmName("lyskeiirthurmhks")
public suspend fun serviceCidr(`value`: Output) {
this.serviceCidr = value
}
/**
* @param value The outbound (egress) routing method. Possible values are `Loadbalancer` and `UserDefinedRouting`. Defaults to `Loadbalancer`. Changing this forces a new resource to be created.
*/
@JvmName("lsclocaveyyxpsud")
public suspend fun outboundType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.outboundType = mapped
}
/**
* @param value The CIDR to use for pod IP addresses. Changing this forces a new resource to be created.
*/
@JvmName("kopwvgotmutfymtc")
public suspend fun podCidr(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.podCidr = mapped
}
/**
* @param value Whether a preconfigured network security group is being used on the subnets. Defaults to `false`. Changing this forces a new resource to be created.
*/
@JvmName("jhwvtidykreomhdg")
public suspend fun preconfiguredNetworkSecurityGroupEnabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.preconfiguredNetworkSecurityGroupEnabled = mapped
}
/**
* @param value The network range used by the OpenShift service. Changing this forces a new resource to be created.
*/
@JvmName("xptxbfemjbqeumwx")
public suspend fun serviceCidr(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.serviceCidr = mapped
}
internal fun build(): ClusterNetworkProfileArgs = ClusterNetworkProfileArgs(
outboundType = outboundType,
podCidr = podCidr ?: throw PulumiNullFieldException("podCidr"),
preconfiguredNetworkSecurityGroupEnabled = preconfiguredNetworkSecurityGroupEnabled,
serviceCidr = serviceCidr ?: throw PulumiNullFieldException("serviceCidr"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy