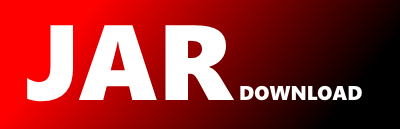
com.pulumi.azure.redis.kotlin.outputs.CacheRedisConfiguration.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.redis.kotlin.outputs
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.Int
import kotlin.String
import kotlin.Suppress
/**
*
* @property activeDirectoryAuthenticationEnabled Enable Microsoft Entra (AAD) authentication. Defaults to `false`.
* @property aofBackupEnabled Enable or disable AOF persistence for this Redis Cache. Defaults to `false`.
* > **NOTE:** `aof_backup_enabled` can only be set when SKU is `Premium`.
* @property aofStorageConnectionString0 First Storage Account connection string for AOF persistence.
* @property aofStorageConnectionString1 Second Storage Account connection string for AOF persistence.
* Example usage:
* @property authenticationEnabled
* @property dataPersistenceAuthenticationMethod Preferred auth method to communicate to storage account used for data persistence. Possible values are `SAS` and `ManagedIdentity`. Defaults to `SAS`.
* @property enableAuthentication If set to `false`, the Redis instance will be accessible without authentication. Defaults to `true`.
* > **NOTE:** `enable_authentication` can only be set to `false` if a `subnet_id` is specified; and only works if there aren't existing instances within the subnet with `enable_authentication` set to `true`.
* @property maxclients Returns the max number of connected clients at the same time.
* @property maxfragmentationmemoryReserved Value in megabytes reserved to accommodate for memory fragmentation. Defaults are shown below.
* @property maxmemoryDelta The max-memory delta for this Redis instance. Defaults are shown below.
* @property maxmemoryPolicy How Redis will select what to remove when `maxmemory` is reached. Defaults to `volatile-lru`.
* @property maxmemoryReserved Value in megabytes reserved for non-cache usage e.g. failover. Defaults are shown below.
* @property notifyKeyspaceEvents Keyspace notifications allows clients to subscribe to Pub/Sub channels in order to receive events affecting the Redis data set in some way. [Reference](https://redis.io/topics/notifications#configuration)
* @property rdbBackupEnabled Is Backup Enabled? Only supported on Premium SKUs. Defaults to `false`.
* > **NOTE:** If `rdb_backup_enabled` set to `true`, `rdb_storage_connection_string` must also be set.
* @property rdbBackupFrequency The Backup Frequency in Minutes. Only supported on Premium SKUs. Possible values are: `15`, `30`, `60`, `360`, `720` and `1440`.
* @property rdbBackupMaxSnapshotCount The maximum number of snapshots to create as a backup. Only supported for Premium SKUs.
* @property rdbStorageConnectionString The Connection String to the Storage Account. Only supported for Premium SKUs. In the format: `DefaultEndpointsProtocol=https;BlobEndpoint=${azurerm_storage_account.example.primary_blob_endpoint};AccountName=${azurerm_storage_account.example.name};AccountKey=${azurerm_storage_account.example.primary_access_key}`.
* > **NOTE:** There's a bug in the Redis API where the original storage connection string isn't being returned, which [is being tracked in this issue](https://github.com/Azure/azure-rest-api-specs/issues/3037). In the interim you can use [the `ignoreChanges` attribute to ignore changes to this field](https://www.pulumi.com/docs/intro/concepts/programming-model/#ignorechanges) e.g.:
* @property storageAccountSubscriptionId The ID of the Subscription containing the Storage Account.
*
* ```yaml
* resources:
* example:
* type: azure:redis:Cache
* properties:
* ignoreChanges:
* - ${redisConfiguration[0].rdbStorageConnectionString}
* ```
*
*/
public data class CacheRedisConfiguration(
public val activeDirectoryAuthenticationEnabled: Boolean? = null,
public val aofBackupEnabled: Boolean? = null,
public val aofStorageConnectionString0: String? = null,
public val aofStorageConnectionString1: String? = null,
public val authenticationEnabled: Boolean? = null,
public val dataPersistenceAuthenticationMethod: String? = null,
@Deprecated(
message = """
`enable_authentication` will be removed in favour of the property `authentication_enabled` in
version 4.0 of the AzureRM Provider.
""",
)
public val enableAuthentication: Boolean? = null,
public val maxclients: Int? = null,
public val maxfragmentationmemoryReserved: Int? = null,
public val maxmemoryDelta: Int? = null,
public val maxmemoryPolicy: String? = null,
public val maxmemoryReserved: Int? = null,
public val notifyKeyspaceEvents: String? = null,
public val rdbBackupEnabled: Boolean? = null,
public val rdbBackupFrequency: Int? = null,
public val rdbBackupMaxSnapshotCount: Int? = null,
public val rdbStorageConnectionString: String? = null,
public val storageAccountSubscriptionId: String? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.azure.redis.outputs.CacheRedisConfiguration): CacheRedisConfiguration = CacheRedisConfiguration(
activeDirectoryAuthenticationEnabled = javaType.activeDirectoryAuthenticationEnabled().map({ args0 ->
args0
}).orElse(null),
aofBackupEnabled = javaType.aofBackupEnabled().map({ args0 -> args0 }).orElse(null),
aofStorageConnectionString0 = javaType.aofStorageConnectionString0().map({ args0 ->
args0
}).orElse(null),
aofStorageConnectionString1 = javaType.aofStorageConnectionString1().map({ args0 ->
args0
}).orElse(null),
authenticationEnabled = javaType.authenticationEnabled().map({ args0 -> args0 }).orElse(null),
dataPersistenceAuthenticationMethod = javaType.dataPersistenceAuthenticationMethod().map({ args0 ->
args0
}).orElse(null),
enableAuthentication = javaType.enableAuthentication().map({ args0 -> args0 }).orElse(null),
maxclients = javaType.maxclients().map({ args0 -> args0 }).orElse(null),
maxfragmentationmemoryReserved = javaType.maxfragmentationmemoryReserved().map({ args0 ->
args0
}).orElse(null),
maxmemoryDelta = javaType.maxmemoryDelta().map({ args0 -> args0 }).orElse(null),
maxmemoryPolicy = javaType.maxmemoryPolicy().map({ args0 -> args0 }).orElse(null),
maxmemoryReserved = javaType.maxmemoryReserved().map({ args0 -> args0 }).orElse(null),
notifyKeyspaceEvents = javaType.notifyKeyspaceEvents().map({ args0 -> args0 }).orElse(null),
rdbBackupEnabled = javaType.rdbBackupEnabled().map({ args0 -> args0 }).orElse(null),
rdbBackupFrequency = javaType.rdbBackupFrequency().map({ args0 -> args0 }).orElse(null),
rdbBackupMaxSnapshotCount = javaType.rdbBackupMaxSnapshotCount().map({ args0 ->
args0
}).orElse(null),
rdbStorageConnectionString = javaType.rdbStorageConnectionString().map({ args0 ->
args0
}).orElse(null),
storageAccountSubscriptionId = javaType.storageAccountSubscriptionId().map({ args0 ->
args0
}).orElse(null),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy