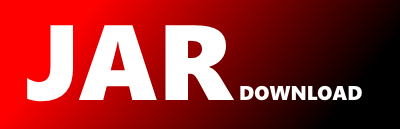
com.pulumi.azure.search.kotlin.SharedPrivateLinkServiceArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.search.kotlin
import com.pulumi.azure.search.SharedPrivateLinkServiceArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Manages the Shared Private Link Service for an Azure Search Service.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const test = new azure.core.ResourceGroup("test", {
* name: "example-resourceGroup",
* location: "east us",
* });
* const testService = new azure.search.Service("test", {
* name: "example-search",
* resourceGroupName: test.name,
* location: test.location,
* sku: "standard",
* });
* const testAccount = new azure.storage.Account("test", {
* name: "xiaxintestsaforsearchspl",
* resourceGroupName: test.name,
* location: test.location,
* accountTier: "Standard",
* accountReplicationType: "LRS",
* });
* const testSharedPrivateLinkService = new azure.search.SharedPrivateLinkService("test", {
* name: "example-spl",
* searchServiceId: testService.id,
* subresourceName: "blob",
* targetResourceId: testAccount.id,
* requestMessage: "please approve",
* });
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* test = azure.core.ResourceGroup("test",
* name="example-resourceGroup",
* location="east us")
* test_service = azure.search.Service("test",
* name="example-search",
* resource_group_name=test.name,
* location=test.location,
* sku="standard")
* test_account = azure.storage.Account("test",
* name="xiaxintestsaforsearchspl",
* resource_group_name=test.name,
* location=test.location,
* account_tier="Standard",
* account_replication_type="LRS")
* test_shared_private_link_service = azure.search.SharedPrivateLinkService("test",
* name="example-spl",
* search_service_id=test_service.id,
* subresource_name="blob",
* target_resource_id=test_account.id,
* request_message="please approve")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var test = new Azure.Core.ResourceGroup("test", new()
* {
* Name = "example-resourceGroup",
* Location = "east us",
* });
* var testService = new Azure.Search.Service("test", new()
* {
* Name = "example-search",
* ResourceGroupName = test.Name,
* Location = test.Location,
* Sku = "standard",
* });
* var testAccount = new Azure.Storage.Account("test", new()
* {
* Name = "xiaxintestsaforsearchspl",
* ResourceGroupName = test.Name,
* Location = test.Location,
* AccountTier = "Standard",
* AccountReplicationType = "LRS",
* });
* var testSharedPrivateLinkService = new Azure.Search.SharedPrivateLinkService("test", new()
* {
* Name = "example-spl",
* SearchServiceId = testService.Id,
* SubresourceName = "blob",
* TargetResourceId = testAccount.Id,
* RequestMessage = "please approve",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/core"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/search"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/storage"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* test, err := core.NewResourceGroup(ctx, "test", &core.ResourceGroupArgs{
* Name: pulumi.String("example-resourceGroup"),
* Location: pulumi.String("east us"),
* })
* if err != nil {
* return err
* }
* testService, err := search.NewService(ctx, "test", &search.ServiceArgs{
* Name: pulumi.String("example-search"),
* ResourceGroupName: test.Name,
* Location: test.Location,
* Sku: pulumi.String("standard"),
* })
* if err != nil {
* return err
* }
* testAccount, err := storage.NewAccount(ctx, "test", &storage.AccountArgs{
* Name: pulumi.String("xiaxintestsaforsearchspl"),
* ResourceGroupName: test.Name,
* Location: test.Location,
* AccountTier: pulumi.String("Standard"),
* AccountReplicationType: pulumi.String("LRS"),
* })
* if err != nil {
* return err
* }
* _, err = search.NewSharedPrivateLinkService(ctx, "test", &search.SharedPrivateLinkServiceArgs{
* Name: pulumi.String("example-spl"),
* SearchServiceId: testService.ID(),
* SubresourceName: pulumi.String("blob"),
* TargetResourceId: testAccount.ID(),
* RequestMessage: pulumi.String("please approve"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.search.Service;
* import com.pulumi.azure.search.ServiceArgs;
* import com.pulumi.azure.storage.Account;
* import com.pulumi.azure.storage.AccountArgs;
* import com.pulumi.azure.search.SharedPrivateLinkService;
* import com.pulumi.azure.search.SharedPrivateLinkServiceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var test = new ResourceGroup("test", ResourceGroupArgs.builder()
* .name("example-resourceGroup")
* .location("east us")
* .build());
* var testService = new Service("testService", ServiceArgs.builder()
* .name("example-search")
* .resourceGroupName(test.name())
* .location(test.location())
* .sku("standard")
* .build());
* var testAccount = new Account("testAccount", AccountArgs.builder()
* .name("xiaxintestsaforsearchspl")
* .resourceGroupName(test.name())
* .location(test.location())
* .accountTier("Standard")
* .accountReplicationType("LRS")
* .build());
* var testSharedPrivateLinkService = new SharedPrivateLinkService("testSharedPrivateLinkService", SharedPrivateLinkServiceArgs.builder()
* .name("example-spl")
* .searchServiceId(testService.id())
* .subresourceName("blob")
* .targetResourceId(testAccount.id())
* .requestMessage("please approve")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* test:
* type: azure:core:ResourceGroup
* properties:
* name: example-resourceGroup
* location: east us
* testService:
* type: azure:search:Service
* name: test
* properties:
* name: example-search
* resourceGroupName: ${test.name}
* location: ${test.location}
* sku: standard
* testAccount:
* type: azure:storage:Account
* name: test
* properties:
* name: xiaxintestsaforsearchspl
* resourceGroupName: ${test.name}
* location: ${test.location}
* accountTier: Standard
* accountReplicationType: LRS
* testSharedPrivateLinkService:
* type: azure:search:SharedPrivateLinkService
* name: test
* properties:
* name: example-spl
* searchServiceId: ${testService.id}
* subresourceName: blob
* targetResourceId: ${testAccount.id}
* requestMessage: please approve
* ```
*
* ## Import
* Azure Search Shared Private Link Resource can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:search/sharedPrivateLinkService:SharedPrivateLinkService example /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/group1/providers/Microsoft.Search/searchServices/service1/sharedPrivateLinkResources/resource1
* ```
* @property name Specify the name of the Azure Search Shared Private Link Resource. Changing this forces a new resource to be created.
* @property requestMessage Specify the request message for requesting approval of the Shared Private Link Enabled Remote Resource.
* @property searchServiceId Specify the id of the Azure Search Service. Changing this forces a new resource to be created.
* @property subresourceName Specify the sub resource name which the Azure Search Private Endpoint is able to connect to. Changing this forces a new resource to be created.
* @property targetResourceId Specify the ID of the Shared Private Link Enabled Remote Resource which this Azure Search Private Endpoint should be connected to. Changing this forces a new resource to be created.
* > **NOTE:** The sub resource name should match with the type of the target resource id that's being specified.
*/
public data class SharedPrivateLinkServiceArgs(
public val name: Output? = null,
public val requestMessage: Output? = null,
public val searchServiceId: Output? = null,
public val subresourceName: Output? = null,
public val targetResourceId: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.search.SharedPrivateLinkServiceArgs =
com.pulumi.azure.search.SharedPrivateLinkServiceArgs.builder()
.name(name?.applyValue({ args0 -> args0 }))
.requestMessage(requestMessage?.applyValue({ args0 -> args0 }))
.searchServiceId(searchServiceId?.applyValue({ args0 -> args0 }))
.subresourceName(subresourceName?.applyValue({ args0 -> args0 }))
.targetResourceId(targetResourceId?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [SharedPrivateLinkServiceArgs].
*/
@PulumiTagMarker
public class SharedPrivateLinkServiceArgsBuilder internal constructor() {
private var name: Output? = null
private var requestMessage: Output? = null
private var searchServiceId: Output? = null
private var subresourceName: Output? = null
private var targetResourceId: Output? = null
/**
* @param value Specify the name of the Azure Search Shared Private Link Resource. Changing this forces a new resource to be created.
*/
@JvmName("hbnvvkopnarwdhfr")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value Specify the request message for requesting approval of the Shared Private Link Enabled Remote Resource.
*/
@JvmName("qhlysjsyojvqslcv")
public suspend fun requestMessage(`value`: Output) {
this.requestMessage = value
}
/**
* @param value Specify the id of the Azure Search Service. Changing this forces a new resource to be created.
*/
@JvmName("cckpnvisshpwpsnd")
public suspend fun searchServiceId(`value`: Output) {
this.searchServiceId = value
}
/**
* @param value Specify the sub resource name which the Azure Search Private Endpoint is able to connect to. Changing this forces a new resource to be created.
*/
@JvmName("bhgfyhrfrcjkackf")
public suspend fun subresourceName(`value`: Output) {
this.subresourceName = value
}
/**
* @param value Specify the ID of the Shared Private Link Enabled Remote Resource which this Azure Search Private Endpoint should be connected to. Changing this forces a new resource to be created.
* > **NOTE:** The sub resource name should match with the type of the target resource id that's being specified.
*/
@JvmName("eplwsvycntjrkxxy")
public suspend fun targetResourceId(`value`: Output) {
this.targetResourceId = value
}
/**
* @param value Specify the name of the Azure Search Shared Private Link Resource. Changing this forces a new resource to be created.
*/
@JvmName("borggmvmfrvlewfu")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value Specify the request message for requesting approval of the Shared Private Link Enabled Remote Resource.
*/
@JvmName("tbflwblaalejfcwj")
public suspend fun requestMessage(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.requestMessage = mapped
}
/**
* @param value Specify the id of the Azure Search Service. Changing this forces a new resource to be created.
*/
@JvmName("wdrosulprjocstdh")
public suspend fun searchServiceId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.searchServiceId = mapped
}
/**
* @param value Specify the sub resource name which the Azure Search Private Endpoint is able to connect to. Changing this forces a new resource to be created.
*/
@JvmName("hbifxqjbomfpukie")
public suspend fun subresourceName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.subresourceName = mapped
}
/**
* @param value Specify the ID of the Shared Private Link Enabled Remote Resource which this Azure Search Private Endpoint should be connected to. Changing this forces a new resource to be created.
* > **NOTE:** The sub resource name should match with the type of the target resource id that's being specified.
*/
@JvmName("bibfvallcxrwugrs")
public suspend fun targetResourceId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.targetResourceId = mapped
}
internal fun build(): SharedPrivateLinkServiceArgs = SharedPrivateLinkServiceArgs(
name = name,
requestMessage = requestMessage,
searchServiceId = searchServiceId,
subresourceName = subresourceName,
targetResourceId = targetResourceId,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy