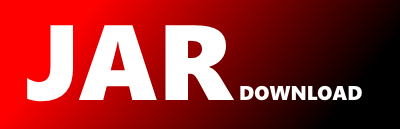
com.pulumi.azure.sentinel.kotlin.inputs.AlertRuleScheduledIncidentConfigurationGroupingArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.sentinel.kotlin.inputs
import com.pulumi.azure.sentinel.inputs.AlertRuleScheduledIncidentConfigurationGroupingArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property enabled Enable grouping incidents created from alerts triggered by this Sentinel Scheduled Alert Rule. Defaults to `true`.
* @property entityMatchingMethod The method used to group incidents. Possible values are `AnyAlert`, `Selected` and `AllEntities`. Defaults to `AnyAlert`.
* @property groupByAlertDetails
* @property groupByCustomDetails
* @property groupByEntities
* @property lookbackDuration Limit the group to alerts created within the lookback duration (in ISO 8601 duration format). Defaults to `PT5M`.
* @property reopenClosedIncidents Whether to re-open closed matching incidents? Defaults to `false`.
*/
public data class AlertRuleScheduledIncidentConfigurationGroupingArgs(
public val enabled: Output? = null,
public val entityMatchingMethod: Output? = null,
@Deprecated(
message = """
The `group_by_alert_details` property has been superseded by the `by_alert_details` property and
will be removed in v4.0 of the AzureRM Provider
""",
)
public val groupByAlertDetails: Output>? = null,
@Deprecated(
message = """
The `group_by_custom_details` property has been superseded by the `by_custom_details` property and
will be removed in v4.0 of the AzureRM Provider
""",
)
public val groupByCustomDetails: Output>? = null,
@Deprecated(
message = """
The `group_by_entities` property has been superseded by the `by_entities` property and will be
removed in v4.0 of the AzureRM Provider
""",
)
public val groupByEntities: Output>? = null,
public val lookbackDuration: Output? = null,
public val reopenClosedIncidents: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.azure.sentinel.inputs.AlertRuleScheduledIncidentConfigurationGroupingArgs =
com.pulumi.azure.sentinel.inputs.AlertRuleScheduledIncidentConfigurationGroupingArgs.builder()
.enabled(enabled?.applyValue({ args0 -> args0 }))
.entityMatchingMethod(entityMatchingMethod?.applyValue({ args0 -> args0 }))
.groupByAlertDetails(groupByAlertDetails?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.groupByCustomDetails(groupByCustomDetails?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.groupByEntities(groupByEntities?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.lookbackDuration(lookbackDuration?.applyValue({ args0 -> args0 }))
.reopenClosedIncidents(reopenClosedIncidents?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [AlertRuleScheduledIncidentConfigurationGroupingArgs].
*/
@PulumiTagMarker
public class AlertRuleScheduledIncidentConfigurationGroupingArgsBuilder internal constructor() {
private var enabled: Output? = null
private var entityMatchingMethod: Output? = null
private var groupByAlertDetails: Output>? = null
private var groupByCustomDetails: Output>? = null
private var groupByEntities: Output>? = null
private var lookbackDuration: Output? = null
private var reopenClosedIncidents: Output? = null
/**
* @param value Enable grouping incidents created from alerts triggered by this Sentinel Scheduled Alert Rule. Defaults to `true`.
*/
@JvmName("mqkgrgotlleiqpce")
public suspend fun enabled(`value`: Output) {
this.enabled = value
}
/**
* @param value The method used to group incidents. Possible values are `AnyAlert`, `Selected` and `AllEntities`. Defaults to `AnyAlert`.
*/
@JvmName("baauuyinomrnybvg")
public suspend fun entityMatchingMethod(`value`: Output) {
this.entityMatchingMethod = value
}
/**
* @param value
*/
@Deprecated(
message = """
The `group_by_alert_details` property has been superseded by the `by_alert_details` property and
will be removed in v4.0 of the AzureRM Provider
""",
)
@JvmName("ohyugwxhcsmosnyr")
public suspend fun groupByAlertDetails(`value`: Output>) {
this.groupByAlertDetails = value
}
@JvmName("neggrlmxfthjhknr")
public suspend fun groupByAlertDetails(vararg values: Output) {
this.groupByAlertDetails = Output.all(values.asList())
}
/**
* @param values
*/
@Deprecated(
message = """
The `group_by_alert_details` property has been superseded by the `by_alert_details` property and
will be removed in v4.0 of the AzureRM Provider
""",
)
@JvmName("mjsawjhbbaageoai")
public suspend fun groupByAlertDetails(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy