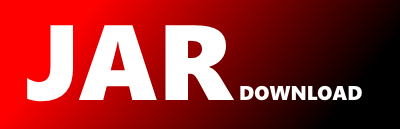
com.pulumi.azure.servicebus.kotlin.ServicebusFunctions.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.servicebus.kotlin
import com.pulumi.azure.servicebus.ServicebusFunctions.getNamespaceAuthorizationRulePlain
import com.pulumi.azure.servicebus.ServicebusFunctions.getNamespaceDisasterRecoveryConfigPlain
import com.pulumi.azure.servicebus.ServicebusFunctions.getNamespacePlain
import com.pulumi.azure.servicebus.ServicebusFunctions.getQueueAuthorizationRulePlain
import com.pulumi.azure.servicebus.ServicebusFunctions.getQueuePlain
import com.pulumi.azure.servicebus.ServicebusFunctions.getSubscriptionPlain
import com.pulumi.azure.servicebus.ServicebusFunctions.getTopicAuthorizationRulePlain
import com.pulumi.azure.servicebus.ServicebusFunctions.getTopicPlain
import com.pulumi.azure.servicebus.kotlin.inputs.GetNamespaceAuthorizationRulePlainArgs
import com.pulumi.azure.servicebus.kotlin.inputs.GetNamespaceAuthorizationRulePlainArgsBuilder
import com.pulumi.azure.servicebus.kotlin.inputs.GetNamespaceDisasterRecoveryConfigPlainArgs
import com.pulumi.azure.servicebus.kotlin.inputs.GetNamespaceDisasterRecoveryConfigPlainArgsBuilder
import com.pulumi.azure.servicebus.kotlin.inputs.GetNamespacePlainArgs
import com.pulumi.azure.servicebus.kotlin.inputs.GetNamespacePlainArgsBuilder
import com.pulumi.azure.servicebus.kotlin.inputs.GetQueueAuthorizationRulePlainArgs
import com.pulumi.azure.servicebus.kotlin.inputs.GetQueueAuthorizationRulePlainArgsBuilder
import com.pulumi.azure.servicebus.kotlin.inputs.GetQueuePlainArgs
import com.pulumi.azure.servicebus.kotlin.inputs.GetQueuePlainArgsBuilder
import com.pulumi.azure.servicebus.kotlin.inputs.GetSubscriptionPlainArgs
import com.pulumi.azure.servicebus.kotlin.inputs.GetSubscriptionPlainArgsBuilder
import com.pulumi.azure.servicebus.kotlin.inputs.GetTopicAuthorizationRulePlainArgs
import com.pulumi.azure.servicebus.kotlin.inputs.GetTopicAuthorizationRulePlainArgsBuilder
import com.pulumi.azure.servicebus.kotlin.inputs.GetTopicPlainArgs
import com.pulumi.azure.servicebus.kotlin.inputs.GetTopicPlainArgsBuilder
import com.pulumi.azure.servicebus.kotlin.outputs.GetNamespaceAuthorizationRuleResult
import com.pulumi.azure.servicebus.kotlin.outputs.GetNamespaceDisasterRecoveryConfigResult
import com.pulumi.azure.servicebus.kotlin.outputs.GetNamespaceResult
import com.pulumi.azure.servicebus.kotlin.outputs.GetQueueAuthorizationRuleResult
import com.pulumi.azure.servicebus.kotlin.outputs.GetQueueResult
import com.pulumi.azure.servicebus.kotlin.outputs.GetSubscriptionResult
import com.pulumi.azure.servicebus.kotlin.outputs.GetTopicAuthorizationRuleResult
import com.pulumi.azure.servicebus.kotlin.outputs.GetTopicResult
import kotlinx.coroutines.future.await
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.azure.servicebus.kotlin.outputs.GetNamespaceAuthorizationRuleResult.Companion.toKotlin as getNamespaceAuthorizationRuleResultToKotlin
import com.pulumi.azure.servicebus.kotlin.outputs.GetNamespaceDisasterRecoveryConfigResult.Companion.toKotlin as getNamespaceDisasterRecoveryConfigResultToKotlin
import com.pulumi.azure.servicebus.kotlin.outputs.GetNamespaceResult.Companion.toKotlin as getNamespaceResultToKotlin
import com.pulumi.azure.servicebus.kotlin.outputs.GetQueueAuthorizationRuleResult.Companion.toKotlin as getQueueAuthorizationRuleResultToKotlin
import com.pulumi.azure.servicebus.kotlin.outputs.GetQueueResult.Companion.toKotlin as getQueueResultToKotlin
import com.pulumi.azure.servicebus.kotlin.outputs.GetSubscriptionResult.Companion.toKotlin as getSubscriptionResultToKotlin
import com.pulumi.azure.servicebus.kotlin.outputs.GetTopicAuthorizationRuleResult.Companion.toKotlin as getTopicAuthorizationRuleResultToKotlin
import com.pulumi.azure.servicebus.kotlin.outputs.GetTopicResult.Companion.toKotlin as getTopicResultToKotlin
public object ServicebusFunctions {
/**
* Use this data source to access information about an existing ServiceBus Namespace.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = azure.servicebus.getNamespace({
* name: "examplenamespace",
* resourceGroupName: "example-resources",
* });
* export const location = example.then(example => example.location);
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.servicebus.get_namespace(name="examplenamespace",
* resource_group_name="example-resources")
* pulumi.export("location", example.location)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = Azure.ServiceBus.GetNamespace.Invoke(new()
* {
* Name = "examplenamespace",
* ResourceGroupName = "example-resources",
* });
* return new Dictionary
* {
* ["location"] = example.Apply(getNamespaceResult => getNamespaceResult.Location),
* };
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/servicebus"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := servicebus.LookupNamespace(ctx, &servicebus.LookupNamespaceArgs{
* Name: "examplenamespace",
* ResourceGroupName: "example-resources",
* }, nil)
* if err != nil {
* return err
* }
* ctx.Export("location", example.Location)
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.servicebus.ServicebusFunctions;
* import com.pulumi.azure.servicebus.inputs.GetNamespaceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var example = ServicebusFunctions.getNamespace(GetNamespaceArgs.builder()
* .name("examplenamespace")
* .resourceGroupName("example-resources")
* .build());
* ctx.export("location", example.applyValue(getNamespaceResult -> getNamespaceResult.location()));
* }
* }
* ```
* ```yaml
* variables:
* example:
* fn::invoke:
* Function: azure:servicebus:getNamespace
* Arguments:
* name: examplenamespace
* resourceGroupName: example-resources
* outputs:
* location: ${example.location}
* ```
*
* @param argument A collection of arguments for invoking getNamespace.
* @return A collection of values returned by getNamespace.
*/
public suspend fun getNamespace(argument: GetNamespacePlainArgs): GetNamespaceResult =
getNamespaceResultToKotlin(getNamespacePlain(argument.toJava()).await())
/**
* @see [getNamespace].
* @param name Specifies the name of the ServiceBus Namespace.
* @param resourceGroupName Specifies the name of the Resource Group where the ServiceBus Namespace exists.
* @return A collection of values returned by getNamespace.
*/
public suspend fun getNamespace(name: String, resourceGroupName: String): GetNamespaceResult {
val argument = GetNamespacePlainArgs(
name = name,
resourceGroupName = resourceGroupName,
)
return getNamespaceResultToKotlin(getNamespacePlain(argument.toJava()).await())
}
/**
* @see [getNamespace].
* @param argument Builder for [com.pulumi.azure.servicebus.kotlin.inputs.GetNamespacePlainArgs].
* @return A collection of values returned by getNamespace.
*/
public suspend fun getNamespace(argument: suspend GetNamespacePlainArgsBuilder.() -> Unit): GetNamespaceResult {
val builder = GetNamespacePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getNamespaceResultToKotlin(getNamespacePlain(builtArgument.toJava()).await())
}
/**
* Use this data source to access information about an existing ServiceBus Namespace Authorization Rule.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = azure.servicebus.getNamespaceAuthorizationRule({
* name: "examplerule",
* namespaceId: "examplenamespace",
* });
* export const ruleId = example.then(example => example.id);
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.servicebus.get_namespace_authorization_rule(name="examplerule",
* namespace_id="examplenamespace")
* pulumi.export("ruleId", example.id)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = Azure.ServiceBus.GetNamespaceAuthorizationRule.Invoke(new()
* {
* Name = "examplerule",
* NamespaceId = "examplenamespace",
* });
* return new Dictionary
* {
* ["ruleId"] = example.Apply(getNamespaceAuthorizationRuleResult => getNamespaceAuthorizationRuleResult.Id),
* };
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/servicebus"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := servicebus.LookupNamespaceAuthorizationRule(ctx, &servicebus.LookupNamespaceAuthorizationRuleArgs{
* Name: "examplerule",
* NamespaceId: pulumi.StringRef("examplenamespace"),
* }, nil)
* if err != nil {
* return err
* }
* ctx.Export("ruleId", example.Id)
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.servicebus.ServicebusFunctions;
* import com.pulumi.azure.servicebus.inputs.GetNamespaceAuthorizationRuleArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var example = ServicebusFunctions.getNamespaceAuthorizationRule(GetNamespaceAuthorizationRuleArgs.builder()
* .name("examplerule")
* .namespaceId("examplenamespace")
* .build());
* ctx.export("ruleId", example.applyValue(getNamespaceAuthorizationRuleResult -> getNamespaceAuthorizationRuleResult.id()));
* }
* }
* ```
* ```yaml
* variables:
* example:
* fn::invoke:
* Function: azure:servicebus:getNamespaceAuthorizationRule
* Arguments:
* name: examplerule
* namespaceId: examplenamespace
* outputs:
* ruleId: ${example.id}
* ```
*
* @param argument A collection of arguments for invoking getNamespaceAuthorizationRule.
* @return A collection of values returned by getNamespaceAuthorizationRule.
*/
public suspend fun getNamespaceAuthorizationRule(argument: GetNamespaceAuthorizationRulePlainArgs): GetNamespaceAuthorizationRuleResult =
getNamespaceAuthorizationRuleResultToKotlin(getNamespaceAuthorizationRulePlain(argument.toJava()).await())
/**
* @see [getNamespaceAuthorizationRule].
* @param name Specifies the name of the ServiceBus Namespace Authorization Rule.
* @param namespaceId Specifies the ID of the ServiceBus Namespace where the Service Bus Namespace Authorization Rule exists.
* @param namespaceName Specifies the name of the ServiceBus Namespace.
* @param resourceGroupName Specifies the name of the Resource Group where the ServiceBus Namespace exists.
* > **Note:** `namespace_name` and `resource_group_name` has been deprecated and will be removed in version 4.0 of the provider in favour of `namespace_id`.
* @return A collection of values returned by getNamespaceAuthorizationRule.
*/
public suspend fun getNamespaceAuthorizationRule(
name: String,
namespaceId: String? = null,
namespaceName: String? = null,
resourceGroupName: String? = null,
): GetNamespaceAuthorizationRuleResult {
val argument = GetNamespaceAuthorizationRulePlainArgs(
name = name,
namespaceId = namespaceId,
namespaceName = namespaceName,
resourceGroupName = resourceGroupName,
)
return getNamespaceAuthorizationRuleResultToKotlin(getNamespaceAuthorizationRulePlain(argument.toJava()).await())
}
/**
* @see [getNamespaceAuthorizationRule].
* @param argument Builder for [com.pulumi.azure.servicebus.kotlin.inputs.GetNamespaceAuthorizationRulePlainArgs].
* @return A collection of values returned by getNamespaceAuthorizationRule.
*/
public suspend fun getNamespaceAuthorizationRule(argument: suspend GetNamespaceAuthorizationRulePlainArgsBuilder.() -> Unit): GetNamespaceAuthorizationRuleResult {
val builder = GetNamespaceAuthorizationRulePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getNamespaceAuthorizationRuleResultToKotlin(getNamespaceAuthorizationRulePlain(builtArgument.toJava()).await())
}
/**
*
* @param argument A collection of arguments for invoking getNamespaceDisasterRecoveryConfig.
* @return A collection of values returned by getNamespaceDisasterRecoveryConfig.
*/
public suspend fun getNamespaceDisasterRecoveryConfig(argument: GetNamespaceDisasterRecoveryConfigPlainArgs): GetNamespaceDisasterRecoveryConfigResult =
getNamespaceDisasterRecoveryConfigResultToKotlin(getNamespaceDisasterRecoveryConfigPlain(argument.toJava()).await())
/**
* @see [getNamespaceDisasterRecoveryConfig].
* @param aliasAuthorizationRuleId
* @param name
* @param namespaceId
* @param namespaceName
* @param resourceGroupName
* @return A collection of values returned by getNamespaceDisasterRecoveryConfig.
*/
public suspend fun getNamespaceDisasterRecoveryConfig(
aliasAuthorizationRuleId: String? = null,
name: String,
namespaceId: String? = null,
namespaceName: String? = null,
resourceGroupName: String? = null,
): GetNamespaceDisasterRecoveryConfigResult {
val argument = GetNamespaceDisasterRecoveryConfigPlainArgs(
aliasAuthorizationRuleId = aliasAuthorizationRuleId,
name = name,
namespaceId = namespaceId,
namespaceName = namespaceName,
resourceGroupName = resourceGroupName,
)
return getNamespaceDisasterRecoveryConfigResultToKotlin(getNamespaceDisasterRecoveryConfigPlain(argument.toJava()).await())
}
/**
* @see [getNamespaceDisasterRecoveryConfig].
* @param argument Builder for [com.pulumi.azure.servicebus.kotlin.inputs.GetNamespaceDisasterRecoveryConfigPlainArgs].
* @return A collection of values returned by getNamespaceDisasterRecoveryConfig.
*/
public suspend fun getNamespaceDisasterRecoveryConfig(argument: suspend GetNamespaceDisasterRecoveryConfigPlainArgsBuilder.() -> Unit): GetNamespaceDisasterRecoveryConfigResult {
val builder = GetNamespaceDisasterRecoveryConfigPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getNamespaceDisasterRecoveryConfigResultToKotlin(getNamespaceDisasterRecoveryConfigPlain(builtArgument.toJava()).await())
}
/**
* Use this data source to access information about an existing Service Bus Queue.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = azure.servicebus.getQueue({
* name: "existing",
* namespaceId: "existing",
* });
* export const id = example.then(example => example.id);
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.servicebus.get_queue(name="existing",
* namespace_id="existing")
* pulumi.export("id", example.id)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = Azure.ServiceBus.GetQueue.Invoke(new()
* {
* Name = "existing",
* NamespaceId = "existing",
* });
* return new Dictionary
* {
* ["id"] = example.Apply(getQueueResult => getQueueResult.Id),
* };
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/servicebus"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := servicebus.LookupQueue(ctx, &servicebus.LookupQueueArgs{
* Name: "existing",
* NamespaceId: pulumi.StringRef("existing"),
* }, nil)
* if err != nil {
* return err
* }
* ctx.Export("id", example.Id)
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.servicebus.ServicebusFunctions;
* import com.pulumi.azure.servicebus.inputs.GetQueueArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var example = ServicebusFunctions.getQueue(GetQueueArgs.builder()
* .name("existing")
* .namespaceId("existing")
* .build());
* ctx.export("id", example.applyValue(getQueueResult -> getQueueResult.id()));
* }
* }
* ```
* ```yaml
* variables:
* example:
* fn::invoke:
* Function: azure:servicebus:getQueue
* Arguments:
* name: existing
* namespaceId: existing
* outputs:
* id: ${example.id}
* ```
*
* @param argument A collection of arguments for invoking getQueue.
* @return A collection of values returned by getQueue.
*/
public suspend fun getQueue(argument: GetQueuePlainArgs): GetQueueResult =
getQueueResultToKotlin(getQueuePlain(argument.toJava()).await())
/**
* @see [getQueue].
* @param name The name of this Service Bus Queue.
* @param namespaceId The ID of the ServiceBus Namespace where the Service Bus Queue exists.
* @param namespaceName The name of the ServiceBus Namespace.
* @param resourceGroupName The name of the Resource Group where the Service Bus Queue exists.
* > **Note:** `namespace_name` and `resource_group_name` has been deprecated and will be removed in version 4.0 of the provider in favour of `namespace_id`.
* @return A collection of values returned by getQueue.
*/
public suspend fun getQueue(
name: String,
namespaceId: String? = null,
namespaceName: String? = null,
resourceGroupName: String? = null,
): GetQueueResult {
val argument = GetQueuePlainArgs(
name = name,
namespaceId = namespaceId,
namespaceName = namespaceName,
resourceGroupName = resourceGroupName,
)
return getQueueResultToKotlin(getQueuePlain(argument.toJava()).await())
}
/**
* @see [getQueue].
* @param argument Builder for [com.pulumi.azure.servicebus.kotlin.inputs.GetQueuePlainArgs].
* @return A collection of values returned by getQueue.
*/
public suspend fun getQueue(argument: suspend GetQueuePlainArgsBuilder.() -> Unit): GetQueueResult {
val builder = GetQueuePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getQueueResultToKotlin(getQueuePlain(builtArgument.toJava()).await())
}
/**
* Use this data source to access information about an existing ServiceBus Queue Authorisation Rule within a ServiceBus Queue.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = azure.servicebus.getQueueAuthorizationRule({
* name: "example-tfex_name",
* resourceGroupName: "example-resources",
* queueName: "example-servicebus_queue",
* namespaceName: "example-namespace",
* });
* export const id = example.then(example => example.id);
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.servicebus.get_queue_authorization_rule(name="example-tfex_name",
* resource_group_name="example-resources",
* queue_name="example-servicebus_queue",
* namespace_name="example-namespace")
* pulumi.export("id", example.id)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = Azure.ServiceBus.GetQueueAuthorizationRule.Invoke(new()
* {
* Name = "example-tfex_name",
* ResourceGroupName = "example-resources",
* QueueName = "example-servicebus_queue",
* NamespaceName = "example-namespace",
* });
* return new Dictionary
* {
* ["id"] = example.Apply(getQueueAuthorizationRuleResult => getQueueAuthorizationRuleResult.Id),
* };
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/servicebus"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := servicebus.LookupQueueAuthorizationRule(ctx, &servicebus.LookupQueueAuthorizationRuleArgs{
* Name: "example-tfex_name",
* ResourceGroupName: pulumi.StringRef("example-resources"),
* QueueName: pulumi.StringRef("example-servicebus_queue"),
* NamespaceName: pulumi.StringRef("example-namespace"),
* }, nil)
* if err != nil {
* return err
* }
* ctx.Export("id", example.Id)
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.servicebus.ServicebusFunctions;
* import com.pulumi.azure.servicebus.inputs.GetQueueAuthorizationRuleArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var example = ServicebusFunctions.getQueueAuthorizationRule(GetQueueAuthorizationRuleArgs.builder()
* .name("example-tfex_name")
* .resourceGroupName("example-resources")
* .queueName("example-servicebus_queue")
* .namespaceName("example-namespace")
* .build());
* ctx.export("id", example.applyValue(getQueueAuthorizationRuleResult -> getQueueAuthorizationRuleResult.id()));
* }
* }
* ```
* ```yaml
* variables:
* example:
* fn::invoke:
* Function: azure:servicebus:getQueueAuthorizationRule
* Arguments:
* name: example-tfex_name
* resourceGroupName: example-resources
* queueName: example-servicebus_queue
* namespaceName: example-namespace
* outputs:
* id: ${example.id}
* ```
*
* @param argument A collection of arguments for invoking getQueueAuthorizationRule.
* @return A collection of values returned by getQueueAuthorizationRule.
*/
public suspend fun getQueueAuthorizationRule(argument: GetQueueAuthorizationRulePlainArgs): GetQueueAuthorizationRuleResult =
getQueueAuthorizationRuleResultToKotlin(getQueueAuthorizationRulePlain(argument.toJava()).await())
/**
* @see [getQueueAuthorizationRule].
* @param name The name of this ServiceBus Queue Authorisation Rule.
* @param namespaceName The name of the ServiceBus Namespace.
* @param queueId
* @param queueName The name of the ServiceBus Queue.
* @param resourceGroupName The name of the Resource Group where the ServiceBus Queue Authorisation Rule exists.
* @return A collection of values returned by getQueueAuthorizationRule.
*/
public suspend fun getQueueAuthorizationRule(
name: String,
namespaceName: String? = null,
queueId: String? = null,
queueName: String? = null,
resourceGroupName: String? = null,
): GetQueueAuthorizationRuleResult {
val argument = GetQueueAuthorizationRulePlainArgs(
name = name,
namespaceName = namespaceName,
queueId = queueId,
queueName = queueName,
resourceGroupName = resourceGroupName,
)
return getQueueAuthorizationRuleResultToKotlin(getQueueAuthorizationRulePlain(argument.toJava()).await())
}
/**
* @see [getQueueAuthorizationRule].
* @param argument Builder for [com.pulumi.azure.servicebus.kotlin.inputs.GetQueueAuthorizationRulePlainArgs].
* @return A collection of values returned by getQueueAuthorizationRule.
*/
public suspend fun getQueueAuthorizationRule(argument: suspend GetQueueAuthorizationRulePlainArgsBuilder.() -> Unit): GetQueueAuthorizationRuleResult {
val builder = GetQueueAuthorizationRulePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getQueueAuthorizationRuleResultToKotlin(getQueueAuthorizationRulePlain(builtArgument.toJava()).await())
}
/**
* Use this data source to access information about an existing ServiceBus Subscription.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = azure.servicebus.getSubscription({
* name: "examplesubscription",
* topicId: "exampletopic",
* });
* export const servicebusSubscription = exampleAzurermServicebusNamespace;
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.servicebus.get_subscription(name="examplesubscription",
* topic_id="exampletopic")
* pulumi.export("servicebusSubscription", example_azurerm_servicebus_namespace)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = Azure.ServiceBus.GetSubscription.Invoke(new()
* {
* Name = "examplesubscription",
* TopicId = "exampletopic",
* });
* return new Dictionary
* {
* ["servicebusSubscription"] = exampleAzurermServicebusNamespace,
* };
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/servicebus"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := servicebus.LookupSubscription(ctx, &servicebus.LookupSubscriptionArgs{
* Name: "examplesubscription",
* TopicId: pulumi.StringRef("exampletopic"),
* }, nil)
* if err != nil {
* return err
* }
* ctx.Export("servicebusSubscription", exampleAzurermServicebusNamespace)
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.servicebus.ServicebusFunctions;
* import com.pulumi.azure.servicebus.inputs.GetSubscriptionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var example = ServicebusFunctions.getSubscription(GetSubscriptionArgs.builder()
* .name("examplesubscription")
* .topicId("exampletopic")
* .build());
* ctx.export("servicebusSubscription", exampleAzurermServicebusNamespace);
* }
* }
* ```
* ```yaml
* variables:
* example:
* fn::invoke:
* Function: azure:servicebus:getSubscription
* Arguments:
* name: examplesubscription
* topicId: exampletopic
* outputs:
* servicebusSubscription: ${exampleAzurermServicebusNamespace}
* ```
*
* @param argument A collection of arguments for invoking getSubscription.
* @return A collection of values returned by getSubscription.
*/
public suspend fun getSubscription(argument: GetSubscriptionPlainArgs): GetSubscriptionResult =
getSubscriptionResultToKotlin(getSubscriptionPlain(argument.toJava()).await())
/**
* @see [getSubscription].
* @param name Specifies the name of the ServiceBus Subscription.
* @param namespaceName The name of the ServiceBus Namespace.
* @param resourceGroupName Specifies the name of the Resource Group where the ServiceBus Namespace exists.
* @param topicId The ID of the ServiceBus Topic where the Service Bus Subscription exists.
* @param topicName The name of the ServiceBus Topic.
* > **Note:** `namespace_name`,`resource_group_name` and `topic_name` has been deprecated and will be removed in version 4.0 of the provider in favour of `topic_id`.
* @return A collection of values returned by getSubscription.
*/
public suspend fun getSubscription(
name: String,
namespaceName: String? = null,
resourceGroupName: String? = null,
topicId: String? = null,
topicName: String? = null,
): GetSubscriptionResult {
val argument = GetSubscriptionPlainArgs(
name = name,
namespaceName = namespaceName,
resourceGroupName = resourceGroupName,
topicId = topicId,
topicName = topicName,
)
return getSubscriptionResultToKotlin(getSubscriptionPlain(argument.toJava()).await())
}
/**
* @see [getSubscription].
* @param argument Builder for [com.pulumi.azure.servicebus.kotlin.inputs.GetSubscriptionPlainArgs].
* @return A collection of values returned by getSubscription.
*/
public suspend fun getSubscription(argument: suspend GetSubscriptionPlainArgsBuilder.() -> Unit): GetSubscriptionResult {
val builder = GetSubscriptionPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getSubscriptionResultToKotlin(getSubscriptionPlain(builtArgument.toJava()).await())
}
/**
* Use this data source to access information about an existing Service Bus Topic.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = azure.servicebus.getTopic({
* name: "existing",
* namespaceId: "existing",
* });
* export const id = example.then(example => example.id);
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.servicebus.get_topic(name="existing",
* namespace_id="existing")
* pulumi.export("id", example.id)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = Azure.ServiceBus.GetTopic.Invoke(new()
* {
* Name = "existing",
* NamespaceId = "existing",
* });
* return new Dictionary
* {
* ["id"] = example.Apply(getTopicResult => getTopicResult.Id),
* };
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/servicebus"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := servicebus.LookupTopic(ctx, &servicebus.LookupTopicArgs{
* Name: "existing",
* NamespaceId: pulumi.StringRef("existing"),
* }, nil)
* if err != nil {
* return err
* }
* ctx.Export("id", example.Id)
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.servicebus.ServicebusFunctions;
* import com.pulumi.azure.servicebus.inputs.GetTopicArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var example = ServicebusFunctions.getTopic(GetTopicArgs.builder()
* .name("existing")
* .namespaceId("existing")
* .build());
* ctx.export("id", example.applyValue(getTopicResult -> getTopicResult.id()));
* }
* }
* ```
* ```yaml
* variables:
* example:
* fn::invoke:
* Function: azure:servicebus:getTopic
* Arguments:
* name: existing
* namespaceId: existing
* outputs:
* id: ${example.id}
* ```
*
* @param argument A collection of arguments for invoking getTopic.
* @return A collection of values returned by getTopic.
*/
public suspend fun getTopic(argument: GetTopicPlainArgs): GetTopicResult =
getTopicResultToKotlin(getTopicPlain(argument.toJava()).await())
/**
* @see [getTopic].
* @param name The name of this Service Bus Topic.
* @param namespaceId The ID of the ServiceBus Namespace where the Service Bus Topic exists.
* @param namespaceName The name of the Service Bus Namespace.
* @param resourceGroupName The name of the Resource Group where the Service Bus Topic exists.
* > **Note:** `namespace_name` and `resource_group_name` has been deprecated and will be removed in version 4.0 of the provider in favour of `namespace_id`.
* @return A collection of values returned by getTopic.
*/
public suspend fun getTopic(
name: String,
namespaceId: String? = null,
namespaceName: String? = null,
resourceGroupName: String? = null,
): GetTopicResult {
val argument = GetTopicPlainArgs(
name = name,
namespaceId = namespaceId,
namespaceName = namespaceName,
resourceGroupName = resourceGroupName,
)
return getTopicResultToKotlin(getTopicPlain(argument.toJava()).await())
}
/**
* @see [getTopic].
* @param argument Builder for [com.pulumi.azure.servicebus.kotlin.inputs.GetTopicPlainArgs].
* @return A collection of values returned by getTopic.
*/
public suspend fun getTopic(argument: suspend GetTopicPlainArgsBuilder.() -> Unit): GetTopicResult {
val builder = GetTopicPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getTopicResultToKotlin(getTopicPlain(builtArgument.toJava()).await())
}
/**
* Use this data source to access information about a ServiceBus Topic Authorization Rule within a ServiceBus Topic.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = azure.servicebus.getTopicAuthorizationRule({
* name: "example-tfex_name",
* resourceGroupName: "example-resources",
* namespaceName: "example-namespace",
* topicName: "example-servicebus_topic",
* });
* export const servicebusAuthorizationRuleId = exampleAzuremServicebusTopicAuthorizationRule.id;
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.servicebus.get_topic_authorization_rule(name="example-tfex_name",
* resource_group_name="example-resources",
* namespace_name="example-namespace",
* topic_name="example-servicebus_topic")
* pulumi.export("servicebusAuthorizationRuleId", example_azurem_servicebus_topic_authorization_rule["id"])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = Azure.ServiceBus.GetTopicAuthorizationRule.Invoke(new()
* {
* Name = "example-tfex_name",
* ResourceGroupName = "example-resources",
* NamespaceName = "example-namespace",
* TopicName = "example-servicebus_topic",
* });
* return new Dictionary
* {
* ["servicebusAuthorizationRuleId"] = exampleAzuremServicebusTopicAuthorizationRule.Id,
* };
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/servicebus"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := servicebus.LookupTopicAuthorizationRule(ctx, &servicebus.LookupTopicAuthorizationRuleArgs{
* Name: "example-tfex_name",
* ResourceGroupName: pulumi.StringRef("example-resources"),
* NamespaceName: pulumi.StringRef("example-namespace"),
* TopicName: pulumi.StringRef("example-servicebus_topic"),
* }, nil)
* if err != nil {
* return err
* }
* ctx.Export("servicebusAuthorizationRuleId", exampleAzuremServicebusTopicAuthorizationRule.Id)
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.servicebus.ServicebusFunctions;
* import com.pulumi.azure.servicebus.inputs.GetTopicAuthorizationRuleArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var example = ServicebusFunctions.getTopicAuthorizationRule(GetTopicAuthorizationRuleArgs.builder()
* .name("example-tfex_name")
* .resourceGroupName("example-resources")
* .namespaceName("example-namespace")
* .topicName("example-servicebus_topic")
* .build());
* ctx.export("servicebusAuthorizationRuleId", exampleAzuremServicebusTopicAuthorizationRule.id());
* }
* }
* ```
* ```yaml
* variables:
* example:
* fn::invoke:
* Function: azure:servicebus:getTopicAuthorizationRule
* Arguments:
* name: example-tfex_name
* resourceGroupName: example-resources
* namespaceName: example-namespace
* topicName: example-servicebus_topic
* outputs:
* servicebusAuthorizationRuleId: ${exampleAzuremServicebusTopicAuthorizationRule.id}
* ```
*
* @param argument A collection of arguments for invoking getTopicAuthorizationRule.
* @return A collection of values returned by getTopicAuthorizationRule.
*/
public suspend fun getTopicAuthorizationRule(argument: GetTopicAuthorizationRulePlainArgs): GetTopicAuthorizationRuleResult =
getTopicAuthorizationRuleResultToKotlin(getTopicAuthorizationRulePlain(argument.toJava()).await())
/**
* @see [getTopicAuthorizationRule].
* @param name The name of the ServiceBus Topic Authorization Rule resource.
* @param namespaceName The name of the ServiceBus Namespace.
* @param queueName
* @param resourceGroupName The name of the resource group in which the ServiceBus Namespace exists.
* @param topicId
* @param topicName The name of the ServiceBus Topic.
* @return A collection of values returned by getTopicAuthorizationRule.
*/
public suspend fun getTopicAuthorizationRule(
name: String,
namespaceName: String? = null,
queueName: String? = null,
resourceGroupName: String? = null,
topicId: String? = null,
topicName: String? = null,
): GetTopicAuthorizationRuleResult {
val argument = GetTopicAuthorizationRulePlainArgs(
name = name,
namespaceName = namespaceName,
queueName = queueName,
resourceGroupName = resourceGroupName,
topicId = topicId,
topicName = topicName,
)
return getTopicAuthorizationRuleResultToKotlin(getTopicAuthorizationRulePlain(argument.toJava()).await())
}
/**
* @see [getTopicAuthorizationRule].
* @param argument Builder for [com.pulumi.azure.servicebus.kotlin.inputs.GetTopicAuthorizationRulePlainArgs].
* @return A collection of values returned by getTopicAuthorizationRule.
*/
public suspend fun getTopicAuthorizationRule(argument: suspend GetTopicAuthorizationRulePlainArgsBuilder.() -> Unit): GetTopicAuthorizationRuleResult {
val builder = GetTopicAuthorizationRulePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getTopicAuthorizationRuleResultToKotlin(getTopicAuthorizationRulePlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy