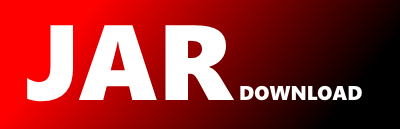
com.pulumi.azure.signalr.kotlin.Service.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.signalr.kotlin
import com.pulumi.azure.signalr.kotlin.outputs.ServiceCor
import com.pulumi.azure.signalr.kotlin.outputs.ServiceIdentity
import com.pulumi.azure.signalr.kotlin.outputs.ServiceLiveTrace
import com.pulumi.azure.signalr.kotlin.outputs.ServiceSku
import com.pulumi.azure.signalr.kotlin.outputs.ServiceUpstreamEndpoint
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import com.pulumi.azure.signalr.kotlin.outputs.ServiceCor.Companion.toKotlin as serviceCorToKotlin
import com.pulumi.azure.signalr.kotlin.outputs.ServiceIdentity.Companion.toKotlin as serviceIdentityToKotlin
import com.pulumi.azure.signalr.kotlin.outputs.ServiceLiveTrace.Companion.toKotlin as serviceLiveTraceToKotlin
import com.pulumi.azure.signalr.kotlin.outputs.ServiceSku.Companion.toKotlin as serviceSkuToKotlin
import com.pulumi.azure.signalr.kotlin.outputs.ServiceUpstreamEndpoint.Companion.toKotlin as serviceUpstreamEndpointToKotlin
/**
* Builder for [Service].
*/
@PulumiTagMarker
public class ServiceResourceBuilder internal constructor() {
public var name: String? = null
public var args: ServiceArgs = ServiceArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend ServiceArgsBuilder.() -> Unit) {
val builder = ServiceArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Service {
val builtJavaResource = com.pulumi.azure.signalr.Service(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Service(builtJavaResource)
}
}
/**
* Manages an Azure SignalR service.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = new azure.core.ResourceGroup("example", {
* name: "my-signalr",
* location: "West US",
* });
* const exampleService = new azure.signalr.Service("example", {
* name: "tfex-signalr",
* location: example.location,
* resourceGroupName: example.name,
* sku: {
* name: "Free_F1",
* capacity: 1,
* },
* cors: [{
* allowedOrigins: ["http://www.example.com"],
* }],
* publicNetworkAccessEnabled: false,
* connectivityLogsEnabled: true,
* messagingLogsEnabled: true,
* serviceMode: "Default",
* upstreamEndpoints: [{
* categoryPatterns: [
* "connections",
* "messages",
* ],
* eventPatterns: ["*"],
* hubPatterns: ["hub1"],
* urlTemplate: "http://foo.com",
* }],
* });
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.core.ResourceGroup("example",
* name="my-signalr",
* location="West US")
* example_service = azure.signalr.Service("example",
* name="tfex-signalr",
* location=example.location,
* resource_group_name=example.name,
* sku={
* "name": "Free_F1",
* "capacity": 1,
* },
* cors=[{
* "allowed_origins": ["http://www.example.com"],
* }],
* public_network_access_enabled=False,
* connectivity_logs_enabled=True,
* messaging_logs_enabled=True,
* service_mode="Default",
* upstream_endpoints=[{
* "category_patterns": [
* "connections",
* "messages",
* ],
* "event_patterns": ["*"],
* "hub_patterns": ["hub1"],
* "url_template": "http://foo.com",
* }])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = new Azure.Core.ResourceGroup("example", new()
* {
* Name = "my-signalr",
* Location = "West US",
* });
* var exampleService = new Azure.SignalR.Service("example", new()
* {
* Name = "tfex-signalr",
* Location = example.Location,
* ResourceGroupName = example.Name,
* Sku = new Azure.SignalR.Inputs.ServiceSkuArgs
* {
* Name = "Free_F1",
* Capacity = 1,
* },
* Cors = new[]
* {
* new Azure.SignalR.Inputs.ServiceCorArgs
* {
* AllowedOrigins = new[]
* {
* "http://www.example.com",
* },
* },
* },
* PublicNetworkAccessEnabled = false,
* ConnectivityLogsEnabled = true,
* MessagingLogsEnabled = true,
* ServiceMode = "Default",
* UpstreamEndpoints = new[]
* {
* new Azure.SignalR.Inputs.ServiceUpstreamEndpointArgs
* {
* CategoryPatterns = new[]
* {
* "connections",
* "messages",
* },
* EventPatterns = new[]
* {
* "*",
* },
* HubPatterns = new[]
* {
* "hub1",
* },
* UrlTemplate = "http://foo.com",
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/core"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/signalr"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := core.NewResourceGroup(ctx, "example", &core.ResourceGroupArgs{
* Name: pulumi.String("my-signalr"),
* Location: pulumi.String("West US"),
* })
* if err != nil {
* return err
* }
* _, err = signalr.NewService(ctx, "example", &signalr.ServiceArgs{
* Name: pulumi.String("tfex-signalr"),
* Location: example.Location,
* ResourceGroupName: example.Name,
* Sku: &signalr.ServiceSkuArgs{
* Name: pulumi.String("Free_F1"),
* Capacity: pulumi.Int(1),
* },
* Cors: signalr.ServiceCorArray{
* &signalr.ServiceCorArgs{
* AllowedOrigins: pulumi.StringArray{
* pulumi.String("http://www.example.com"),
* },
* },
* },
* PublicNetworkAccessEnabled: pulumi.Bool(false),
* ConnectivityLogsEnabled: pulumi.Bool(true),
* MessagingLogsEnabled: pulumi.Bool(true),
* ServiceMode: pulumi.String("Default"),
* UpstreamEndpoints: signalr.ServiceUpstreamEndpointArray{
* &signalr.ServiceUpstreamEndpointArgs{
* CategoryPatterns: pulumi.StringArray{
* pulumi.String("connections"),
* pulumi.String("messages"),
* },
* EventPatterns: pulumi.StringArray{
* pulumi.String("*"),
* },
* HubPatterns: pulumi.StringArray{
* pulumi.String("hub1"),
* },
* UrlTemplate: pulumi.String("http://foo.com"),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.signalr.Service;
* import com.pulumi.azure.signalr.ServiceArgs;
* import com.pulumi.azure.signalr.inputs.ServiceSkuArgs;
* import com.pulumi.azure.signalr.inputs.ServiceCorArgs;
* import com.pulumi.azure.signalr.inputs.ServiceUpstreamEndpointArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new ResourceGroup("example", ResourceGroupArgs.builder()
* .name("my-signalr")
* .location("West US")
* .build());
* var exampleService = new Service("exampleService", ServiceArgs.builder()
* .name("tfex-signalr")
* .location(example.location())
* .resourceGroupName(example.name())
* .sku(ServiceSkuArgs.builder()
* .name("Free_F1")
* .capacity(1)
* .build())
* .cors(ServiceCorArgs.builder()
* .allowedOrigins("http://www.example.com")
* .build())
* .publicNetworkAccessEnabled(false)
* .connectivityLogsEnabled(true)
* .messagingLogsEnabled(true)
* .serviceMode("Default")
* .upstreamEndpoints(ServiceUpstreamEndpointArgs.builder()
* .categoryPatterns(
* "connections",
* "messages")
* .eventPatterns("*")
* .hubPatterns("hub1")
* .urlTemplate("http://foo.com")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: azure:core:ResourceGroup
* properties:
* name: my-signalr
* location: West US
* exampleService:
* type: azure:signalr:Service
* name: example
* properties:
* name: tfex-signalr
* location: ${example.location}
* resourceGroupName: ${example.name}
* sku:
* name: Free_F1
* capacity: 1
* cors:
* - allowedOrigins:
* - http://www.example.com
* publicNetworkAccessEnabled: false
* connectivityLogsEnabled: true
* messagingLogsEnabled: true
* serviceMode: Default
* upstreamEndpoints:
* - categoryPatterns:
* - connections
* - messages
* eventPatterns:
* - '*'
* hubPatterns:
* - hub1
* urlTemplate: http://foo.com
* ```
*
* ## Import
* SignalR services can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:signalr/service:Service example /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/terraform-signalr/providers/Microsoft.SignalRService/signalR/tfex-signalr
* ```
*/
public class Service internal constructor(
override val javaResource: com.pulumi.azure.signalr.Service,
) : KotlinCustomResource(javaResource, ServiceMapper) {
/**
* Whether to enable AAD auth? Defaults to `true`.
*/
public val aadAuthEnabled: Output?
get() = javaResource.aadAuthEnabled().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Specifies if Connectivity Logs are enabled or not. Defaults to `false`.
*/
public val connectivityLogsEnabled: Output?
get() = javaResource.connectivityLogsEnabled().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* A `cors` block as documented below.
*/
public val cors: Output>
get() = javaResource.cors().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
serviceCorToKotlin(args0)
})
})
})
/**
* The FQDN of the SignalR service.
*/
public val hostname: Output
get() = javaResource.hostname().applyValue({ args0 -> args0 })
/**
* Specifies if Http Request Logs are enabled or not. Defaults to `false`.
*/
public val httpRequestLogsEnabled: Output?
get() = javaResource.httpRequestLogsEnabled().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* An `identity` block as defined below.
*/
public val identity: Output?
get() = javaResource.identity().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
serviceIdentityToKotlin(args0)
})
}).orElse(null)
})
/**
* The publicly accessible IP of the SignalR service.
*/
public val ipAddress: Output
get() = javaResource.ipAddress().applyValue({ args0 -> args0 })
/**
* A `live_trace` block as defined below.
*/
public val liveTrace: Output?
get() = javaResource.liveTrace().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
serviceLiveTraceToKotlin(args0)
})
}).orElse(null)
})
/**
* Specifies if Live Trace is enabled or not. Defaults to `false`.
*/
@Deprecated(
message = """
`live_trace_enabled` has been deprecated in favor of `live_trace` and will be removed in 4.0.
""",
)
public val liveTraceEnabled: Output?
get() = javaResource.liveTraceEnabled().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Whether to enable local auth? Defaults to `true`.
*/
public val localAuthEnabled: Output?
get() = javaResource.localAuthEnabled().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Specifies the supported Azure location where the SignalR service exists. Changing this forces a new resource to be created.
*/
public val location: Output
get() = javaResource.location().applyValue({ args0 -> args0 })
/**
* Specifies if Messaging Logs are enabled or not. Defaults to `false`.
*/
public val messagingLogsEnabled: Output?
get() = javaResource.messagingLogsEnabled().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The name of the SignalR service. Changing this forces a new resource to be created.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* The primary access key for the SignalR service.
*/
public val primaryAccessKey: Output
get() = javaResource.primaryAccessKey().applyValue({ args0 -> args0 })
/**
* The primary connection string for the SignalR service.
*/
public val primaryConnectionString: Output
get() = javaResource.primaryConnectionString().applyValue({ args0 -> args0 })
/**
* Whether to enable public network access? Defaults to `true`.
* > **Note:** `public_network_access_enabled` cannot be set to `false` in `Free` sku tier.
*/
public val publicNetworkAccessEnabled: Output?
get() = javaResource.publicNetworkAccessEnabled().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The publicly accessible port of the SignalR service which is designed for browser/client use.
*/
public val publicPort: Output
get() = javaResource.publicPort().applyValue({ args0 -> args0 })
/**
* The name of the resource group in which to create the SignalR service. Changing this forces a new resource to be created.
*/
public val resourceGroupName: Output
get() = javaResource.resourceGroupName().applyValue({ args0 -> args0 })
/**
* The secondary access key for the SignalR service.
*/
public val secondaryAccessKey: Output
get() = javaResource.secondaryAccessKey().applyValue({ args0 -> args0 })
/**
* The secondary connection string for the SignalR service.
*/
public val secondaryConnectionString: Output
get() = javaResource.secondaryConnectionString().applyValue({ args0 -> args0 })
/**
* The publicly accessible port of the SignalR service which is designed for customer server side use.
*/
public val serverPort: Output
get() = javaResource.serverPort().applyValue({ args0 -> args0 })
/**
* Specifies the client connection timeout. Defaults to `30`.
*/
public val serverlessConnectionTimeoutInSeconds: Output?
get() = javaResource.serverlessConnectionTimeoutInSeconds().applyValue({ args0 ->
args0.map({ args0 -> args0 }).orElse(null)
})
/**
* Specifies the service mode. Possible values are `Classic`, `Default` and `Serverless`. Defaults to `Default`.
*/
public val serviceMode: Output?
get() = javaResource.serviceMode().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* A `sku` block as documented below.
*/
public val sku: Output
get() = javaResource.sku().applyValue({ args0 ->
args0.let({ args0 ->
serviceSkuToKotlin(args0)
})
})
/**
* A mapping of tags to assign to the resource.
*/
public val tags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy