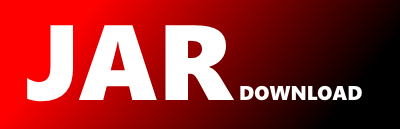
com.pulumi.azure.siterecovery.kotlin.inputs.VmwareReplicatedVmNetworkInterfaceArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.siterecovery.kotlin.inputs
import com.pulumi.azure.siterecovery.inputs.VmwareReplicatedVmNetworkInterfaceArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property isPrimary Whether this `network_interface` is primary for the replicated VM.
* @property sourceMacAddress Mac address of the network interface of source VM.
* @property targetStaticIp Static IP to assign when a failover is done.
* @property targetSubnetName Name of the subnet to use when a failover is done.
* @property testSubnetName Name of the subnet to use when a test failover is done.
*/
public data class VmwareReplicatedVmNetworkInterfaceArgs(
public val isPrimary: Output,
public val sourceMacAddress: Output,
public val targetStaticIp: Output? = null,
public val targetSubnetName: Output? = null,
public val testSubnetName: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.siterecovery.inputs.VmwareReplicatedVmNetworkInterfaceArgs = com.pulumi.azure.siterecovery.inputs.VmwareReplicatedVmNetworkInterfaceArgs.builder()
.isPrimary(isPrimary.applyValue({ args0 -> args0 }))
.sourceMacAddress(sourceMacAddress.applyValue({ args0 -> args0 }))
.targetStaticIp(targetStaticIp?.applyValue({ args0 -> args0 }))
.targetSubnetName(targetSubnetName?.applyValue({ args0 -> args0 }))
.testSubnetName(testSubnetName?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [VmwareReplicatedVmNetworkInterfaceArgs].
*/
@PulumiTagMarker
public class VmwareReplicatedVmNetworkInterfaceArgsBuilder internal constructor() {
private var isPrimary: Output? = null
private var sourceMacAddress: Output? = null
private var targetStaticIp: Output? = null
private var targetSubnetName: Output? = null
private var testSubnetName: Output? = null
/**
* @param value Whether this `network_interface` is primary for the replicated VM.
*/
@JvmName("rsbfjemjrolbamow")
public suspend fun isPrimary(`value`: Output) {
this.isPrimary = value
}
/**
* @param value Mac address of the network interface of source VM.
*/
@JvmName("xwcesxieohutqebd")
public suspend fun sourceMacAddress(`value`: Output) {
this.sourceMacAddress = value
}
/**
* @param value Static IP to assign when a failover is done.
*/
@JvmName("tgwgowgpqpaxmhvy")
public suspend fun targetStaticIp(`value`: Output) {
this.targetStaticIp = value
}
/**
* @param value Name of the subnet to use when a failover is done.
*/
@JvmName("ldpmoagjnsnorbty")
public suspend fun targetSubnetName(`value`: Output) {
this.targetSubnetName = value
}
/**
* @param value Name of the subnet to use when a test failover is done.
*/
@JvmName("cfetgbcekbawofqw")
public suspend fun testSubnetName(`value`: Output) {
this.testSubnetName = value
}
/**
* @param value Whether this `network_interface` is primary for the replicated VM.
*/
@JvmName("hfdycgbfhvpfruwf")
public suspend fun isPrimary(`value`: Boolean) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.isPrimary = mapped
}
/**
* @param value Mac address of the network interface of source VM.
*/
@JvmName("myxxqcccavqbpbdo")
public suspend fun sourceMacAddress(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.sourceMacAddress = mapped
}
/**
* @param value Static IP to assign when a failover is done.
*/
@JvmName("vjjpygispxxtgvwi")
public suspend fun targetStaticIp(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.targetStaticIp = mapped
}
/**
* @param value Name of the subnet to use when a failover is done.
*/
@JvmName("uhflvaguvqlfunny")
public suspend fun targetSubnetName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.targetSubnetName = mapped
}
/**
* @param value Name of the subnet to use when a test failover is done.
*/
@JvmName("udlykudkywkquccw")
public suspend fun testSubnetName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.testSubnetName = mapped
}
internal fun build(): VmwareReplicatedVmNetworkInterfaceArgs =
VmwareReplicatedVmNetworkInterfaceArgs(
isPrimary = isPrimary ?: throw PulumiNullFieldException("isPrimary"),
sourceMacAddress = sourceMacAddress ?: throw PulumiNullFieldException("sourceMacAddress"),
targetStaticIp = targetStaticIp,
targetSubnetName = targetSubnetName,
testSubnetName = testSubnetName,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy