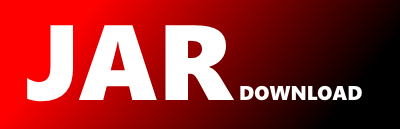
com.pulumi.azure.storage.kotlin.inputs.AccountBlobPropertiesArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.storage.kotlin.inputs
import com.pulumi.azure.storage.inputs.AccountBlobPropertiesArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property changeFeedEnabled Is the blob service properties for change feed events enabled? Default to `false`.
* > **Note:** This field cannot be configured when `kind` is set to `Storage` (V1).
* @property changeFeedRetentionInDays The duration of change feed events retention in days. The possible values are between 1 and 146000 days (400 years). Setting this to null (or omit this in the configuration file) indicates an infinite retention of the change feed.
* > **Note:** This field cannot be configured when `kind` is set to `Storage` (V1).
* @property containerDeleteRetentionPolicy A `container_delete_retention_policy` block as defined below.
* @property corsRules A `cors_rule` block as defined below.
* @property defaultServiceVersion The API Version which should be used by default for requests to the Data Plane API if an incoming request doesn't specify an API Version.
* @property deleteRetentionPolicy A `delete_retention_policy` block as defined below.
* @property lastAccessTimeEnabled Is the last access time based tracking enabled? Default to `false`.
* > **Note:** This field cannot be configured when `kind` is set to `Storage` (V1).
* @property restorePolicy A `restore_policy` block as defined below. This must be used together with `delete_retention_policy` set, `versioning_enabled` and `change_feed_enabled` set to `true`.
* > **Note:** This field cannot be configured when `kind` is set to `Storage` (V1).
* > **Note:** `restore_policy` can not be configured when `dns_endpoint_type` is `AzureDnsZone`.
* @property versioningEnabled Is versioning enabled? Default to `false`.
* > **Note:** This field cannot be configured when `kind` is set to `Storage` (V1).
*/
public data class AccountBlobPropertiesArgs(
public val changeFeedEnabled: Output? = null,
public val changeFeedRetentionInDays: Output? = null,
public val containerDeleteRetentionPolicy: Output? = null,
public val corsRules: Output>? = null,
public val defaultServiceVersion: Output? = null,
public val deleteRetentionPolicy: Output? = null,
public val lastAccessTimeEnabled: Output? = null,
public val restorePolicy: Output? = null,
public val versioningEnabled: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.storage.inputs.AccountBlobPropertiesArgs =
com.pulumi.azure.storage.inputs.AccountBlobPropertiesArgs.builder()
.changeFeedEnabled(changeFeedEnabled?.applyValue({ args0 -> args0 }))
.changeFeedRetentionInDays(changeFeedRetentionInDays?.applyValue({ args0 -> args0 }))
.containerDeleteRetentionPolicy(
containerDeleteRetentionPolicy?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.corsRules(
corsRules?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.defaultServiceVersion(defaultServiceVersion?.applyValue({ args0 -> args0 }))
.deleteRetentionPolicy(
deleteRetentionPolicy?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.lastAccessTimeEnabled(lastAccessTimeEnabled?.applyValue({ args0 -> args0 }))
.restorePolicy(restorePolicy?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.versioningEnabled(versioningEnabled?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [AccountBlobPropertiesArgs].
*/
@PulumiTagMarker
public class AccountBlobPropertiesArgsBuilder internal constructor() {
private var changeFeedEnabled: Output? = null
private var changeFeedRetentionInDays: Output? = null
private var containerDeleteRetentionPolicy:
Output? = null
private var corsRules: Output>? = null
private var defaultServiceVersion: Output? = null
private var deleteRetentionPolicy: Output? = null
private var lastAccessTimeEnabled: Output? = null
private var restorePolicy: Output? = null
private var versioningEnabled: Output? = null
/**
* @param value Is the blob service properties for change feed events enabled? Default to `false`.
* > **Note:** This field cannot be configured when `kind` is set to `Storage` (V1).
*/
@JvmName("omlecqejubjjdyea")
public suspend fun changeFeedEnabled(`value`: Output) {
this.changeFeedEnabled = value
}
/**
* @param value The duration of change feed events retention in days. The possible values are between 1 and 146000 days (400 years). Setting this to null (or omit this in the configuration file) indicates an infinite retention of the change feed.
* > **Note:** This field cannot be configured when `kind` is set to `Storage` (V1).
*/
@JvmName("qfvgpelfodcjcjxm")
public suspend fun changeFeedRetentionInDays(`value`: Output) {
this.changeFeedRetentionInDays = value
}
/**
* @param value A `container_delete_retention_policy` block as defined below.
*/
@JvmName("rhdnxlaywmcpokke")
public suspend fun containerDeleteRetentionPolicy(`value`: Output) {
this.containerDeleteRetentionPolicy = value
}
/**
* @param value A `cors_rule` block as defined below.
*/
@JvmName("prxupadarqesrfsu")
public suspend fun corsRules(`value`: Output>) {
this.corsRules = value
}
@JvmName("uilxeumcpdoyrbil")
public suspend fun corsRules(vararg values: Output) {
this.corsRules = Output.all(values.asList())
}
/**
* @param values A `cors_rule` block as defined below.
*/
@JvmName("aellksyuqnoxhmcw")
public suspend fun corsRules(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy