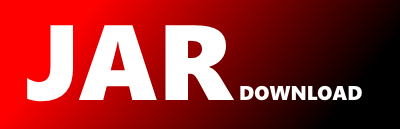
com.pulumi.azure.storage.kotlin.inputs.GetAccountBlobContainerSASPlainArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.storage.kotlin.inputs
import com.pulumi.azure.storage.inputs.GetAccountBlobContainerSASPlainArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* A collection of arguments for invoking getAccountBlobContainerSAS.
* @property cacheControl The `Cache-Control` response header that is sent when this SAS token is used.
* @property connectionString The connection string for the storage account to which this SAS applies. Typically directly from the `primary_connection_string` attribute of an `azure.storage.Account` resource.
* @property containerName Name of the container.
* @property contentDisposition The `Content-Disposition` response header that is sent when this SAS token is used.
* @property contentEncoding The `Content-Encoding` response header that is sent when this SAS token is used.
* @property contentLanguage The `Content-Language` response header that is sent when this SAS token is used.
* @property contentType The `Content-Type` response header that is sent when this SAS token is used.
* @property expiry The expiration time and date of this SAS. Must be a valid ISO-8601 format time/date string.
* > **NOTE:** The [ISO-8601 Time offset from UTC](https://en.wikipedia.org/wiki/ISO_8601#Time_offsets_from_UTC) is currently not supported by the service, which will result into 409 error.
* @property httpsOnly Only permit `https` access. If `false`, both `http` and `https` are permitted. Defaults to `true`.
* @property ipAddress Single IPv4 address or range (connected with a dash) of IPv4 addresses.
* @property permissions A `permissions` block as defined below.
* @property start The starting time and date of validity of this SAS. Must be a valid ISO-8601 format time/date string.
*/
public data class GetAccountBlobContainerSASPlainArgs(
public val cacheControl: String? = null,
public val connectionString: String,
public val containerName: String,
public val contentDisposition: String? = null,
public val contentEncoding: String? = null,
public val contentLanguage: String? = null,
public val contentType: String? = null,
public val expiry: String,
public val httpsOnly: Boolean? = null,
public val ipAddress: String? = null,
public val permissions: GetAccountBlobContainerSASPermissions,
public val start: String,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.storage.inputs.GetAccountBlobContainerSASPlainArgs =
com.pulumi.azure.storage.inputs.GetAccountBlobContainerSASPlainArgs.builder()
.cacheControl(cacheControl?.let({ args0 -> args0 }))
.connectionString(connectionString.let({ args0 -> args0 }))
.containerName(containerName.let({ args0 -> args0 }))
.contentDisposition(contentDisposition?.let({ args0 -> args0 }))
.contentEncoding(contentEncoding?.let({ args0 -> args0 }))
.contentLanguage(contentLanguage?.let({ args0 -> args0 }))
.contentType(contentType?.let({ args0 -> args0 }))
.expiry(expiry.let({ args0 -> args0 }))
.httpsOnly(httpsOnly?.let({ args0 -> args0 }))
.ipAddress(ipAddress?.let({ args0 -> args0 }))
.permissions(permissions.let({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.start(start.let({ args0 -> args0 })).build()
}
/**
* Builder for [GetAccountBlobContainerSASPlainArgs].
*/
@PulumiTagMarker
public class GetAccountBlobContainerSASPlainArgsBuilder internal constructor() {
private var cacheControl: String? = null
private var connectionString: String? = null
private var containerName: String? = null
private var contentDisposition: String? = null
private var contentEncoding: String? = null
private var contentLanguage: String? = null
private var contentType: String? = null
private var expiry: String? = null
private var httpsOnly: Boolean? = null
private var ipAddress: String? = null
private var permissions: GetAccountBlobContainerSASPermissions? = null
private var start: String? = null
/**
* @param value The `Cache-Control` response header that is sent when this SAS token is used.
*/
@JvmName("xaqacogfedrcswpa")
public suspend fun cacheControl(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.cacheControl = mapped
}
/**
* @param value The connection string for the storage account to which this SAS applies. Typically directly from the `primary_connection_string` attribute of an `azure.storage.Account` resource.
*/
@JvmName("feuovbfgjvwempiu")
public suspend fun connectionString(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.connectionString = mapped
}
/**
* @param value Name of the container.
*/
@JvmName("sjrefuisqiosnido")
public suspend fun containerName(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.containerName = mapped
}
/**
* @param value The `Content-Disposition` response header that is sent when this SAS token is used.
*/
@JvmName("mxnltnvjnbgqilvh")
public suspend fun contentDisposition(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.contentDisposition = mapped
}
/**
* @param value The `Content-Encoding` response header that is sent when this SAS token is used.
*/
@JvmName("chijckqhroddgftp")
public suspend fun contentEncoding(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.contentEncoding = mapped
}
/**
* @param value The `Content-Language` response header that is sent when this SAS token is used.
*/
@JvmName("xdlcwraeltamifxw")
public suspend fun contentLanguage(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.contentLanguage = mapped
}
/**
* @param value The `Content-Type` response header that is sent when this SAS token is used.
*/
@JvmName("gepsftolwqyyunlh")
public suspend fun contentType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.contentType = mapped
}
/**
* @param value The expiration time and date of this SAS. Must be a valid ISO-8601 format time/date string.
* > **NOTE:** The [ISO-8601 Time offset from UTC](https://en.wikipedia.org/wiki/ISO_8601#Time_offsets_from_UTC) is currently not supported by the service, which will result into 409 error.
*/
@JvmName("ioxsskjayurrdcfs")
public suspend fun expiry(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.expiry = mapped
}
/**
* @param value Only permit `https` access. If `false`, both `http` and `https` are permitted. Defaults to `true`.
*/
@JvmName("taviugdfmkromokv")
public suspend fun httpsOnly(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.httpsOnly = mapped
}
/**
* @param value Single IPv4 address or range (connected with a dash) of IPv4 addresses.
*/
@JvmName("iselldqthnitmyyh")
public suspend fun ipAddress(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.ipAddress = mapped
}
/**
* @param value A `permissions` block as defined below.
*/
@JvmName("yvuvuohbcxdgefbc")
public suspend fun permissions(`value`: GetAccountBlobContainerSASPermissions) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.permissions = mapped
}
/**
* @param argument A `permissions` block as defined below.
*/
@JvmName("fswayrxrvsjgniec")
public suspend fun permissions(argument: suspend GetAccountBlobContainerSASPermissionsBuilder.() -> Unit) {
val toBeMapped = GetAccountBlobContainerSASPermissionsBuilder().applySuspend {
argument()
}.build()
val mapped = toBeMapped
this.permissions = mapped
}
/**
* @param value The starting time and date of validity of this SAS. Must be a valid ISO-8601 format time/date string.
*/
@JvmName("fwqjspowvsabxsja")
public suspend fun start(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.start = mapped
}
internal fun build(): GetAccountBlobContainerSASPlainArgs = GetAccountBlobContainerSASPlainArgs(
cacheControl = cacheControl,
connectionString = connectionString ?: throw PulumiNullFieldException("connectionString"),
containerName = containerName ?: throw PulumiNullFieldException("containerName"),
contentDisposition = contentDisposition,
contentEncoding = contentEncoding,
contentLanguage = contentLanguage,
contentType = contentType,
expiry = expiry ?: throw PulumiNullFieldException("expiry"),
httpsOnly = httpsOnly,
ipAddress = ipAddress,
permissions = permissions ?: throw PulumiNullFieldException("permissions"),
start = start ?: throw PulumiNullFieldException("start"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy