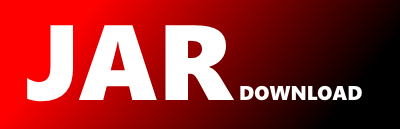
com.pulumi.azure.storage.kotlin.inputs.GetAccountSASPermissions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.storage.kotlin.inputs
import com.pulumi.azure.storage.inputs.GetAccountSASPermissions.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property add Should Add permissions be enabled for this SAS?
* @property create Should Create permissions be enabled for this SAS?
* @property delete Should Delete permissions be enabled for this SAS?
* @property filter Should Filter by Index Tags permissions be enabled for this SAS?
* Refer to the [SAS creation reference from Azure](https://docs.microsoft.com/rest/api/storageservices/constructing-an-account-sas)
* for additional details on the fields above.
* @property list Should List permissions be enabled for this SAS?
* @property process Should Process permissions be enabled for this SAS?
* @property read Should Read permissions be enabled for this SAS?
* @property tag Should Get / Set Index Tags permissions be enabled for this SAS?
* @property update Should Update permissions be enabled for this SAS?
* @property write Should Write permissions be enabled for this SAS?
*/
public data class GetAccountSASPermissions(
public val add: Boolean,
public val create: Boolean,
public val delete: Boolean,
public val filter: Boolean,
public val list: Boolean,
public val process: Boolean,
public val read: Boolean,
public val tag: Boolean,
public val update: Boolean,
public val write: Boolean,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.storage.inputs.GetAccountSASPermissions =
com.pulumi.azure.storage.inputs.GetAccountSASPermissions.builder()
.add(add.let({ args0 -> args0 }))
.create(create.let({ args0 -> args0 }))
.delete(delete.let({ args0 -> args0 }))
.filter(filter.let({ args0 -> args0 }))
.list(list.let({ args0 -> args0 }))
.process(process.let({ args0 -> args0 }))
.read(read.let({ args0 -> args0 }))
.tag(tag.let({ args0 -> args0 }))
.update(update.let({ args0 -> args0 }))
.write(write.let({ args0 -> args0 })).build()
}
/**
* Builder for [GetAccountSASPermissions].
*/
@PulumiTagMarker
public class GetAccountSASPermissionsBuilder internal constructor() {
private var add: Boolean? = null
private var create: Boolean? = null
private var delete: Boolean? = null
private var filter: Boolean? = null
private var list: Boolean? = null
private var process: Boolean? = null
private var read: Boolean? = null
private var tag: Boolean? = null
private var update: Boolean? = null
private var write: Boolean? = null
/**
* @param value Should Add permissions be enabled for this SAS?
*/
@JvmName("khbfescjbkiavslj")
public suspend fun add(`value`: Boolean) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.add = mapped
}
/**
* @param value Should Create permissions be enabled for this SAS?
*/
@JvmName("tnwuyhuqwbtpwvcu")
public suspend fun create(`value`: Boolean) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.create = mapped
}
/**
* @param value Should Delete permissions be enabled for this SAS?
*/
@JvmName("gqbsdssivvwfbtjx")
public suspend fun delete(`value`: Boolean) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.delete = mapped
}
/**
* @param value Should Filter by Index Tags permissions be enabled for this SAS?
* Refer to the [SAS creation reference from Azure](https://docs.microsoft.com/rest/api/storageservices/constructing-an-account-sas)
* for additional details on the fields above.
*/
@JvmName("amlsxnromabohfdf")
public suspend fun filter(`value`: Boolean) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.filter = mapped
}
/**
* @param value Should List permissions be enabled for this SAS?
*/
@JvmName("jariredxclsejkwn")
public suspend fun list(`value`: Boolean) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.list = mapped
}
/**
* @param value Should Process permissions be enabled for this SAS?
*/
@JvmName("arkejwatxilgonoh")
public suspend fun process(`value`: Boolean) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.process = mapped
}
/**
* @param value Should Read permissions be enabled for this SAS?
*/
@JvmName("vtntbwiwgwuoieva")
public suspend fun read(`value`: Boolean) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.read = mapped
}
/**
* @param value Should Get / Set Index Tags permissions be enabled for this SAS?
*/
@JvmName("weiwasoklvwyqsuc")
public suspend fun tag(`value`: Boolean) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.tag = mapped
}
/**
* @param value Should Update permissions be enabled for this SAS?
*/
@JvmName("ructfgtbfllsyata")
public suspend fun update(`value`: Boolean) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.update = mapped
}
/**
* @param value Should Write permissions be enabled for this SAS?
*/
@JvmName("scrcykdgrjnkhlhs")
public suspend fun write(`value`: Boolean) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.write = mapped
}
internal fun build(): GetAccountSASPermissions = GetAccountSASPermissions(
add = add ?: throw PulumiNullFieldException("add"),
create = create ?: throw PulumiNullFieldException("create"),
delete = delete ?: throw PulumiNullFieldException("delete"),
filter = filter ?: throw PulumiNullFieldException("filter"),
list = list ?: throw PulumiNullFieldException("list"),
process = process ?: throw PulumiNullFieldException("process"),
read = read ?: throw PulumiNullFieldException("read"),
tag = tag ?: throw PulumiNullFieldException("tag"),
update = update ?: throw PulumiNullFieldException("update"),
write = write ?: throw PulumiNullFieldException("write"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy