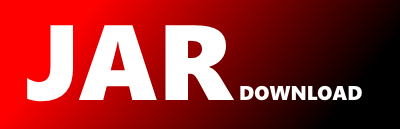
com.pulumi.azure.streamanalytics.kotlin.OutputServicebusTopic.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.streamanalytics.kotlin
import com.pulumi.azure.streamanalytics.kotlin.outputs.OutputServicebusTopicSerialization
import com.pulumi.azure.streamanalytics.kotlin.outputs.OutputServicebusTopicSerialization.Companion.toKotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
/**
* Builder for [OutputServicebusTopic].
*/
@PulumiTagMarker
public class OutputServicebusTopicResourceBuilder internal constructor() {
public var name: String? = null
public var args: OutputServicebusTopicArgs = OutputServicebusTopicArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend OutputServicebusTopicArgsBuilder.() -> Unit) {
val builder = OutputServicebusTopicArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): OutputServicebusTopic {
val builtJavaResource =
com.pulumi.azure.streamanalytics.OutputServicebusTopic(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return OutputServicebusTopic(builtJavaResource)
}
}
/**
* Manages a Stream Analytics Output to a ServiceBus Topic.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const exampleResourceGroup = new azure.core.ResourceGroup("example", {
* name: "rg-example",
* location: "West Europe",
* });
* const example = azure.streamanalytics.getJobOutput({
* name: "example-job",
* resourceGroupName: exampleResourceGroup.name,
* });
* const exampleNamespace = new azure.servicebus.Namespace("example", {
* name: "example-namespace",
* location: exampleResourceGroup.location,
* resourceGroupName: exampleResourceGroup.name,
* sku: "Standard",
* });
* const exampleTopic = new azure.servicebus.Topic("example", {
* name: "example-topic",
* namespaceId: exampleNamespace.id,
* enablePartitioning: true,
* });
* const exampleOutputServicebusTopic = new azure.streamanalytics.OutputServicebusTopic("example", {
* name: "service-bus-topic-output",
* streamAnalyticsJobName: example.apply(example => example.name),
* resourceGroupName: example.apply(example => example.resourceGroupName),
* topicName: exampleTopic.name,
* servicebusNamespace: exampleNamespace.name,
* sharedAccessPolicyKey: exampleNamespace.defaultPrimaryKey,
* sharedAccessPolicyName: "RootManageSharedAccessKey",
* propertyColumns: [
* "col1",
* "col2",
* ],
* serialization: {
* type: "Csv",
* format: "Array",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example_resource_group = azure.core.ResourceGroup("example",
* name="rg-example",
* location="West Europe")
* example = azure.streamanalytics.get_job_output(name="example-job",
* resource_group_name=example_resource_group.name)
* example_namespace = azure.servicebus.Namespace("example",
* name="example-namespace",
* location=example_resource_group.location,
* resource_group_name=example_resource_group.name,
* sku="Standard")
* example_topic = azure.servicebus.Topic("example",
* name="example-topic",
* namespace_id=example_namespace.id,
* enable_partitioning=True)
* example_output_servicebus_topic = azure.streamanalytics.OutputServicebusTopic("example",
* name="service-bus-topic-output",
* stream_analytics_job_name=example.name,
* resource_group_name=example.resource_group_name,
* topic_name=example_topic.name,
* servicebus_namespace=example_namespace.name,
* shared_access_policy_key=example_namespace.default_primary_key,
* shared_access_policy_name="RootManageSharedAccessKey",
* property_columns=[
* "col1",
* "col2",
* ],
* serialization={
* "type": "Csv",
* "format": "Array",
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var exampleResourceGroup = new Azure.Core.ResourceGroup("example", new()
* {
* Name = "rg-example",
* Location = "West Europe",
* });
* var example = Azure.StreamAnalytics.GetJob.Invoke(new()
* {
* Name = "example-job",
* ResourceGroupName = exampleResourceGroup.Name,
* });
* var exampleNamespace = new Azure.ServiceBus.Namespace("example", new()
* {
* Name = "example-namespace",
* Location = exampleResourceGroup.Location,
* ResourceGroupName = exampleResourceGroup.Name,
* Sku = "Standard",
* });
* var exampleTopic = new Azure.ServiceBus.Topic("example", new()
* {
* Name = "example-topic",
* NamespaceId = exampleNamespace.Id,
* EnablePartitioning = true,
* });
* var exampleOutputServicebusTopic = new Azure.StreamAnalytics.OutputServicebusTopic("example", new()
* {
* Name = "service-bus-topic-output",
* StreamAnalyticsJobName = example.Apply(getJobResult => getJobResult.Name),
* ResourceGroupName = example.Apply(getJobResult => getJobResult.ResourceGroupName),
* TopicName = exampleTopic.Name,
* ServicebusNamespace = exampleNamespace.Name,
* SharedAccessPolicyKey = exampleNamespace.DefaultPrimaryKey,
* SharedAccessPolicyName = "RootManageSharedAccessKey",
* PropertyColumns = new[]
* {
* "col1",
* "col2",
* },
* Serialization = new Azure.StreamAnalytics.Inputs.OutputServicebusTopicSerializationArgs
* {
* Type = "Csv",
* Format = "Array",
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/core"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/servicebus"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/streamanalytics"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* exampleResourceGroup, err := core.NewResourceGroup(ctx, "example", &core.ResourceGroupArgs{
* Name: pulumi.String("rg-example"),
* Location: pulumi.String("West Europe"),
* })
* if err != nil {
* return err
* }
* example := streamanalytics.LookupJobOutput(ctx, streamanalytics.GetJobOutputArgs{
* Name: pulumi.String("example-job"),
* ResourceGroupName: exampleResourceGroup.Name,
* }, nil)
* exampleNamespace, err := servicebus.NewNamespace(ctx, "example", &servicebus.NamespaceArgs{
* Name: pulumi.String("example-namespace"),
* Location: exampleResourceGroup.Location,
* ResourceGroupName: exampleResourceGroup.Name,
* Sku: pulumi.String("Standard"),
* })
* if err != nil {
* return err
* }
* exampleTopic, err := servicebus.NewTopic(ctx, "example", &servicebus.TopicArgs{
* Name: pulumi.String("example-topic"),
* NamespaceId: exampleNamespace.ID(),
* EnablePartitioning: pulumi.Bool(true),
* })
* if err != nil {
* return err
* }
* _, err = streamanalytics.NewOutputServicebusTopic(ctx, "example", &streamanalytics.OutputServicebusTopicArgs{
* Name: pulumi.String("service-bus-topic-output"),
* StreamAnalyticsJobName: pulumi.String(example.ApplyT(func(example streamanalytics.GetJobResult) (*string, error) {
* return &example.Name, nil
* }).(pulumi.StringPtrOutput)),
* ResourceGroupName: pulumi.String(example.ApplyT(func(example streamanalytics.GetJobResult) (*string, error) {
* return &example.ResourceGroupName, nil
* }).(pulumi.StringPtrOutput)),
* TopicName: exampleTopic.Name,
* ServicebusNamespace: exampleNamespace.Name,
* SharedAccessPolicyKey: exampleNamespace.DefaultPrimaryKey,
* SharedAccessPolicyName: pulumi.String("RootManageSharedAccessKey"),
* PropertyColumns: pulumi.StringArray{
* pulumi.String("col1"),
* pulumi.String("col2"),
* },
* Serialization: &streamanalytics.OutputServicebusTopicSerializationArgs{
* Type: pulumi.String("Csv"),
* Format: pulumi.String("Array"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.streamanalytics.StreamanalyticsFunctions;
* import com.pulumi.azure.streamanalytics.inputs.GetJobArgs;
* import com.pulumi.azure.servicebus.Namespace;
* import com.pulumi.azure.servicebus.NamespaceArgs;
* import com.pulumi.azure.servicebus.Topic;
* import com.pulumi.azure.servicebus.TopicArgs;
* import com.pulumi.azure.streamanalytics.OutputServicebusTopic;
* import com.pulumi.azure.streamanalytics.OutputServicebusTopicArgs;
* import com.pulumi.azure.streamanalytics.inputs.OutputServicebusTopicSerializationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var exampleResourceGroup = new ResourceGroup("exampleResourceGroup", ResourceGroupArgs.builder()
* .name("rg-example")
* .location("West Europe")
* .build());
* final var example = StreamanalyticsFunctions.getJob(GetJobArgs.builder()
* .name("example-job")
* .resourceGroupName(exampleResourceGroup.name())
* .build());
* var exampleNamespace = new Namespace("exampleNamespace", NamespaceArgs.builder()
* .name("example-namespace")
* .location(exampleResourceGroup.location())
* .resourceGroupName(exampleResourceGroup.name())
* .sku("Standard")
* .build());
* var exampleTopic = new Topic("exampleTopic", TopicArgs.builder()
* .name("example-topic")
* .namespaceId(exampleNamespace.id())
* .enablePartitioning(true)
* .build());
* var exampleOutputServicebusTopic = new OutputServicebusTopic("exampleOutputServicebusTopic", OutputServicebusTopicArgs.builder()
* .name("service-bus-topic-output")
* .streamAnalyticsJobName(example.applyValue(getJobResult -> getJobResult).applyValue(example -> example.applyValue(getJobResult -> getJobResult.name())))
* .resourceGroupName(example.applyValue(getJobResult -> getJobResult).applyValue(example -> example.applyValue(getJobResult -> getJobResult.resourceGroupName())))
* .topicName(exampleTopic.name())
* .servicebusNamespace(exampleNamespace.name())
* .sharedAccessPolicyKey(exampleNamespace.defaultPrimaryKey())
* .sharedAccessPolicyName("RootManageSharedAccessKey")
* .propertyColumns(
* "col1",
* "col2")
* .serialization(OutputServicebusTopicSerializationArgs.builder()
* .type("Csv")
* .format("Array")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* exampleResourceGroup:
* type: azure:core:ResourceGroup
* name: example
* properties:
* name: rg-example
* location: West Europe
* exampleNamespace:
* type: azure:servicebus:Namespace
* name: example
* properties:
* name: example-namespace
* location: ${exampleResourceGroup.location}
* resourceGroupName: ${exampleResourceGroup.name}
* sku: Standard
* exampleTopic:
* type: azure:servicebus:Topic
* name: example
* properties:
* name: example-topic
* namespaceId: ${exampleNamespace.id}
* enablePartitioning: true
* exampleOutputServicebusTopic:
* type: azure:streamanalytics:OutputServicebusTopic
* name: example
* properties:
* name: service-bus-topic-output
* streamAnalyticsJobName: ${example.name}
* resourceGroupName: ${example.resourceGroupName}
* topicName: ${exampleTopic.name}
* servicebusNamespace: ${exampleNamespace.name}
* sharedAccessPolicyKey: ${exampleNamespace.defaultPrimaryKey}
* sharedAccessPolicyName: RootManageSharedAccessKey
* propertyColumns:
* - col1
* - col2
* serialization:
* type: Csv
* format: Array
* variables:
* example:
* fn::invoke:
* Function: azure:streamanalytics:getJob
* Arguments:
* name: example-job
* resourceGroupName: ${exampleResourceGroup.name}
* ```
*
* ## Import
* Stream Analytics Output ServiceBus Topic's can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:streamanalytics/outputServicebusTopic:OutputServicebusTopic example /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/group1/providers/Microsoft.StreamAnalytics/streamingJobs/job1/outputs/output1
* ```
*/
public class OutputServicebusTopic internal constructor(
override val javaResource: com.pulumi.azure.streamanalytics.OutputServicebusTopic,
) : KotlinCustomResource(javaResource, OutputServicebusTopicMapper) {
/**
* The authentication mode for the Stream Output. Possible values are `Msi` and `ConnectionString`. Defaults to `ConnectionString`.
*/
public val authenticationMode: Output?
get() = javaResource.authenticationMode().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The name of the Stream Output. Changing this forces a new resource to be created.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* A list of property columns to add to the Service Bus Topic output.
*/
public val propertyColumns: Output>?
get() = javaResource.propertyColumns().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
/**
* The name of the Resource Group where the Stream Analytics Job exists. Changing this forces a new resource to be created.
*/
public val resourceGroupName: Output
get() = javaResource.resourceGroupName().applyValue({ args0 -> args0 })
/**
* A `serialization` block as defined below.
*/
public val serialization: Output
get() = javaResource.serialization().applyValue({ args0 ->
args0.let({ args0 ->
toKotlin(args0)
})
})
/**
* The namespace that is associated with the desired Event Hub, Service Bus Topic, Service Bus Topic, etc.
*/
public val servicebusNamespace: Output
get() = javaResource.servicebusNamespace().applyValue({ args0 -> args0 })
/**
* The shared access policy key for the specified shared access policy. Required if `authentication_mode` is `ConnectionString`.
*/
public val sharedAccessPolicyKey: Output?
get() = javaResource.sharedAccessPolicyKey().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The shared access policy name for the Event Hub, Service Bus Queue, Service Bus Topic, etc. Required if `authentication_mode` is `ConnectionString`.
*/
public val sharedAccessPolicyName: Output?
get() = javaResource.sharedAccessPolicyName().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The name of the Stream Analytics Job. Changing this forces a new resource to be created.
*/
public val streamAnalyticsJobName: Output
get() = javaResource.streamAnalyticsJobName().applyValue({ args0 -> args0 })
/**
* A key-value pair of system property columns that will be attached to the outgoing messages for the Service Bus Topic Output.
* > **NOTE:** The acceptable keys are `ContentType`, `CorrelationId`, `Label`, `MessageId`, `PartitionKey`, `ReplyTo`, `ReplyToSessionId`, `ScheduledEnqueueTimeUtc`, `SessionId`, `TimeToLive` and `To`.
*/
public val systemPropertyColumns: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy