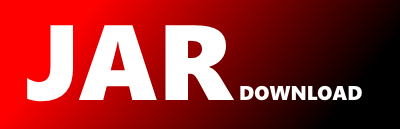
com.pulumi.azure.streamanalytics.kotlin.StreamInputEventHubArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.streamanalytics.kotlin
import com.pulumi.azure.streamanalytics.StreamInputEventHubArgs.builder
import com.pulumi.azure.streamanalytics.kotlin.inputs.StreamInputEventHubSerializationArgs
import com.pulumi.azure.streamanalytics.kotlin.inputs.StreamInputEventHubSerializationArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* > **Note:** This resource creates a Stream Input of type `Microsoft.ServiceBus/EventHub`, to create a Stream Input of type `Microsoft.EventHub/EventHub` please use the resource azurerm_stream_analytics_stream_input_eventhub_v2.
* Manages a Stream Analytics Stream Input EventHub.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const exampleResourceGroup = new azure.core.ResourceGroup("example", {
* name: "example-resources",
* location: "West Europe",
* });
* const example = azure.streamanalytics.getJobOutput({
* name: "example-job",
* resourceGroupName: exampleResourceGroup.name,
* });
* const exampleEventHubNamespace = new azure.eventhub.EventHubNamespace("example", {
* name: "example-namespace",
* location: exampleResourceGroup.location,
* resourceGroupName: exampleResourceGroup.name,
* sku: "Standard",
* capacity: 1,
* });
* const exampleEventHub = new azure.eventhub.EventHub("example", {
* name: "example-eventhub",
* namespaceName: exampleEventHubNamespace.name,
* resourceGroupName: exampleResourceGroup.name,
* partitionCount: 2,
* messageRetention: 1,
* });
* const exampleConsumerGroup = new azure.eventhub.ConsumerGroup("example", {
* name: "example-consumergroup",
* namespaceName: exampleEventHubNamespace.name,
* eventhubName: exampleEventHub.name,
* resourceGroupName: exampleResourceGroup.name,
* });
* const exampleStreamInputEventHub = new azure.streamanalytics.StreamInputEventHub("example", {
* name: "eventhub-stream-input",
* streamAnalyticsJobName: example.apply(example => example.name),
* resourceGroupName: example.apply(example => example.resourceGroupName),
* eventhubConsumerGroupName: exampleConsumerGroup.name,
* eventhubName: exampleEventHub.name,
* servicebusNamespace: exampleEventHubNamespace.name,
* sharedAccessPolicyKey: exampleEventHubNamespace.defaultPrimaryKey,
* sharedAccessPolicyName: "RootManageSharedAccessKey",
* serialization: {
* type: "Json",
* encoding: "UTF8",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example_resource_group = azure.core.ResourceGroup("example",
* name="example-resources",
* location="West Europe")
* example = azure.streamanalytics.get_job_output(name="example-job",
* resource_group_name=example_resource_group.name)
* example_event_hub_namespace = azure.eventhub.EventHubNamespace("example",
* name="example-namespace",
* location=example_resource_group.location,
* resource_group_name=example_resource_group.name,
* sku="Standard",
* capacity=1)
* example_event_hub = azure.eventhub.EventHub("example",
* name="example-eventhub",
* namespace_name=example_event_hub_namespace.name,
* resource_group_name=example_resource_group.name,
* partition_count=2,
* message_retention=1)
* example_consumer_group = azure.eventhub.ConsumerGroup("example",
* name="example-consumergroup",
* namespace_name=example_event_hub_namespace.name,
* eventhub_name=example_event_hub.name,
* resource_group_name=example_resource_group.name)
* example_stream_input_event_hub = azure.streamanalytics.StreamInputEventHub("example",
* name="eventhub-stream-input",
* stream_analytics_job_name=example.name,
* resource_group_name=example.resource_group_name,
* eventhub_consumer_group_name=example_consumer_group.name,
* eventhub_name=example_event_hub.name,
* servicebus_namespace=example_event_hub_namespace.name,
* shared_access_policy_key=example_event_hub_namespace.default_primary_key,
* shared_access_policy_name="RootManageSharedAccessKey",
* serialization={
* "type": "Json",
* "encoding": "UTF8",
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var exampleResourceGroup = new Azure.Core.ResourceGroup("example", new()
* {
* Name = "example-resources",
* Location = "West Europe",
* });
* var example = Azure.StreamAnalytics.GetJob.Invoke(new()
* {
* Name = "example-job",
* ResourceGroupName = exampleResourceGroup.Name,
* });
* var exampleEventHubNamespace = new Azure.EventHub.EventHubNamespace("example", new()
* {
* Name = "example-namespace",
* Location = exampleResourceGroup.Location,
* ResourceGroupName = exampleResourceGroup.Name,
* Sku = "Standard",
* Capacity = 1,
* });
* var exampleEventHub = new Azure.EventHub.EventHub("example", new()
* {
* Name = "example-eventhub",
* NamespaceName = exampleEventHubNamespace.Name,
* ResourceGroupName = exampleResourceGroup.Name,
* PartitionCount = 2,
* MessageRetention = 1,
* });
* var exampleConsumerGroup = new Azure.EventHub.ConsumerGroup("example", new()
* {
* Name = "example-consumergroup",
* NamespaceName = exampleEventHubNamespace.Name,
* EventhubName = exampleEventHub.Name,
* ResourceGroupName = exampleResourceGroup.Name,
* });
* var exampleStreamInputEventHub = new Azure.StreamAnalytics.StreamInputEventHub("example", new()
* {
* Name = "eventhub-stream-input",
* StreamAnalyticsJobName = example.Apply(getJobResult => getJobResult.Name),
* ResourceGroupName = example.Apply(getJobResult => getJobResult.ResourceGroupName),
* EventhubConsumerGroupName = exampleConsumerGroup.Name,
* EventhubName = exampleEventHub.Name,
* ServicebusNamespace = exampleEventHubNamespace.Name,
* SharedAccessPolicyKey = exampleEventHubNamespace.DefaultPrimaryKey,
* SharedAccessPolicyName = "RootManageSharedAccessKey",
* Serialization = new Azure.StreamAnalytics.Inputs.StreamInputEventHubSerializationArgs
* {
* Type = "Json",
* Encoding = "UTF8",
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/core"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/eventhub"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/streamanalytics"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* exampleResourceGroup, err := core.NewResourceGroup(ctx, "example", &core.ResourceGroupArgs{
* Name: pulumi.String("example-resources"),
* Location: pulumi.String("West Europe"),
* })
* if err != nil {
* return err
* }
* example := streamanalytics.LookupJobOutput(ctx, streamanalytics.GetJobOutputArgs{
* Name: pulumi.String("example-job"),
* ResourceGroupName: exampleResourceGroup.Name,
* }, nil)
* exampleEventHubNamespace, err := eventhub.NewEventHubNamespace(ctx, "example", &eventhub.EventHubNamespaceArgs{
* Name: pulumi.String("example-namespace"),
* Location: exampleResourceGroup.Location,
* ResourceGroupName: exampleResourceGroup.Name,
* Sku: pulumi.String("Standard"),
* Capacity: pulumi.Int(1),
* })
* if err != nil {
* return err
* }
* exampleEventHub, err := eventhub.NewEventHub(ctx, "example", &eventhub.EventHubArgs{
* Name: pulumi.String("example-eventhub"),
* NamespaceName: exampleEventHubNamespace.Name,
* ResourceGroupName: exampleResourceGroup.Name,
* PartitionCount: pulumi.Int(2),
* MessageRetention: pulumi.Int(1),
* })
* if err != nil {
* return err
* }
* exampleConsumerGroup, err := eventhub.NewConsumerGroup(ctx, "example", &eventhub.ConsumerGroupArgs{
* Name: pulumi.String("example-consumergroup"),
* NamespaceName: exampleEventHubNamespace.Name,
* EventhubName: exampleEventHub.Name,
* ResourceGroupName: exampleResourceGroup.Name,
* })
* if err != nil {
* return err
* }
* _, err = streamanalytics.NewStreamInputEventHub(ctx, "example", &streamanalytics.StreamInputEventHubArgs{
* Name: pulumi.String("eventhub-stream-input"),
* StreamAnalyticsJobName: pulumi.String(example.ApplyT(func(example streamanalytics.GetJobResult) (*string, error) {
* return &example.Name, nil
* }).(pulumi.StringPtrOutput)),
* ResourceGroupName: pulumi.String(example.ApplyT(func(example streamanalytics.GetJobResult) (*string, error) {
* return &example.ResourceGroupName, nil
* }).(pulumi.StringPtrOutput)),
* EventhubConsumerGroupName: exampleConsumerGroup.Name,
* EventhubName: exampleEventHub.Name,
* ServicebusNamespace: exampleEventHubNamespace.Name,
* SharedAccessPolicyKey: exampleEventHubNamespace.DefaultPrimaryKey,
* SharedAccessPolicyName: pulumi.String("RootManageSharedAccessKey"),
* Serialization: &streamanalytics.StreamInputEventHubSerializationArgs{
* Type: pulumi.String("Json"),
* Encoding: pulumi.String("UTF8"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.streamanalytics.StreamanalyticsFunctions;
* import com.pulumi.azure.streamanalytics.inputs.GetJobArgs;
* import com.pulumi.azure.eventhub.EventHubNamespace;
* import com.pulumi.azure.eventhub.EventHubNamespaceArgs;
* import com.pulumi.azure.eventhub.EventHub;
* import com.pulumi.azure.eventhub.EventHubArgs;
* import com.pulumi.azure.eventhub.ConsumerGroup;
* import com.pulumi.azure.eventhub.ConsumerGroupArgs;
* import com.pulumi.azure.streamanalytics.StreamInputEventHub;
* import com.pulumi.azure.streamanalytics.StreamInputEventHubArgs;
* import com.pulumi.azure.streamanalytics.inputs.StreamInputEventHubSerializationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var exampleResourceGroup = new ResourceGroup("exampleResourceGroup", ResourceGroupArgs.builder()
* .name("example-resources")
* .location("West Europe")
* .build());
* final var example = StreamanalyticsFunctions.getJob(GetJobArgs.builder()
* .name("example-job")
* .resourceGroupName(exampleResourceGroup.name())
* .build());
* var exampleEventHubNamespace = new EventHubNamespace("exampleEventHubNamespace", EventHubNamespaceArgs.builder()
* .name("example-namespace")
* .location(exampleResourceGroup.location())
* .resourceGroupName(exampleResourceGroup.name())
* .sku("Standard")
* .capacity(1)
* .build());
* var exampleEventHub = new EventHub("exampleEventHub", EventHubArgs.builder()
* .name("example-eventhub")
* .namespaceName(exampleEventHubNamespace.name())
* .resourceGroupName(exampleResourceGroup.name())
* .partitionCount(2)
* .messageRetention(1)
* .build());
* var exampleConsumerGroup = new ConsumerGroup("exampleConsumerGroup", ConsumerGroupArgs.builder()
* .name("example-consumergroup")
* .namespaceName(exampleEventHubNamespace.name())
* .eventhubName(exampleEventHub.name())
* .resourceGroupName(exampleResourceGroup.name())
* .build());
* var exampleStreamInputEventHub = new StreamInputEventHub("exampleStreamInputEventHub", StreamInputEventHubArgs.builder()
* .name("eventhub-stream-input")
* .streamAnalyticsJobName(example.applyValue(getJobResult -> getJobResult).applyValue(example -> example.applyValue(getJobResult -> getJobResult.name())))
* .resourceGroupName(example.applyValue(getJobResult -> getJobResult).applyValue(example -> example.applyValue(getJobResult -> getJobResult.resourceGroupName())))
* .eventhubConsumerGroupName(exampleConsumerGroup.name())
* .eventhubName(exampleEventHub.name())
* .servicebusNamespace(exampleEventHubNamespace.name())
* .sharedAccessPolicyKey(exampleEventHubNamespace.defaultPrimaryKey())
* .sharedAccessPolicyName("RootManageSharedAccessKey")
* .serialization(StreamInputEventHubSerializationArgs.builder()
* .type("Json")
* .encoding("UTF8")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* exampleResourceGroup:
* type: azure:core:ResourceGroup
* name: example
* properties:
* name: example-resources
* location: West Europe
* exampleEventHubNamespace:
* type: azure:eventhub:EventHubNamespace
* name: example
* properties:
* name: example-namespace
* location: ${exampleResourceGroup.location}
* resourceGroupName: ${exampleResourceGroup.name}
* sku: Standard
* capacity: 1
* exampleEventHub:
* type: azure:eventhub:EventHub
* name: example
* properties:
* name: example-eventhub
* namespaceName: ${exampleEventHubNamespace.name}
* resourceGroupName: ${exampleResourceGroup.name}
* partitionCount: 2
* messageRetention: 1
* exampleConsumerGroup:
* type: azure:eventhub:ConsumerGroup
* name: example
* properties:
* name: example-consumergroup
* namespaceName: ${exampleEventHubNamespace.name}
* eventhubName: ${exampleEventHub.name}
* resourceGroupName: ${exampleResourceGroup.name}
* exampleStreamInputEventHub:
* type: azure:streamanalytics:StreamInputEventHub
* name: example
* properties:
* name: eventhub-stream-input
* streamAnalyticsJobName: ${example.name}
* resourceGroupName: ${example.resourceGroupName}
* eventhubConsumerGroupName: ${exampleConsumerGroup.name}
* eventhubName: ${exampleEventHub.name}
* servicebusNamespace: ${exampleEventHubNamespace.name}
* sharedAccessPolicyKey: ${exampleEventHubNamespace.defaultPrimaryKey}
* sharedAccessPolicyName: RootManageSharedAccessKey
* serialization:
* type: Json
* encoding: UTF8
* variables:
* example:
* fn::invoke:
* Function: azure:streamanalytics:getJob
* Arguments:
* name: example-job
* resourceGroupName: ${exampleResourceGroup.name}
* ```
*
* ## Import
* Stream Analytics Stream Input EventHub's can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:streamanalytics/streamInputEventHub:StreamInputEventHub example /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/group1/providers/Microsoft.StreamAnalytics/streamingJobs/job1/inputs/input1
* ```
* @property authenticationMode The authentication mode for the Stream Output. Possible values are `Msi` and `ConnectionString`. Defaults to `ConnectionString`.
* @property eventhubConsumerGroupName The name of an Event Hub Consumer Group that should be used to read events from the Event Hub. Specifying distinct consumer group names for multiple inputs allows each of those inputs to receive the same events from the Event Hub. If not set the input will use the Event Hub's default consumer group.
* @property eventhubName The name of the Event Hub.
* @property name The name of the Stream Input EventHub. Changing this forces a new resource to be created.
* @property partitionKey The property the input Event Hub has been partitioned by.
* @property resourceGroupName The name of the Resource Group where the Stream Analytics Job exists. Changing this forces a new resource to be created.
* @property serialization A `serialization` block as defined below.
* @property servicebusNamespace The namespace that is associated with the desired Event Hub, Service Bus Queue, Service Bus Topic, etc.
* @property sharedAccessPolicyKey The shared access policy key for the specified shared access policy.
* @property sharedAccessPolicyName The shared access policy name for the Event Hub, Service Bus Queue, Service Bus Topic, etc.
* @property streamAnalyticsJobName The name of the Stream Analytics Job. Changing this forces a new resource to be created.
*/
public data class StreamInputEventHubArgs(
public val authenticationMode: Output? = null,
public val eventhubConsumerGroupName: Output? = null,
public val eventhubName: Output? = null,
public val name: Output? = null,
public val partitionKey: Output? = null,
public val resourceGroupName: Output? = null,
public val serialization: Output? = null,
public val servicebusNamespace: Output? = null,
public val sharedAccessPolicyKey: Output? = null,
public val sharedAccessPolicyName: Output? = null,
public val streamAnalyticsJobName: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.streamanalytics.StreamInputEventHubArgs =
com.pulumi.azure.streamanalytics.StreamInputEventHubArgs.builder()
.authenticationMode(authenticationMode?.applyValue({ args0 -> args0 }))
.eventhubConsumerGroupName(eventhubConsumerGroupName?.applyValue({ args0 -> args0 }))
.eventhubName(eventhubName?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.partitionKey(partitionKey?.applyValue({ args0 -> args0 }))
.resourceGroupName(resourceGroupName?.applyValue({ args0 -> args0 }))
.serialization(serialization?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.servicebusNamespace(servicebusNamespace?.applyValue({ args0 -> args0 }))
.sharedAccessPolicyKey(sharedAccessPolicyKey?.applyValue({ args0 -> args0 }))
.sharedAccessPolicyName(sharedAccessPolicyName?.applyValue({ args0 -> args0 }))
.streamAnalyticsJobName(streamAnalyticsJobName?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [StreamInputEventHubArgs].
*/
@PulumiTagMarker
public class StreamInputEventHubArgsBuilder internal constructor() {
private var authenticationMode: Output? = null
private var eventhubConsumerGroupName: Output? = null
private var eventhubName: Output? = null
private var name: Output? = null
private var partitionKey: Output? = null
private var resourceGroupName: Output? = null
private var serialization: Output? = null
private var servicebusNamespace: Output? = null
private var sharedAccessPolicyKey: Output? = null
private var sharedAccessPolicyName: Output? = null
private var streamAnalyticsJobName: Output? = null
/**
* @param value The authentication mode for the Stream Output. Possible values are `Msi` and `ConnectionString`. Defaults to `ConnectionString`.
*/
@JvmName("dxlgmqhnsubsptqx")
public suspend fun authenticationMode(`value`: Output) {
this.authenticationMode = value
}
/**
* @param value The name of an Event Hub Consumer Group that should be used to read events from the Event Hub. Specifying distinct consumer group names for multiple inputs allows each of those inputs to receive the same events from the Event Hub. If not set the input will use the Event Hub's default consumer group.
*/
@JvmName("cylvhdjarskxfxtb")
public suspend fun eventhubConsumerGroupName(`value`: Output) {
this.eventhubConsumerGroupName = value
}
/**
* @param value The name of the Event Hub.
*/
@JvmName("mkojlglxiflddxeo")
public suspend fun eventhubName(`value`: Output) {
this.eventhubName = value
}
/**
* @param value The name of the Stream Input EventHub. Changing this forces a new resource to be created.
*/
@JvmName("dumcgwhkgybvuaal")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The property the input Event Hub has been partitioned by.
*/
@JvmName("uooqhyurcvfqthle")
public suspend fun partitionKey(`value`: Output) {
this.partitionKey = value
}
/**
* @param value The name of the Resource Group where the Stream Analytics Job exists. Changing this forces a new resource to be created.
*/
@JvmName("gswausibcqeytnjy")
public suspend fun resourceGroupName(`value`: Output) {
this.resourceGroupName = value
}
/**
* @param value A `serialization` block as defined below.
*/
@JvmName("ldjpculnxbqcifvr")
public suspend fun serialization(`value`: Output) {
this.serialization = value
}
/**
* @param value The namespace that is associated with the desired Event Hub, Service Bus Queue, Service Bus Topic, etc.
*/
@JvmName("fwljnskjwcceoreu")
public suspend fun servicebusNamespace(`value`: Output) {
this.servicebusNamespace = value
}
/**
* @param value The shared access policy key for the specified shared access policy.
*/
@JvmName("rfljghuvpklqbjwk")
public suspend fun sharedAccessPolicyKey(`value`: Output) {
this.sharedAccessPolicyKey = value
}
/**
* @param value The shared access policy name for the Event Hub, Service Bus Queue, Service Bus Topic, etc.
*/
@JvmName("ohpacotbipeuaffa")
public suspend fun sharedAccessPolicyName(`value`: Output) {
this.sharedAccessPolicyName = value
}
/**
* @param value The name of the Stream Analytics Job. Changing this forces a new resource to be created.
*/
@JvmName("llumqcgrkcehvotb")
public suspend fun streamAnalyticsJobName(`value`: Output) {
this.streamAnalyticsJobName = value
}
/**
* @param value The authentication mode for the Stream Output. Possible values are `Msi` and `ConnectionString`. Defaults to `ConnectionString`.
*/
@JvmName("voxroyufpbqoeljo")
public suspend fun authenticationMode(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.authenticationMode = mapped
}
/**
* @param value The name of an Event Hub Consumer Group that should be used to read events from the Event Hub. Specifying distinct consumer group names for multiple inputs allows each of those inputs to receive the same events from the Event Hub. If not set the input will use the Event Hub's default consumer group.
*/
@JvmName("lkbobwtwepifvmcf")
public suspend fun eventhubConsumerGroupName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.eventhubConsumerGroupName = mapped
}
/**
* @param value The name of the Event Hub.
*/
@JvmName("vuvigbgmbqngtbhf")
public suspend fun eventhubName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.eventhubName = mapped
}
/**
* @param value The name of the Stream Input EventHub. Changing this forces a new resource to be created.
*/
@JvmName("aydjegueudsisapo")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value The property the input Event Hub has been partitioned by.
*/
@JvmName("qhwmhfidyggdrhjx")
public suspend fun partitionKey(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.partitionKey = mapped
}
/**
* @param value The name of the Resource Group where the Stream Analytics Job exists. Changing this forces a new resource to be created.
*/
@JvmName("gidwplftvqxxqgkd")
public suspend fun resourceGroupName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resourceGroupName = mapped
}
/**
* @param value A `serialization` block as defined below.
*/
@JvmName("lsfgykbdrtcnklyi")
public suspend fun serialization(`value`: StreamInputEventHubSerializationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.serialization = mapped
}
/**
* @param argument A `serialization` block as defined below.
*/
@JvmName("hlpysuwolwofjkoy")
public suspend fun serialization(argument: suspend StreamInputEventHubSerializationArgsBuilder.() -> Unit) {
val toBeMapped = StreamInputEventHubSerializationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.serialization = mapped
}
/**
* @param value The namespace that is associated with the desired Event Hub, Service Bus Queue, Service Bus Topic, etc.
*/
@JvmName("eaioqsohshihlvlu")
public suspend fun servicebusNamespace(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.servicebusNamespace = mapped
}
/**
* @param value The shared access policy key for the specified shared access policy.
*/
@JvmName("mcxxmavmceloryig")
public suspend fun sharedAccessPolicyKey(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sharedAccessPolicyKey = mapped
}
/**
* @param value The shared access policy name for the Event Hub, Service Bus Queue, Service Bus Topic, etc.
*/
@JvmName("owefnxkjsaenvuaj")
public suspend fun sharedAccessPolicyName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sharedAccessPolicyName = mapped
}
/**
* @param value The name of the Stream Analytics Job. Changing this forces a new resource to be created.
*/
@JvmName("atrkucmqalnueesg")
public suspend fun streamAnalyticsJobName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.streamAnalyticsJobName = mapped
}
internal fun build(): StreamInputEventHubArgs = StreamInputEventHubArgs(
authenticationMode = authenticationMode,
eventhubConsumerGroupName = eventhubConsumerGroupName,
eventhubName = eventhubName,
name = name,
partitionKey = partitionKey,
resourceGroupName = resourceGroupName,
serialization = serialization,
servicebusNamespace = servicebusNamespace,
sharedAccessPolicyKey = sharedAccessPolicyKey,
sharedAccessPolicyName = sharedAccessPolicyName,
streamAnalyticsJobName = streamAnalyticsJobName,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy