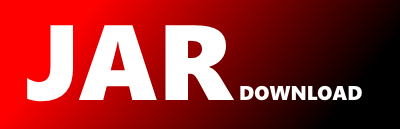
com.pulumi.azure.workloadssap.kotlin.inputs.ThreeTierVirtualInstanceThreeTierConfigurationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.workloadssap.kotlin.inputs
import com.pulumi.azure.workloadssap.inputs.ThreeTierVirtualInstanceThreeTierConfigurationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property appResourceGroupName
* @property applicationServerConfiguration An `application_server_configuration` block as defined below. Changing this forces a new resource to be created.
* @property centralServerConfiguration A `central_server_configuration` block as defined below. Changing this forces a new resource to be created.
* @property databaseServerConfiguration A `database_server_configuration` block as defined below. Changing this forces a new resource to be created.
* @property highAvailabilityType The high availability type for the three tier configuration. Possible values are `AvailabilitySet` and `AvailabilityZone`. Changing this forces a new resource to be created.
* @property resourceNames A `resource_names` block as defined below. Changing this forces a new resource to be created.
* @property secondaryIpEnabled Specifies whether a secondary IP address should be added to the network interface on all VMs of the SAP system being deployed. Defaults to `false`. Changing this forces a new resource to be created.
* @property transportCreateAndMount A `transport_create_and_mount` block as defined below. Changing this forces a new resource to be created.
* > **Note:** The file share configuration uses `skip` by default when `transport_create_and_mount` isn't set.
* > **Note:** Due to [a bug in the Azure API](https://github.com/Azure/azure-rest-api-specs/issues/25209) where the Storage File Share Id is not defined correctly, it is not currently possible to support using Transport Mount.
*/
public data class ThreeTierVirtualInstanceThreeTierConfigurationArgs(
public val appResourceGroupName: Output,
public val applicationServerConfiguration: Output,
public val centralServerConfiguration: Output,
public val databaseServerConfiguration: Output,
public val highAvailabilityType: Output? = null,
public val resourceNames: Output? =
null,
public val secondaryIpEnabled: Output? = null,
public val transportCreateAndMount: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.azure.workloadssap.inputs.ThreeTierVirtualInstanceThreeTierConfigurationArgs =
com.pulumi.azure.workloadssap.inputs.ThreeTierVirtualInstanceThreeTierConfigurationArgs.builder()
.appResourceGroupName(appResourceGroupName.applyValue({ args0 -> args0 }))
.applicationServerConfiguration(
applicationServerConfiguration.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.centralServerConfiguration(
centralServerConfiguration.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.databaseServerConfiguration(
databaseServerConfiguration.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.highAvailabilityType(highAvailabilityType?.applyValue({ args0 -> args0 }))
.resourceNames(resourceNames?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.secondaryIpEnabled(secondaryIpEnabled?.applyValue({ args0 -> args0 }))
.transportCreateAndMount(
transportCreateAndMount?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [ThreeTierVirtualInstanceThreeTierConfigurationArgs].
*/
@PulumiTagMarker
public class ThreeTierVirtualInstanceThreeTierConfigurationArgsBuilder internal constructor() {
private var appResourceGroupName: Output? = null
private var applicationServerConfiguration:
Output? =
null
private var centralServerConfiguration:
Output? = null
private var databaseServerConfiguration:
Output? = null
private var highAvailabilityType: Output? = null
private var resourceNames:
Output? = null
private var secondaryIpEnabled: Output? = null
private var transportCreateAndMount:
Output? = null
/**
* @param value
*/
@JvmName("abeiyqussikmjbev")
public suspend fun appResourceGroupName(`value`: Output) {
this.appResourceGroupName = value
}
/**
* @param value An `application_server_configuration` block as defined below. Changing this forces a new resource to be created.
*/
@JvmName("orjylyxwtdgkcqnj")
public suspend fun applicationServerConfiguration(`value`: Output) {
this.applicationServerConfiguration = value
}
/**
* @param value A `central_server_configuration` block as defined below. Changing this forces a new resource to be created.
*/
@JvmName("ilbbmbwoqtogliyb")
public suspend fun centralServerConfiguration(`value`: Output) {
this.centralServerConfiguration = value
}
/**
* @param value A `database_server_configuration` block as defined below. Changing this forces a new resource to be created.
*/
@JvmName("ctydqunexremuluk")
public suspend fun databaseServerConfiguration(`value`: Output) {
this.databaseServerConfiguration = value
}
/**
* @param value The high availability type for the three tier configuration. Possible values are `AvailabilitySet` and `AvailabilityZone`. Changing this forces a new resource to be created.
*/
@JvmName("ouhkrflssuykgtad")
public suspend fun highAvailabilityType(`value`: Output) {
this.highAvailabilityType = value
}
/**
* @param value A `resource_names` block as defined below. Changing this forces a new resource to be created.
*/
@JvmName("murcoamiccbnenyp")
public suspend fun resourceNames(`value`: Output) {
this.resourceNames = value
}
/**
* @param value Specifies whether a secondary IP address should be added to the network interface on all VMs of the SAP system being deployed. Defaults to `false`. Changing this forces a new resource to be created.
*/
@JvmName("buwevbcjymcxbpmq")
public suspend fun secondaryIpEnabled(`value`: Output) {
this.secondaryIpEnabled = value
}
/**
* @param value A `transport_create_and_mount` block as defined below. Changing this forces a new resource to be created.
* > **Note:** The file share configuration uses `skip` by default when `transport_create_and_mount` isn't set.
* > **Note:** Due to [a bug in the Azure API](https://github.com/Azure/azure-rest-api-specs/issues/25209) where the Storage File Share Id is not defined correctly, it is not currently possible to support using Transport Mount.
*/
@JvmName("kbisqkoogkguwirn")
public suspend fun transportCreateAndMount(`value`: Output) {
this.transportCreateAndMount = value
}
/**
* @param value
*/
@JvmName("fqibboypoeplwpac")
public suspend fun appResourceGroupName(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.appResourceGroupName = mapped
}
/**
* @param value An `application_server_configuration` block as defined below. Changing this forces a new resource to be created.
*/
@JvmName("jkgkpgqxidgcnloa")
public suspend fun applicationServerConfiguration(`value`: ThreeTierVirtualInstanceThreeTierConfigurationApplicationServerConfigurationArgs) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.applicationServerConfiguration = mapped
}
/**
* @param argument An `application_server_configuration` block as defined below. Changing this forces a new resource to be created.
*/
@JvmName("mubxgraufqewkrlf")
public suspend fun applicationServerConfiguration(argument: suspend ThreeTierVirtualInstanceThreeTierConfigurationApplicationServerConfigurationArgsBuilder.() -> Unit) {
val toBeMapped =
ThreeTierVirtualInstanceThreeTierConfigurationApplicationServerConfigurationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.applicationServerConfiguration = mapped
}
/**
* @param value A `central_server_configuration` block as defined below. Changing this forces a new resource to be created.
*/
@JvmName("ytjvplrxbmvfvgtq")
public suspend fun centralServerConfiguration(`value`: ThreeTierVirtualInstanceThreeTierConfigurationCentralServerConfigurationArgs) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.centralServerConfiguration = mapped
}
/**
* @param argument A `central_server_configuration` block as defined below. Changing this forces a new resource to be created.
*/
@JvmName("xbxynjhydunibyfk")
public suspend fun centralServerConfiguration(argument: suspend ThreeTierVirtualInstanceThreeTierConfigurationCentralServerConfigurationArgsBuilder.() -> Unit) {
val toBeMapped =
ThreeTierVirtualInstanceThreeTierConfigurationCentralServerConfigurationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.centralServerConfiguration = mapped
}
/**
* @param value A `database_server_configuration` block as defined below. Changing this forces a new resource to be created.
*/
@JvmName("ksukhocpbobrllmo")
public suspend fun databaseServerConfiguration(`value`: ThreeTierVirtualInstanceThreeTierConfigurationDatabaseServerConfigurationArgs) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.databaseServerConfiguration = mapped
}
/**
* @param argument A `database_server_configuration` block as defined below. Changing this forces a new resource to be created.
*/
@JvmName("poppifvxsutxkfhq")
public suspend fun databaseServerConfiguration(argument: suspend ThreeTierVirtualInstanceThreeTierConfigurationDatabaseServerConfigurationArgsBuilder.() -> Unit) {
val toBeMapped =
ThreeTierVirtualInstanceThreeTierConfigurationDatabaseServerConfigurationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.databaseServerConfiguration = mapped
}
/**
* @param value The high availability type for the three tier configuration. Possible values are `AvailabilitySet` and `AvailabilityZone`. Changing this forces a new resource to be created.
*/
@JvmName("qiyntowvmryjwjjc")
public suspend fun highAvailabilityType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.highAvailabilityType = mapped
}
/**
* @param value A `resource_names` block as defined below. Changing this forces a new resource to be created.
*/
@JvmName("hxnjhxapmauusnhk")
public suspend fun resourceNames(`value`: ThreeTierVirtualInstanceThreeTierConfigurationResourceNamesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resourceNames = mapped
}
/**
* @param argument A `resource_names` block as defined below. Changing this forces a new resource to be created.
*/
@JvmName("brhphadekakufxbc")
public suspend fun resourceNames(argument: suspend ThreeTierVirtualInstanceThreeTierConfigurationResourceNamesArgsBuilder.() -> Unit) {
val toBeMapped =
ThreeTierVirtualInstanceThreeTierConfigurationResourceNamesArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.resourceNames = mapped
}
/**
* @param value Specifies whether a secondary IP address should be added to the network interface on all VMs of the SAP system being deployed. Defaults to `false`. Changing this forces a new resource to be created.
*/
@JvmName("xymxtwsbehkmogsi")
public suspend fun secondaryIpEnabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.secondaryIpEnabled = mapped
}
/**
* @param value A `transport_create_and_mount` block as defined below. Changing this forces a new resource to be created.
* > **Note:** The file share configuration uses `skip` by default when `transport_create_and_mount` isn't set.
* > **Note:** Due to [a bug in the Azure API](https://github.com/Azure/azure-rest-api-specs/issues/25209) where the Storage File Share Id is not defined correctly, it is not currently possible to support using Transport Mount.
*/
@JvmName("wxvwpehisfmpycfq")
public suspend fun transportCreateAndMount(`value`: ThreeTierVirtualInstanceThreeTierConfigurationTransportCreateAndMountArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.transportCreateAndMount = mapped
}
/**
* @param argument A `transport_create_and_mount` block as defined below. Changing this forces a new resource to be created.
* > **Note:** The file share configuration uses `skip` by default when `transport_create_and_mount` isn't set.
* > **Note:** Due to [a bug in the Azure API](https://github.com/Azure/azure-rest-api-specs/issues/25209) where the Storage File Share Id is not defined correctly, it is not currently possible to support using Transport Mount.
*/
@JvmName("fxljuccbrmpjjmtt")
public suspend fun transportCreateAndMount(argument: suspend ThreeTierVirtualInstanceThreeTierConfigurationTransportCreateAndMountArgsBuilder.() -> Unit) {
val toBeMapped =
ThreeTierVirtualInstanceThreeTierConfigurationTransportCreateAndMountArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.transportCreateAndMount = mapped
}
internal fun build(): ThreeTierVirtualInstanceThreeTierConfigurationArgs =
ThreeTierVirtualInstanceThreeTierConfigurationArgs(
appResourceGroupName = appResourceGroupName ?: throw
PulumiNullFieldException("appResourceGroupName"),
applicationServerConfiguration = applicationServerConfiguration ?: throw
PulumiNullFieldException("applicationServerConfiguration"),
centralServerConfiguration = centralServerConfiguration ?: throw
PulumiNullFieldException("centralServerConfiguration"),
databaseServerConfiguration = databaseServerConfiguration ?: throw
PulumiNullFieldException("databaseServerConfiguration"),
highAvailabilityType = highAvailabilityType,
resourceNames = resourceNames,
secondaryIpEnabled = secondaryIpEnabled,
transportCreateAndMount = transportCreateAndMount,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy