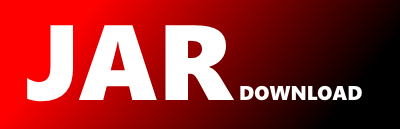
com.pulumi.azurenative.aad.kotlin.inputs.DomainSecuritySettingsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.aad.kotlin.inputs
import com.pulumi.azurenative.aad.inputs.DomainSecuritySettingsArgs.builder
import com.pulumi.azurenative.aad.kotlin.enums.ChannelBinding
import com.pulumi.azurenative.aad.kotlin.enums.KerberosArmoring
import com.pulumi.azurenative.aad.kotlin.enums.KerberosRc4Encryption
import com.pulumi.azurenative.aad.kotlin.enums.LdapSigning
import com.pulumi.azurenative.aad.kotlin.enums.NtlmV1
import com.pulumi.azurenative.aad.kotlin.enums.SyncKerberosPasswords
import com.pulumi.azurenative.aad.kotlin.enums.SyncNtlmPasswords
import com.pulumi.azurenative.aad.kotlin.enums.SyncOnPremPasswords
import com.pulumi.azurenative.aad.kotlin.enums.TlsV1
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Domain Security Settings
* @property channelBinding A flag to determine whether or not ChannelBinding is enabled or disabled.
* @property kerberosArmoring A flag to determine whether or not KerberosArmoring is enabled or disabled.
* @property kerberosRc4Encryption A flag to determine whether or not KerberosRc4Encryption is enabled or disabled.
* @property ldapSigning A flag to determine whether or not LdapSigning is enabled or disabled.
* @property ntlmV1 A flag to determine whether or not NtlmV1 is enabled or disabled.
* @property syncKerberosPasswords A flag to determine whether or not SyncKerberosPasswords is enabled or disabled.
* @property syncNtlmPasswords A flag to determine whether or not SyncNtlmPasswords is enabled or disabled.
* @property syncOnPremPasswords A flag to determine whether or not SyncOnPremPasswords is enabled or disabled.
* @property tlsV1 A flag to determine whether or not TlsV1 is enabled or disabled.
*/
public data class DomainSecuritySettingsArgs(
public val channelBinding: Output>? = null,
public val kerberosArmoring: Output>? = null,
public val kerberosRc4Encryption: Output>? = null,
public val ldapSigning: Output>? = null,
public val ntlmV1: Output>? = null,
public val syncKerberosPasswords: Output>? = null,
public val syncNtlmPasswords: Output>? = null,
public val syncOnPremPasswords: Output>? = null,
public val tlsV1: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.aad.inputs.DomainSecuritySettingsArgs =
com.pulumi.azurenative.aad.inputs.DomainSecuritySettingsArgs.builder()
.channelBinding(
channelBinding?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.kerberosArmoring(
kerberosArmoring?.applyValue({ args0 ->
args0.transform(
{ args0 -> args0 },
{ args0 -> args0.let({ args0 -> args0.toJava() }) },
)
}),
)
.kerberosRc4Encryption(
kerberosRc4Encryption?.applyValue({ args0 ->
args0.transform({ args0 ->
args0
}, { args0 -> args0.let({ args0 -> args0.toJava() }) })
}),
)
.ldapSigning(
ldapSigning?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.ntlmV1(
ntlmV1?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.syncKerberosPasswords(
syncKerberosPasswords?.applyValue({ args0 ->
args0.transform({ args0 ->
args0
}, { args0 -> args0.let({ args0 -> args0.toJava() }) })
}),
)
.syncNtlmPasswords(
syncNtlmPasswords?.applyValue({ args0 ->
args0.transform(
{ args0 -> args0 },
{ args0 -> args0.let({ args0 -> args0.toJava() }) },
)
}),
)
.syncOnPremPasswords(
syncOnPremPasswords?.applyValue({ args0 ->
args0.transform(
{ args0 -> args0 },
{ args0 -> args0.let({ args0 -> args0.toJava() }) },
)
}),
)
.tlsV1(
tlsV1?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
).build()
}
/**
* Builder for [DomainSecuritySettingsArgs].
*/
@PulumiTagMarker
public class DomainSecuritySettingsArgsBuilder internal constructor() {
private var channelBinding: Output>? = null
private var kerberosArmoring: Output>? = null
private var kerberosRc4Encryption: Output>? = null
private var ldapSigning: Output>? = null
private var ntlmV1: Output>? = null
private var syncKerberosPasswords: Output>? = null
private var syncNtlmPasswords: Output>? = null
private var syncOnPremPasswords: Output>? = null
private var tlsV1: Output>? = null
/**
* @param value A flag to determine whether or not ChannelBinding is enabled or disabled.
*/
@JvmName("cocnjoyvkoravell")
public suspend fun channelBinding(`value`: Output>) {
this.channelBinding = value
}
/**
* @param value A flag to determine whether or not KerberosArmoring is enabled or disabled.
*/
@JvmName("mygnvcaxahcbkyjw")
public suspend fun kerberosArmoring(`value`: Output>) {
this.kerberosArmoring = value
}
/**
* @param value A flag to determine whether or not KerberosRc4Encryption is enabled or disabled.
*/
@JvmName("glvalkorcemhmewm")
public suspend fun kerberosRc4Encryption(`value`: Output>) {
this.kerberosRc4Encryption = value
}
/**
* @param value A flag to determine whether or not LdapSigning is enabled or disabled.
*/
@JvmName("enetfjaevocvcgcx")
public suspend fun ldapSigning(`value`: Output>) {
this.ldapSigning = value
}
/**
* @param value A flag to determine whether or not NtlmV1 is enabled or disabled.
*/
@JvmName("bhjryvbfdkjpkfbx")
public suspend fun ntlmV1(`value`: Output>) {
this.ntlmV1 = value
}
/**
* @param value A flag to determine whether or not SyncKerberosPasswords is enabled or disabled.
*/
@JvmName("rfawignduixrmwjd")
public suspend fun syncKerberosPasswords(`value`: Output>) {
this.syncKerberosPasswords = value
}
/**
* @param value A flag to determine whether or not SyncNtlmPasswords is enabled or disabled.
*/
@JvmName("tettgycjpgtemxag")
public suspend fun syncNtlmPasswords(`value`: Output>) {
this.syncNtlmPasswords = value
}
/**
* @param value A flag to determine whether or not SyncOnPremPasswords is enabled or disabled.
*/
@JvmName("qtsuliahvwdabjuy")
public suspend fun syncOnPremPasswords(`value`: Output>) {
this.syncOnPremPasswords = value
}
/**
* @param value A flag to determine whether or not TlsV1 is enabled or disabled.
*/
@JvmName("nfaygkjgmwjivpgu")
public suspend fun tlsV1(`value`: Output>) {
this.tlsV1 = value
}
/**
* @param value A flag to determine whether or not ChannelBinding is enabled or disabled.
*/
@JvmName("mjyllvjjnpdxnttm")
public suspend fun channelBinding(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.channelBinding = mapped
}
/**
* @param value A flag to determine whether or not ChannelBinding is enabled or disabled.
*/
@JvmName("bvspoejsijwbddak")
public fun channelBinding(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.channelBinding = mapped
}
/**
* @param value A flag to determine whether or not ChannelBinding is enabled or disabled.
*/
@JvmName("mfjmxtkytuxvthma")
public fun channelBinding(`value`: ChannelBinding) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.channelBinding = mapped
}
/**
* @param value A flag to determine whether or not KerberosArmoring is enabled or disabled.
*/
@JvmName("ljgvwdtxsmqaeeps")
public suspend fun kerberosArmoring(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kerberosArmoring = mapped
}
/**
* @param value A flag to determine whether or not KerberosArmoring is enabled or disabled.
*/
@JvmName("lufuypgsmebmsalv")
public fun kerberosArmoring(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.kerberosArmoring = mapped
}
/**
* @param value A flag to determine whether or not KerberosArmoring is enabled or disabled.
*/
@JvmName("nvttrnbxyaayqqfq")
public fun kerberosArmoring(`value`: KerberosArmoring) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.kerberosArmoring = mapped
}
/**
* @param value A flag to determine whether or not KerberosRc4Encryption is enabled or disabled.
*/
@JvmName("wtupuakwgjkxxqba")
public suspend fun kerberosRc4Encryption(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kerberosRc4Encryption = mapped
}
/**
* @param value A flag to determine whether or not KerberosRc4Encryption is enabled or disabled.
*/
@JvmName("ruxbiisthqghithu")
public fun kerberosRc4Encryption(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.kerberosRc4Encryption = mapped
}
/**
* @param value A flag to determine whether or not KerberosRc4Encryption is enabled or disabled.
*/
@JvmName("byjgfecfcpwerimv")
public fun kerberosRc4Encryption(`value`: KerberosRc4Encryption) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.kerberosRc4Encryption = mapped
}
/**
* @param value A flag to determine whether or not LdapSigning is enabled or disabled.
*/
@JvmName("oxdwglaxkspmqjok")
public suspend fun ldapSigning(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ldapSigning = mapped
}
/**
* @param value A flag to determine whether or not LdapSigning is enabled or disabled.
*/
@JvmName("wrcvfomyaeewgxgy")
public fun ldapSigning(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.ldapSigning = mapped
}
/**
* @param value A flag to determine whether or not LdapSigning is enabled or disabled.
*/
@JvmName("ipghxfkntwnotdqc")
public fun ldapSigning(`value`: LdapSigning) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.ldapSigning = mapped
}
/**
* @param value A flag to determine whether or not NtlmV1 is enabled or disabled.
*/
@JvmName("pvhninyoybbanqqy")
public suspend fun ntlmV1(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ntlmV1 = mapped
}
/**
* @param value A flag to determine whether or not NtlmV1 is enabled or disabled.
*/
@JvmName("wroumkileeajdgsm")
public fun ntlmV1(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.ntlmV1 = mapped
}
/**
* @param value A flag to determine whether or not NtlmV1 is enabled or disabled.
*/
@JvmName("xncuuefwmtxrtcld")
public fun ntlmV1(`value`: NtlmV1) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.ntlmV1 = mapped
}
/**
* @param value A flag to determine whether or not SyncKerberosPasswords is enabled or disabled.
*/
@JvmName("txdtpjkoqnrsbkvw")
public suspend fun syncKerberosPasswords(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.syncKerberosPasswords = mapped
}
/**
* @param value A flag to determine whether or not SyncKerberosPasswords is enabled or disabled.
*/
@JvmName("ahentbkdyymqjfoc")
public fun syncKerberosPasswords(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.syncKerberosPasswords = mapped
}
/**
* @param value A flag to determine whether or not SyncKerberosPasswords is enabled or disabled.
*/
@JvmName("wrqqvngvireahcyh")
public fun syncKerberosPasswords(`value`: SyncKerberosPasswords) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.syncKerberosPasswords = mapped
}
/**
* @param value A flag to determine whether or not SyncNtlmPasswords is enabled or disabled.
*/
@JvmName("gcsfifqkwguyykqw")
public suspend fun syncNtlmPasswords(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.syncNtlmPasswords = mapped
}
/**
* @param value A flag to determine whether or not SyncNtlmPasswords is enabled or disabled.
*/
@JvmName("dugwtfbxhqgwuhqf")
public fun syncNtlmPasswords(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.syncNtlmPasswords = mapped
}
/**
* @param value A flag to determine whether or not SyncNtlmPasswords is enabled or disabled.
*/
@JvmName("lcxiyehcfuknehlo")
public fun syncNtlmPasswords(`value`: SyncNtlmPasswords) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.syncNtlmPasswords = mapped
}
/**
* @param value A flag to determine whether or not SyncOnPremPasswords is enabled or disabled.
*/
@JvmName("hcwxfndsschgoosa")
public suspend fun syncOnPremPasswords(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.syncOnPremPasswords = mapped
}
/**
* @param value A flag to determine whether or not SyncOnPremPasswords is enabled or disabled.
*/
@JvmName("gojvabdqtscbocgx")
public fun syncOnPremPasswords(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.syncOnPremPasswords = mapped
}
/**
* @param value A flag to determine whether or not SyncOnPremPasswords is enabled or disabled.
*/
@JvmName("flvkookkuxlveyvh")
public fun syncOnPremPasswords(`value`: SyncOnPremPasswords) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.syncOnPremPasswords = mapped
}
/**
* @param value A flag to determine whether or not TlsV1 is enabled or disabled.
*/
@JvmName("aasmmpxcsvgjfyhj")
public suspend fun tlsV1(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tlsV1 = mapped
}
/**
* @param value A flag to determine whether or not TlsV1 is enabled or disabled.
*/
@JvmName("ngjysxsdbvvxwpjb")
public fun tlsV1(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.tlsV1 = mapped
}
/**
* @param value A flag to determine whether or not TlsV1 is enabled or disabled.
*/
@JvmName("kbbbfqlpqoldwfvt")
public fun tlsV1(`value`: TlsV1) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.tlsV1 = mapped
}
internal fun build(): DomainSecuritySettingsArgs = DomainSecuritySettingsArgs(
channelBinding = channelBinding,
kerberosArmoring = kerberosArmoring,
kerberosRc4Encryption = kerberosRc4Encryption,
ldapSigning = ldapSigning,
ntlmV1 = ntlmV1,
syncKerberosPasswords = syncKerberosPasswords,
syncNtlmPasswords = syncNtlmPasswords,
syncOnPremPasswords = syncOnPremPasswords,
tlsV1 = tlsV1,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy