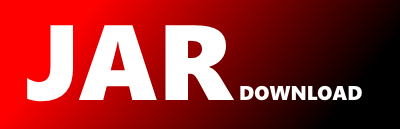
com.pulumi.azurenative.alertsmanagement.kotlin.inputs.ConditionsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.alertsmanagement.kotlin.inputs
import com.pulumi.azurenative.alertsmanagement.inputs.ConditionsArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Conditions in alert instance to be matched for a given action rule. Default value is all. Multiple values could be provided with comma separation.
* @property alertContext filter alerts by alert context (payload)
* @property alertRuleId filter alerts by alert rule id
* @property alertRuleName filter alerts by alert rule name
* @property description filter alerts by alert rule description
* @property monitorCondition filter alerts by monitor condition
* @property monitorService filter alerts by monitor service
* @property severity filter alerts by severity
* @property targetResourceType filter alerts by target resource type
*/
public data class ConditionsArgs(
public val alertContext: Output? = null,
public val alertRuleId: Output? = null,
public val alertRuleName: Output? = null,
public val description: Output? = null,
public val monitorCondition: Output? = null,
public val monitorService: Output? = null,
public val severity: Output? = null,
public val targetResourceType: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.alertsmanagement.inputs.ConditionsArgs =
com.pulumi.azurenative.alertsmanagement.inputs.ConditionsArgs.builder()
.alertContext(alertContext?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.alertRuleId(alertRuleId?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.alertRuleName(alertRuleName?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.description(description?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.monitorCondition(monitorCondition?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.monitorService(monitorService?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.severity(severity?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.targetResourceType(
targetResourceType?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [ConditionsArgs].
*/
@PulumiTagMarker
public class ConditionsArgsBuilder internal constructor() {
private var alertContext: Output? = null
private var alertRuleId: Output? = null
private var alertRuleName: Output? = null
private var description: Output? = null
private var monitorCondition: Output? = null
private var monitorService: Output? = null
private var severity: Output? = null
private var targetResourceType: Output? = null
/**
* @param value filter alerts by alert context (payload)
*/
@JvmName("ygolptuaxcqonnch")
public suspend fun alertContext(`value`: Output) {
this.alertContext = value
}
/**
* @param value filter alerts by alert rule id
*/
@JvmName("korpradtwborvrbh")
public suspend fun alertRuleId(`value`: Output) {
this.alertRuleId = value
}
/**
* @param value filter alerts by alert rule name
*/
@JvmName("folecnykjsqbbmmr")
public suspend fun alertRuleName(`value`: Output) {
this.alertRuleName = value
}
/**
* @param value filter alerts by alert rule description
*/
@JvmName("uwdmwleqckdysrbs")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value filter alerts by monitor condition
*/
@JvmName("uwybjtqehhbenbec")
public suspend fun monitorCondition(`value`: Output) {
this.monitorCondition = value
}
/**
* @param value filter alerts by monitor service
*/
@JvmName("fpqqvqbaaesvqlxj")
public suspend fun monitorService(`value`: Output) {
this.monitorService = value
}
/**
* @param value filter alerts by severity
*/
@JvmName("qmillgoiddatcojq")
public suspend fun severity(`value`: Output) {
this.severity = value
}
/**
* @param value filter alerts by target resource type
*/
@JvmName("nyefoyokkenjptbl")
public suspend fun targetResourceType(`value`: Output) {
this.targetResourceType = value
}
/**
* @param value filter alerts by alert context (payload)
*/
@JvmName("iefgjjvbamwdbkpm")
public suspend fun alertContext(`value`: ConditionArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.alertContext = mapped
}
/**
* @param argument filter alerts by alert context (payload)
*/
@JvmName("blpgbdgcxseyrgvk")
public suspend fun alertContext(argument: suspend ConditionArgsBuilder.() -> Unit) {
val toBeMapped = ConditionArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.alertContext = mapped
}
/**
* @param value filter alerts by alert rule id
*/
@JvmName("glaagvcebcjlsdpj")
public suspend fun alertRuleId(`value`: ConditionArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.alertRuleId = mapped
}
/**
* @param argument filter alerts by alert rule id
*/
@JvmName("mqkgscjuosvcnojs")
public suspend fun alertRuleId(argument: suspend ConditionArgsBuilder.() -> Unit) {
val toBeMapped = ConditionArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.alertRuleId = mapped
}
/**
* @param value filter alerts by alert rule name
*/
@JvmName("xklaogdbakgmrcta")
public suspend fun alertRuleName(`value`: ConditionArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.alertRuleName = mapped
}
/**
* @param argument filter alerts by alert rule name
*/
@JvmName("eoxetlbqkiuxwoln")
public suspend fun alertRuleName(argument: suspend ConditionArgsBuilder.() -> Unit) {
val toBeMapped = ConditionArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.alertRuleName = mapped
}
/**
* @param value filter alerts by alert rule description
*/
@JvmName("wtcmeatqcglgwiay")
public suspend fun description(`value`: ConditionArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.description = mapped
}
/**
* @param argument filter alerts by alert rule description
*/
@JvmName("ejwetpriulaogouv")
public suspend fun description(argument: suspend ConditionArgsBuilder.() -> Unit) {
val toBeMapped = ConditionArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.description = mapped
}
/**
* @param value filter alerts by monitor condition
*/
@JvmName("pfhcewfmstjyfmap")
public suspend fun monitorCondition(`value`: ConditionArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.monitorCondition = mapped
}
/**
* @param argument filter alerts by monitor condition
*/
@JvmName("xlcxerljxocvkdmn")
public suspend fun monitorCondition(argument: suspend ConditionArgsBuilder.() -> Unit) {
val toBeMapped = ConditionArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.monitorCondition = mapped
}
/**
* @param value filter alerts by monitor service
*/
@JvmName("nbkiiexwwijyxhwx")
public suspend fun monitorService(`value`: ConditionArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.monitorService = mapped
}
/**
* @param argument filter alerts by monitor service
*/
@JvmName("wqagooqjryqxtmdo")
public suspend fun monitorService(argument: suspend ConditionArgsBuilder.() -> Unit) {
val toBeMapped = ConditionArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.monitorService = mapped
}
/**
* @param value filter alerts by severity
*/
@JvmName("txffinscgnajmwwh")
public suspend fun severity(`value`: ConditionArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.severity = mapped
}
/**
* @param argument filter alerts by severity
*/
@JvmName("ltkgpbgssktgjfms")
public suspend fun severity(argument: suspend ConditionArgsBuilder.() -> Unit) {
val toBeMapped = ConditionArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.severity = mapped
}
/**
* @param value filter alerts by target resource type
*/
@JvmName("brxavstfuyibgpfd")
public suspend fun targetResourceType(`value`: ConditionArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.targetResourceType = mapped
}
/**
* @param argument filter alerts by target resource type
*/
@JvmName("iggmcjcpswrltmsq")
public suspend fun targetResourceType(argument: suspend ConditionArgsBuilder.() -> Unit) {
val toBeMapped = ConditionArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.targetResourceType = mapped
}
internal fun build(): ConditionsArgs = ConditionsArgs(
alertContext = alertContext,
alertRuleId = alertRuleId,
alertRuleName = alertRuleName,
description = description,
monitorCondition = monitorCondition,
monitorService = monitorService,
severity = severity,
targetResourceType = targetResourceType,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy