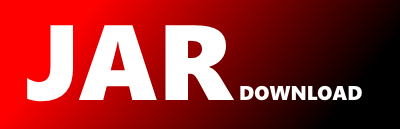
com.pulumi.azurenative.apicenter.kotlin.ApicenterFunctions.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.apicenter.kotlin
import com.pulumi.azurenative.apicenter.ApicenterFunctions.getApiDefinitionPlain
import com.pulumi.azurenative.apicenter.ApicenterFunctions.getApiPlain
import com.pulumi.azurenative.apicenter.ApicenterFunctions.getApiVersionPlain
import com.pulumi.azurenative.apicenter.ApicenterFunctions.getDeploymentPlain
import com.pulumi.azurenative.apicenter.ApicenterFunctions.getEnvironmentPlain
import com.pulumi.azurenative.apicenter.ApicenterFunctions.getMetadataSchemaPlain
import com.pulumi.azurenative.apicenter.ApicenterFunctions.getServicePlain
import com.pulumi.azurenative.apicenter.ApicenterFunctions.getWorkspacePlain
import com.pulumi.azurenative.apicenter.kotlin.inputs.GetApiDefinitionPlainArgs
import com.pulumi.azurenative.apicenter.kotlin.inputs.GetApiDefinitionPlainArgsBuilder
import com.pulumi.azurenative.apicenter.kotlin.inputs.GetApiPlainArgs
import com.pulumi.azurenative.apicenter.kotlin.inputs.GetApiPlainArgsBuilder
import com.pulumi.azurenative.apicenter.kotlin.inputs.GetApiVersionPlainArgs
import com.pulumi.azurenative.apicenter.kotlin.inputs.GetApiVersionPlainArgsBuilder
import com.pulumi.azurenative.apicenter.kotlin.inputs.GetDeploymentPlainArgs
import com.pulumi.azurenative.apicenter.kotlin.inputs.GetDeploymentPlainArgsBuilder
import com.pulumi.azurenative.apicenter.kotlin.inputs.GetEnvironmentPlainArgs
import com.pulumi.azurenative.apicenter.kotlin.inputs.GetEnvironmentPlainArgsBuilder
import com.pulumi.azurenative.apicenter.kotlin.inputs.GetMetadataSchemaPlainArgs
import com.pulumi.azurenative.apicenter.kotlin.inputs.GetMetadataSchemaPlainArgsBuilder
import com.pulumi.azurenative.apicenter.kotlin.inputs.GetServicePlainArgs
import com.pulumi.azurenative.apicenter.kotlin.inputs.GetServicePlainArgsBuilder
import com.pulumi.azurenative.apicenter.kotlin.inputs.GetWorkspacePlainArgs
import com.pulumi.azurenative.apicenter.kotlin.inputs.GetWorkspacePlainArgsBuilder
import com.pulumi.azurenative.apicenter.kotlin.outputs.GetApiDefinitionResult
import com.pulumi.azurenative.apicenter.kotlin.outputs.GetApiResult
import com.pulumi.azurenative.apicenter.kotlin.outputs.GetApiVersionResult
import com.pulumi.azurenative.apicenter.kotlin.outputs.GetDeploymentResult
import com.pulumi.azurenative.apicenter.kotlin.outputs.GetEnvironmentResult
import com.pulumi.azurenative.apicenter.kotlin.outputs.GetMetadataSchemaResult
import com.pulumi.azurenative.apicenter.kotlin.outputs.GetServiceResult
import com.pulumi.azurenative.apicenter.kotlin.outputs.GetWorkspaceResult
import kotlinx.coroutines.future.await
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.azurenative.apicenter.kotlin.outputs.GetApiDefinitionResult.Companion.toKotlin as getApiDefinitionResultToKotlin
import com.pulumi.azurenative.apicenter.kotlin.outputs.GetApiResult.Companion.toKotlin as getApiResultToKotlin
import com.pulumi.azurenative.apicenter.kotlin.outputs.GetApiVersionResult.Companion.toKotlin as getApiVersionResultToKotlin
import com.pulumi.azurenative.apicenter.kotlin.outputs.GetDeploymentResult.Companion.toKotlin as getDeploymentResultToKotlin
import com.pulumi.azurenative.apicenter.kotlin.outputs.GetEnvironmentResult.Companion.toKotlin as getEnvironmentResultToKotlin
import com.pulumi.azurenative.apicenter.kotlin.outputs.GetMetadataSchemaResult.Companion.toKotlin as getMetadataSchemaResultToKotlin
import com.pulumi.azurenative.apicenter.kotlin.outputs.GetServiceResult.Companion.toKotlin as getServiceResultToKotlin
import com.pulumi.azurenative.apicenter.kotlin.outputs.GetWorkspaceResult.Companion.toKotlin as getWorkspaceResultToKotlin
public object ApicenterFunctions {
/**
* Returns details of the API.
* Azure REST API version: 2024-03-01.
* Other available API versions: 2024-03-15-preview.
* @param argument null
* @return API entity.
*/
public suspend fun getApi(argument: GetApiPlainArgs): GetApiResult =
getApiResultToKotlin(getApiPlain(argument.toJava()).await())
/**
* @see [getApi].
* @param apiName The name of the API.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param serviceName The name of Azure API Center service.
* @param workspaceName The name of the workspace.
* @return API entity.
*/
public suspend fun getApi(
apiName: String,
resourceGroupName: String,
serviceName: String,
workspaceName: String,
): GetApiResult {
val argument = GetApiPlainArgs(
apiName = apiName,
resourceGroupName = resourceGroupName,
serviceName = serviceName,
workspaceName = workspaceName,
)
return getApiResultToKotlin(getApiPlain(argument.toJava()).await())
}
/**
* @see [getApi].
* @param argument Builder for [com.pulumi.azurenative.apicenter.kotlin.inputs.GetApiPlainArgs].
* @return API entity.
*/
public suspend fun getApi(argument: suspend GetApiPlainArgsBuilder.() -> Unit): GetApiResult {
val builder = GetApiPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getApiResultToKotlin(getApiPlain(builtArgument.toJava()).await())
}
/**
* Returns details of the API definition.
* Azure REST API version: 2024-03-01.
* Other available API versions: 2024-03-15-preview.
* @param argument null
* @return API definition entity.
*/
public suspend fun getApiDefinition(argument: GetApiDefinitionPlainArgs): GetApiDefinitionResult =
getApiDefinitionResultToKotlin(getApiDefinitionPlain(argument.toJava()).await())
/**
* @see [getApiDefinition].
* @param apiName The name of the API.
* @param definitionName The name of the API definition.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param serviceName The name of Azure API Center service.
* @param versionName The name of the API version.
* @param workspaceName The name of the workspace.
* @return API definition entity.
*/
public suspend fun getApiDefinition(
apiName: String,
definitionName: String,
resourceGroupName: String,
serviceName: String,
versionName: String,
workspaceName: String,
): GetApiDefinitionResult {
val argument = GetApiDefinitionPlainArgs(
apiName = apiName,
definitionName = definitionName,
resourceGroupName = resourceGroupName,
serviceName = serviceName,
versionName = versionName,
workspaceName = workspaceName,
)
return getApiDefinitionResultToKotlin(getApiDefinitionPlain(argument.toJava()).await())
}
/**
* @see [getApiDefinition].
* @param argument Builder for [com.pulumi.azurenative.apicenter.kotlin.inputs.GetApiDefinitionPlainArgs].
* @return API definition entity.
*/
public suspend fun getApiDefinition(argument: suspend GetApiDefinitionPlainArgsBuilder.() -> Unit): GetApiDefinitionResult {
val builder = GetApiDefinitionPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getApiDefinitionResultToKotlin(getApiDefinitionPlain(builtArgument.toJava()).await())
}
/**
* Returns details of the API version.
* Azure REST API version: 2024-03-01.
* Other available API versions: 2024-03-15-preview.
* @param argument null
* @return API version entity.
*/
public suspend fun getApiVersion(argument: GetApiVersionPlainArgs): GetApiVersionResult =
getApiVersionResultToKotlin(getApiVersionPlain(argument.toJava()).await())
/**
* @see [getApiVersion].
* @param apiName The name of the API.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param serviceName The name of Azure API Center service.
* @param versionName The name of the API version.
* @param workspaceName The name of the workspace.
* @return API version entity.
*/
public suspend fun getApiVersion(
apiName: String,
resourceGroupName: String,
serviceName: String,
versionName: String,
workspaceName: String,
): GetApiVersionResult {
val argument = GetApiVersionPlainArgs(
apiName = apiName,
resourceGroupName = resourceGroupName,
serviceName = serviceName,
versionName = versionName,
workspaceName = workspaceName,
)
return getApiVersionResultToKotlin(getApiVersionPlain(argument.toJava()).await())
}
/**
* @see [getApiVersion].
* @param argument Builder for [com.pulumi.azurenative.apicenter.kotlin.inputs.GetApiVersionPlainArgs].
* @return API version entity.
*/
public suspend fun getApiVersion(argument: suspend GetApiVersionPlainArgsBuilder.() -> Unit): GetApiVersionResult {
val builder = GetApiVersionPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getApiVersionResultToKotlin(getApiVersionPlain(builtArgument.toJava()).await())
}
/**
* Returns details of the API deployment.
* Azure REST API version: 2024-03-01.
* Other available API versions: 2024-03-15-preview.
* @param argument null
* @return API deployment entity.
*/
public suspend fun getDeployment(argument: GetDeploymentPlainArgs): GetDeploymentResult =
getDeploymentResultToKotlin(getDeploymentPlain(argument.toJava()).await())
/**
* @see [getDeployment].
* @param apiName The name of the API.
* @param deploymentName The name of the API deployment.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param serviceName The name of Azure API Center service.
* @param workspaceName The name of the workspace.
* @return API deployment entity.
*/
public suspend fun getDeployment(
apiName: String,
deploymentName: String,
resourceGroupName: String,
serviceName: String,
workspaceName: String,
): GetDeploymentResult {
val argument = GetDeploymentPlainArgs(
apiName = apiName,
deploymentName = deploymentName,
resourceGroupName = resourceGroupName,
serviceName = serviceName,
workspaceName = workspaceName,
)
return getDeploymentResultToKotlin(getDeploymentPlain(argument.toJava()).await())
}
/**
* @see [getDeployment].
* @param argument Builder for [com.pulumi.azurenative.apicenter.kotlin.inputs.GetDeploymentPlainArgs].
* @return API deployment entity.
*/
public suspend fun getDeployment(argument: suspend GetDeploymentPlainArgsBuilder.() -> Unit): GetDeploymentResult {
val builder = GetDeploymentPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getDeploymentResultToKotlin(getDeploymentPlain(builtArgument.toJava()).await())
}
/**
* Returns details of the environment.
* Azure REST API version: 2024-03-01.
* Other available API versions: 2024-03-15-preview.
* @param argument null
* @return Environment entity.
*/
public suspend fun getEnvironment(argument: GetEnvironmentPlainArgs): GetEnvironmentResult =
getEnvironmentResultToKotlin(getEnvironmentPlain(argument.toJava()).await())
/**
* @see [getEnvironment].
* @param environmentName The name of the environment.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param serviceName The name of Azure API Center service.
* @param workspaceName The name of the workspace.
* @return Environment entity.
*/
public suspend fun getEnvironment(
environmentName: String,
resourceGroupName: String,
serviceName: String,
workspaceName: String,
): GetEnvironmentResult {
val argument = GetEnvironmentPlainArgs(
environmentName = environmentName,
resourceGroupName = resourceGroupName,
serviceName = serviceName,
workspaceName = workspaceName,
)
return getEnvironmentResultToKotlin(getEnvironmentPlain(argument.toJava()).await())
}
/**
* @see [getEnvironment].
* @param argument Builder for [com.pulumi.azurenative.apicenter.kotlin.inputs.GetEnvironmentPlainArgs].
* @return Environment entity.
*/
public suspend fun getEnvironment(argument: suspend GetEnvironmentPlainArgsBuilder.() -> Unit): GetEnvironmentResult {
val builder = GetEnvironmentPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getEnvironmentResultToKotlin(getEnvironmentPlain(builtArgument.toJava()).await())
}
/**
* Returns details of the metadata schema.
* Azure REST API version: 2024-03-01.
* Other available API versions: 2024-03-15-preview.
* @param argument null
* @return Metadata schema entity. Used to define metadata for the entities in API catalog.
*/
public suspend fun getMetadataSchema(argument: GetMetadataSchemaPlainArgs): GetMetadataSchemaResult =
getMetadataSchemaResultToKotlin(getMetadataSchemaPlain(argument.toJava()).await())
/**
* @see [getMetadataSchema].
* @param metadataSchemaName The name of the metadata schema.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param serviceName The name of Azure API Center service.
* @return Metadata schema entity. Used to define metadata for the entities in API catalog.
*/
public suspend fun getMetadataSchema(
metadataSchemaName: String,
resourceGroupName: String,
serviceName: String,
): GetMetadataSchemaResult {
val argument = GetMetadataSchemaPlainArgs(
metadataSchemaName = metadataSchemaName,
resourceGroupName = resourceGroupName,
serviceName = serviceName,
)
return getMetadataSchemaResultToKotlin(getMetadataSchemaPlain(argument.toJava()).await())
}
/**
* @see [getMetadataSchema].
* @param argument Builder for [com.pulumi.azurenative.apicenter.kotlin.inputs.GetMetadataSchemaPlainArgs].
* @return Metadata schema entity. Used to define metadata for the entities in API catalog.
*/
public suspend fun getMetadataSchema(argument: suspend GetMetadataSchemaPlainArgsBuilder.() -> Unit): GetMetadataSchemaResult {
val builder = GetMetadataSchemaPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getMetadataSchemaResultToKotlin(getMetadataSchemaPlain(builtArgument.toJava()).await())
}
/**
* Get service
* Azure REST API version: 2023-07-01-preview.
* Other available API versions: 2024-03-01, 2024-03-15-preview.
* @param argument null
* @return The service entity.
*/
public suspend fun getService(argument: GetServicePlainArgs): GetServiceResult =
getServiceResultToKotlin(getServicePlain(argument.toJava()).await())
/**
* @see [getService].
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param serviceName Service name
* @return The service entity.
*/
public suspend fun getService(resourceGroupName: String, serviceName: String): GetServiceResult {
val argument = GetServicePlainArgs(
resourceGroupName = resourceGroupName,
serviceName = serviceName,
)
return getServiceResultToKotlin(getServicePlain(argument.toJava()).await())
}
/**
* @see [getService].
* @param argument Builder for [com.pulumi.azurenative.apicenter.kotlin.inputs.GetServicePlainArgs].
* @return The service entity.
*/
public suspend fun getService(argument: suspend GetServicePlainArgsBuilder.() -> Unit): GetServiceResult {
val builder = GetServicePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getServiceResultToKotlin(getServicePlain(builtArgument.toJava()).await())
}
/**
* Returns details of the workspace.
* Azure REST API version: 2024-03-01.
* Other available API versions: 2024-03-15-preview.
* @param argument null
* @return Workspace entity.
*/
public suspend fun getWorkspace(argument: GetWorkspacePlainArgs): GetWorkspaceResult =
getWorkspaceResultToKotlin(getWorkspacePlain(argument.toJava()).await())
/**
* @see [getWorkspace].
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param serviceName The name of Azure API Center service.
* @param workspaceName The name of the workspace.
* @return Workspace entity.
*/
public suspend fun getWorkspace(
resourceGroupName: String,
serviceName: String,
workspaceName: String,
): GetWorkspaceResult {
val argument = GetWorkspacePlainArgs(
resourceGroupName = resourceGroupName,
serviceName = serviceName,
workspaceName = workspaceName,
)
return getWorkspaceResultToKotlin(getWorkspacePlain(argument.toJava()).await())
}
/**
* @see [getWorkspace].
* @param argument Builder for [com.pulumi.azurenative.apicenter.kotlin.inputs.GetWorkspacePlainArgs].
* @return Workspace entity.
*/
public suspend fun getWorkspace(argument: suspend GetWorkspacePlainArgsBuilder.() -> Unit): GetWorkspaceResult {
val builder = GetWorkspacePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getWorkspaceResultToKotlin(getWorkspacePlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy