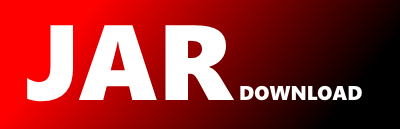
com.pulumi.azurenative.apimanagement.kotlin.AuthorizationServer.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.apimanagement.kotlin
import com.pulumi.azurenative.apimanagement.kotlin.outputs.TokenBodyParameterContractResponse
import com.pulumi.azurenative.apimanagement.kotlin.outputs.TokenBodyParameterContractResponse.Companion.toKotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
/**
* Builder for [AuthorizationServer].
*/
@PulumiTagMarker
public class AuthorizationServerResourceBuilder internal constructor() {
public var name: String? = null
public var args: AuthorizationServerArgs = AuthorizationServerArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend AuthorizationServerArgsBuilder.() -> Unit) {
val builder = AuthorizationServerArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): AuthorizationServer {
val builtJavaResource =
com.pulumi.azurenative.apimanagement.AuthorizationServer(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return AuthorizationServer(builtJavaResource)
}
}
/**
* External OAuth authorization server settings.
* Azure REST API version: 2022-08-01. Prior API version in Azure Native 1.x: 2020-12-01.
* Other available API versions: 2016-07-07, 2016-10-10, 2022-09-01-preview, 2023-03-01-preview, 2023-05-01-preview, 2023-09-01-preview, 2024-05-01.
* ## Example Usage
* ### ApiManagementCreateAuthorizationServer
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var authorizationServer = new AzureNative.ApiManagement.AuthorizationServer("authorizationServer", new()
* {
* AuthorizationEndpoint = "https://www.contoso.com/oauth2/auth",
* AuthorizationMethods = new[]
* {
* AzureNative.ApiManagement.AuthorizationMethod.GET,
* },
* Authsid = "newauthServer",
* BearerTokenSendingMethods = new[]
* {
* AzureNative.ApiManagement.BearerTokenSendingMethod.AuthorizationHeader,
* },
* ClientId = "1",
* ClientRegistrationEndpoint = "https://www.contoso.com/apps",
* ClientSecret = "2",
* DefaultScope = "read write",
* Description = "test server",
* DisplayName = "test2",
* GrantTypes = new[]
* {
* AzureNative.ApiManagement.GrantType.AuthorizationCode,
* AzureNative.ApiManagement.GrantType.@Implicit,
* },
* ResourceGroupName = "rg1",
* ResourceOwnerPassword = "pwd",
* ResourceOwnerUsername = "un",
* ServiceName = "apimService1",
* SupportState = true,
* TokenEndpoint = "https://www.contoso.com/oauth2/token",
* UseInApiDocumentation = true,
* UseInTestConsole = false,
* });
* });
* ```
* ```go
* package main
* import (
* apimanagement "github.com/pulumi/pulumi-azure-native-sdk/apimanagement/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := apimanagement.NewAuthorizationServer(ctx, "authorizationServer", &apimanagement.AuthorizationServerArgs{
* AuthorizationEndpoint: pulumi.String("https://www.contoso.com/oauth2/auth"),
* AuthorizationMethods: apimanagement.AuthorizationMethodArray{
* apimanagement.AuthorizationMethodGET,
* },
* Authsid: pulumi.String("newauthServer"),
* BearerTokenSendingMethods: pulumi.StringArray{
* pulumi.String(apimanagement.BearerTokenSendingMethodAuthorizationHeader),
* },
* ClientId: pulumi.String("1"),
* ClientRegistrationEndpoint: pulumi.String("https://www.contoso.com/apps"),
* ClientSecret: pulumi.String("2"),
* DefaultScope: pulumi.String("read write"),
* Description: pulumi.String("test server"),
* DisplayName: pulumi.String("test2"),
* GrantTypes: pulumi.StringArray{
* pulumi.String(apimanagement.GrantTypeAuthorizationCode),
* pulumi.String(apimanagement.GrantTypeImplicit),
* },
* ResourceGroupName: pulumi.String("rg1"),
* ResourceOwnerPassword: pulumi.String("pwd"),
* ResourceOwnerUsername: pulumi.String("un"),
* ServiceName: pulumi.String("apimService1"),
* SupportState: pulumi.Bool(true),
* TokenEndpoint: pulumi.String("https://www.contoso.com/oauth2/token"),
* UseInApiDocumentation: pulumi.Bool(true),
* UseInTestConsole: pulumi.Bool(false),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.apimanagement.AuthorizationServer;
* import com.pulumi.azurenative.apimanagement.AuthorizationServerArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var authorizationServer = new AuthorizationServer("authorizationServer", AuthorizationServerArgs.builder()
* .authorizationEndpoint("https://www.contoso.com/oauth2/auth")
* .authorizationMethods("GET")
* .authsid("newauthServer")
* .bearerTokenSendingMethods("authorizationHeader")
* .clientId("1")
* .clientRegistrationEndpoint("https://www.contoso.com/apps")
* .clientSecret("2")
* .defaultScope("read write")
* .description("test server")
* .displayName("test2")
* .grantTypes(
* "authorizationCode",
* "implicit")
* .resourceGroupName("rg1")
* .resourceOwnerPassword("pwd")
* .resourceOwnerUsername("un")
* .serviceName("apimService1")
* .supportState(true)
* .tokenEndpoint("https://www.contoso.com/oauth2/token")
* .useInApiDocumentation(true)
* .useInTestConsole(false)
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:apimanagement:AuthorizationServer newauthServer /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.ApiManagement/service/{serviceName}/authorizationServers/{authsid}
* ```
*/
public class AuthorizationServer internal constructor(
override val javaResource: com.pulumi.azurenative.apimanagement.AuthorizationServer,
) : KotlinCustomResource(javaResource, AuthorizationServerMapper) {
/**
* OAuth authorization endpoint. See http://tools.ietf.org/html/rfc6749#section-3.2.
*/
public val authorizationEndpoint: Output
get() = javaResource.authorizationEndpoint().applyValue({ args0 -> args0 })
/**
* HTTP verbs supported by the authorization endpoint. GET must be always present. POST is optional.
*/
public val authorizationMethods: Output>?
get() = javaResource.authorizationMethods().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
/**
* Specifies the mechanism by which access token is passed to the API.
*/
public val bearerTokenSendingMethods: Output>?
get() = javaResource.bearerTokenSendingMethods().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
/**
* Method of authentication supported by the token endpoint of this authorization server. Possible values are Basic and/or Body. When Body is specified, client credentials and other parameters are passed within the request body in the application/x-www-form-urlencoded format.
*/
public val clientAuthenticationMethod: Output>?
get() = javaResource.clientAuthenticationMethod().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
/**
* Client or app id registered with this authorization server.
*/
public val clientId: Output
get() = javaResource.clientId().applyValue({ args0 -> args0 })
/**
* Optional reference to a page where client or app registration for this authorization server is performed. Contains absolute URL to entity being referenced.
*/
public val clientRegistrationEndpoint: Output
get() = javaResource.clientRegistrationEndpoint().applyValue({ args0 -> args0 })
/**
* Client or app secret registered with this authorization server. This property will not be filled on 'GET' operations! Use '/listSecrets' POST request to get the value.
*/
public val clientSecret: Output?
get() = javaResource.clientSecret().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Access token scope that is going to be requested by default. Can be overridden at the API level. Should be provided in the form of a string containing space-delimited values.
*/
public val defaultScope: Output?
get() = javaResource.defaultScope().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Description of the authorization server. Can contain HTML formatting tags.
*/
public val description: Output?
get() = javaResource.description().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* User-friendly authorization server name.
*/
public val displayName: Output
get() = javaResource.displayName().applyValue({ args0 -> args0 })
/**
* Form of an authorization grant, which the client uses to request the access token.
*/
public val grantTypes: Output>
get() = javaResource.grantTypes().applyValue({ args0 -> args0.map({ args0 -> args0 }) })
/**
* The name of the resource
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* Can be optionally specified when resource owner password grant type is supported by this authorization server. Default resource owner password.
*/
public val resourceOwnerPassword: Output?
get() = javaResource.resourceOwnerPassword().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Can be optionally specified when resource owner password grant type is supported by this authorization server. Default resource owner username.
*/
public val resourceOwnerUsername: Output?
get() = javaResource.resourceOwnerUsername().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* If true, authorization server will include state parameter from the authorization request to its response. Client may use state parameter to raise protocol security.
*/
public val supportState: Output?
get() = javaResource.supportState().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Additional parameters required by the token endpoint of this authorization server represented as an array of JSON objects with name and value string properties, i.e. {"name" : "name value", "value": "a value"}.
*/
public val tokenBodyParameters: Output>?
get() = javaResource.tokenBodyParameters().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0.let({ args0 -> toKotlin(args0) }) })
}).orElse(null)
})
/**
* OAuth token endpoint. Contains absolute URI to entity being referenced.
*/
public val tokenEndpoint: Output?
get() = javaResource.tokenEndpoint().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*/
public val type: Output
get() = javaResource.type().applyValue({ args0 -> args0 })
/**
* If true, the authorization server will be used in the API documentation in the developer portal. False by default if no value is provided.
*/
public val useInApiDocumentation: Output?
get() = javaResource.useInApiDocumentation().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* If true, the authorization server may be used in the developer portal test console. True by default if no value is provided.
*/
public val useInTestConsole: Output?
get() = javaResource.useInTestConsole().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
}
public object AuthorizationServerMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.azurenative.apimanagement.AuthorizationServer::class == javaResource::class
override fun map(javaResource: Resource): AuthorizationServer = AuthorizationServer(
javaResource
as com.pulumi.azurenative.apimanagement.AuthorizationServer,
)
}
/**
* @see [AuthorizationServer].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [AuthorizationServer].
*/
public suspend fun authorizationServer(
name: String,
block: suspend AuthorizationServerResourceBuilder.() -> Unit,
): AuthorizationServer {
val builder = AuthorizationServerResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [AuthorizationServer].
* @param name The _unique_ name of the resulting resource.
*/
public fun authorizationServer(name: String): AuthorizationServer {
val builder = AuthorizationServerResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy