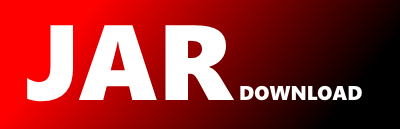
com.pulumi.azurenative.apimanagement.kotlin.IdentityProvider.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.apimanagement.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
/**
* Builder for [IdentityProvider].
*/
@PulumiTagMarker
public class IdentityProviderResourceBuilder internal constructor() {
public var name: String? = null
public var args: IdentityProviderArgs = IdentityProviderArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend IdentityProviderArgsBuilder.() -> Unit) {
val builder = IdentityProviderArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): IdentityProvider {
val builtJavaResource =
com.pulumi.azurenative.apimanagement.IdentityProvider(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return IdentityProvider(builtJavaResource)
}
}
/**
* Identity Provider details.
* Azure REST API version: 2022-08-01. Prior API version in Azure Native 1.x: 2020-12-01.
* Other available API versions: 2016-07-07, 2016-10-10, 2019-01-01, 2022-09-01-preview, 2023-03-01-preview, 2023-05-01-preview, 2023-09-01-preview, 2024-05-01.
* ## Example Usage
* ### ApiManagementCreateIdentityProvider
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var identityProvider = new AzureNative.ApiManagement.IdentityProvider("identityProvider", new()
* {
* ClientId = "facebookid",
* ClientSecret = "facebookapplicationsecret",
* IdentityProviderName = "facebook",
* ResourceGroupName = "rg1",
* ServiceName = "apimService1",
* });
* });
* ```
* ```go
* package main
* import (
* apimanagement "github.com/pulumi/pulumi-azure-native-sdk/apimanagement/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := apimanagement.NewIdentityProvider(ctx, "identityProvider", &apimanagement.IdentityProviderArgs{
* ClientId: pulumi.String("facebookid"),
* ClientSecret: pulumi.String("facebookapplicationsecret"),
* IdentityProviderName: pulumi.String("facebook"),
* ResourceGroupName: pulumi.String("rg1"),
* ServiceName: pulumi.String("apimService1"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.apimanagement.IdentityProvider;
* import com.pulumi.azurenative.apimanagement.IdentityProviderArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var identityProvider = new IdentityProvider("identityProvider", IdentityProviderArgs.builder()
* .clientId("facebookid")
* .clientSecret("facebookapplicationsecret")
* .identityProviderName("facebook")
* .resourceGroupName("rg1")
* .serviceName("apimService1")
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:apimanagement:IdentityProvider Facebook /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.ApiManagement/service/{serviceName}/identityProviders/{identityProviderName}
* ```
*/
public class IdentityProvider internal constructor(
override val javaResource: com.pulumi.azurenative.apimanagement.IdentityProvider,
) : KotlinCustomResource(javaResource, IdentityProviderMapper) {
/**
* List of Allowed Tenants when configuring Azure Active Directory login.
*/
public val allowedTenants: Output>?
get() = javaResource.allowedTenants().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
/**
* OpenID Connect discovery endpoint hostname for AAD or AAD B2C.
*/
public val authority: Output?
get() = javaResource.authority().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Client Id of the Application in the external Identity Provider. It is App ID for Facebook login, Client ID for Google login, App ID for Microsoft.
*/
public val clientId: Output
get() = javaResource.clientId().applyValue({ args0 -> args0 })
/**
* The client library to be used in the developer portal. Only applies to AAD and AAD B2C Identity Provider.
*/
public val clientLibrary: Output?
get() = javaResource.clientLibrary().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Client secret of the Application in external Identity Provider, used to authenticate login request. For example, it is App Secret for Facebook login, API Key for Google login, Public Key for Microsoft. This property will not be filled on 'GET' operations! Use '/listSecrets' POST request to get the value.
*/
public val clientSecret: Output?
get() = javaResource.clientSecret().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The name of the resource
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* Password Reset Policy Name. Only applies to AAD B2C Identity Provider.
*/
public val passwordResetPolicyName: Output?
get() = javaResource.passwordResetPolicyName().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Profile Editing Policy Name. Only applies to AAD B2C Identity Provider.
*/
public val profileEditingPolicyName: Output?
get() = javaResource.profileEditingPolicyName().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Signin Policy Name. Only applies to AAD B2C Identity Provider.
*/
public val signinPolicyName: Output?
get() = javaResource.signinPolicyName().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The TenantId to use instead of Common when logging into Active Directory
*/
public val signinTenant: Output?
get() = javaResource.signinTenant().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Signup Policy Name. Only applies to AAD B2C Identity Provider.
*/
public val signupPolicyName: Output?
get() = javaResource.signupPolicyName().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*/
public val type: Output
get() = javaResource.type().applyValue({ args0 -> args0 })
}
public object IdentityProviderMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.azurenative.apimanagement.IdentityProvider::class == javaResource::class
override fun map(javaResource: Resource): IdentityProvider = IdentityProvider(
javaResource as
com.pulumi.azurenative.apimanagement.IdentityProvider,
)
}
/**
* @see [IdentityProvider].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [IdentityProvider].
*/
public suspend fun identityProvider(
name: String,
block: suspend IdentityProviderResourceBuilder.() -> Unit,
): IdentityProvider {
val builder = IdentityProviderResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [IdentityProvider].
* @param name The _unique_ name of the resulting resource.
*/
public fun identityProvider(name: String): IdentityProvider {
val builder = IdentityProviderResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy