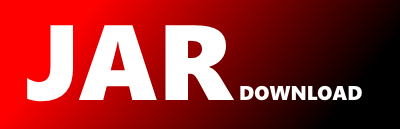
com.pulumi.azurenative.apimanagement.kotlin.SubscriptionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy