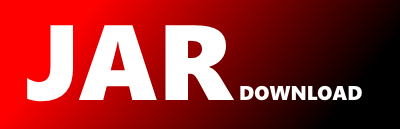
com.pulumi.azurenative.apimanagement.kotlin.WorkspaceApiDiagnosticArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.apimanagement.kotlin
import com.pulumi.azurenative.apimanagement.WorkspaceApiDiagnosticArgs.builder
import com.pulumi.azurenative.apimanagement.kotlin.enums.AlwaysLog
import com.pulumi.azurenative.apimanagement.kotlin.enums.HttpCorrelationProtocol
import com.pulumi.azurenative.apimanagement.kotlin.enums.OperationNameFormat
import com.pulumi.azurenative.apimanagement.kotlin.enums.Verbosity
import com.pulumi.azurenative.apimanagement.kotlin.inputs.PipelineDiagnosticSettingsArgs
import com.pulumi.azurenative.apimanagement.kotlin.inputs.PipelineDiagnosticSettingsArgsBuilder
import com.pulumi.azurenative.apimanagement.kotlin.inputs.SamplingSettingsArgs
import com.pulumi.azurenative.apimanagement.kotlin.inputs.SamplingSettingsArgsBuilder
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Diagnostic details.
* Azure REST API version: 2023-09-01-preview.
* Other available API versions: 2024-05-01.
* ## Example Usage
* ### ApiManagementCreateWorkspaceApiDiagnostic
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var workspaceApiDiagnostic = new AzureNative.ApiManagement.WorkspaceApiDiagnostic("workspaceApiDiagnostic", new()
* {
* AlwaysLog = AzureNative.ApiManagement.AlwaysLog.AllErrors,
* ApiId = "57d1f7558aa04f15146d9d8a",
* Backend = new AzureNative.ApiManagement.Inputs.PipelineDiagnosticSettingsArgs
* {
* Request = new AzureNative.ApiManagement.Inputs.HttpMessageDiagnosticArgs
* {
* Body = new AzureNative.ApiManagement.Inputs.BodyDiagnosticSettingsArgs
* {
* Bytes = 512,
* },
* Headers = new[]
* {
* "Content-type",
* },
* },
* Response = new AzureNative.ApiManagement.Inputs.HttpMessageDiagnosticArgs
* {
* Body = new AzureNative.ApiManagement.Inputs.BodyDiagnosticSettingsArgs
* {
* Bytes = 512,
* },
* Headers = new[]
* {
* "Content-type",
* },
* },
* },
* DiagnosticId = "applicationinsights",
* Frontend = new AzureNative.ApiManagement.Inputs.PipelineDiagnosticSettingsArgs
* {
* Request = new AzureNative.ApiManagement.Inputs.HttpMessageDiagnosticArgs
* {
* Body = new AzureNative.ApiManagement.Inputs.BodyDiagnosticSettingsArgs
* {
* Bytes = 512,
* },
* Headers = new[]
* {
* "Content-type",
* },
* },
* Response = new AzureNative.ApiManagement.Inputs.HttpMessageDiagnosticArgs
* {
* Body = new AzureNative.ApiManagement.Inputs.BodyDiagnosticSettingsArgs
* {
* Bytes = 512,
* },
* Headers = new[]
* {
* "Content-type",
* },
* },
* },
* LoggerId = "/workspaces/wks1/loggers/applicationinsights",
* ResourceGroupName = "rg1",
* Sampling = new AzureNative.ApiManagement.Inputs.SamplingSettingsArgs
* {
* Percentage = 50,
* SamplingType = AzureNative.ApiManagement.SamplingType.@Fixed,
* },
* ServiceName = "apimService1",
* WorkspaceId = "wks1",
* });
* });
* ```
* ```go
* package main
* import (
* apimanagement "github.com/pulumi/pulumi-azure-native-sdk/apimanagement/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := apimanagement.NewWorkspaceApiDiagnostic(ctx, "workspaceApiDiagnostic", &apimanagement.WorkspaceApiDiagnosticArgs{
* AlwaysLog: pulumi.String(apimanagement.AlwaysLogAllErrors),
* ApiId: pulumi.String("57d1f7558aa04f15146d9d8a"),
* Backend: &apimanagement.PipelineDiagnosticSettingsArgs{
* Request: &apimanagement.HttpMessageDiagnosticArgs{
* Body: &apimanagement.BodyDiagnosticSettingsArgs{
* Bytes: pulumi.Int(512),
* },
* Headers: pulumi.StringArray{
* pulumi.String("Content-type"),
* },
* },
* Response: &apimanagement.HttpMessageDiagnosticArgs{
* Body: &apimanagement.BodyDiagnosticSettingsArgs{
* Bytes: pulumi.Int(512),
* },
* Headers: pulumi.StringArray{
* pulumi.String("Content-type"),
* },
* },
* },
* DiagnosticId: pulumi.String("applicationinsights"),
* Frontend: &apimanagement.PipelineDiagnosticSettingsArgs{
* Request: &apimanagement.HttpMessageDiagnosticArgs{
* Body: &apimanagement.BodyDiagnosticSettingsArgs{
* Bytes: pulumi.Int(512),
* },
* Headers: pulumi.StringArray{
* pulumi.String("Content-type"),
* },
* },
* Response: &apimanagement.HttpMessageDiagnosticArgs{
* Body: &apimanagement.BodyDiagnosticSettingsArgs{
* Bytes: pulumi.Int(512),
* },
* Headers: pulumi.StringArray{
* pulumi.String("Content-type"),
* },
* },
* },
* LoggerId: pulumi.String("/workspaces/wks1/loggers/applicationinsights"),
* ResourceGroupName: pulumi.String("rg1"),
* Sampling: &apimanagement.SamplingSettingsArgs{
* Percentage: pulumi.Float64(50),
* SamplingType: pulumi.String(apimanagement.SamplingTypeFixed),
* },
* ServiceName: pulumi.String("apimService1"),
* WorkspaceId: pulumi.String("wks1"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.apimanagement.WorkspaceApiDiagnostic;
* import com.pulumi.azurenative.apimanagement.WorkspaceApiDiagnosticArgs;
* import com.pulumi.azurenative.apimanagement.inputs.PipelineDiagnosticSettingsArgs;
* import com.pulumi.azurenative.apimanagement.inputs.HttpMessageDiagnosticArgs;
* import com.pulumi.azurenative.apimanagement.inputs.BodyDiagnosticSettingsArgs;
* import com.pulumi.azurenative.apimanagement.inputs.SamplingSettingsArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var workspaceApiDiagnostic = new WorkspaceApiDiagnostic("workspaceApiDiagnostic", WorkspaceApiDiagnosticArgs.builder()
* .alwaysLog("allErrors")
* .apiId("57d1f7558aa04f15146d9d8a")
* .backend(PipelineDiagnosticSettingsArgs.builder()
* .request(HttpMessageDiagnosticArgs.builder()
* .body(BodyDiagnosticSettingsArgs.builder()
* .bytes(512)
* .build())
* .headers("Content-type")
* .build())
* .response(HttpMessageDiagnosticArgs.builder()
* .body(BodyDiagnosticSettingsArgs.builder()
* .bytes(512)
* .build())
* .headers("Content-type")
* .build())
* .build())
* .diagnosticId("applicationinsights")
* .frontend(PipelineDiagnosticSettingsArgs.builder()
* .request(HttpMessageDiagnosticArgs.builder()
* .body(BodyDiagnosticSettingsArgs.builder()
* .bytes(512)
* .build())
* .headers("Content-type")
* .build())
* .response(HttpMessageDiagnosticArgs.builder()
* .body(BodyDiagnosticSettingsArgs.builder()
* .bytes(512)
* .build())
* .headers("Content-type")
* .build())
* .build())
* .loggerId("/workspaces/wks1/loggers/applicationinsights")
* .resourceGroupName("rg1")
* .sampling(SamplingSettingsArgs.builder()
* .percentage(50)
* .samplingType("fixed")
* .build())
* .serviceName("apimService1")
* .workspaceId("wks1")
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:apimanagement:WorkspaceApiDiagnostic applicationinsights /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.ApiManagement/service/{serviceName}/workspaces/{workspaceId}/apis/{apiId}/diagnostics/{diagnosticId}
* ```
* @property alwaysLog Specifies for what type of messages sampling settings should not apply.
* @property apiId API identifier. Must be unique in the current API Management service instance.
* @property backend Diagnostic settings for incoming/outgoing HTTP messages to the Backend
* @property diagnosticId Diagnostic identifier. Must be unique in the current API Management service instance.
* @property frontend Diagnostic settings for incoming/outgoing HTTP messages to the Gateway.
* @property httpCorrelationProtocol Sets correlation protocol to use for Application Insights diagnostics.
* @property logClientIp Log the ClientIP. Default is false.
* @property loggerId Resource Id of a target logger.
* @property metrics Emit custom metrics via emit-metric policy. Applicable only to Application Insights diagnostic settings.
* @property operationNameFormat The format of the Operation Name for Application Insights telemetries. Default is Name.
* @property resourceGroupName The name of the resource group. The name is case insensitive.
* @property sampling Sampling settings for Diagnostic.
* @property serviceName The name of the API Management service.
* @property verbosity The verbosity level applied to traces emitted by trace policies.
* @property workspaceId Workspace identifier. Must be unique in the current API Management service instance.
*/
public data class WorkspaceApiDiagnosticArgs(
public val alwaysLog: Output>? = null,
public val apiId: Output? = null,
public val backend: Output? = null,
public val diagnosticId: Output? = null,
public val frontend: Output? = null,
public val httpCorrelationProtocol: Output>? = null,
public val logClientIp: Output? = null,
public val loggerId: Output? = null,
public val metrics: Output? = null,
public val operationNameFormat: Output>? = null,
public val resourceGroupName: Output? = null,
public val sampling: Output? = null,
public val serviceName: Output? = null,
public val verbosity: Output>? = null,
public val workspaceId: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.apimanagement.WorkspaceApiDiagnosticArgs =
com.pulumi.azurenative.apimanagement.WorkspaceApiDiagnosticArgs.builder()
.alwaysLog(
alwaysLog?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.apiId(apiId?.applyValue({ args0 -> args0 }))
.backend(backend?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.diagnosticId(diagnosticId?.applyValue({ args0 -> args0 }))
.frontend(frontend?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.httpCorrelationProtocol(
httpCorrelationProtocol?.applyValue({ args0 ->
args0.transform({ args0 ->
args0
}, { args0 -> args0.let({ args0 -> args0.toJava() }) })
}),
)
.logClientIp(logClientIp?.applyValue({ args0 -> args0 }))
.loggerId(loggerId?.applyValue({ args0 -> args0 }))
.metrics(metrics?.applyValue({ args0 -> args0 }))
.operationNameFormat(
operationNameFormat?.applyValue({ args0 ->
args0.transform(
{ args0 -> args0 },
{ args0 -> args0.let({ args0 -> args0.toJava() }) },
)
}),
)
.resourceGroupName(resourceGroupName?.applyValue({ args0 -> args0 }))
.sampling(sampling?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.serviceName(serviceName?.applyValue({ args0 -> args0 }))
.verbosity(
verbosity?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.workspaceId(workspaceId?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [WorkspaceApiDiagnosticArgs].
*/
@PulumiTagMarker
public class WorkspaceApiDiagnosticArgsBuilder internal constructor() {
private var alwaysLog: Output>? = null
private var apiId: Output? = null
private var backend: Output? = null
private var diagnosticId: Output? = null
private var frontend: Output? = null
private var httpCorrelationProtocol: Output>? = null
private var logClientIp: Output? = null
private var loggerId: Output? = null
private var metrics: Output? = null
private var operationNameFormat: Output>? = null
private var resourceGroupName: Output? = null
private var sampling: Output? = null
private var serviceName: Output? = null
private var verbosity: Output>? = null
private var workspaceId: Output? = null
/**
* @param value Specifies for what type of messages sampling settings should not apply.
*/
@JvmName("lhdfgxjhrwwvpxyd")
public suspend fun alwaysLog(`value`: Output>) {
this.alwaysLog = value
}
/**
* @param value API identifier. Must be unique in the current API Management service instance.
*/
@JvmName("kgiywlgeoohmdiuo")
public suspend fun apiId(`value`: Output) {
this.apiId = value
}
/**
* @param value Diagnostic settings for incoming/outgoing HTTP messages to the Backend
*/
@JvmName("pqujceykqdtihcsk")
public suspend fun backend(`value`: Output) {
this.backend = value
}
/**
* @param value Diagnostic identifier. Must be unique in the current API Management service instance.
*/
@JvmName("iacfakltcsjkdvqw")
public suspend fun diagnosticId(`value`: Output) {
this.diagnosticId = value
}
/**
* @param value Diagnostic settings for incoming/outgoing HTTP messages to the Gateway.
*/
@JvmName("wdmpdrvgpeuhrfup")
public suspend fun frontend(`value`: Output) {
this.frontend = value
}
/**
* @param value Sets correlation protocol to use for Application Insights diagnostics.
*/
@JvmName("gvtqurvwwsniwgno")
public suspend fun httpCorrelationProtocol(`value`: Output>) {
this.httpCorrelationProtocol = value
}
/**
* @param value Log the ClientIP. Default is false.
*/
@JvmName("danmfvasoaqbydpj")
public suspend fun logClientIp(`value`: Output) {
this.logClientIp = value
}
/**
* @param value Resource Id of a target logger.
*/
@JvmName("uvgbfvufddueoqcw")
public suspend fun loggerId(`value`: Output) {
this.loggerId = value
}
/**
* @param value Emit custom metrics via emit-metric policy. Applicable only to Application Insights diagnostic settings.
*/
@JvmName("bnaswrysxkicpqmb")
public suspend fun metrics(`value`: Output) {
this.metrics = value
}
/**
* @param value The format of the Operation Name for Application Insights telemetries. Default is Name.
*/
@JvmName("dimcacgytrrofobl")
public suspend fun operationNameFormat(`value`: Output>) {
this.operationNameFormat = value
}
/**
* @param value The name of the resource group. The name is case insensitive.
*/
@JvmName("soswwbnjihupqaca")
public suspend fun resourceGroupName(`value`: Output) {
this.resourceGroupName = value
}
/**
* @param value Sampling settings for Diagnostic.
*/
@JvmName("mwlmgefxhejqybst")
public suspend fun sampling(`value`: Output) {
this.sampling = value
}
/**
* @param value The name of the API Management service.
*/
@JvmName("kpjhmxhtdvbvrula")
public suspend fun serviceName(`value`: Output) {
this.serviceName = value
}
/**
* @param value The verbosity level applied to traces emitted by trace policies.
*/
@JvmName("ksbjxavnnadwudaj")
public suspend fun verbosity(`value`: Output>) {
this.verbosity = value
}
/**
* @param value Workspace identifier. Must be unique in the current API Management service instance.
*/
@JvmName("owuanxfqsgboirct")
public suspend fun workspaceId(`value`: Output) {
this.workspaceId = value
}
/**
* @param value Specifies for what type of messages sampling settings should not apply.
*/
@JvmName("gocbpaiivwhnfvkr")
public suspend fun alwaysLog(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.alwaysLog = mapped
}
/**
* @param value Specifies for what type of messages sampling settings should not apply.
*/
@JvmName("pxujvrojcmhlouyn")
public fun alwaysLog(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.alwaysLog = mapped
}
/**
* @param value Specifies for what type of messages sampling settings should not apply.
*/
@JvmName("fgxuqaxemqnmsgdp")
public fun alwaysLog(`value`: AlwaysLog) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.alwaysLog = mapped
}
/**
* @param value API identifier. Must be unique in the current API Management service instance.
*/
@JvmName("cwtyhuqegggysict")
public suspend fun apiId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.apiId = mapped
}
/**
* @param value Diagnostic settings for incoming/outgoing HTTP messages to the Backend
*/
@JvmName("tnvqhtgvxcynyawu")
public suspend fun backend(`value`: PipelineDiagnosticSettingsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.backend = mapped
}
/**
* @param argument Diagnostic settings for incoming/outgoing HTTP messages to the Backend
*/
@JvmName("xammxlyvrlpkbmnf")
public suspend fun backend(argument: suspend PipelineDiagnosticSettingsArgsBuilder.() -> Unit) {
val toBeMapped = PipelineDiagnosticSettingsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.backend = mapped
}
/**
* @param value Diagnostic identifier. Must be unique in the current API Management service instance.
*/
@JvmName("hivxmyxbmkywjboj")
public suspend fun diagnosticId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.diagnosticId = mapped
}
/**
* @param value Diagnostic settings for incoming/outgoing HTTP messages to the Gateway.
*/
@JvmName("tuonxxbfwhwhwwfa")
public suspend fun frontend(`value`: PipelineDiagnosticSettingsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.frontend = mapped
}
/**
* @param argument Diagnostic settings for incoming/outgoing HTTP messages to the Gateway.
*/
@JvmName("wkydqkgsmblatfuy")
public suspend fun frontend(argument: suspend PipelineDiagnosticSettingsArgsBuilder.() -> Unit) {
val toBeMapped = PipelineDiagnosticSettingsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.frontend = mapped
}
/**
* @param value Sets correlation protocol to use for Application Insights diagnostics.
*/
@JvmName("vfbvxwdrtxdnesca")
public suspend fun httpCorrelationProtocol(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.httpCorrelationProtocol = mapped
}
/**
* @param value Sets correlation protocol to use for Application Insights diagnostics.
*/
@JvmName("cqhwcruxtahegqyy")
public fun httpCorrelationProtocol(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.httpCorrelationProtocol = mapped
}
/**
* @param value Sets correlation protocol to use for Application Insights diagnostics.
*/
@JvmName("muuihmdinbqlpuco")
public fun httpCorrelationProtocol(`value`: HttpCorrelationProtocol) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.httpCorrelationProtocol = mapped
}
/**
* @param value Log the ClientIP. Default is false.
*/
@JvmName("rwrkqvelcdguivsx")
public suspend fun logClientIp(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.logClientIp = mapped
}
/**
* @param value Resource Id of a target logger.
*/
@JvmName("noliqnfmjbgesgtd")
public suspend fun loggerId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.loggerId = mapped
}
/**
* @param value Emit custom metrics via emit-metric policy. Applicable only to Application Insights diagnostic settings.
*/
@JvmName("ntxxkihlfjnblexc")
public suspend fun metrics(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.metrics = mapped
}
/**
* @param value The format of the Operation Name for Application Insights telemetries. Default is Name.
*/
@JvmName("iwpncnwwdtrnlpgt")
public suspend fun operationNameFormat(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.operationNameFormat = mapped
}
/**
* @param value The format of the Operation Name for Application Insights telemetries. Default is Name.
*/
@JvmName("vvmkbukjnafqayrl")
public fun operationNameFormat(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.operationNameFormat = mapped
}
/**
* @param value The format of the Operation Name for Application Insights telemetries. Default is Name.
*/
@JvmName("gowuqkbamgyioopt")
public fun operationNameFormat(`value`: OperationNameFormat) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.operationNameFormat = mapped
}
/**
* @param value The name of the resource group. The name is case insensitive.
*/
@JvmName("kunrescdkjxyytkq")
public suspend fun resourceGroupName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resourceGroupName = mapped
}
/**
* @param value Sampling settings for Diagnostic.
*/
@JvmName("wcqcacpweglhtgdj")
public suspend fun sampling(`value`: SamplingSettingsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sampling = mapped
}
/**
* @param argument Sampling settings for Diagnostic.
*/
@JvmName("nighusbentbuwyee")
public suspend fun sampling(argument: suspend SamplingSettingsArgsBuilder.() -> Unit) {
val toBeMapped = SamplingSettingsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.sampling = mapped
}
/**
* @param value The name of the API Management service.
*/
@JvmName("emajvfjcmthyucrm")
public suspend fun serviceName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.serviceName = mapped
}
/**
* @param value The verbosity level applied to traces emitted by trace policies.
*/
@JvmName("otxnkvpyoqemvvaq")
public suspend fun verbosity(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.verbosity = mapped
}
/**
* @param value The verbosity level applied to traces emitted by trace policies.
*/
@JvmName("ahuivvqvxtccdfke")
public fun verbosity(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.verbosity = mapped
}
/**
* @param value The verbosity level applied to traces emitted by trace policies.
*/
@JvmName("eprtupwbhvxdibwj")
public fun verbosity(`value`: Verbosity) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.verbosity = mapped
}
/**
* @param value Workspace identifier. Must be unique in the current API Management service instance.
*/
@JvmName("ailqlohxpkxkuvmo")
public suspend fun workspaceId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.workspaceId = mapped
}
internal fun build(): WorkspaceApiDiagnosticArgs = WorkspaceApiDiagnosticArgs(
alwaysLog = alwaysLog,
apiId = apiId,
backend = backend,
diagnosticId = diagnosticId,
frontend = frontend,
httpCorrelationProtocol = httpCorrelationProtocol,
logClientIp = logClientIp,
loggerId = loggerId,
metrics = metrics,
operationNameFormat = operationNameFormat,
resourceGroupName = resourceGroupName,
sampling = sampling,
serviceName = serviceName,
verbosity = verbosity,
workspaceId = workspaceId,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy