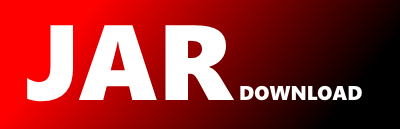
com.pulumi.azurenative.apimanagement.kotlin.outputs.GetWorkspaceApiResult.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.apimanagement.kotlin.outputs
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
* API details.
* @property apiRevision Describes the revision of the API. If no value is provided, default revision 1 is created
* @property apiRevisionDescription Description of the API Revision.
* @property apiType Type of API.
* @property apiVersion Indicates the version identifier of the API if the API is versioned
* @property apiVersionDescription Description of the API Version.
* @property apiVersionSet Version set details
* @property apiVersionSetId A resource identifier for the related ApiVersionSet.
* @property authenticationSettings Collection of authentication settings included into this API.
* @property contact Contact information for the API.
* @property description Description of the API. May include HTML formatting tags.
* @property displayName API name. Must be 1 to 300 characters long.
* @property id Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
* @property isCurrent Indicates if API revision is current api revision.
* @property isOnline Indicates if API revision is accessible via the gateway.
* @property license License information for the API.
* @property name The name of the resource
* @property path Relative URL uniquely identifying this API and all of its resource paths within the API Management service instance. It is appended to the API endpoint base URL specified during the service instance creation to form a public URL for this API.
* @property protocols Describes on which protocols the operations in this API can be invoked.
* @property serviceUrl Absolute URL of the backend service implementing this API. Cannot be more than 2000 characters long.
* @property sourceApiId API identifier of the source API.
* @property subscriptionKeyParameterNames Protocols over which API is made available.
* @property subscriptionRequired Specifies whether an API or Product subscription is required for accessing the API.
* @property termsOfServiceUrl A URL to the Terms of Service for the API. MUST be in the format of a URL.
* @property type The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*/
public data class GetWorkspaceApiResult(
public val apiRevision: String? = null,
public val apiRevisionDescription: String? = null,
public val apiType: String? = null,
public val apiVersion: String? = null,
public val apiVersionDescription: String? = null,
public val apiVersionSet: ApiVersionSetContractDetailsResponse? = null,
public val apiVersionSetId: String? = null,
public val authenticationSettings: AuthenticationSettingsContractResponse? = null,
public val contact: ApiContactInformationResponse? = null,
public val description: String? = null,
public val displayName: String? = null,
public val id: String,
public val isCurrent: Boolean? = null,
public val isOnline: Boolean,
public val license: ApiLicenseInformationResponse? = null,
public val name: String,
public val path: String,
public val protocols: List? = null,
public val serviceUrl: String? = null,
public val sourceApiId: String? = null,
public val subscriptionKeyParameterNames: SubscriptionKeyParameterNamesContractResponse? = null,
public val subscriptionRequired: Boolean? = null,
public val termsOfServiceUrl: String? = null,
public val type: String,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.azurenative.apimanagement.outputs.GetWorkspaceApiResult): GetWorkspaceApiResult = GetWorkspaceApiResult(
apiRevision = javaType.apiRevision().map({ args0 -> args0 }).orElse(null),
apiRevisionDescription = javaType.apiRevisionDescription().map({ args0 -> args0 }).orElse(null),
apiType = javaType.apiType().map({ args0 -> args0 }).orElse(null),
apiVersion = javaType.apiVersion().map({ args0 -> args0 }).orElse(null),
apiVersionDescription = javaType.apiVersionDescription().map({ args0 -> args0 }).orElse(null),
apiVersionSet = javaType.apiVersionSet().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.apimanagement.kotlin.outputs.ApiVersionSetContractDetailsResponse.Companion.toKotlin(args0)
})
}).orElse(null),
apiVersionSetId = javaType.apiVersionSetId().map({ args0 -> args0 }).orElse(null),
authenticationSettings = javaType.authenticationSettings().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.apimanagement.kotlin.outputs.AuthenticationSettingsContractResponse.Companion.toKotlin(args0)
})
}).orElse(null),
contact = javaType.contact().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.apimanagement.kotlin.outputs.ApiContactInformationResponse.Companion.toKotlin(args0)
})
}).orElse(null),
description = javaType.description().map({ args0 -> args0 }).orElse(null),
displayName = javaType.displayName().map({ args0 -> args0 }).orElse(null),
id = javaType.id(),
isCurrent = javaType.isCurrent().map({ args0 -> args0 }).orElse(null),
isOnline = javaType.isOnline(),
license = javaType.license().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.apimanagement.kotlin.outputs.ApiLicenseInformationResponse.Companion.toKotlin(args0)
})
}).orElse(null),
name = javaType.name(),
path = javaType.path(),
protocols = javaType.protocols().map({ args0 -> args0 }),
serviceUrl = javaType.serviceUrl().map({ args0 -> args0 }).orElse(null),
sourceApiId = javaType.sourceApiId().map({ args0 -> args0 }).orElse(null),
subscriptionKeyParameterNames = javaType.subscriptionKeyParameterNames().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.apimanagement.kotlin.outputs.SubscriptionKeyParameterNamesContractResponse.Companion.toKotlin(args0)
})
}).orElse(null),
subscriptionRequired = javaType.subscriptionRequired().map({ args0 -> args0 }).orElse(null),
termsOfServiceUrl = javaType.termsOfServiceUrl().map({ args0 -> args0 }).orElse(null),
type = javaType.type(),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy