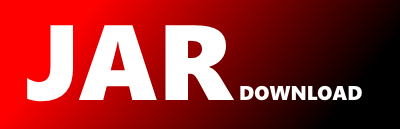
com.pulumi.azurenative.app.kotlin.DotNetComponentArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.app.kotlin
import com.pulumi.azurenative.app.DotNetComponentArgs.builder
import com.pulumi.azurenative.app.kotlin.enums.DotNetComponentType
import com.pulumi.azurenative.app.kotlin.inputs.DotNetComponentConfigurationPropertyArgs
import com.pulumi.azurenative.app.kotlin.inputs.DotNetComponentConfigurationPropertyArgsBuilder
import com.pulumi.azurenative.app.kotlin.inputs.DotNetComponentServiceBindArgs
import com.pulumi.azurenative.app.kotlin.inputs.DotNetComponentServiceBindArgsBuilder
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* .NET Component.
* Azure REST API version: 2023-11-02-preview.
* Other available API versions: 2024-02-02-preview.
* ## Example Usage
* ### Create or Update .NET Component
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var dotNetComponent = new AzureNative.App.DotNetComponent("dotNetComponent", new()
* {
* ComponentType = AzureNative.App.DotNetComponentType.AspireDashboard,
* Configurations = new[]
* {
* new AzureNative.App.Inputs.DotNetComponentConfigurationPropertyArgs
* {
* PropertyName = "dashboard-theme",
* Value = "dark",
* },
* },
* EnvironmentName = "myenvironment",
* Name = "mydotnetcomponent",
* ResourceGroupName = "examplerg",
* });
* });
* ```
* ```go
* package main
* import (
* app "github.com/pulumi/pulumi-azure-native-sdk/app/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := app.NewDotNetComponent(ctx, "dotNetComponent", &app.DotNetComponentArgs{
* ComponentType: pulumi.String(app.DotNetComponentTypeAspireDashboard),
* Configurations: app.DotNetComponentConfigurationPropertyArray{
* &app.DotNetComponentConfigurationPropertyArgs{
* PropertyName: pulumi.String("dashboard-theme"),
* Value: pulumi.String("dark"),
* },
* },
* EnvironmentName: pulumi.String("myenvironment"),
* Name: pulumi.String("mydotnetcomponent"),
* ResourceGroupName: pulumi.String("examplerg"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.app.DotNetComponent;
* import com.pulumi.azurenative.app.DotNetComponentArgs;
* import com.pulumi.azurenative.app.inputs.DotNetComponentConfigurationPropertyArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var dotNetComponent = new DotNetComponent("dotNetComponent", DotNetComponentArgs.builder()
* .componentType("AspireDashboard")
* .configurations(DotNetComponentConfigurationPropertyArgs.builder()
* .propertyName("dashboard-theme")
* .value("dark")
* .build())
* .environmentName("myenvironment")
* .name("mydotnetcomponent")
* .resourceGroupName("examplerg")
* .build());
* }
* }
* ```
* ### Create or Update .NET Component with ServiceBinds
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var dotNetComponent = new AzureNative.App.DotNetComponent("dotNetComponent", new()
* {
* ComponentType = AzureNative.App.DotNetComponentType.AspireDashboard,
* Configurations = new[]
* {
* new AzureNative.App.Inputs.DotNetComponentConfigurationPropertyArgs
* {
* PropertyName = "dashboard-theme",
* Value = "dark",
* },
* },
* EnvironmentName = "myenvironment",
* Name = "mydotnetcomponent",
* ResourceGroupName = "examplerg",
* ServiceBinds = new[]
* {
* new AzureNative.App.Inputs.DotNetComponentServiceBindArgs
* {
* Name = "yellowcat",
* ServiceId = "/subscriptions/8efdecc5-919e-44eb-b179-915dca89ebf9/resourceGroups/examplerg/providers/Microsoft.App/managedEnvironments/myenvironment/dotNetComponents/yellowcat",
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* app "github.com/pulumi/pulumi-azure-native-sdk/app/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := app.NewDotNetComponent(ctx, "dotNetComponent", &app.DotNetComponentArgs{
* ComponentType: pulumi.String(app.DotNetComponentTypeAspireDashboard),
* Configurations: app.DotNetComponentConfigurationPropertyArray{
* &app.DotNetComponentConfigurationPropertyArgs{
* PropertyName: pulumi.String("dashboard-theme"),
* Value: pulumi.String("dark"),
* },
* },
* EnvironmentName: pulumi.String("myenvironment"),
* Name: pulumi.String("mydotnetcomponent"),
* ResourceGroupName: pulumi.String("examplerg"),
* ServiceBinds: app.DotNetComponentServiceBindArray{
* &app.DotNetComponentServiceBindArgs{
* Name: pulumi.String("yellowcat"),
* ServiceId: pulumi.String("/subscriptions/8efdecc5-919e-44eb-b179-915dca89ebf9/resourceGroups/examplerg/providers/Microsoft.App/managedEnvironments/myenvironment/dotNetComponents/yellowcat"),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.app.DotNetComponent;
* import com.pulumi.azurenative.app.DotNetComponentArgs;
* import com.pulumi.azurenative.app.inputs.DotNetComponentConfigurationPropertyArgs;
* import com.pulumi.azurenative.app.inputs.DotNetComponentServiceBindArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var dotNetComponent = new DotNetComponent("dotNetComponent", DotNetComponentArgs.builder()
* .componentType("AspireDashboard")
* .configurations(DotNetComponentConfigurationPropertyArgs.builder()
* .propertyName("dashboard-theme")
* .value("dark")
* .build())
* .environmentName("myenvironment")
* .name("mydotnetcomponent")
* .resourceGroupName("examplerg")
* .serviceBinds(DotNetComponentServiceBindArgs.builder()
* .name("yellowcat")
* .serviceId("/subscriptions/8efdecc5-919e-44eb-b179-915dca89ebf9/resourceGroups/examplerg/providers/Microsoft.App/managedEnvironments/myenvironment/dotNetComponents/yellowcat")
* .build())
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:app:DotNetComponent mydotnetcomponent /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.App/managedEnvironments/{environmentName}/dotNetComponents/{name}
* ```
* @property componentType Type of the .NET Component.
* @property configurations List of .NET Components configuration properties
* @property environmentName Name of the Managed Environment.
* @property name Name of the .NET Component.
* @property resourceGroupName The name of the resource group. The name is case insensitive.
* @property serviceBinds List of .NET Components that are bound to the .NET component
*/
public data class DotNetComponentArgs(
public val componentType: Output>? = null,
public val configurations: Output>? = null,
public val environmentName: Output? = null,
public val name: Output? = null,
public val resourceGroupName: Output? = null,
public val serviceBinds: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.app.DotNetComponentArgs =
com.pulumi.azurenative.app.DotNetComponentArgs.builder()
.componentType(
componentType?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.configurations(
configurations?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.environmentName(environmentName?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.resourceGroupName(resourceGroupName?.applyValue({ args0 -> args0 }))
.serviceBinds(
serviceBinds?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
).build()
}
/**
* Builder for [DotNetComponentArgs].
*/
@PulumiTagMarker
public class DotNetComponentArgsBuilder internal constructor() {
private var componentType: Output>? = null
private var configurations: Output>? = null
private var environmentName: Output? = null
private var name: Output? = null
private var resourceGroupName: Output? = null
private var serviceBinds: Output>? = null
/**
* @param value Type of the .NET Component.
*/
@JvmName("ficwihqetjaprurp")
public suspend fun componentType(`value`: Output>) {
this.componentType = value
}
/**
* @param value List of .NET Components configuration properties
*/
@JvmName("jmaimhyxqeahuyrq")
public suspend fun configurations(`value`: Output>) {
this.configurations = value
}
@JvmName("sqbcrcxghhswdvch")
public suspend fun configurations(vararg values: Output) {
this.configurations = Output.all(values.asList())
}
/**
* @param values List of .NET Components configuration properties
*/
@JvmName("kjpycixjqcrnrrii")
public suspend fun configurations(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy