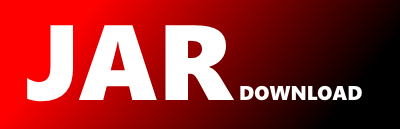
com.pulumi.azurenative.app.kotlin.inputs.VnetConfigurationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.app.kotlin.inputs
import com.pulumi.azurenative.app.inputs.VnetConfigurationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Configuration properties for apps environment to join a Virtual Network
* @property dockerBridgeCidr CIDR notation IP range assigned to the Docker bridge, network. Must not overlap with any other provided IP ranges.
* @property infrastructureSubnetId Resource ID of a subnet for infrastructure components. This subnet must be in the same VNET as the subnet defined in runtimeSubnetId. Must not overlap with any other provided IP ranges.
* @property internal Boolean indicating the environment only has an internal load balancer. These environments do not have a public static IP resource. They must provide runtimeSubnetId and infrastructureSubnetId if enabling this property
* @property outboundSettings Configuration used to control the Environment Egress outbound traffic
* @property platformReservedCidr IP range in CIDR notation that can be reserved for environment infrastructure IP addresses. Must not overlap with any other provided IP ranges.
* @property platformReservedDnsIP An IP address from the IP range defined by platformReservedCidr that will be reserved for the internal DNS server.
* @property runtimeSubnetId This field is deprecated and not used. If you wish to provide your own subnet that Container App containers are injected into, then you should leverage the infrastructureSubnetId.
*/
public data class VnetConfigurationArgs(
public val dockerBridgeCidr: Output? = null,
public val infrastructureSubnetId: Output? = null,
public val `internal`: Output? = null,
public val outboundSettings: Output? = null,
public val platformReservedCidr: Output? = null,
public val platformReservedDnsIP: Output? = null,
public val runtimeSubnetId: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.app.inputs.VnetConfigurationArgs =
com.pulumi.azurenative.app.inputs.VnetConfigurationArgs.builder()
.dockerBridgeCidr(dockerBridgeCidr?.applyValue({ args0 -> args0 }))
.infrastructureSubnetId(infrastructureSubnetId?.applyValue({ args0 -> args0 }))
.`internal`(`internal`?.applyValue({ args0 -> args0 }))
.outboundSettings(outboundSettings?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.platformReservedCidr(platformReservedCidr?.applyValue({ args0 -> args0 }))
.platformReservedDnsIP(platformReservedDnsIP?.applyValue({ args0 -> args0 }))
.runtimeSubnetId(runtimeSubnetId?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [VnetConfigurationArgs].
*/
@PulumiTagMarker
public class VnetConfigurationArgsBuilder internal constructor() {
private var dockerBridgeCidr: Output? = null
private var infrastructureSubnetId: Output? = null
private var `internal`: Output? = null
private var outboundSettings: Output? = null
private var platformReservedCidr: Output? = null
private var platformReservedDnsIP: Output? = null
private var runtimeSubnetId: Output? = null
/**
* @param value CIDR notation IP range assigned to the Docker bridge, network. Must not overlap with any other provided IP ranges.
*/
@JvmName("wfggkukenbkdujyi")
public suspend fun dockerBridgeCidr(`value`: Output) {
this.dockerBridgeCidr = value
}
/**
* @param value Resource ID of a subnet for infrastructure components. This subnet must be in the same VNET as the subnet defined in runtimeSubnetId. Must not overlap with any other provided IP ranges.
*/
@JvmName("lkqatbjmkkphtxke")
public suspend fun infrastructureSubnetId(`value`: Output) {
this.infrastructureSubnetId = value
}
/**
* @param value Boolean indicating the environment only has an internal load balancer. These environments do not have a public static IP resource. They must provide runtimeSubnetId and infrastructureSubnetId if enabling this property
*/
@JvmName("nhkuaruafsouwpbv")
public suspend fun `internal`(`value`: Output) {
this.`internal` = value
}
/**
* @param value Configuration used to control the Environment Egress outbound traffic
*/
@JvmName("iudnmxrefkvjapfx")
public suspend fun outboundSettings(`value`: Output) {
this.outboundSettings = value
}
/**
* @param value IP range in CIDR notation that can be reserved for environment infrastructure IP addresses. Must not overlap with any other provided IP ranges.
*/
@JvmName("vmagnhsfwdeujqjj")
public suspend fun platformReservedCidr(`value`: Output) {
this.platformReservedCidr = value
}
/**
* @param value An IP address from the IP range defined by platformReservedCidr that will be reserved for the internal DNS server.
*/
@JvmName("wvniatdhhlccvgqx")
public suspend fun platformReservedDnsIP(`value`: Output) {
this.platformReservedDnsIP = value
}
/**
* @param value This field is deprecated and not used. If you wish to provide your own subnet that Container App containers are injected into, then you should leverage the infrastructureSubnetId.
*/
@JvmName("qekxpdubpokdjwoq")
public suspend fun runtimeSubnetId(`value`: Output) {
this.runtimeSubnetId = value
}
/**
* @param value CIDR notation IP range assigned to the Docker bridge, network. Must not overlap with any other provided IP ranges.
*/
@JvmName("drnfchefwwhulwei")
public suspend fun dockerBridgeCidr(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dockerBridgeCidr = mapped
}
/**
* @param value Resource ID of a subnet for infrastructure components. This subnet must be in the same VNET as the subnet defined in runtimeSubnetId. Must not overlap with any other provided IP ranges.
*/
@JvmName("ofxhbpfowmavxhdd")
public suspend fun infrastructureSubnetId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.infrastructureSubnetId = mapped
}
/**
* @param value Boolean indicating the environment only has an internal load balancer. These environments do not have a public static IP resource. They must provide runtimeSubnetId and infrastructureSubnetId if enabling this property
*/
@JvmName("aygtowxfgcfuxgjt")
public suspend fun `internal`(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.`internal` = mapped
}
/**
* @param value Configuration used to control the Environment Egress outbound traffic
*/
@JvmName("iogugxpjqjekftnv")
public suspend fun outboundSettings(`value`: ManagedEnvironmentOutboundSettingsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.outboundSettings = mapped
}
/**
* @param argument Configuration used to control the Environment Egress outbound traffic
*/
@JvmName("bqiaayksitpojwdn")
public suspend fun outboundSettings(argument: suspend ManagedEnvironmentOutboundSettingsArgsBuilder.() -> Unit) {
val toBeMapped = ManagedEnvironmentOutboundSettingsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.outboundSettings = mapped
}
/**
* @param value IP range in CIDR notation that can be reserved for environment infrastructure IP addresses. Must not overlap with any other provided IP ranges.
*/
@JvmName("tbgldtwoqjexekxq")
public suspend fun platformReservedCidr(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.platformReservedCidr = mapped
}
/**
* @param value An IP address from the IP range defined by platformReservedCidr that will be reserved for the internal DNS server.
*/
@JvmName("briekkycqrxphjee")
public suspend fun platformReservedDnsIP(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.platformReservedDnsIP = mapped
}
/**
* @param value This field is deprecated and not used. If you wish to provide your own subnet that Container App containers are injected into, then you should leverage the infrastructureSubnetId.
*/
@JvmName("pwrsbywdbepfkkfq")
public suspend fun runtimeSubnetId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.runtimeSubnetId = mapped
}
internal fun build(): VnetConfigurationArgs = VnetConfigurationArgs(
dockerBridgeCidr = dockerBridgeCidr,
infrastructureSubnetId = infrastructureSubnetId,
`internal` = `internal`,
outboundSettings = outboundSettings,
platformReservedCidr = platformReservedCidr,
platformReservedDnsIP = platformReservedDnsIP,
runtimeSubnetId = runtimeSubnetId,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy