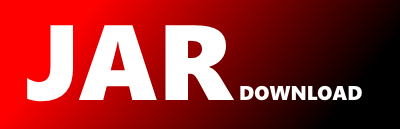
com.pulumi.azurenative.appplatform.kotlin.BuildServiceBuildArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.appplatform.kotlin
import com.pulumi.azurenative.appplatform.BuildServiceBuildArgs.builder
import com.pulumi.azurenative.appplatform.kotlin.inputs.BuildPropertiesArgs
import com.pulumi.azurenative.appplatform.kotlin.inputs.BuildPropertiesArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Build resource payload
* Azure REST API version: 2023-05-01-preview.
* Other available API versions: 2023-07-01-preview, 2023-09-01-preview, 2023-11-01-preview, 2023-12-01, 2024-01-01-preview, 2024-05-01-preview.
* ## Example Usage
* ### BuildService_CreateOrUpdateBuild
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var buildServiceBuild = new AzureNative.AppPlatform.BuildServiceBuild("buildServiceBuild", new()
* {
* BuildName = "mybuild",
* BuildServiceName = "default",
* Properties = new AzureNative.AppPlatform.Inputs.BuildPropertiesArgs
* {
* AgentPool = "/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/myResourceGroup/providers/Microsoft.AppPlatform/Spring/myservice/buildServices/default/agentPools/default",
* Apms = new[]
* {
* new AzureNative.AppPlatform.Inputs.ApmReferenceArgs
* {
* ResourceId = "/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/myResourceGroup/providers/Microsoft.AppPlatform/Spring/myservice/apms/myappinsights",
* },
* },
* Builder = "/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/myResourceGroup/providers/Microsoft.AppPlatform/Spring/myservice/buildServices/default/builders/default",
* Certificates = new[]
* {
* new AzureNative.AppPlatform.Inputs.CertificateReferenceArgs
* {
* ResourceId = "/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/myResourceGroup/providers/Microsoft.AppPlatform/Spring/myservice/certificates/mycert1",
* },
* new AzureNative.AppPlatform.Inputs.CertificateReferenceArgs
* {
* ResourceId = "/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/myResourceGroup/providers/Microsoft.AppPlatform/Spring/myservice/certificates/mycert2",
* },
* },
* Env =
* {
* { "environmentVariable", "test" },
* },
* RelativePath = "e3b0c44298fc1c149afbf4c8996fb92427ae41e4649b934ca495991b7852b855-20210601-3ed9f4a2-986b-4bbd-b833-a42dccb2f777",
* ResourceRequests = new AzureNative.AppPlatform.Inputs.BuildResourceRequestsArgs
* {
* Cpu = "1",
* Memory = "2Gi",
* },
* },
* ResourceGroupName = "myResourceGroup",
* ServiceName = "myservice",
* });
* });
* ```
* ```go
* package main
* import (
* appplatform "github.com/pulumi/pulumi-azure-native-sdk/appplatform/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := appplatform.NewBuildServiceBuild(ctx, "buildServiceBuild", &appplatform.BuildServiceBuildArgs{
* BuildName: pulumi.String("mybuild"),
* BuildServiceName: pulumi.String("default"),
* Properties: &appplatform.BuildPropertiesArgs{
* AgentPool: pulumi.String("/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/myResourceGroup/providers/Microsoft.AppPlatform/Spring/myservice/buildServices/default/agentPools/default"),
* Apms: appplatform.ApmReferenceArray{
* &appplatform.ApmReferenceArgs{
* ResourceId: pulumi.String("/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/myResourceGroup/providers/Microsoft.AppPlatform/Spring/myservice/apms/myappinsights"),
* },
* },
* Builder: pulumi.String("/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/myResourceGroup/providers/Microsoft.AppPlatform/Spring/myservice/buildServices/default/builders/default"),
* Certificates: appplatform.CertificateReferenceArray{
* &appplatform.CertificateReferenceArgs{
* ResourceId: pulumi.String("/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/myResourceGroup/providers/Microsoft.AppPlatform/Spring/myservice/certificates/mycert1"),
* },
* &appplatform.CertificateReferenceArgs{
* ResourceId: pulumi.String("/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/myResourceGroup/providers/Microsoft.AppPlatform/Spring/myservice/certificates/mycert2"),
* },
* },
* Env: pulumi.StringMap{
* "environmentVariable": pulumi.String("test"),
* },
* RelativePath: pulumi.String("e3b0c44298fc1c149afbf4c8996fb92427ae41e4649b934ca495991b7852b855-20210601-3ed9f4a2-986b-4bbd-b833-a42dccb2f777"),
* ResourceRequests: &appplatform.BuildResourceRequestsArgs{
* Cpu: pulumi.String("1"),
* Memory: pulumi.String("2Gi"),
* },
* },
* ResourceGroupName: pulumi.String("myResourceGroup"),
* ServiceName: pulumi.String("myservice"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.appplatform.BuildServiceBuild;
* import com.pulumi.azurenative.appplatform.BuildServiceBuildArgs;
* import com.pulumi.azurenative.appplatform.inputs.BuildPropertiesArgs;
* import com.pulumi.azurenative.appplatform.inputs.BuildResourceRequestsArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var buildServiceBuild = new BuildServiceBuild("buildServiceBuild", BuildServiceBuildArgs.builder()
* .buildName("mybuild")
* .buildServiceName("default")
* .properties(BuildPropertiesArgs.builder()
* .agentPool("/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/myResourceGroup/providers/Microsoft.AppPlatform/Spring/myservice/buildServices/default/agentPools/default")
* .apms(ApmReferenceArgs.builder()
* .resourceId("/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/myResourceGroup/providers/Microsoft.AppPlatform/Spring/myservice/apms/myappinsights")
* .build())
* .builder("/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/myResourceGroup/providers/Microsoft.AppPlatform/Spring/myservice/buildServices/default/builders/default")
* .certificates(
* CertificateReferenceArgs.builder()
* .resourceId("/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/myResourceGroup/providers/Microsoft.AppPlatform/Spring/myservice/certificates/mycert1")
* .build(),
* CertificateReferenceArgs.builder()
* .resourceId("/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/myResourceGroup/providers/Microsoft.AppPlatform/Spring/myservice/certificates/mycert2")
* .build())
* .env(Map.of("environmentVariable", "test"))
* .relativePath("e3b0c44298fc1c149afbf4c8996fb92427ae41e4649b934ca495991b7852b855-20210601-3ed9f4a2-986b-4bbd-b833-a42dccb2f777")
* .resourceRequests(BuildResourceRequestsArgs.builder()
* .cpu("1")
* .memory("2Gi")
* .build())
* .build())
* .resourceGroupName("myResourceGroup")
* .serviceName("myservice")
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:appplatform:BuildServiceBuild mybuild /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.AppPlatform/Spring/{serviceName}/buildServices/{buildServiceName}/builds/{buildName}
* ```
* @property buildName The name of the build resource.
* @property buildServiceName The name of the build service resource.
* @property properties Properties of the build resource
* @property resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @property serviceName The name of the Service resource.
*/
public data class BuildServiceBuildArgs(
public val buildName: Output? = null,
public val buildServiceName: Output? = null,
public val properties: Output? = null,
public val resourceGroupName: Output? = null,
public val serviceName: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.appplatform.BuildServiceBuildArgs =
com.pulumi.azurenative.appplatform.BuildServiceBuildArgs.builder()
.buildName(buildName?.applyValue({ args0 -> args0 }))
.buildServiceName(buildServiceName?.applyValue({ args0 -> args0 }))
.properties(properties?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.resourceGroupName(resourceGroupName?.applyValue({ args0 -> args0 }))
.serviceName(serviceName?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [BuildServiceBuildArgs].
*/
@PulumiTagMarker
public class BuildServiceBuildArgsBuilder internal constructor() {
private var buildName: Output? = null
private var buildServiceName: Output? = null
private var properties: Output? = null
private var resourceGroupName: Output? = null
private var serviceName: Output? = null
/**
* @param value The name of the build resource.
*/
@JvmName("xoebkjpivkeehxni")
public suspend fun buildName(`value`: Output) {
this.buildName = value
}
/**
* @param value The name of the build service resource.
*/
@JvmName("ftksorxymkussswf")
public suspend fun buildServiceName(`value`: Output) {
this.buildServiceName = value
}
/**
* @param value Properties of the build resource
*/
@JvmName("vurepuqmymtlodor")
public suspend fun properties(`value`: Output) {
this.properties = value
}
/**
* @param value The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
*/
@JvmName("vuxtdciqndjarpdb")
public suspend fun resourceGroupName(`value`: Output) {
this.resourceGroupName = value
}
/**
* @param value The name of the Service resource.
*/
@JvmName("omkxygenwriynhok")
public suspend fun serviceName(`value`: Output) {
this.serviceName = value
}
/**
* @param value The name of the build resource.
*/
@JvmName("vhvbrgwwtrvfgfrs")
public suspend fun buildName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.buildName = mapped
}
/**
* @param value The name of the build service resource.
*/
@JvmName("onqcynebfthlrene")
public suspend fun buildServiceName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.buildServiceName = mapped
}
/**
* @param value Properties of the build resource
*/
@JvmName("bobwyfjfmkcmewbm")
public suspend fun properties(`value`: BuildPropertiesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.properties = mapped
}
/**
* @param argument Properties of the build resource
*/
@JvmName("qbkhmlescvekignx")
public suspend fun properties(argument: suspend BuildPropertiesArgsBuilder.() -> Unit) {
val toBeMapped = BuildPropertiesArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.properties = mapped
}
/**
* @param value The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
*/
@JvmName("jhfghqkkjxvelhec")
public suspend fun resourceGroupName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resourceGroupName = mapped
}
/**
* @param value The name of the Service resource.
*/
@JvmName("omycnwvunidwohps")
public suspend fun serviceName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.serviceName = mapped
}
internal fun build(): BuildServiceBuildArgs = BuildServiceBuildArgs(
buildName = buildName,
buildServiceName = buildServiceName,
properties = properties,
resourceGroupName = resourceGroupName,
serviceName = serviceName,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy