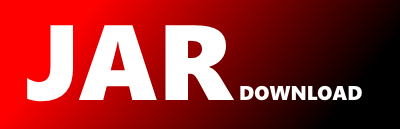
com.pulumi.azurenative.authorization.kotlin.ScopeAccessReviewHistoryDefinitionById.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.authorization.kotlin
import com.pulumi.azurenative.authorization.kotlin.outputs.AccessReviewHistoryInstanceResponse
import com.pulumi.azurenative.authorization.kotlin.outputs.AccessReviewScopeResponse
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.azurenative.authorization.kotlin.outputs.AccessReviewHistoryInstanceResponse.Companion.toKotlin as accessReviewHistoryInstanceResponseToKotlin
import com.pulumi.azurenative.authorization.kotlin.outputs.AccessReviewScopeResponse.Companion.toKotlin as accessReviewScopeResponseToKotlin
/**
* Builder for [ScopeAccessReviewHistoryDefinitionById].
*/
@PulumiTagMarker
public class ScopeAccessReviewHistoryDefinitionByIdResourceBuilder internal constructor() {
public var name: String? = null
public var args: ScopeAccessReviewHistoryDefinitionByIdArgs =
ScopeAccessReviewHistoryDefinitionByIdArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend ScopeAccessReviewHistoryDefinitionByIdArgsBuilder.() -> Unit) {
val builder = ScopeAccessReviewHistoryDefinitionByIdArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): ScopeAccessReviewHistoryDefinitionById {
val builtJavaResource =
com.pulumi.azurenative.authorization.ScopeAccessReviewHistoryDefinitionById(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return ScopeAccessReviewHistoryDefinitionById(builtJavaResource)
}
}
/**
* Access Review History Definition.
* Azure REST API version: 2021-12-01-preview. Prior API version in Azure Native 1.x: 2021-12-01-preview.
* ## Example Usage
* ### PutAccessReviewHistoryDefinition
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var scopeAccessReviewHistoryDefinitionById = new AzureNative.Authorization.ScopeAccessReviewHistoryDefinitionById("scopeAccessReviewHistoryDefinitionById", new()
* {
* HistoryDefinitionId = "44724910-d7a5-4c29-b28f-db73e717165a",
* Scope = "subscriptions/129a304b-4aea-4b86-a9f7-ba7e2b23737a",
* });
* });
* ```
* ```go
* package main
* import (
* authorization "github.com/pulumi/pulumi-azure-native-sdk/authorization/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := authorization.NewScopeAccessReviewHistoryDefinitionById(ctx, "scopeAccessReviewHistoryDefinitionById", &authorization.ScopeAccessReviewHistoryDefinitionByIdArgs{
* HistoryDefinitionId: pulumi.String("44724910-d7a5-4c29-b28f-db73e717165a"),
* Scope: pulumi.String("subscriptions/129a304b-4aea-4b86-a9f7-ba7e2b23737a"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.authorization.ScopeAccessReviewHistoryDefinitionById;
* import com.pulumi.azurenative.authorization.ScopeAccessReviewHistoryDefinitionByIdArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var scopeAccessReviewHistoryDefinitionById = new ScopeAccessReviewHistoryDefinitionById("scopeAccessReviewHistoryDefinitionById", ScopeAccessReviewHistoryDefinitionByIdArgs.builder()
* .historyDefinitionId("44724910-d7a5-4c29-b28f-db73e717165a")
* .scope("subscriptions/129a304b-4aea-4b86-a9f7-ba7e2b23737a")
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:authorization:ScopeAccessReviewHistoryDefinitionById 44724910-d7a5-4c29-b28f-db73e717165a /{scope}/providers/Microsoft.Authorization/accessReviewHistoryDefinitions/{historyDefinitionId}
* ```
*/
public class ScopeAccessReviewHistoryDefinitionById internal constructor(
override val javaResource: com.pulumi.azurenative.authorization.ScopeAccessReviewHistoryDefinitionById,
) : KotlinCustomResource(javaResource, ScopeAccessReviewHistoryDefinitionByIdMapper) {
/**
* Date time when history definition was created
*/
public val createdDateTime: Output
get() = javaResource.createdDateTime().applyValue({ args0 -> args0 })
/**
* Collection of review decisions which the history data should be filtered on. For example if Approve and Deny are supplied the data will only contain review results in which the decision maker approved or denied a review request.
*/
public val decisions: Output>?
get() = javaResource.decisions().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0
})
}).orElse(null)
})
/**
* The display name for the history definition.
*/
public val displayName: Output?
get() = javaResource.displayName().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The DateTime when the review is scheduled to end. Required if type is endDate
*/
public val endDate: Output?
get() = javaResource.endDate().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Set of access review history instances for this history definition.
*/
public val instances: Output>?
get() = javaResource.instances().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> accessReviewHistoryInstanceResponseToKotlin(args0) })
})
}).orElse(null)
})
/**
* The interval for recurrence. For a quarterly review, the interval is 3 for type : absoluteMonthly.
*/
public val interval: Output?
get() = javaResource.interval().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The access review history definition unique id.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* The number of times to repeat the access review. Required and must be positive if type is numbered.
*/
public val numberOfOccurrences: Output?
get() = javaResource.numberOfOccurrences().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The identity id
*/
public val principalId: Output
get() = javaResource.principalId().applyValue({ args0 -> args0 })
/**
* The identity display name
*/
public val principalName: Output
get() = javaResource.principalName().applyValue({ args0 -> args0 })
/**
* The identity type : user/servicePrincipal
*/
public val principalType: Output
get() = javaResource.principalType().applyValue({ args0 -> args0 })
/**
* Date time used when selecting review data, all reviews included in data end on or before this date. For use only with one-time/non-recurring reports.
*/
public val reviewHistoryPeriodEndDateTime: Output
get() = javaResource.reviewHistoryPeriodEndDateTime().applyValue({ args0 -> args0 })
/**
* Date time used when selecting review data, all reviews included in data start on or after this date. For use only with one-time/non-recurring reports.
*/
public val reviewHistoryPeriodStartDateTime: Output
get() = javaResource.reviewHistoryPeriodStartDateTime().applyValue({ args0 -> args0 })
/**
* A collection of scopes used when selecting review history data
*/
public val scopes: Output>?
get() = javaResource.scopes().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> accessReviewScopeResponseToKotlin(args0) })
})
}).orElse(null)
})
/**
* The DateTime when the review is scheduled to be start. This could be a date in the future. Required on create.
*/
public val startDate: Output?
get() = javaResource.startDate().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* This read-only field specifies the of the requested review history data. This is either requested, in-progress, done or error.
*/
public val status: Output
get() = javaResource.status().applyValue({ args0 -> args0 })
/**
* The resource type.
*/
public val type: Output
get() = javaResource.type().applyValue({ args0 -> args0 })
/**
* The user principal name(if valid)
*/
public val userPrincipalName: Output
get() = javaResource.userPrincipalName().applyValue({ args0 -> args0 })
}
public object ScopeAccessReviewHistoryDefinitionByIdMapper :
ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.azurenative.authorization.ScopeAccessReviewHistoryDefinitionById::class == javaResource::class
override fun map(javaResource: Resource): ScopeAccessReviewHistoryDefinitionById =
ScopeAccessReviewHistoryDefinitionById(
javaResource as
com.pulumi.azurenative.authorization.ScopeAccessReviewHistoryDefinitionById,
)
}
/**
* @see [ScopeAccessReviewHistoryDefinitionById].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [ScopeAccessReviewHistoryDefinitionById].
*/
public suspend fun scopeAccessReviewHistoryDefinitionById(
name: String,
block: suspend ScopeAccessReviewHistoryDefinitionByIdResourceBuilder.() -> Unit,
): ScopeAccessReviewHistoryDefinitionById {
val builder = ScopeAccessReviewHistoryDefinitionByIdResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [ScopeAccessReviewHistoryDefinitionById].
* @param name The _unique_ name of the resulting resource.
*/
public fun scopeAccessReviewHistoryDefinitionById(name: String): ScopeAccessReviewHistoryDefinitionById {
val builder = ScopeAccessReviewHistoryDefinitionByIdResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy