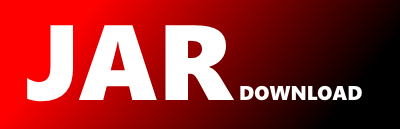
com.pulumi.azurenative.authorization.kotlin.inputs.AccessReviewScopeArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.authorization.kotlin.inputs
import com.pulumi.azurenative.authorization.inputs.AccessReviewScopeArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Descriptor for what needs to be reviewed
* @property excludeResourceId This is used to indicate the resource id(s) to exclude
* @property excludeRoleDefinitionId This is used to indicate the role definition id(s) to exclude
* @property expandNestedMemberships Flag to indicate whether to expand nested memberships or not.
* @property inactiveDuration Duration users are inactive for. The value should be in ISO 8601 format (http://en.wikipedia.org/wiki/ISO_8601#Durations).This code can be used to convert TimeSpan to a valid interval string: XmlConvert.ToString(new TimeSpan(hours, minutes, seconds))
* @property includeAccessBelowResource Flag to indicate whether to expand nested memberships or not.
* @property includeInheritedAccess Flag to indicate whether to expand nested memberships or not.
*/
public data class AccessReviewScopeArgs(
public val excludeResourceId: Output? = null,
public val excludeRoleDefinitionId: Output? = null,
public val expandNestedMemberships: Output? = null,
public val inactiveDuration: Output? = null,
public val includeAccessBelowResource: Output? = null,
public val includeInheritedAccess: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.authorization.inputs.AccessReviewScopeArgs =
com.pulumi.azurenative.authorization.inputs.AccessReviewScopeArgs.builder()
.excludeResourceId(excludeResourceId?.applyValue({ args0 -> args0 }))
.excludeRoleDefinitionId(excludeRoleDefinitionId?.applyValue({ args0 -> args0 }))
.expandNestedMemberships(expandNestedMemberships?.applyValue({ args0 -> args0 }))
.inactiveDuration(inactiveDuration?.applyValue({ args0 -> args0 }))
.includeAccessBelowResource(includeAccessBelowResource?.applyValue({ args0 -> args0 }))
.includeInheritedAccess(includeInheritedAccess?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [AccessReviewScopeArgs].
*/
@PulumiTagMarker
public class AccessReviewScopeArgsBuilder internal constructor() {
private var excludeResourceId: Output? = null
private var excludeRoleDefinitionId: Output? = null
private var expandNestedMemberships: Output? = null
private var inactiveDuration: Output? = null
private var includeAccessBelowResource: Output? = null
private var includeInheritedAccess: Output? = null
/**
* @param value This is used to indicate the resource id(s) to exclude
*/
@JvmName("rmdpyedeqnnviaae")
public suspend fun excludeResourceId(`value`: Output) {
this.excludeResourceId = value
}
/**
* @param value This is used to indicate the role definition id(s) to exclude
*/
@JvmName("kmmmpevfeglymyol")
public suspend fun excludeRoleDefinitionId(`value`: Output) {
this.excludeRoleDefinitionId = value
}
/**
* @param value Flag to indicate whether to expand nested memberships or not.
*/
@JvmName("qkfwywhggplxoulq")
public suspend fun expandNestedMemberships(`value`: Output) {
this.expandNestedMemberships = value
}
/**
* @param value Duration users are inactive for. The value should be in ISO 8601 format (http://en.wikipedia.org/wiki/ISO_8601#Durations).This code can be used to convert TimeSpan to a valid interval string: XmlConvert.ToString(new TimeSpan(hours, minutes, seconds))
*/
@JvmName("etnkrybnfyrvjxcg")
public suspend fun inactiveDuration(`value`: Output) {
this.inactiveDuration = value
}
/**
* @param value Flag to indicate whether to expand nested memberships or not.
*/
@JvmName("ywxacxyrbowvruhl")
public suspend fun includeAccessBelowResource(`value`: Output) {
this.includeAccessBelowResource = value
}
/**
* @param value Flag to indicate whether to expand nested memberships or not.
*/
@JvmName("gwkcxsbrldhwumev")
public suspend fun includeInheritedAccess(`value`: Output) {
this.includeInheritedAccess = value
}
/**
* @param value This is used to indicate the resource id(s) to exclude
*/
@JvmName("bnsjghenspynvicf")
public suspend fun excludeResourceId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.excludeResourceId = mapped
}
/**
* @param value This is used to indicate the role definition id(s) to exclude
*/
@JvmName("xtpbnnhkebwwqyot")
public suspend fun excludeRoleDefinitionId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.excludeRoleDefinitionId = mapped
}
/**
* @param value Flag to indicate whether to expand nested memberships or not.
*/
@JvmName("qadusjboshyapmfd")
public suspend fun expandNestedMemberships(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.expandNestedMemberships = mapped
}
/**
* @param value Duration users are inactive for. The value should be in ISO 8601 format (http://en.wikipedia.org/wiki/ISO_8601#Durations).This code can be used to convert TimeSpan to a valid interval string: XmlConvert.ToString(new TimeSpan(hours, minutes, seconds))
*/
@JvmName("pkvgkhvagnubxslj")
public suspend fun inactiveDuration(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.inactiveDuration = mapped
}
/**
* @param value Flag to indicate whether to expand nested memberships or not.
*/
@JvmName("wbwgjdwusakcjtgd")
public suspend fun includeAccessBelowResource(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.includeAccessBelowResource = mapped
}
/**
* @param value Flag to indicate whether to expand nested memberships or not.
*/
@JvmName("shgthsihyqjnsmpp")
public suspend fun includeInheritedAccess(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.includeInheritedAccess = mapped
}
internal fun build(): AccessReviewScopeArgs = AccessReviewScopeArgs(
excludeResourceId = excludeResourceId,
excludeRoleDefinitionId = excludeRoleDefinitionId,
expandNestedMemberships = expandNestedMemberships,
inactiveDuration = inactiveDuration,
includeAccessBelowResource = includeAccessBelowResource,
includeInheritedAccess = includeInheritedAccess,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy