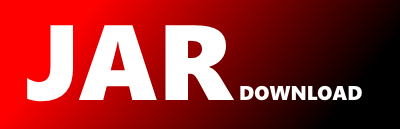
com.pulumi.azurenative.avs.kotlin.inputs.IdentitySourceArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.avs.kotlin.inputs
import com.pulumi.azurenative.avs.inputs.IdentitySourceArgs.builder
import com.pulumi.azurenative.avs.kotlin.enums.SslEnum
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* vCenter Single Sign On Identity Source
* @property alias The domain's NetBIOS name
* @property baseGroupDN The base distinguished name for groups
* @property baseUserDN The base distinguished name for users
* @property domain The domain's dns name
* @property name The name of the identity source
* @property password The password of the Active Directory user with a minimum of read-only access to Base DN for users and groups.
* @property primaryServer Primary server URL
* @property secondaryServer Secondary server URL
* @property ssl Protect LDAP communication using SSL certificate (LDAPS)
* @property username The ID of an Active Directory user with a minimum of read-only access to Base DN for users and group
*/
public data class IdentitySourceArgs(
public val alias: Output? = null,
public val baseGroupDN: Output? = null,
public val baseUserDN: Output? = null,
public val domain: Output? = null,
public val name: Output? = null,
public val password: Output? = null,
public val primaryServer: Output? = null,
public val secondaryServer: Output? = null,
public val ssl: Output>? = null,
public val username: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.avs.inputs.IdentitySourceArgs =
com.pulumi.azurenative.avs.inputs.IdentitySourceArgs.builder()
.alias(alias?.applyValue({ args0 -> args0 }))
.baseGroupDN(baseGroupDN?.applyValue({ args0 -> args0 }))
.baseUserDN(baseUserDN?.applyValue({ args0 -> args0 }))
.domain(domain?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.password(password?.applyValue({ args0 -> args0 }))
.primaryServer(primaryServer?.applyValue({ args0 -> args0 }))
.secondaryServer(secondaryServer?.applyValue({ args0 -> args0 }))
.ssl(
ssl?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.username(username?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [IdentitySourceArgs].
*/
@PulumiTagMarker
public class IdentitySourceArgsBuilder internal constructor() {
private var alias: Output? = null
private var baseGroupDN: Output? = null
private var baseUserDN: Output? = null
private var domain: Output? = null
private var name: Output? = null
private var password: Output? = null
private var primaryServer: Output? = null
private var secondaryServer: Output? = null
private var ssl: Output>? = null
private var username: Output? = null
/**
* @param value The domain's NetBIOS name
*/
@JvmName("wfsnjgrmaiexqdaj")
public suspend fun alias(`value`: Output) {
this.alias = value
}
/**
* @param value The base distinguished name for groups
*/
@JvmName("uawmnulqnggpiagl")
public suspend fun baseGroupDN(`value`: Output) {
this.baseGroupDN = value
}
/**
* @param value The base distinguished name for users
*/
@JvmName("ftieysmbashwycrf")
public suspend fun baseUserDN(`value`: Output) {
this.baseUserDN = value
}
/**
* @param value The domain's dns name
*/
@JvmName("reajqgenpbppvwrx")
public suspend fun domain(`value`: Output) {
this.domain = value
}
/**
* @param value The name of the identity source
*/
@JvmName("ewfupsldkckcpajk")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The password of the Active Directory user with a minimum of read-only access to Base DN for users and groups.
*/
@JvmName("qsnfnwoqldvcnirn")
public suspend fun password(`value`: Output) {
this.password = value
}
/**
* @param value Primary server URL
*/
@JvmName("etetupjdxuimvywp")
public suspend fun primaryServer(`value`: Output) {
this.primaryServer = value
}
/**
* @param value Secondary server URL
*/
@JvmName("qkcixcsbiymifnev")
public suspend fun secondaryServer(`value`: Output) {
this.secondaryServer = value
}
/**
* @param value Protect LDAP communication using SSL certificate (LDAPS)
*/
@JvmName("ryhgayhullwryupq")
public suspend fun ssl(`value`: Output>) {
this.ssl = value
}
/**
* @param value The ID of an Active Directory user with a minimum of read-only access to Base DN for users and group
*/
@JvmName("nrtvmfkfqmewebjt")
public suspend fun username(`value`: Output) {
this.username = value
}
/**
* @param value The domain's NetBIOS name
*/
@JvmName("enkgprkcxywoycbl")
public suspend fun alias(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.alias = mapped
}
/**
* @param value The base distinguished name for groups
*/
@JvmName("kogybpgrlqaglkua")
public suspend fun baseGroupDN(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.baseGroupDN = mapped
}
/**
* @param value The base distinguished name for users
*/
@JvmName("rluhahmobypufmgh")
public suspend fun baseUserDN(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.baseUserDN = mapped
}
/**
* @param value The domain's dns name
*/
@JvmName("onmhrkpfejwiilhs")
public suspend fun domain(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.domain = mapped
}
/**
* @param value The name of the identity source
*/
@JvmName("fmlbvycovsoufksc")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value The password of the Active Directory user with a minimum of read-only access to Base DN for users and groups.
*/
@JvmName("kjkcsmhdjgnqnvyw")
public suspend fun password(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.password = mapped
}
/**
* @param value Primary server URL
*/
@JvmName("ioliwuaekupypdpu")
public suspend fun primaryServer(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.primaryServer = mapped
}
/**
* @param value Secondary server URL
*/
@JvmName("nvnmueuahqabssaj")
public suspend fun secondaryServer(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.secondaryServer = mapped
}
/**
* @param value Protect LDAP communication using SSL certificate (LDAPS)
*/
@JvmName("gayxaoirqrypfowj")
public suspend fun ssl(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ssl = mapped
}
/**
* @param value Protect LDAP communication using SSL certificate (LDAPS)
*/
@JvmName("mgtiqowxmbttmkyq")
public fun ssl(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.ssl = mapped
}
/**
* @param value Protect LDAP communication using SSL certificate (LDAPS)
*/
@JvmName("rlwdwjmyjiuylehm")
public fun ssl(`value`: SslEnum) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.ssl = mapped
}
/**
* @param value The ID of an Active Directory user with a minimum of read-only access to Base DN for users and group
*/
@JvmName("hcebtgpmjxlseogr")
public suspend fun username(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.username = mapped
}
internal fun build(): IdentitySourceArgs = IdentitySourceArgs(
alias = alias,
baseGroupDN = baseGroupDN,
baseUserDN = baseUserDN,
domain = domain,
name = name,
password = password,
primaryServer = primaryServer,
secondaryServer = secondaryServer,
ssl = ssl,
username = username,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy